Update Docker Compose Envvars
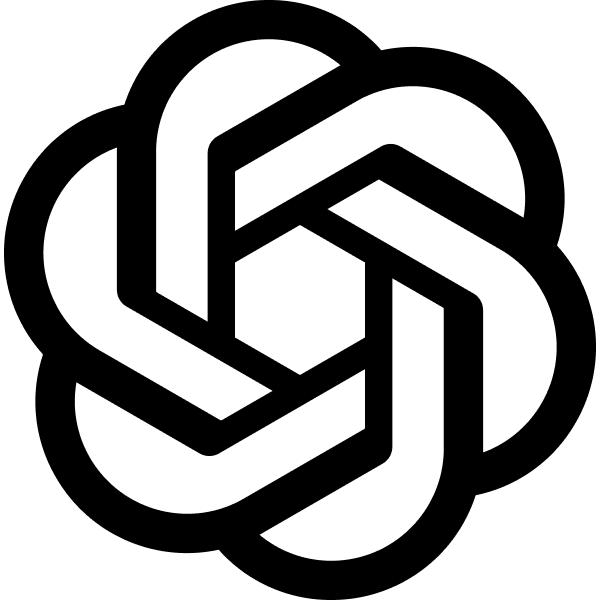
TODO: collapasable, copyable, download, etc
How do I update env variables using docker compose config
?To update environment variables using docker compose config
, you cannot do it directly because docker compose config
is primarily used for validating and viewing the configuration of your docker-compose.yml
file. However, you can use a combination of shell commands and docker compose config
to achieve this. Here's a general approach:
Define Environment Variables in a
.env
File: It's a common practice to define environment variables in a.env
file whichdocker-compose
automatically picks up.Update the
.env
File: You can manually edit this file or use shell commands to update the variables.Use
docker compose config
to Validate: After updating the.env
file, you can rundocker compose config
to ensure that yourdocker-compose.yml
file is correctly configured with the new environment variables.Restart the Services: If your services are already running, you'll need to restart them to pick up the new environment variable values.
Here's an example workflow:
Edit
.env
File:VARIABLE_NAME=new_value
Validate the Configuration:
docker compose config
Restart the Services:
docker compose up -d
This ensures that your services are using the updated environment variables. Remember that any changes to environment variables require a restart of the affected services to take effect.I have a command that I expect to run locally, but it doesn't seem to run. In my Dockerfile it says # Apply schema migrations to mysql-service (except on docker build stage) RUN if [[ -z "${GITHUB_ACTIONS}" ]]; then npx prisma db push; else echo "No prisma migration will apply on Github Actions"; fi
The command you've included in your Dockerfile is designed to conditionally execute based on the presence of an environment variable named GITHUB_ACTIONS
. This setup is typically used to differentiate between running the Docker build process locally and running it in a CI/CD environment like GitHub Actions. Let's break down what the command does:
RUN if [[ -z "${GITHUB_ACTIONS}" ]]; then npx prisma db push; else echo "No prisma migration will apply on Github Actions"; fi
if [[ -z "${GITHUB_ACTIONS}" ]]; then
: This checks if theGITHUB_ACTIONS
environment variable is unset or empty. If it is, the following command is executed.npx prisma db push
: This command is executed when building the Docker image locally (i.e., whenGITHUB_ACTIONS
is not set). It applies schema migrations to themysql-service
.else echo "No prisma migration will apply on Github Actions"
: This is executed ifGITHUB_ACTIONS
is set, indicating that the build process is happening in GitHub Actions. In this case, it simply prints a message and does not run the Prisma migration.fi
: Ends the if statement.
Troubleshooting Steps
Check Environment Variable:
- Ensure that the
GITHUB_ACTIONS
environment variable is not set in your local environment. If it is set, thenpx prisma db push
command will not run.
- Ensure that the
Run the Command Manually:
- Try running
npx prisma db push
manually in your local environment to see if it executes correctly outside of the Docker build process.
- Try running
Build Context:
- Ensure that your Docker build context includes all necessary files, such as
prisma/schema.prisma
and any environment files needed for database connections.
- Ensure that your Docker build context includes all necessary files, such as
Docker Build Output:
- Check the output of the Docker build process. The
RUN
command should either show the execution ofnpx prisma db push
or the echo message, depending on the presence of theGITHUB_ACTIONS
environment variable.
- Check the output of the Docker build process. The
Environment Variables in Docker Build:
- If you need to pass environment variables during the Docker build process, you can use
ARG
in the Dockerfile and pass them with--build-arg
duringdocker build
.
- If you need to pass environment variables during the Docker build process, you can use
If after these checks the command still doesn't run as expected, you might need to review other parts of your Dockerfile or the configuration of your local environment to identify any other issues that might be affecting the build process.I don't see this happening during docker image build or run. When should it execute?The command you're referring to in your Dockerfile should execute during the Docker image build process, not when the container is running. The RUN
instruction in a Dockerfile is executed as part of the build process to create the image. Here's how it works:
During Image Build: When you build your Docker image using a command like
docker build
, eachRUN
command in the Dockerfile is executed in an intermediate container. This is used to modify the filesystem of the image. In your case, thenpx prisma db push
command should be executed at this stage, assuming theGITHUB_ACTIONS
environment variable is not set.Not at Runtime: Once the image is built, the
RUN
commands are not executed again when you start a container from that image. Any command that needs to be executed when a container starts should be in theCMD
orENTRYPOINT
directive.
Why You Might Not See It Executing
Environment Variable Set: If the
GITHUB_ACTIONS
environment variable is set (even if by mistake) in your local environment, thenpx prisma db push
command will not execute.Build Output: The output of the
RUN
command should be visible in the terminal output when you rundocker build
. If you don't see it, the condition might be failing (i.e.,GITHUB_ACTIONS
is set), or there could be an issue with the Docker build process itself.Cached Layers: Docker caches layers from previous builds. If the layer with your
RUN
command was cached from a previous build whereGITHUB_ACTIONS
was set, Docker would not re-run this command. You can try rebuilding the image with the--no-cache
option to ensure all layers are built afresh.docker build --no-cache -t your-image-name .
Error in Command: If there's an error in the
npx prisma db push
command or the surrounding script, it might fail silently. Ensure that your Docker build environment has all the necessary dependencies and configurations.
Checking Execution
To explicitly check if the command is executed, you might temporarily modify the Dockerfile to print something before and after the npx prisma db push
command:
RUN echo "Before prisma push" && \
if [[ -z "${GITHUB_ACTIONS}" ]]; then npx prisma db push; else echo "No prisma migration will apply on Github Actions"; fi && \
echo "After prisma push"
This will help you confirm whether the script is reaching the npx prisma db push
command during the build process.
Related Chats
Env File for Docker 0.667
Deploying Docker Compose EC2 0.635
Docker ENV Variables Not Set 0.592
Config Environment Variables Override 0.529
Shutdown Docker Compose with 'docker-compose down' 0.476
Dockerfile for MySQL 8.0.34 0.476
MySQL Docker User Permissions 0.466
Nohup com env em shell 0.459