Vite Bootstrap Setup
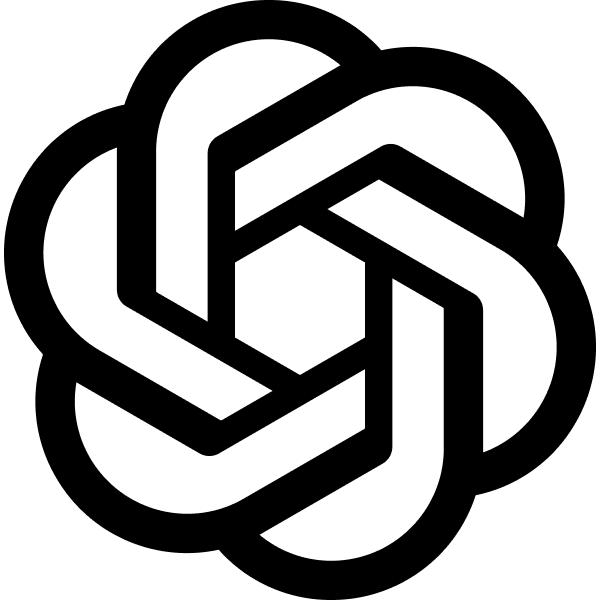
TODO: collapasable, copyable, download, etc
With NodeJs you can do node --require bootstrap.js myserver.js
to run bootstrap code before anything else. Does vite
have a similar capability?Vite, a modern frontend build tool, does offer a way to run some setup or bootstrap code before the rest of your application, similar to Node.js's ability to preload scripts with --require
. In the context of Vite, this can be achieved through its powerful plugin system and through the use of Vite's configuration file.
Here are a few approaches:
Custom Plugin: You can create a custom plugin for Vite where you can execute your bootstrap code. Plugins in Vite are objects that can hook into various stages of the build process or dev server lifecycle. You can use the
configureServer
hook to run code before the server starts.Vite Configuration File: Inside the
vite.config.js
file, you can execute any Node.js code before exporting the configuration. This allows you to run some setup tasks before Vite processes the rest of your application.Using an Entry File: You can create an entry file for your application that runs the bootstrap code first, and then imports the rest of your application. This is a straightforward approach and works well if your bootstrap code is primarily related to the frontend.
Here is an example of how you might use a custom plugin:
// vite.config.js
import { defineConfig } from 'vite';
export default defineConfig({
plugins: [{
name: 'bootstrap-plugin',
configureServer(server) {
// Run your bootstrap code here
console.log('Bootstrap code running before server starts...');
}
}]
});
This script will run your bootstrap code as part of the Vite dev server startup process. It's a flexible approach and can be adapted to various needs.What is the equivilient of annotations for javascriptIn JavaScript, there isn't a direct equivalent to annotations as they are used in languages like Java or Python. Annotations, in those languages, are a form of metadata added to code elements like classes, methods, or variables, often used for providing information to the compiler or runtime.
However, in JavaScript, similar functionality can be achieved through a few different mechanisms:
Comments for Documentation: JavaScript supports comments, which can be used to provide information about the code. Tools like JSDoc utilize these comments to generate documentation and even support types annotations for better editor integrations.
/** * Function to add two numbers * @param {number} a First number * @param {number} b Second number * @returns {number} Sum of a and b */ function add(a, b) { return a + b; }
Decorators (Experimental): Decorators are an experimental feature in JavaScript (as of my last update in April 2023) and are available in TypeScript. They are similar to annotations in other languages and can be used to modify classes, methods, and properties.
// TypeScript example function log(target, key, descriptor) { const original = descriptor.value; descriptor.value = function(...args) { console.log(`Calling ${key} with`, args); return original.apply(this, args); } return descriptor; } class Example { @log sum(a, b) { return a + b; } }
Attribute-like Object Properties: Another way to mimic annotations is to use object properties to store metadata.
function process(obj) { if (obj.metadata && obj.metadata.needsProcessing) { // process obj } } const myObj = { data: {...}, metadata: { needsProcessing: true } };
Using TypeScript: TypeScript, a superset of JavaScript, supports type annotations and decorators more robustly. It's a static type checker for JavaScript, and its features can help in scenarios where annotations would typically be used.
