Material Error Correction
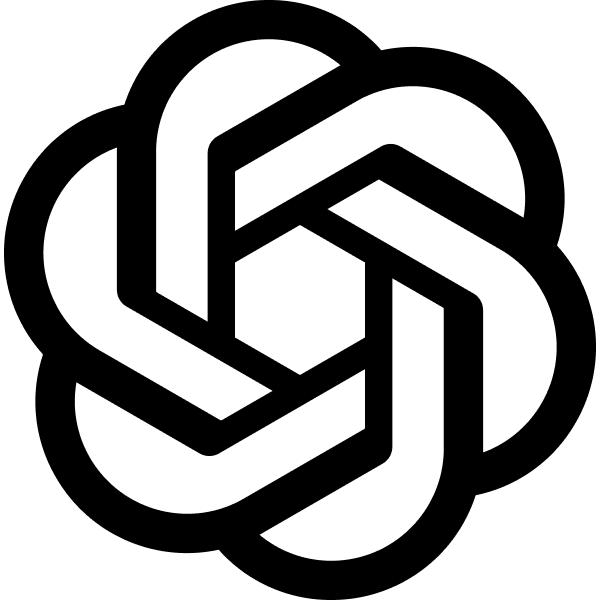
TODO: collapasable, copyable, download, etc
In RealityKit this code ```// // GraphChartCubeModel.swift // RetirementCalculator // // Created by Austin King on 2/9/24. //
import SwiftUI import RealityKit
@MainActor class GraphChartCubeModel: ObservableObject { private var contentEntity = Entity()
func setContentEntity() -> Entity {
return contentEntity
}
func addCube() {
guard
let blueprintTexture = try? TextureResource.load(named: "blueprint")
else {
fatalError("Unable to load blueprint texture")
}
let entity = Entity()
var material = SimpleMaterial()
material.color = .init(texture: .init(blueprintTexture))
/*
let boxMesh = MeshResource.generateBox(width: 0.1, height: 6, depth: 6) // .generateBox(size: 0.1)
let material = SimpleMaterial(color: .green, isMetallic: false)
let boxModel = ModelEntity(mesh: boxMesh, materials: [material])
boxModel.transform.translation += SIMD3<Float>(0.4, 0.1, 0)
*/
/*entity.components.set(ModelComponent(
mesh: .generateBox(width: 0.1, height: 6, depth: 6),
materials: [blueprintTexture]
))*/
entity.components.set(ModelComponent(
mesh: .generateBox(width: 0.5, height: 0.5, depth: 0.5, splitFaces: true),
materials: [blueprintTexture, blueprintTexture, blueprintTexture, blueprintTexture, blueprintTexture, blueprintTexture])
)
entity.position = SIMD3(x: 0, y: 1, z: -2)
contentEntity.addChild(entity)
}
}
#Preview { RetirementCalculatorContainer() .previewLayout(.sizeThatFits) }
on line 44 entity.components.set is giving me the error "Type of expression is ambiguous without a type annotation"The error "Type of expression is ambiguous without a type annotation" indicates that the Swift compiler is unable to infer the type of the expression on line 44. This is typically because the call is too complex or because there's an overload that the compiler can't resolve without more information.
Looking at your code, the issue seems to be with the `materials` parameter in the `.set` method call for `ModelComponent`. You are trying to pass an array of `TextureResource` directly to `materials`, but `materials` expects an array of `Material` objects.
However, it seems there was a mistake in how the `materials` array is being constructed. Instead of passing `[blueprintTexture, blueprintTexture, ...]` (which are `TextureResource` objects), you should be creating `SimpleMaterial` objects using these textures and then passing an array of these `SimpleMaterial` objects to the `materials` parameter.
Here's a corrected snippet for creating a `ModelComponent` with materials correctly applied:
```swift
let material = SimpleMaterial(texture: .init(textureResource: blueprintTexture), isMetallic: false)
entity.components.set(ModelComponent(
mesh: .generateBox(width: 0.5, height: 0.5, depth: 0.5, splitFaces: true),
materials: [material, material, material, material, material, material]
))
Note: The SimpleMaterial
initializer used here assumes that you want to use the same SimpleMaterial
configuration (with the blueprint texture applied) for all faces of the box. If you want different materials for different faces, you'll need to create separate SimpleMaterial
instances for each and configure them as needed.
