SQLite table creation.
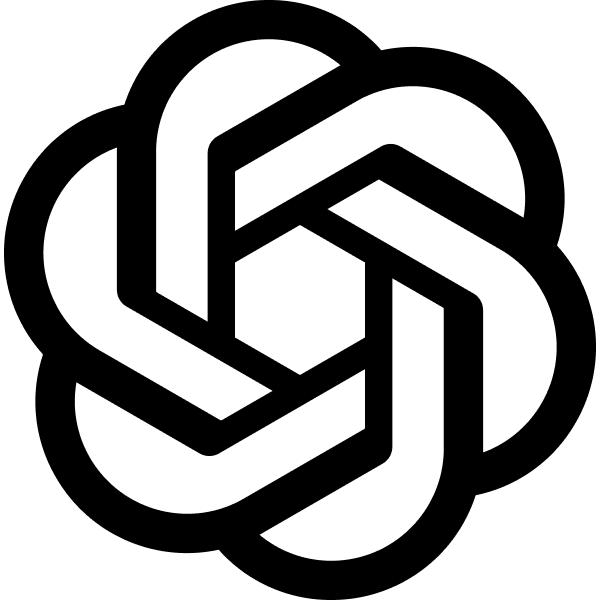
TODO: collapasable, copyable, download, etc
Please create the SQL to create a SQlite table called prices with an id, a float for amount, and a string for frequency.To create a SQLite table called 'prices' with the specified columns, you can use the following SQL statement:
CREATE TABLE prices (
id INTEGER PRIMARY KEY AUTOINCREMENT,
amount REAL,
frequency TEXT
);
This statement creates a table with three columns:
- 'id': An integer column that serves as the primary key and will auto-increment for each new row added to the table.
- 'amount': A REAL column to store the float values for the amount.
- 'frequency': A TEXT column to store the string values for the frequency.What is the difference between REAL and Sqlite's DOUBLE? Are they the same?In SQLite, REAL and DOUBLE are essentially the same. Both types are used to store floating-point numbers. According to the SQLite documentation, the terms REAL, DOUBLE, and DOUBLE PRECISION are interchangeable. All of these types use 8 bytes of storage and can represent floating-point numbers in the IEEE 754 standard for double-precision floating-point numbers.
Here's the relevant excerpt from the SQLite documentation:
"SQLite does not have a storage class set aside for storing dates and/or times. Instead, the built-in Date And Time Functions of SQLite are capable of storing dates and times as TEXT, REAL, or INTEGER values:
- TEXT as ISO8601 strings ("YYYY-MM-DD HH:MM:SS.SSS").
- REAL as Julian day numbers, the number of days since noon in Greenwich on November 24, 4714 B.C. according to the proleptic Gregorian calendar.
- INTEGER as Unix Time, the number of seconds since 1970-01-01 00:00:00 UTC.
Applications can chose to store dates and times in any of these formats and freely convert between formats using the built-in date and time functions."
So, when working with SQLite, you can use REAL or DOUBLE to store floating-point numbers, and they will behave the same way.I ran the following as a migration and it failed with the output near ")": syntax error
. What is wrong?
CREATE TABLE prices ( id INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL, amount DOUBLE NOT NULL, frequency TEXT NOT NULL, );The syntax error in your SQL statement is caused by the trailing comma after the last column definition. SQLite expects another column definition after the comma, but since there isn't one, it throws a syntax error. To fix the issue, simply remove the comma after the last column definition:
CREATE TABLE prices (
id INTEGER PRIMARY KEY AUTOINCREMENT NOT NULL,
amount DOUBLE NOT NULL,
frequency TEXT NOT NULL
);
Now, your CREATE TABLE statement should execute without any syntax errors.I have a SQLite table CREATE TABLE shopify ( url TEXT NOT NULL, crawl_day TEXT NOT NULL, name TEXT NOT NULL, description TEXT NOT NULL, rating DOUBLE NOT NULL, ratings_count INTEGER NOT NULL, PRIMARY KEY (url, crawl_day) )
What is the SQLite SQL to alert the table? I need to change the PRIMARY KEY to a UNIQUE index and I need to add an auto incrementing id.To alter the table and make the desired changes, you need to follow these steps:
- Create a new table with the desired schema.
- Copy data from the existing table to the new table.
- Drop the original table.
- Rename the new table to the original table name.
Here's the SQL to achieve this:
-- Step 1: Create a new table with the desired schema
CREATE TABLE shopify_new (
id INTEGER PRIMARY KEY AUTOINCREMENT,
url TEXT NOT NULL,
crawl_day TEXT NOT NULL,
name TEXT NOT NULL,
description TEXT NOT NULL,
rating DOUBLE NOT NULL,
ratings_count INTEGER NOT NULL
);
-- Step 2: Copy data from the existing table to the new table
INSERT INTO shopify_new (url, crawl_day, name, description, rating, ratings_count)
SELECT url, crawl_day, name, description, rating, ratings_count
FROM shopify;
-- Step 3: Drop the original table
DROP TABLE shopify;
-- Step 4: Rename the new table to the original table name
ALTER TABLE shopify_new RENAME TO shopify;
-- Step 5: Add a UNIQUE index on url and crawl_day
CREATE UNIQUE INDEX shopify_url_crawl_day_idx ON shopify(url, crawl_day);
These steps will create a new table with an auto-incrementing id as the primary key, copy the data from the original table to the new table, drop the original table, rename the new table to the original table name, and finally, create a UNIQUE index on the url
and crawl_day
columns.
Related Chats
Dumping SQLite Data to CSV 0.309
RRD Data Storage Mechanism 0.294
95th percentile explained. 0.257
Data Analysis File Upload 0.247
Tenfold Growth Rule Formula 0.227
Weighted Sampling in Swift 0.222
10,000 ≠ 2% of 2M 0.221