New chat
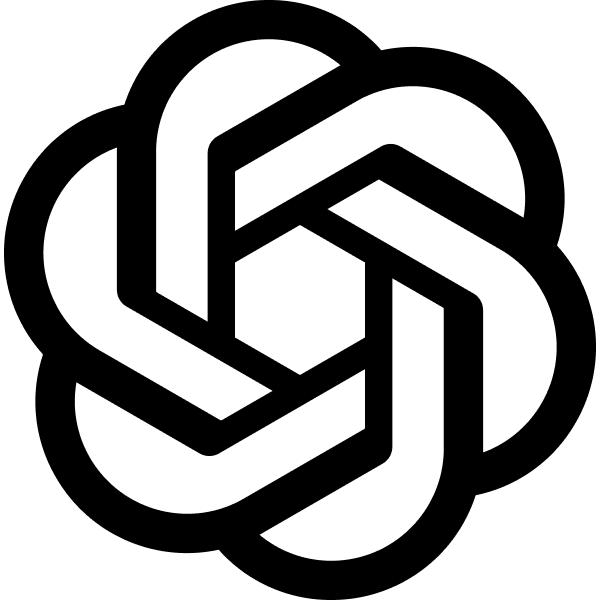
TODO: collapasable, copyable, download, etc
I have a monorepo that doesn't have any special tooling, and my Svelte application is one directory underneath that. How would you go about creating a GetSub module that's a fork of an open source Svelte component? How would I set that up into my monorepo build?Creating a fork of an open source Svelte component and integrating it into your monorepo setup involves a few steps. Here's a simplified breakdown:
Forking the Component:
- Identify the open source Svelte component you want to fork.
- Fork it on the platform it's hosted on, like GitHub. This creates a copy under your account.
Cloning the Fork:
- Clone your forked repository to your local machine.
- You can rename this fork to something like
GetSub
to reflect the purpose it will serve in your project.
Integrating into Your Monorepo:
- Since you mentioned your monorepo doesn't have special tooling, you'll manually add the fork.
- Place the cloned
GetSub
module into a directory within your monorepo, perhaps under a directory likecomponents
orlibraries
.
Adjusting Build Configuration:
- If you're using a build tool like Rollup or Webpack in your Svelte application, ensure it's configured to include and transpile the
GetSub
module. - You might need to adjust import paths in your Svelte application to point to the
GetSub
module.
- If you're using a build tool like Rollup or Webpack in your Svelte application, ensure it's configured to include and transpile the
Handling Dependencies:
- Ensure that any dependencies required by the
GetSub
module are included in your monorepo'spackage.json
. - Run
npm install
oryarn
to install these dependencies.
- Ensure that any dependencies required by the
Development and Testing:
- Make necessary modifications to the
GetSub
module as per your requirements. - Test the module within your Svelte application to ensure it works as expected.
- Make necessary modifications to the
Version Control:
- Commit your changes to your monorepo, including the added
GetSub
module. - Keep your fork updated with the upstream open source project if needed.
- Commit your changes to your monorepo, including the added
Continuous Integration:
- If you have CI/CD pipelines, update them to include the
GetSub
module in the build and deployment processes.
- If you have CI/CD pipelines, update them to include the
Remember, each monorepo setup can be quite unique, so you might need to adjust these steps based on your specific configuration and tools.What are, aside from editing my Vite config andAside from modifying your Vite config, there are several other key steps to consider when integrating a forked Svelte component into your monorepo:
Module Installation and Dependency Management:
- Ensure that all dependencies required by your forked component are included in your project's
package.json
. - Run your package manager (npm or yarn) to install these dependencies.
- Ensure that all dependencies required by your forked component are included in your project's
Importing the Component:
- Modify your Svelte application to import the forked component. Adjust the import paths as necessary to point to the location of your forked component in the monorepo.
Component Customization:
- If needed, make changes to the forked component to suit your application's needs. This might involve tweaking its functionality, styling, or props.
Testing:
- Thoroughly test the integrated component to ensure it functions correctly within your application. Consider unit and integration tests.
Version Control Integration:
- Manage your changes using version control. This includes committing the new component and any changes to your monorepo.
Documentation:
- Update your project's documentation to include information about the new component, how it's used, and any modifications you've made.
7.Can't I do an npm install from a local directory? Couldn't I have the makefile build the open source project and then my package.json point to that local npm package?Absolutely, you can use a local directory as a source for an npm package. This approach can be effective in a monorepo setup. Here's how you can do it:
Build the Open Source Project:
- If the open source Svelte component needs to be built before use, you can do this as part of your Makefile. This process typically involves running commands like
npm install
andnpm run build
in the component's directory.
- If the open source Svelte component needs to be built before use, you can do this as part of your Makefile. This process typically involves running commands like
Reference the Local Package in Your package.json:
- In your Svelte application's
package.json
, you can reference the local version of theGetSub
module. It looks something like this:"dependencies": { "getsub": "file:../path/to/getsub" }
- Replace
../path/to/getsub
with the actual path to the built version of yourGetSub
module within the monorepo.
- In your Svelte application's
Install Dependencies:
- Run
npm install
in your Svelte application directory. npm will recognize the local file path and link the package accordingly.
- Run
Import and Use in Your Svelte Application:
- You can now import and use the
GetSub
module in your Svelte application just like any other npm package.
- You can now import and use the
Keep Your Local Package Updated:
- Each time you make changes to the
GetSub
module, you'll need to rebuild it if necessary. Your Svelte application will pick up these changes the next time it's rebuilt.