Each of these methods serves a different purpose, and the best choice depends on the specific requirements of your project. Decorators and TypeScript provide the closest functionality to traditional annotations, but they come with the caveat of relying on experimental or additional tooling.Using the OTEL SDK, waht is the best way to call span.setAttribute for an object with many properties and values?When using the OpenTelemetry (OTel) SDK and dealing with a situation where you have an object with many properties that you want to record as attributes in a span, the best approach would often depend on the specifics of your use case and the nature of the data. However, there are a few general strategies you can consider:
Iterate Through Object Properties: If the object has a manageable number of properties, and you want to set each property as a separate attribute on the span, you can iterate through the object's properties and call
span.setAttribute
for each one.const objectWithProperties = { prop1: 'value1', prop2: 'value2', // ... more properties }; for (const [key, value] of Object.entries(objectWithProperties)) { span.setAttribute(key, value); }
Selective Attributes: In case the object has a large number of properties, or if some properties are not relevant for tracing, you might want to selectively set only certain attributes. This can be done by specifying which properties to log or by filtering out unnecessary ones.
const importantProperties = ['prop1', 'prop2']; // properties you want to track importantProperties.forEach(prop => { if (objectWithProperties.hasOwnProperty(prop)) { span.setAttribute(prop, objectWithProperties[prop]); } });
Nested Object as an Attribute: If the object's structure is relevant and you want to preserve it, you could consider setting the entire object as a single attribute. However, this depends on whether the OTel implementation and the backend you're using can handle complex types as attributes.
span.setAttribute('entireObject', JSON.stringify(objectWithProperties));
Custom Serialization: For complex objects, especially those with nested structures or special types, you might need to implement a custom serialization method that converts the object into a format suitable for span attributes (like a JSON string).
function customSerialize(obj) { // Your custom serialization logic return JSON.stringify(obj); // simple example } span.setAttribute('serializedObject', customSerialize(objectWithProperties));
Limiting Attribute Size: Be mindful of the size and number of attributes you are adding to a span. Excessive attributes can increase the overhead of your telemetry data and might be truncated by some backends.
Choose the method that best aligns with your tracing requirements, the nature of your data, and the capabilities of your telemetry backend. For large or complex objects, it's often better to record only the most relevant pieces of information to avoid performance issues and data bloat.The vite test framework when I call .toBeCloseTo
it fails if off by 1. How do I allow it to be off by 1?In JavaScript testing frameworks, the .toBeCloseTo
method is typically used for floating point comparisons, where you can specify the precision of the comparison. However, it sounds like you're using it for integer values and want a tolerance of 1.
If you're using a testing framework like Jest with Vite, .toBeCloseTo
doesn't directly allow for an absolute tolerance like "off by 1". Instead, it's designed to handle floating point precision. For example, expect(3.5).toBeCloseTo(3, 0)
asserts that 3.5
is close to 3
with 0 decimal points precision.
For your case, where you want to allow an integer to be off by 1, you would typically use a different approach. One common method is to use greater than or less than assertions. Here's an example:
expect(yourValue).toBeGreaterThan(expectedValue - 2); // yourValue should be greater than or equal to (expectedValue - 1)
expect(yourValue).toBeLessThan(expectedValue + 2); // and less than or equal to (expectedValue + 1)
This pair of assertions ensures that yourValue
is within 1 of expectedValue
.
Alternatively, if your testing framework or assertion library supports custom matchers or you can use a library that extends the capabilities of your assertions, you might find or write a function that directly supports this kind of "off by one" tolerance. However, using the greater than and less than assertions is a straightforward solution that should work in most cases.What ENV variables are set during a playwrite integration test run?During a Playwright integration test run, the environment variables that are set can vary based on the specifics of your test setup and configuration. Playwright itself doesn't set many environment variables automatically, but it allows you to configure and use environment variables in your tests. Commonly set or used environment variables in a Playwright test environment might include:
Test Configuration Variables: These could be custom variables that you set up in your testing environment to configure the behavior of your tests, like URLs for different test environments, feature flags, etc.
