GitHub Actions: Deploy on Change
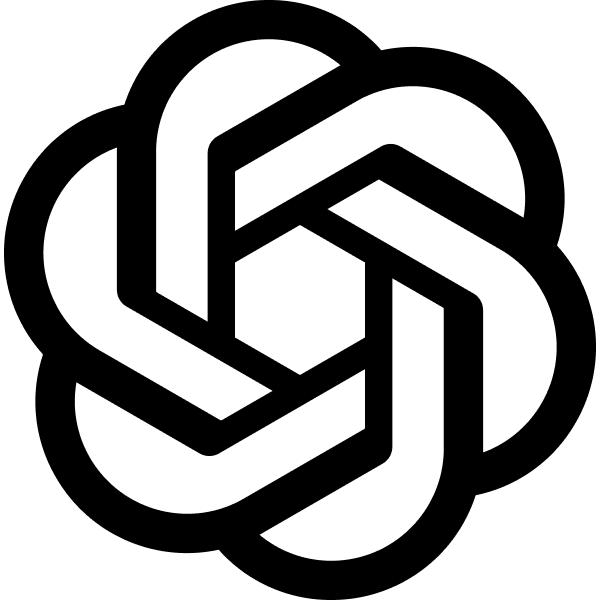
TODO: collapasable, copyable, download, etc
how would you configure github actions to deploy only if a file in a certain sub-directory had changed?To configure GitHub Actions to deploy only if a file in a certain sub-directory has changed, you can use a workflow with a combination of the on
and paths
filters. Here's a step-by-step guide on how to set it up:
Create a Workflow File: If you don't already have a GitHub Actions workflow file, create one in your repository under the
.github/workflows
directory. You can name it something likedeploy.yml
.Define the Workflow:
name: Deploy on File Change on: push: paths: - 'subdirectory/**' # Specify the path to the sub-directory you want to watch for changes
In the
on
section, we use thepush
event, and thepaths
filter is set to the sub-directory you want to monitor. Replace'subdirectory/**'
with the actual path to your sub-directory.Specify Workflow Jobs and Steps:
Add the necessary jobs and steps to your workflow YAML file. This can vary depending on your specific deployment needs, such as deploying to a hosting platform like AWS, Azure, or GitHub Pages. Here's an example of a basic workflow that deploys to GitHub Pages:
jobs: deploy: runs-on: ubuntu-latest steps: - name: Checkout Code uses: actions/checkout@v2 # Add additional steps for building and deploying your project. # For GitHub Pages, you might use a build script and the `gh-pages` branch. - name: Deploy to GitHub Pages if: github.ref == 'refs/heads/main' # Only deploy from the main branch uses: peaceiris/actions-gh-pages@v4 with: github_token: ${{ secrets.GITHUB_TOKEN }} publish_dir: ./subdirectory # Specify the path to your sub-directory
Customize the steps inside the
deploy
job according to your deployment requirements. In this example, it deploys to GitHub Pages if changes are pushed to themain
branch and the files in the specified sub-directory have changed.Commit and Push the Workflow File:
Commit the workflow file and push it to your GitHub repository.
Now, the workflow will only trigger and deploy when changes are pushed to the specified sub-directory. Make sure to adjust the workflow and deployment steps to match your specific project and deployment platform.I have a docker container. In the Dockerfile I have the line RUN npx prisma migrate dev
.
I have a github actions config. In that config, I don't want that line to execute. How can I make that conditional and what is the config change in both of those files?To make the execution of the RUN npx prisma migrate dev
command in your Dockerfile conditional based on GitHub Actions configuration, you can use environment variables. You can set an environment variable in your GitHub Actions workflow and then check its value in your Dockerfile to determine whether to execute the command. Here's how you can do it:
GitHub Actions Workflow:
In your GitHub Actions workflow, set an environment variable using the env
keyword. You can set it to a specific value when you want the command to execute and leave it unset or set it to a different value when you want to skip the command.
name: Build and Deploy
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
# Set the environment variable to control the Dockerfile behavior.
- name: Set Prisma Migration Variable
env:
RUN_PRISMA_MIGRATE: 'true' # Set this to 'true' to enable the command
run: echo "RUN_PRISMA_MIGRATE=true" >> $GITHUB_ENV
- name: Build Docker Image
run: docker build -t my-image .
