Svelte Click Callback Structure
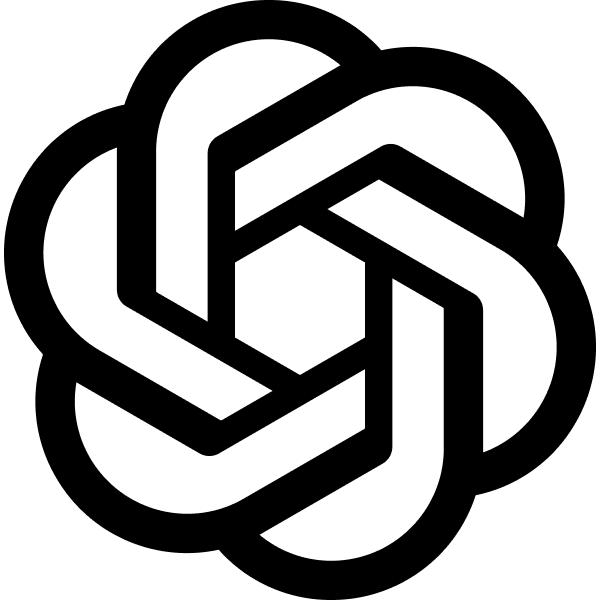
TODO: collapasable, copyable, download, etc
In Svelet, I want to put a click handler on the div in my +page.svelte
file and then in any component, no matter how nested, I want to be able to register for a callback to know that click fired. There may be more han one component registered. How would you structure that?To achieve this in Svelte, you can use a combination of context, custom stores, and event propagation. Here's a structured approach to handle your requirement:
Create a Custom Store: You will create a custom store to manage the callback functions. This store will allow multiple components to register their callbacks.
Set Up Context in
+page.svelte
: Use the Svelte context API to provide the custom store to all child components. This will allow any nested component to access the store and register their callback.Click Handler in
+page.svelte
: Set up a click event handler in your+page.svelte
file. This handler will trigger all the callbacks registered in the store when the div is clicked.Register Callbacks in Nested Components: In your nested components, access the custom store from the context and register the callbacks.
Here's a basic implementation of this approach:
Step 1: Create a Custom Store
// src/stores/callbackStore.js
import { writable } from 'svelte/store';
function createCallbackStore() {
const { subscribe, set, update } = writable([]);
return {
subscribe,
registerCallback: (callback) => {
update(callbacks => [...callbacks, callback]);
},
triggerCallbacks: () => {
update(callbacks => {
callbacks.forEach(callback => callback());
return callbacks;
});
}
};
}
export const callbackStore = createCallbackStore();
Step 2: Set Up Context in +page.svelte
<script>
import { setContext } from 'svelte';
import { callbackStore } from '../stores/callbackStore';
setContext('callbackStore', callbackStore);
const handleClick = () => {
callbackStore.triggerCallbacks();
};
</script>
<div on:click={handleClick}>
<!-- Your content here -->
</div>
Step 3: Register Callbacks in Nested Components
In any nested component:
<script>
import { getContext } from 'svelte';
const callbackStore = getContext('callbackStore');
callbackStore.registerCallback(() => {
console.log('Callback from nested component!');
});
</script>
In this setup:
- The
callbackStore
manages an array of callback functions. - When the div in
+page.svelte
is clicked, it triggerstriggerCallbacks
on the store, which in turn calls all registered callbacks. - Nested components use
getContext
to access thecallbackStore
and register their callbacks viaregisterCallback
.
This approach ensures that any number of components can register for the click event, and the logic is centralized and easily manageable.
Svelte Click Callback Structure created at Tue Jan 20 1970 17:58:25 GMT+0000 (Coordinated Universal Time)Related Chats
Client-Only Component in SvelteKit 0.619
Test Svelte components with Jest. 0.611
Svelte Component Help 0.600
Svelte: Force New Component 0.561
Dropdown Button Component 0.554
Update Svelte Store Item 0.531
Settings Toggle Endpoint Setup 0.527
SSR Error: HTMLElement Not Defined 0.507
New chat 0.495