Dumping SQLite Data to CSV
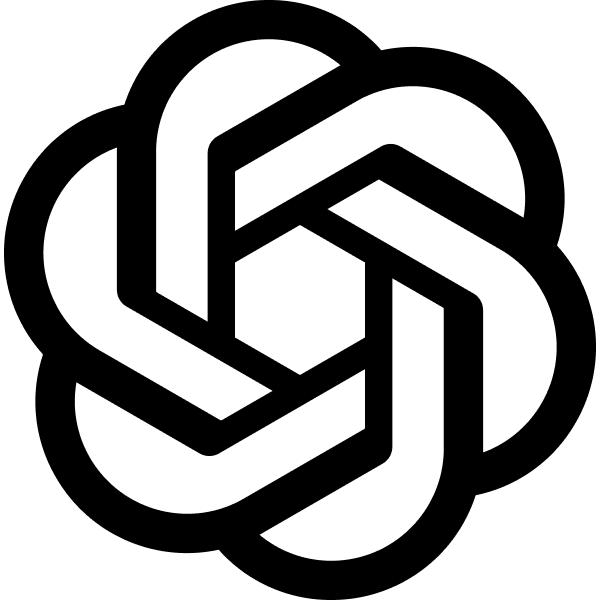
TODO: collapasable, copyable, download, etc
I have a sqlite file. How can I dump all of the data into a series of csv files?Sure, I can guide you through the process. Here are the general steps:
- First, we need to read the SQLite file.
- Then, we can extract the table names from the SQLite file.
- For each table, we can convert the data into a pandas DataFrame.
- Finally, we can save each DataFrame as a CSV file.
To begin with, could you please upload your SQLite file?import sqlite3 import pandas as pd
Connect to SQLite database
conn = sqlite3.connect('/mnt/data/stan.db')
Get the table names
table_names_query = "SELECT name FROM sqlite_master WHERE type='table';" table_names = pd.read_sql_query(table_names_query, conn)
table_names name 0 __diesel_schema_migrations 1 vercel 2 sqlite_sequence 3 shopify 4 prices 5 app_pricesThe tables in your SQLite database are:
__diesel_schema_migrations
vercel
sqlite_sequence
shopify
prices
app_prices
Now, let's extract the data from each table and save it to a corresponding CSV file. We'll save these files in the same directory as your SQLite database.# For each table, convert to DataFrame and save as CSV for table_name in table_names['name']: df = pd.read_sql_query(f"SELECT * FROM {table_name}", conn) df.to_csv(f"/mnt/data/{table_name}.csv", index=False)I have successfully exported the data from each table in your SQLite database to separate CSV files. Here are the links to download them:
Click on each link to download the corresponding file. Let me know if you need any further assistance.If you analyze the shopify data, what are 10 insights?# Let's load the shopify data into a DataFrame df_shopify = pd.read_sql_query("SELECT * FROM shopify", conn)
And let's take a quick look at the first few rows and its structure
df_shopify.info() df_shopify.head()<class 'pandas.core.frame.DataFrame'> RangeIndex: 37872 entries, 0 to 37871 Data columns (total 7 columns):
Column Non-Null Count Dtype
0 id 37872 non-null int64
1 url 37872 non-null object
2 crawl_day 37872 non-null object
3 name 37872 non-null object
4 description 37872 non-null object
5 rating 37872 non-null float64
6 ratings_count 37872 non-null int64
dtypes: float64(1), int64(2), object(4)
memory usage: 2.0+ MB
id url crawl_day
0 7935 https://apps.shopify.com/zigpoll 2023-03-06
1 7936 https://apps.shopify.com/tiktok 2023-03-06
2 7937 https://apps.shopify.com/dsers 2023-03-06
3 7938 https://apps.shopify.com/printful 2023-03-06
4 7939 https://apps.shopify.com/instafeed 2023-03-06
name description rating \
0 Zigpoll Customer Surveys Zigpoll Customer Surveys 5.0
1 TikTok TikTok 4.6
2 DSers‑AliExpress Dropshipping DSers‑AliExpress Dropshipping 5.0
3 Printful: Print on Demand Printful: Print on Demand 4.6
4 Instafeed ‑ Instagram Feed Instafeed ‑ Instagram Feed 4.9
ratings_count
0 133
1 1263
2 13710
3 4291
4 1241 The "shopify" dataset has 37,872 entries and 7 columns, namely:
- 'id': A unique identifier for each entry.
- 'url': The URL of the app on Shopify.
- 'crawl_day': The day the data was crawled.
- 'name': The name of the app.
- 'description': The description of the app.
- 'rating': The rating of the app.
- 'ratings_count': The number of ratings the app has received.
Before we proceed with the insights, I'd like to clarify: insights can be very broad and depend on the questions we are interested in. For instance, we could explore trends in ratings, the relationship between ratings and the number of reviews, the most common words in app descriptions, and many others. Could you please specify what kind of insights you're interested in? For example, are you interested in insights about the ratings, the number of ratings, the descriptions, or anything else?The data is from the Shopify apps marketplace. I am a software developer looking for the best opportunties to create a new Shopify app and make money selling that plugin
Dumping SQLite Data to CSV created at Tue Jan 20 1970 11:59:48 GMT+0000 (Coordinated Universal Time)Related Chats
Dumping SQLite Data to CSV 0.999
New chat 0.549
Data Analysis File Upload 0.451
SQLite table creation. 0.309
Exporting Markdown with Plugins 0.226
Prisma Setup and Usage 0.222
SQL for PostgreSQL Database 0.214
HTML Data Scraping. 0.213