# Add steps for other actions and deployments as needed.
In this example, we set the RUN_PRISMA_MIGRATE
environment variable to 'true'
to enable the command. You can change this value as needed to control whether the Prisma migration command is executed.
Dockerfile:
In your Dockerfile, you can use a conditional statement to check the value of the environment variable and conditionally run the npx prisma migrate dev
command.
# ... other Dockerfile instructions ...
# Check the value of the environment variable before running Prisma migration.
RUN if [ "$RUN_PRISMA_MIGRATE" = "true" ]; then npx prisma migrate dev; fi
# ... other Dockerfile instructions ...
Now, the npx prisma migrate dev
command in your Dockerfile will only execute if the RUN_PRISMA_MIGRATE
environment variable is set to 'true'
in your GitHub Actions workflow. If it's not set or set to a different value, the command will be skipped during the Docker image build.I want the opposite. I want it to always run, unless $SKIP_PRISMA_MIGRATE
is presentIf you want the npx prisma migrate dev
command in your Dockerfile to always run unless the $SKIP_PRISMA_MIGRATE
environment variable is present, you can modify the Dockerfile like this:
Dockerfile:
# ... other Dockerfile instructions ...
# Check if the SKIP_PRISMA_MIGRATE environment variable is NOT present before running Prisma migration.
RUN if [ -z "$SKIP_PRISMA_MIGRATE" ]; then npx prisma migrate dev; fi
# ... other Dockerfile instructions ...
With this Dockerfile modification, the npx prisma migrate dev
command will always run during the Docker image build unless the $SKIP_PRISMA_MIGRATE
environment variable is present. If you set the variable in your GitHub Actions workflow, it will skip the Prisma migration.
In your GitHub Actions workflow, make sure to set the $SKIP_PRISMA_MIGRATE
environment variable only when you want to skip the migration step. Here's how you can do that:
GitHub Actions Workflow:
name: Build and Deploy
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout Code
uses: actions/checkout@v2
# Set the environment variable to skip Prisma migration if needed.
- name: Set Skip Prisma Migration Variable
env:
SKIP_PRISMA_MIGRATE: 'true' # Set this to 'true' to skip Prisma migration
run: echo "SKIP_PRISMA_MIGRATE=true" >> $GITHUB_ENV
- name: Build Docker Image
run: docker build -t my-image .
# Add steps for other actions and deployments as needed.
In this workflow, the SKIP_PRISMA_MIGRATE
environment variable is set to 'true'
to skip Prisma migration when needed. If the variable is not set, the migration will run during the Docker image build.In Dockerfile, can I detect that I'm executing in a Github Actions environment and then not run the command?Yes, you can detect that you're executing in a GitHub Actions environment in your Dockerfile and conditionally choose whether or not to run a command. GitHub Actions provides environment variables that you can check within your Dockerfile to determine if the code is running in a GitHub Actions workflow.
Here's how you can conditionally run a command based on whether your code is running in a GitHub Actions environment or not in your Dockerfile:
# ... other Dockerfile instructions ...
# Check if we are running in a GitHub Actions environment.
# GitHub Actions sets the CI environment variable to "true."
# If CI is true, then we are in a GitHub Actions environment.
RUN if [ "$CI" = "true" ]; then \
echo "Running in GitHub Actions environment"; \
else \
npx prisma migrate dev; \
fi
# ... other Dockerfile instructions ...
In this Dockerfile snippet, we're checking the value of the CI
environment variable. GitHub Actions automatically sets CI
to "true" when running workflows. If CI
is "true," it means you are in a GitHub Actions environment, and we echo a message indicating that. If CI
is not "true," it means you are not in a GitHub Actions environment, and we run the npx prisma migrate dev
command.
This approach allows you to conditionally run the command only when your Dockerfile is executed in a GitHub Actions workflow and skip it in other environments.
GitHub Actions: Deploy on Change created at Tue Jan 20 1970 15:37:22 GMT+0000 (Coordinated Universal Time)Related Chats
CI Workflow for Long-Lived Branch 0.514
Monitor Specific Directories 0.439
Fix GitHub Pull Request 0.406
Git Diff: Compare Branches 0.403
Syncing Git Submodules Correctly 0.389
Submodule Changes in PR 0.386
Git Diff After Rebase 0.347
Fixing PRs after rebasing 0.326
New chat 0.324