Reactivity Troubleshooting in Svelte
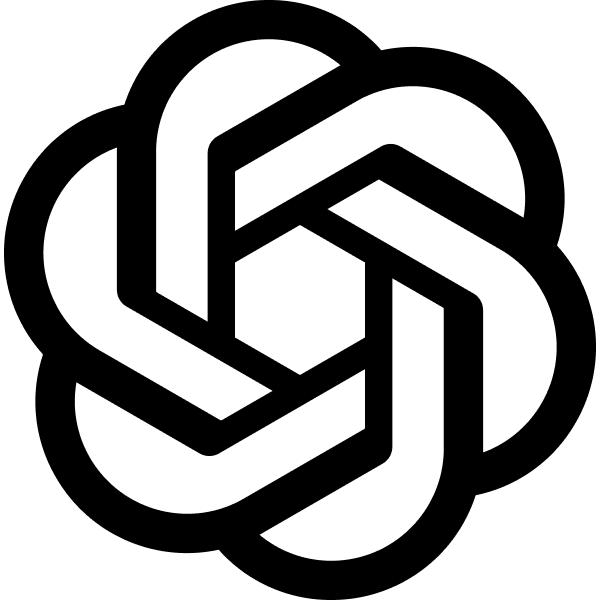
TODO: collapasable, copyable, download, etc
I have a Svelete component A that ``` let groupBy; $: groupBy = $queryBuilderStore.groupBy[groupByIndex]; ... let valid = true; $: valid = isFilterValid(groupBy, attributeDisplayKeyStore);
I then pass valid into component B as a prop <B {valid} .../>
``` export let valid;
B then passes valid into component C as a prop <C {valid} ... />
let bgColor;
$: bgColor = valid ? 'blue' : 'red';
$: console.log('AOK autocomplete valid=', valid);
...
valid={String(valid)}
<a class={`${bgColor}`}>...
When the state changes on queryBuilderStore
, I see isFilterValid
console logging the state change, but the UI doesn't show bgColor
as changing. Also valid=true never updates to false.The issue you're encountering where bgColor
does not update in the UI even though the valid
state changes could stem from several potential causes. Here are a few troubleshooting steps and considerations that can help you identify and resolve the problem:
Reactivity in Svelte Components:
- Ensure that the reactivity statements in Svelte are correctly set up. In your case, it seems you are correctly using the
$:
syntax to makevalid
andbgColor
reactive. However, it's crucial to ensure that changes in thequeryBuilderStore
are correctly propagated through your components.
- Ensure that the reactivity statements in Svelte are correctly set up. In your case, it seems you are correctly using the
Store Subscription:
- Verify that the
queryBuilderStore
andattributeDisplayKeyStore
are correctly set up and that component A properly reacts to their changes. Svelte stores are reactive, but you need to ensure that the component actually reacts to the changes. If you're directly using the store values in a reactive statement, it should work, but issues can arise if the store's update does not trigger a re-render in your component.
- Verify that the
Propagating Props:
- When passing
valid
through multiple components (from A to B to C), ensure that each component correctly handles the prop. It seems you're doing this correctly, but it's worth double-checking that the prop is not inadvertently modified or incorrectly passed at any point in the chain.
- When passing
String Conversion for
valid
:- You mentioned passing
valid={String(valid)}
at one point. This conversion could lead to unexpected behavior if not handled correctly, especially since you're expecting a boolean value for togglingbgColor
. Ensure that this conversion does not interfere with the logic that depends onvalid
being a true boolean value. It's better to pass boolean props directly without conversion, unless there's a specific reason for doing so.
- You mentioned passing
Debugging Reactivity:
- Add more
console.log
statements at various points in your components to trace how thevalid
value changes and propagates through the components. This can help identify where the breakdown in reactivity occurs.
- Add more
Svelte Compilation and Rerendering:
- Sometimes, issues can arise from the Svelte compilation process or from stale state in the browser. Try clearing your browser cache or using a different browser to see if the issue persists. Additionally, make sure you are using the latest version of Svelte and that there are no compilation errors or warnings.
Ensure Reactive Updates:
- In some cases, you might need to explicitly inform Svelte about updates to objects or arrays that it might not automatically detect. If
isFilterValid
depends on deeply nested properties withinqueryBuilderStore
orattributeDisplayKeyStore
, you might need to ensure these changes are detected. However, for simple assignments and store updates, Svelte's reactivity should automatically handle these.
- In some cases, you might need to explicitly inform Svelte about updates to objects or arrays that it might not automatically detect. If
If you've checked these areas and the issue persists, it might be helpful to provide a more detailed code snippet showing how queryBuilderStore
and attributeDisplayKeyStore
are updated and how these updates are supposed to trigger changes in your components. This could offer more insight into where the disconnect in reactivity might be occurring.How would I go about #7?To ensure that Svelte detects updates to objects or arrays, especially when dealing with nested properties or complex state management, you can follow these strategies:
1. Immutable Updates
When updating an object or an array, always create a new object or array rather than modifying the existing one. This approach triggers Svelte's reactivity system because it detects a new reference.
For Arrays:
// Incorrect - Mutates the existing array
myArray.push(newItem);
// Correct - Creates a new array
myArray = [...myArray, newItem];
For Objects:
// Incorrect - Mutates the existing object
myObject.key = newValue;
// Correct - Creates a new object
myObject = { ...myObject, key: newValue };
2. Using Svelte Stores for Complex State
For complex state management, consider using Svelte's built-in stores (writable
, readable
, derived
, etc.). Stores ensure reactivity even with nested properties, as they provide methods to update values that automatically trigger UI updates.
import { writable } from 'svelte/store';
const myStore = writable({ key: 'initialValue' });
// To update
myStore.update(currentState => {
return { ...currentState, key: 'newValue' };
});
3. Manual Trigger of Reactivity
In cases where you're dealing with deeply nested objects and the reactivity might not be triggered by the usual assignment, you can manually trigger a reactivity update by assigning the object or array to itself after making changes.
myObject.nested.key = newValue;
myObject = myObject; // Manually trigger reactivity
myArray[0].property = newValue;
myArray = myArray; // Manually trigger reactivity
Although this approach works, it's generally better to use immutable updates or Svelte stores as they are more in line with Svelte's reactivity model and lead to cleaner code.
4. Using Svelte's get
Function with Stores
If you need to reactively update something based on a store's value without directly subscribing to the store in the component, you can use the get
function from svelte/store
to retrieve the current value of a store and then perform operations based on that value.
import { get } from 'svelte/store';
// Assume `myStore` is a Svelte store
const currentValue = get(myStore);
// Perform operations based on `currentValue`
This approach is useful for reacting to store changes outside of component markup, such as in utility functions or actions.
Conclusion
Adopting these strategies will help ensure that your Svelte components reactively update in response to changes in your application's state, even when dealing with complex nested objects or arrays.
Reactivity Troubleshooting in Svelte created at Tue Jan 20 1970 18:34:10 GMT+0000 (Coordinated Universal Time)Related Chats
Fix ExpandableGroupPanel Filter Error 0.720
New chat 0.718
Value Prop Reactivity Issue 0.709
Svelte Component Help 0.560
Svelte: Force New Component 0.560
Passing inputValue to slot 0.559
Cleaned-Up Svelte Code 0.492