Automated PNG File Organization
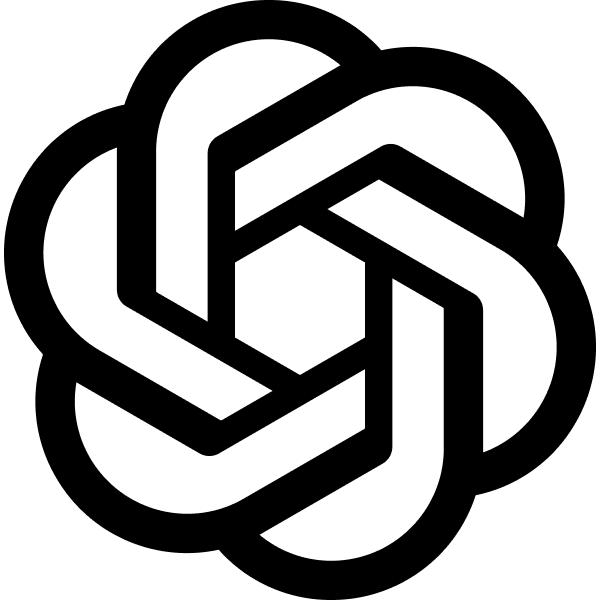
TODO: collapasable, copyable, download, etc
If I have a collection of png files, and I wanted to automatically group them... Here are some ideas, but please be creative and give me more ways to automate this:
look at create timestamp and make a first stab at organnizing them by similar time spans
open the image and analyze the colors to find similar screenshots of similar websitesSure! Organizing and categorizing PNG files can be done in a variety of ways. Building upon your ideas, here are some additional methods:
File Size and Dimensions: Group the images based on their file sizes or dimensions. Images from the same source or of the same type might have similar sizes or resolutions.
Metadata Analysis: Some PNG files may have embedded metadata (like camera model, GPS info, software used to edit, etc.). You can use this to categorize images.
Content Recognition: Utilize machine learning models to recognize the content of images and group them by categories (e.g., landscapes, portraits, animals).
Optical Character Recognition (OCR): If some images contain text, you can use OCR to extract this text and categorize based on the content.
Histogram Analysis: Compare the histograms of images. Images with similar color distributions can be grouped together.
Texture and Pattern Analysis: Some images might have distinct textures or patterns. Algorithms can be used to detect and group by these features.
Image Hashing: Compute perceptual hashes for each image. Images with similar or close hashes might be visually similar.
Folder Path Analysis: Sometimes, the directory path where a file is located can give hints about its category or source.
Filename Patterns: Group images based on certain patterns or keywords in their filenames.
Manual Tagging with AI Assistance: Use AI to suggest possible tags or categories for each image, but have a human verify and make the final decision.
Cluster Analysis: Use unsupervised machine learning to cluster images based on features extracted from them. This will help in grouping similar images even if they don't fit predefined categories.
Remember, the effectiveness of each method might vary based on the nature and diversity of your image collection. Combining multiple methods can also enhance the accuracy of categorization.Can you give me Rust code to perform a histogram analysis?Certainly! Creating a histogram for an image involves counting the number of pixels for each intensity value. If we're dealing with grayscale images, this is straightforward as we'll have values ranging from 0 (black) to 255 (white). For colored images, we typically generate histograms for each of the RGB channels separately.
Below is a basic example of how you can create a histogram for an RGB image using the image
crate in Rust. This code will produce histograms for each of the Red, Green, and Blue channels:
- First, you need to add the required dependencies to your
Cargo.toml
:
[dependencies]
image = "0.23"
- Here's a basic Rust code to generate the RGB histograms:
extern crate image;
use std::fs::File;
use std::path::Path;
use image::{GenericImageView, ImageBuffer, Rgb};
fn main() {
let img_path = Path::new("path_to_your_image.png");
let img = image::open(&img_path).These are full color screenshots of websites. Please design a histogram stragtegy and then output the codeFor full-color screenshots of websites, a histogram analysis can be approached in the following way:
**Histogram Strategy**:
1. **RGB Histograms**: Calculate the histogram for each of the RGB channels. This will give you an idea of the color distribution for red, green, and blue colors in the image.
2. **Luminance Histogram**: Convert the RGB image to a grayscale/luminance representation and then compute its histogram. This gives a sense of the overall brightness distribution in the image.
3. **Normalized Histogram**: After computing the histogram for each channel, normalize it by dividing each bin's value by the total number of pixels. This gives you a probability distribution over the color intensities, which is useful for comparing histograms across images of different sizes.
4. **Cumulative Histogram**: Compute the cumulative histogram, which is the running sum of the bins from start to finish. This is useful for tasks like histogram equalization.
