Svelte: Force New Component
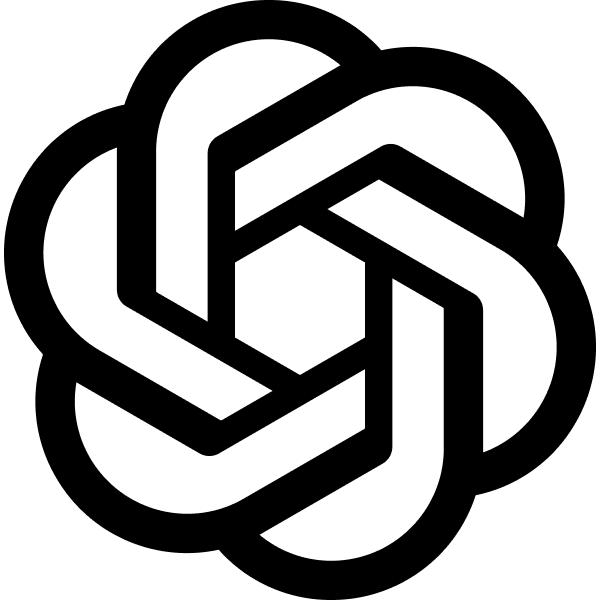
TODO: collapasable, copyable, download, etc
In Svelte, how can I force a completly new instance of a component to be used when I change some of the props that are sent to that child component?In Svelte, to ensure that a completely new instance of a component is used when props change, you can use a key block (#key
). The #key
block in Svelte creates a new instance of the component whenever the key changes. This is useful when you want to reset the state of a component completely in response to changes in its props.
Here's an example of how you can use a #key
block to force the creation of a new instance of a component when certain props change:
<script>
import MyComponent from './MyComponent.svelte';
let key = 0;
let someProp = 'initial value';
// Function to update the prop and key
function updateProp(newValue) {
someProp = newValue;
key++; // Increment key to force a new instance
}
</script>
<!-- Using the key block -->
{#key key}
<MyComponent prop={someProp} />
{/key}
<!-- Button to update the prop -->
<button on:click={() => updateProp('new value')}>Update Prop</button>
In this example, MyComponent
will be recreated from scratch every time the key
value changes. This is achieved by incrementing key
in the updateProp
function, which is called when the button is clicked. The change in key
triggers the #key
block to dispose of the old instance of MyComponent
and create a new one with the updated someProp
value.In the child component, can I print out the key value that was used by the parent?In Svelte, a child component cannot directly access the key value used in the parent's #key
block because the key is scoped only to the parent component. The key in a #key
block is not a prop and is not passed down to the child component. It is only used by Svelte's reactivity system to determine when to recreate the component.
However, if you need the child component to be aware of changes that are causing it to be recreated, you can pass a prop to the child component that changes along with the key. This way, the child component can react to changes in this prop.
Here's how you can modify the previous example to pass a prop to the child component that reflects the changes causing the component to be recreated:
<!-- ParentComponent.svelte -->
<script>
import MyComponent from './MyComponent.svelte';
let key = 0;
let someProp = 'initial value';
function updateProp(newValue) {
someProp = newValue;
key++;
}
</script>
{#key key}
<MyComponent prop={someProp} changeIndicator={key} />
{/key}
<button on:click={() => updateProp('new value')}>Update Prop</button>
<!-- MyComponent.svelte -->
<script>
export let prop;
export let changeIndicator; // This prop changes whenever the parent key changes
// You can react to changes in changeIndicator if needed
$: if (changeIndicator) {
console.log('Component was recreated. Current changeIndicator:', changeIndicator);
}
</script>
<!-- Rest of your component -->
In this setup, changeIndicator
is a prop in MyComponent
that receives the value of the key
from the parent component. When the parent component's key changes, changeIndicator
will also change, allowing MyComponent
to react to these changes.Using onMount and onDestroy... I don't see the component being destoryed and remounted, but I do see that at time step 1 I pass 'p' as the key and at timestep 2 I pass 'p-span.name=' as the keyWhen using Svelte's onMount
and onDestroy
lifecycle functions, you should see them being triggered when a component is mounted and destroyed, respectively. However, if you're observing that onDestroy
is not being called when you expect it to, it could be due to how the #key
block is being used or due to the nature of the keys you are passing.
Let's break down the situation:
Using
onMount
andonDestroy
: These functions are called when a component is first created (onMount
) and when it is about to be removed from the DOM (onDestroy
).Using
#key
block with changing keys: The#key
block should recreate the component instance whenever the key changes. This would meanonMount
runs on each creation, andonDestroy
runs on each destruction of the component instance.Observation of Behavior: You mentioned that the keys you pass change from
'p'
to'p-span.name='
. This should trigger a re-creation of the component if these keys are used within a#key
block.
Here's a basic structure to ensure that onMount
and onDestroy
are being called as expected:
<!-- ParentComponent.svelte -->
<script>
import MyComponent from './MyComponent.svelte';
let key = 'p'; // initial key
function updateKey(newKey) {
key = newKey; // change key to trigger re-creation
}
</script>
{#key key}
<MyComponent />
{/key}
<button on:click={() => updateKey('p-span.name=')}>Change Key</button>
<!-- MyComponent.svelte -->
<script>
import { onMount, onDestroy } from 'svelte';
onMount(() => {
console.log('MyComponent mounted');
});
onDestroy(() => {
console.log('MyComponent will be destroyed');
});
</script>
<!-- Rest of your component -->
If onDestroy
is not being called as expected when the key changes, there could be a few reasons:
Incorrect
#key
Usage: Ensure the#key
block is correctly wrapping your component and that the key really changes.Svelte's Optimizations: Svelte might optimize DOM updates in ways that don't always align with intuitive expectations. This could mean the component isn't actually being destroyed and recreated in the way you expect.
Key Uniqueness: The keys
'p'
and'p-span.name='
should be distinct enough to trigger a re-creation, but ensure there's no other logic interfering with this process.
If you are still facing issues, you might want to review your implementation or share more specific code snippets for a more precise diagnosis.
Svelte: Force New Component created at Tue Jan 20 1970 17:47:58 GMT+0000 (Coordinated Universal Time)Related Chats
Svelte: Force New Component 0.999
Svelte Component Help 0.588
Client-Only Component in SvelteKit 0.585
Update Svelte Store Item 0.584
Svelte Click Callback Structure 0.561
Reactivity Troubleshooting in Svelte 0.560
Test Svelte components with Jest. 0.554
Cleaned-Up Svelte Code 0.542
Listen for Command+K. 0.516