Docker ENV Variables Not Set
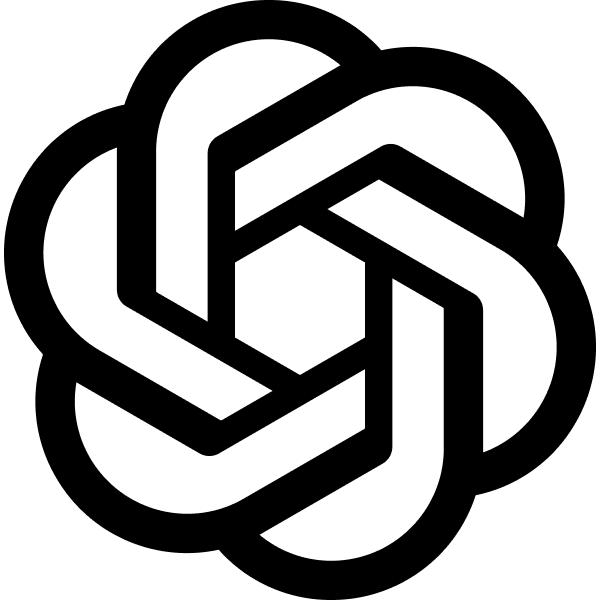
TODO: collapasable, copyable, download, etc
I have a nodejs app in a docker continer. In my Dockerfile I have ENV GOOGLE_CLIENT_ID=532...content.com ENV GOOGLE_OAUTH_SECRET=GO...PqF8Nx
But after the server starts up, process.env doesn't contain these variables. What is going on?If your environment variables set in the Dockerfile are not available in your Node.js application, there could be several reasons for this issue:
Dockerfile Syntax Error: Ensure that the
ENV
instruction is correctly written in your Dockerfile. It should be in the formatENV <key>=<value>
. Check for typos or syntax errors.Docker Build Context: Ensure you are building your Docker image in the correct context where the Dockerfile is located. If the Docker build context is incorrect, it may not be using your intended Dockerfile.
Docker Run: Confirm that you are running the correct Docker image which has the environment variables set.
Overriding Environment Variables: Check if the environment variables are being overridden somewhere else in your Dockerfile or during the Docker run command. Sometimes environment variables can be set or overridden in
docker-compose.yml
files or in the command line using the-e
flag withdocker run
.Code Accessing Environment Variables: Verify that the code in your Node.js application is correctly accessing the environment variables. In Node.js, environment variables can be accessed with
process.env.VARIABLE_NAME
.Order of Instructions in Dockerfile: Make sure that the
ENV
instructions are placed before any command that might require these environment variables. If you set them after commands that start your application, they won't be available to the application.Layer Caching Issues: Sometimes, Docker's layer caching can cause issues. Try rebuilding the Docker image with no cache using the
--no-cache
option in thedocker build
command.Multi-Stage Builds: If you are using a multi-stage build, ensure that the environment variables are set in the correct stage.
You can debug this by adding a command like RUN printenv
in your Dockerfile after setting the environment variables to see if they are correctly set during the build process. Also, consider logging the environment variables at the start of your Node.js application to check their availability.In NodeJS, what does the *
mean in this snippet function* (x) { for (let i = x; i > 0; --i) { yield i; } return 0; },
In Node.js, the *
symbol in function*
indicates that the function is a generator function. Generator functions are a special type of function that can pause execution and resume it later, allowing them to produce a sequence of results over time rather than computing them all at once.
Here's a breakdown of how generator functions and the yield
keyword work:
Generator Function: When you define a function with
function*
, you are creating a generator function. This function does not execute its code when called but instead returns a special type of iterator, known as a generator.yield
Keyword: Inside a generator function, you can use theyield
keyword to pause the function's execution and return a value. The function's state is saved at this point, and it can be resumed later.Iterating Over Generators: The returned generator can be iterated over using a
for...of
loop or manually by calling itsnext()
method. Each call tonext()
resumes the function's execution from where it was last paused withyield
.Example Explained: In your provided example, the generator function takes an argument
x
and then enters a loop. In each iteration, it yields the current value ofi
(starting fromx
and decreasing by 1 each time). Oncei
reaches 0, the loop ends, and the function returns 0. This return value signifies the end of the iteration.
Here's a brief example of how you might use this generator:
const countdown = function* (x) {
for (let i = x; i > 0; --i) {
yield i;
}
return 0;
};
const gen = countdown(5);
for (let value of gen) {
console.log(value); // Outputs: 5, 4, 3, 2, 1, 0
}
In this example, each time the for...of
loop requests the next value, the generator resumes, yields the next value, and then pauses again. This process continues until the generator function completes, providing a powerful way to handle sequences of data without computing them all at once.How can I turn this into a geneator function that takes a data
parameter? let dataTypes = fromInfer(data); dataTypes?.forEach((datum) => controller.enqueue(encoder.encode(JSON.stringify(datum))) );
To convert the given code snippet into a generator function that takes a data
parameter, you'll need to make a few adjustments. First, let's analyze what the original code does:
- It calls
fromInfer(data)
to presumably infer data types or process thedata
in some way. - It iterates over the result of
fromInfer(data)
, which isdataTypes
. - For each
datum
indataTypes
, it enqueues an encoded JSON string into acontroller
.
Now, to turn this into a generator function, you would do the following:
- Define the function with
function*
. - Instead of calling
controller.enqueue
, useyield
to produce each encoded datum. - The generator will yield each encoded JSON string, which can be iterated over or used as needed.
Here's how the code might look:
function* generateEncodedData(data) {
let dataTypes = fromInfer(data);
if (dataTypes) {
for (let datum of dataTypes) {
yield encoder.encode(JSON.stringify(datum));
}
}
}
// Example usage:
const data = /* your data */;
for (let encodedDatum of generateEncodedData(data)) {
controller.enqueue(encodedDatum);
}
In this version:
generateEncodedData
is a generator function that takesdata
as its parameter.- It processes
data
withfromInfer
and iterates over the result. - For each
datum
, it yields an encoded JSON string. - You can then use this generator in a
for...of
loop (or another iteration method) to enqueue each item to the controller as before.
This approach makes the function more flexible and reusable, as the encoding and generation of data are now separated from the specific action of enqueuing it to the controller.Why did you switch forEach
to for of
?Switching from forEach
to a for...of
loop in the context of a generator function is primarily due to how generator functions and their iterators work.
Compatibility with
yield
: The key feature of a generator function is its ability toyield
values. Theyield
keyword can only be used directly inside the generator function's body.forEach
, being a higher-order function, takes a callback function, and you cannot useyield
within this callback in a way that it affects the generator's flow. Theyield
keyword needs to be in the direct body of the generator function to pause and resume its execution and produce values.Iteration Control: The
for...of
loop is inherently compatible with iterators, which is what generator functions return. It allows for a seamless and natural way to iterate over the values being yielded by the generator. Each iteration of thefor...of
loop receives a value yielded by the generator until the generator is done.Simplicity and Readability: The
for...of
loop tends to be more straightforward when used with generator functions. It clearly indicates that the code is designed to handle an iterable sequence of values, making it more readable and maintainable, especially in the context of generators.
In summary, using for...of
with a generator function is a natural fit due to its direct compatibility with the iteration protocol and the ability to use yield
effectively.
Related Chats
Env File for Docker 0.599
Update Docker Compose Envvars 0.592
Nohup com env em shell 0.574
Deploying Docker Compose EC2 0.491
Read File as Env 0.486
Config Environment Variables Override 0.473
Dockerfile for PostgreSQL 16.1 0.418