Here's the Rust code for RGB and luminance histogram computation using the `image` crate:
```rust
extern crate image;
use std::fs::File;
use std::path::Path;
use image::{GenericImageView, ImageBuffer, Rgb, Luma};
const BINS: usize = 256;
fn compute_histogram<T: image::Pixel<Subpixel=u8>>(img: &ImageBuffer<T, Vec<u8>>) -> [usize; BINS] {
let mut histogram = [0usize; BINS];
for pixel in img.pixels() {
for &channel_value in pixel.2.channels() {
histogram[channel_value as usize] += 1;
}
}
histogram
}
fn main() {
let img_path = Path::new("path_to_your_image.png");
let img = image::open(&img_path).unwrap();
let red_histogram = compute_histogram::<Rgb<u8>>(&img.to_rgb8());
let green_histogram = compute_histogram::<Rgb<u8>>(&img.to_rgb8());
let blue_histogram = compute_histogram::<Rgb<u8>>(&img.to_rgb8());
let luminance_img = img.to_luma8();
let luminance_histogram = compute_histogram::<Luma<u8>>(&luminance_img);
println!("Red Histogram: {:?}", red_histogram);
println!("Green Histogram: {:?}", green_histogram);
println!("Blue Histogram: {:?}", blue_histogram);
println!("Luminance Histogram: {:?}", luminance_histogram);
}
Remember to add the image
crate to your Cargo.toml
. Adjust the path to your image in the img_path
variable before running the program. This code will give you the histograms for the RGB channels and luminance. Adjust as needed for your specific use case.I got this error ```error[E0609]: no field 2
on type &T
--> src/image_histogram.rs:15:37
|
11 | fn compute_histogram<T: image::Pixel<Subpixel=u8>>(img: &ImageBuffer<T, Vec>) -> [usize; BINS] {
| - type parameter 'T' declared here
...
15 | for &channel_value in pixel.2.channels() {
| ^
The error arises because we are trying to access the pixel values using tuple indexing (`pixel.2`), which is incorrect for this scenario. Instead, we should be accessing the pixel's color channels directly.
Here's the corrected function to compute the histogram:
```rust
fn compute_histogram<T: image::Pixel<Subpixel=u8>>(img: &ImageBuffer<T, Vec<u8>>) -> [usize; BINS] {
let mut histogram = [0usize; BINS];
for (_x, _y, pixel) in img.enumerate_pixels() {
for &channel_value in pixel.channels() {
histogram[channel_value as usize] += 1;
}
}
histogram
}
I've changed for pixel in img.pixels()
to for (_x, _y, pixel) in img.enumerate_pixels()
and removed the .2
indexing from pixel.2.channels()
. This will correctly iterate over the pixel values of the image.
Try this correction and it should resolve the error.Given a histogram for image A and another for image B, how can I calculate a similarity or distance score?To compute similarity or distance between two histograms, several methods can be employed. A few commonly used ones are:
Euclidean Distance (L2 Norm): Calculate the distance between two histograms by summing the square differences between their corresponding bins, and then taking the square root of that sum. Lower values indicate more similarity.
( \text{distance}(A, B) = \sqrt{\sum_{i=1}^{n} (A_i - B_i)^2} )
Manhattan Distance (L1 Norm): Compute the distance by summing the absolute differences between corresponding bins.
( \text{distance}(A, B) = \sum_{i=1}^{n} |A_i - B_i| )
Chi-squared Distance:
( \chi^2(A, B) = \sum_{i} \frac{(A_i - B_i)^2}{(A_i + B_i)} )
Be cautious of bins where the sum of the two histograms is zero, as it'll cause division by zero.
Histogram Intersection: The similarity metric is computed by summing the minimum value for each bin in the two histograms. Higher values indicate more similarity.
( \text{similarity}(A, B) = \sum_{i=1}^{n} \min(A_i, B_i) )
Bhattacharyya distance: This is used especially for measuring the similarity between two probability distributions. It's defined for two normalized histograms A and B:
( \text{distance}(A, B) = -\ln \left( \sum_{i=1}^{n} \sqrt{A_i \times B_i} \right) )
Cosine Similarity: It measures the cosine of the angle between two vectors. A cosine similarity of 1 means that the histograms are identical, and a cosine similarity of 0 means that the histograms are orthogonal or dissimilar.
( \text{similarity}(A, B) = \frac{\sum_{i=1}^{n} A_i \times B_i}{\sqrt{\sum_{i=1}^{n} A_i^2} \times \sqrt{\sum_{i=1}^{n} B_i^2}} )
Here's a Rust function to compute the Euclidean distance between two histograms:
fn euclidean_distance(hist_a: &[usize; BINS], hist_b: &[usize; BINS]) -> f64 {
let sum: f64 = hist_a.iter()
.zip(hist_b.iter())
.map(|(&a, &b)| (a as f64 - b as f64).powi(2))
.sum();
sum.sqrt()
}
You can create similar functions for other distance or similarity measures as needed. When interpreting the values, remember that for distances, smaller values generally indicate more similarity, whereas for similarity metrics, larger values indicate more similarity.
Automated PNG File Organization created at Tue Jan 20 1970 15:24:30 GMT+0000 (Coordinated Universal Time)Related Chats
Automated PNG File Organization 1.000
PhotoCity: 3D Models Game 0.348
Stencil Vector Graphic Program 0.333
Weighted Scoring Model 0.327
SwiftUI App, Centered Image 0.317
Image Carousel with Vanilla JS 0.315
Farm Features & Services 0.294
Data Analysis File Upload 0.286
New chat 0.278