Use Promise.allSettled refactor code
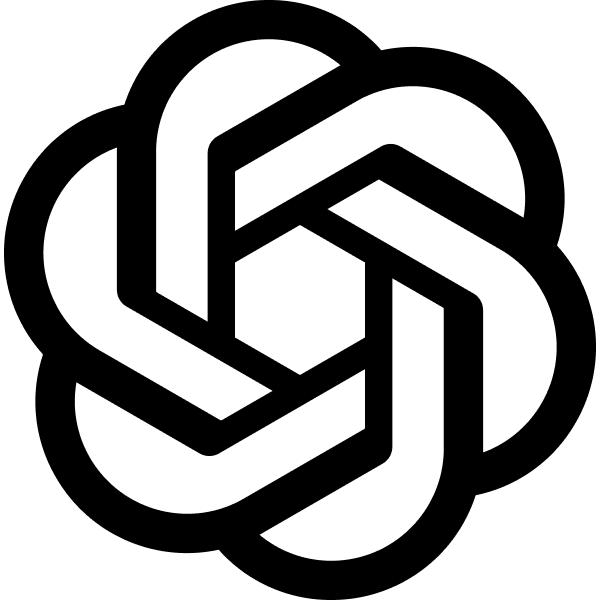
TODO: collapasable, copyable, download, etc
I have this code: ``` function loadDataIfNeeded() { (async () => { if (item.needsData && !loading) { loading = true;
hasError = false;
// TODO: Promise.allSettled()
let results = await loadMetricData(item.query).catch((error) => {
hasError = true;
errorMessage = error.message;
});
item.needsData = false;
item.series = results;
if (!!item.rightQuery) {
console.log('weve got a right query', item.rightQuery);
let rightResults = await loadMetricData(item.rightQuery).catch((error) => {
console.error('right series had an error', error);
// hasError = true;
// errorMessage = error.message;
});
rightResults.forEach((result) => {
result.yAxis = 1;
item.series.push(result);
});
// item.rightSeries = results;
for (let i=0; i < item.rightSeries.length; i++) {
item.rightSeries[i].yAxis = 1;
}
console.log('rightSeries', rightResults);
console.log('TODO rightSeries should have yAxis: 1');
}
}
})();
}
`loadMetricData` returns a promise. I would like to change this code to use `Promise.allSettled` to manage the concurrency. What would that look like?To use `Promise.allSettled` in your `loadDataIfNeeded` function, you need to modify the way the promises are handled. `Promise.allSettled` takes an array of promises and resolves once all of the promises have either fulfilled or rejected. It returns an array of objects describing the outcome of each promise, making it easy to handle both successful and failed operations.
Given that `loadMetricData` returns a promise, you can refactor your code to use `Promise.allSettled` like this:
```javascript
function loadDataIfNeeded() {
(async () => {
if (item.needsData && !loading) {
loading = true;
hasError = false;
errorMessage = '';
let promises = [loadMetricData(item.query)];
if (item.rightQuery) {
promises.push(loadMetricData(item.rightQuery));
}
let results = await Promise.allSettled(promises);
// Process the results
results.forEach((result, index) => {
if (result.status === 'fulfilled') {
let data = result.value;
if (index === 0) {
// This is the result of the first promise
item.series = data;
} else if (index === 1) {
// This is the result of the second promise
data.forEach(d => d.yAxis = 1);
item.series.push(...data);
}
} else {
// Handle errors
console.error('Error loading data:', result.reason);
hasError = true;
errorMessage = result.reason.message;
}
});
item.needsData = false;
console.log('Series:', item.series);
}
})();
}
In this refactored version:
- An array of promises is created, containing the promise from
loadMetricData(item.query)
and optionallyloadMetricData(item.rightQuery)
ifitem.rightQuery
exists. Promise.allSettled(promises)
is used to wait for all promises to settle.- Each result is processed in a
forEach
loop. If a promise is fulfilled, its value is used to updateitem.series
. If a promise is rejected, the error is logged, andhasError
anderrorMessage
are updated. - The
item.needsData
is set tofalse
after processing the promises.
This approach ensures that both promises are handled concurrently, and their outcomes are processed regardless of whether they are fulfilled or rejected.
Use Promise.allSettled refactor code created at Tue Jan 20 1970 16:32:04 GMT+0000 (Coordinated Universal Time)Related Chats
Use Promise.allSettled refactor code 0.999
Mounting Issue with useEffect 0.527
Fix Return Local Reference 0.474
Fix HttpResponse Type Mismatch 0.468
Streaming JSON Parsing Issue 0.459
Fix ExpandableGroupPanel Filter Error 0.441
Async Handler Type Declaration 0.422
New chat 0.383