Ensure the SimpleMaterial
initialization correctly references the texture, as the original attempt to set .color
with a texture was not the correct approach. The SimpleMaterial
initializer that takes a texture resource directly should be used instead.After fixing an error, I have two remaining errors:
- Cannot infer contextual base in reference to member 'init'
- Extra argument 'texture' in callThe errors you're encountering now are related to the incorrect usage of the
SimpleMaterial
initializer. Based on the errors:
Cannot infer contextual base in reference to member 'init': This error suggests that Swift cannot determine the correct type or constructor because the usage context does not provide enough information.
Extra argument 'texture' in call: This suggests that you're providing an argument to a function or initializer that does not accept it in its definition.
Given these errors, it seems there was confusion in my initial guidance regarding the SimpleMaterial
initializer. The SimpleMaterial
does not have an initializer that directly takes a TextureResource
as an argument in the manner shown. Instead, you should create a MaterialColorParameter
using the texture, and then pass that to the SimpleMaterial
initializer. Here's how to correct this:
do {
let blueprintTexture = try TextureResource.load(named: "blueprint")
let material = SimpleMaterial(color: MaterialColorParameter.texture(blueprintTexture), isMetallic: false)
entity.components.set(ModelComponent(
mesh: .generateBox(width: 0.5, height: 0.5, depth: 0.5, splitFaces: true),
materials: [material, material, material, material, material, material]
))
} catch {
fatalError("Failed to load texture: \(error)")
}
In this corrected code snippet:
- I've encapsulated the
TextureResource.load
call within ado-catch
block to handle potential errors, which is a safer way to load resources that might fail. - The
SimpleMaterial
is initialized with acolor
parameter that usesMaterialColorParameter.texture(blueprintTexture)
to correctly apply the texture to the material. This is the correct way to create a material with a texture in RealityKit.
This approach should resolve the errors you're encountering and correctly apply the texture to the materials for the cube.Cannot convert value of type 'MaterialColorParameter' to expected argument type 'SimpleMaterial.Color' (aka 'UIColor')The error message you're seeing, "Cannot convert value of type 'MaterialColorParameter' to expected argument type 'SimpleMaterial.Color' (aka 'UIColor')", indicates a misunderstanding in the way textures are used with materials in RealityKit. My previous instructions were incorrect in suggesting you can directly use a MaterialColorParameter
with SimpleMaterial
's color property, which expects a color type, not a texture.
In RealityKit, to apply a texture to a SimpleMaterial
, you should use the baseColor
property of the material's materialProperties
. However, this direct approach is more applicable to custom materials or when using ModelEntity
with ModelComponent
that can take an array of Material
.
Since SimpleMaterial
does not provide a direct initializer that takes a TextureResource
for the base color in the version you are likely using, you'd typically work with the texture in a different manner. Unfortunately, my previous advice was misleading in this context.
For applying a texture to a material in RealityKit, the correct approach would involve creating a material that supports texture application. RealityKit's SimpleMaterial
can take a texture, but it needs to be set correctly:
do {
let blueprintTexture = try TextureResource.load(named: "blueprint")
var material = SimpleMaterial()
material.baseColor = MaterialColorParameter.texture(blueprintTexture)
let entity = ModelEntity(mesh: .generateBox(width: 0.5, height: 0.5, depth: 0.5, cornerRadius: 0.1), materials: [material])
entity.position = SIMD3(x: 0, y: 1, z: -2)
contentEntity.addChild(entity)
} catch {
fatalError("Unable to load blueprint texture")
}
In this corrected version:
- We create a
SimpleMaterial
and then directly set itsbaseColor
to aMaterialColorParameter.texture
with the loadedTextureResource
. - Then, this material is used to create a
ModelEntity
with the specified mesh and material.
This approach should align with RealityKit's API for applying textures to materials, avoiding the type mismatch error and accurately reflecting how textures are intended to be used with materials in RealityKit.
Material Error Correction created at Tue Jan 20 1970 18:18:09 GMT+0000 (Coordinated Universal Time)Related Chats
Material Error Correction 0.999
Code Structure Improvements 0.482
Provide EnvironmentObject TrainerView 0.392
Generate Sphere in AR. 0.374
Frame Photos with SwiftUI 0.370
SwiftUI Drag Rotation Implementation 0.355
Knuckle Position Synchronization 0.347
Convert Entity to ModelEntity 0.344
Use Promise.allSettled refactor code 0.343
Add Function to SwiftUI. 0.321