- Each time you make changes to the
This method is particularly useful in monorepos as it keeps your package management straightforward and allows you to work on the package and the consuming application simultaneously without having to publish the package to a registry.This happens in Docker. Will that be an issue?Running your setup in Docker adds a layer of complexity, but it's definitely manageable. Here are key considerations for handling a local npm package within a Docker environment:
Dockerfile Configuration:
- Ensure your Dockerfile copies both your Svelte application and the
GetSub
module into the Docker image. You might have something like:COPY ./path/to/getsub /app/path/to/getsub COPY ./path/to/svelte-app /app/path/to/svelte-app
- Adjust the paths based on your monorepo structure.
- Ensure your Dockerfile copies both your Svelte application and the
Building the
GetSub
Module:- If
GetSub
needs to be built before being used by your Svelte app, include these build steps in your Dockerfile. - You could run the Makefile or specific npm commands in the Docker build process to ensure
GetSub
is ready to be used.
- If
Handling npm Install:
- In your Svelte application's
package.json
, the local dependency will still be referenced as:"dependencies": { "getsub": "file:../path/to/getsub" }
- When Docker builds the image, it will run
npm install
and should correctly link to the localGetSub
module, provided the paths in the Dockerfile andpackage.json
are correct.
- In your Svelte application's
Volume Mounts for Development:
- For development, consider using Docker volumes to mount your local source code into the container. This allows for live-reloading and easier development.
- Example:
docker run -v $(pwd)/getsub:/app/getsub -v $(pwd)/svelte-app:/app/svelte-app your-image
Consistency Across Environments:
- Ensure that your local development environment mirrors the Docker environment as closely as possible. This helps in reducing "it works on my machine" problems.
Network and Resource Access:
- Make sure that if
GetSub
requires external resources or network access during build or runtime, these are accessible within the Docker container.
- Make sure that if
By addressing these points, you should be able to effectively manage and use a locally referenced npm package within a Dockerized environment. Remember, Docker is great at encapsulating environments, so as long as everything GetSub
needs is included in the Docker image, it should work seamlessly.For the two techniques we've talked about, which do you recommend? What are the pros and cons of each?Both techniques have their own advantages and disadvantages. Let's compare them:
Directly Integrating the Fork into the Monorepo:
Pros:
- Simplicity: It's straightforward; just place the forked code in your repo and use it.
- No Dependency Management Needed: No need to manage it as a separate npm package.
- Immediate Updates: Any changes made to the fork are instantly reflected in your application.
Cons:
- Complexity in Large Projects: As your monorepo grows, directly including more projects can make it unwieldy.
- Build Time: Increases the overall build time of your monorepo.
- Scalability Issues: If you start adding more such components, it can become harder to manage.
Using a Local npm Install from a Directory:
Pros:
- Modularity: Keeps the component separate, which is cleaner, especially for larger projects.
- Independent Development: Allows the component to be developed, built, and versioned independently.
- Reusable: Makes it easier to reuse the component in other projects.
Cons:
- Setup Complexity: Requires a more complex setup with npm linking or specifying a file path in
package.json
. - Dependency Management: You need to ensure that the local paths are correctly set up and maintained.
- Docker Considerations: Extra care is needed to ensure paths and volumes are correctly mapped in Docker.
Recommendation:
- If your project is relatively small and you don't anticipate adding many more components, directly integrating the fork into your monorepo might be simpler and more efficient.
- If you expect your project to grow, or if you value modularity and potential reusability, setting it up as a local npm package would be more scalable and maintainable in the long run.
Ultimately, the best approach depends on your project's specific needs and how you anticipate it will evolve.SvelteKit invite expect files to be in specific places. I don't see how I can configure it for the first option to pick up source code in a cloned repo underneath my application.Given that SvelteKit expects files to be in specific locations, integrating a cloned repo directly into your SvelteKit application can be challenging. This is where the second option, treating the cloned repo as a local npm package, becomes more advantageous. Let me explain how it aligns better with SvelteKit's structure and expectations:
SvelteKit's Structure:
- SvelteKit has a conventional project structure (like having a
src
directory). Integrating external code directly into this structure can be tricky if the external code doesn't conform to these conventions.
- SvelteKit has a conventional project structure (like having a
Using a Local npm Package:
- By treating the cloned repo as an npm package, you bypass the need to fit its code directly into SvelteKit's expected file structure.
- You simply install it as a local dependency. SvelteKit will then treat it like any other imported module, irrespective of its physical location in your monorepo.
Advantages of the npm Approach with SvelteKit:
- Modularity: Keeps your SvelteKit app clean and adherent to its structure, while still allowing the use of the external component.
- Ease of Update: When you update the component, you don't have to move files around in your SvelteKit project. Just rebuild or re-link the npm package.
- Isolation: It isolates the component's dependencies from your main app, reducing the risk of version conflicts.
Implementation:
- Place the cloned repo in your monorepo but outside of your SvelteKit app's directory.
- Reference it in your SvelteKit app's
package.json
using a local path. - Import and use it in your SvelteKit app as you would with any module.
In summary, given SvelteKit's specific expectations for file locations, treating the cloned repo as a local npm package is likely the more feasible and less intrusive approach. It maintains the integrity of your SvelteKit app's structure and provides a cleaner integration method.
New chat created at Tue Jan 20 1970 18:03:12 GMT+0000 (Coordinated Universal Time)Related Chats
New chat 0.513
Server-Side Code Placement 0.478
Client-Only Component in SvelteKit 0.477
SvelteKit Route Naming Conventions 0.439
Fix SvelteKit Playwright Test 0.436
Privacy Page 404 Error 0.431
Svelte Click Callback Structure 0.415