Continuous Integration (CI) Variables: If you are running your Playwright tests in a CI/CD pipeline, the CI environment might set its own variables. These can include build numbers, branch names, commit hashes, etc.
Playwright-Specific Variables: While Playwright itself might not set many environment variables, it does use some environment variables for configuration purposes, like
PLAYWRIGHT_BROWSERS_PATH
to specify where to download browsers.Debugging Variables: Variables like
DEBUG=pw:api
can be set to enable debugging for Playwright API calls.Browser-Specific Variables: If your tests involve specific browser configurations, you might set environment variables that influence the behavior of the browser being automated by Playwright.
Node.js and System Variables: Variables like
NODE_ENV
(often used to specify the environment like 'development' or 'production') or system-specific variables can also be present.Custom Script Variables: If your Playwright tests are initiated by custom scripts, you might have additional environment variables set by these scripts for various purposes.
Remember, environment variables are a way to pass configuration and runtime information to your tests. You can set these variables in your test scripts, CI/CD configuration, or through your operating system's environment variable settings.
To access an environment variable in your Playwright tests, you would typically use process.env.VAR_NAME
in Node.js. For example:
const baseUrl = process.env.BASE_URL || 'http://localhost:3000';
This line would use the BASE_URL
environment variable if it's set, or default to 'http://localhost:3000' if it's not.how does logging when you run in kubernetes usually work? Do you log to different files or just std out?In Kubernetes, the recommended and most common approach for logging is to write log messages to standard output (stdout) and standard error (stderr) streams. This approach is aligned with the twelve-factor app methodology and is particularly suitable for containerized environments like Kubernetes for several reasons:
Simplicity and Convention: Writing to stdout/stderr is straightforward and doesn't require additional logging dependencies or configurations specific to the file system.
Container Logs Management: Kubernetes automatically collects logs from container stdout/stderr streams. These logs are then retrievable using the
kubectl logs
command.Integration with Logging Backends: Kubernetes' logging architecture easily integrates with various log backends and aggregators (like Fluentd, ELK stack - Elasticsearch, Logstash, Kibana, or Splunk). These systems can collect, aggregate, and manage logs more effectively than relying on log files within containers.
Ephemeral Nature of Containers: Containers are generally ephemeral and can be destroyed and recreated frequently. Writing logs to files within containers can lead to log loss unless the files are stored on a persistent volume, which adds complexity.
Sidecar Containers: In some advanced use cases, a sidecar container pattern can be used. This pattern involves running an additional container in the same pod as the main application, where the sidecar container is responsible for log collection, processing, or forwarding.
Standardized Logging Across Multiple Sources: When logs from all containers are streamed to stdout/stderr, it becomes easier to standardize logging across the entire application stack, regardless of the language or logging framework used by individual components.
Scalability and Management: By centralizing log management outside of individual containers, you can scale, query, and manage logs more effectively, especially in a distributed and dynamic environment like Kubernetes.
Implementing Logging in Kubernetes
Application Level: Configure your application to log to stdout/stderr. This can usually be done with minimal configuration in most logging frameworks.
Kubernetes Level: Ensure that your Kubernetes deployment configuration doesn’t redirect these logs to files inside the container.
Cluster Level: Set up a cluster-level logging mechanism that collects and stores logs. This often involves deploying a log aggregator or collector within the cluster, configured to collect logs from the Kubernetes nodes and forward them to a centralized logging solution.
Observability and Analysis: Use tools and services for log analysis and monitoring, which can provide insights and alerting based on log data.
By following these practices, you can create an effective logging strategy in Kubernetes that enhances the observability and reliability of your applications.
Vite Bootstrap Setup created at Tue Jan 20 1970 17:07:49 GMT+0000 (Coordinated Universal Time)Related Chats
Vite Bootstrap Setup 1.000
Read File as Env 0.513
React Framework Alternatives: Blitz, Astro, Redwood. 0.446
Next.js Middleware & HOC 0.442
Logseq Plugin Development Guide 0.430
DB Connection Middleware Setup 0.410
Server-Side Code Placement 0.400
Detecting SvelteKit Environment: Logging 0.388