Debugging Docker SvelteKit Logs
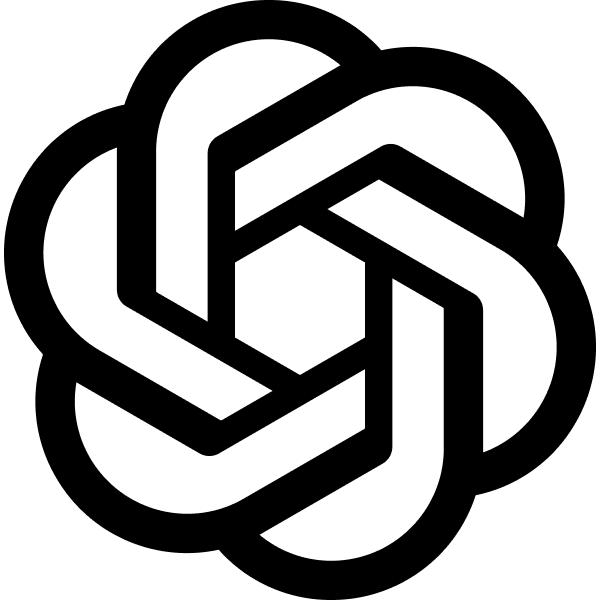
TODO: collapasable, copyable, download, etc
I have a sveltekit app in a docker container. It is run via RUN npm run build
and then CMD ["node", "build"]
When I do a console.log
or a process.stdout.write
I don't see any output in the docker container logs. How do I fix this? My job depends on itNot seeing logs from your SvelteKit application running in a Docker container can be frustrating. Let's troubleshoot the potential reasons for this issue and find a solution.
Check the Logging Level: Ensure that the logging level in your SvelteKit app is set to a level that includes console logs. Some applications might be configured to suppress console output in production environments.
Buffering Issues: Node.js may buffer
stdout
andstderr
outputs. In a Docker context, this can sometimes cause delays in logs appearing. You can force Node.js to run in an interactive terminal mode, which may reduce stdout buffering, by adding the-i
flag. However, this is not a common practice in production and typically isn't necessary.Ensure Proper Docker Logging Configuration: Docker can be configured to use different logging drivers. The default logging driver is
json-file
which should collectstdout
andstderr
from your application. Check your Docker daemon configuration to ensure that it is set to use a logging driver that outputs to a place you can access.Check How You Start the Docker Container: If you're running the Docker container in detached mode (
-d
), make sure you fetch the logs usingdocker logs <container_id>
. Also, ensure that your Docker run command is not silencing the output with options like--quiet
.Inspect Your Dockerfile and npm scripts: Your
Dockerfile
usesRUN npm run build
for the build step andCMD ["node", "build"]
to start the application. Ensure that your npm build script and the CMD command are correctly configured:- The
npm run build
command should correctly build the SvelteKit app without errors. - Ensure that the
CMD ["node", "build"]
command is correct. Typically, thebuild
directory is not what you execute directly with Node.js. Instead, you would start a server script that you have configured in yourpackage.json
file. For instance,CMD ["node", "build/index.js"]
if your entry point isindex.js
inside the build directory.
- The
Run Docker Container Interactively: For debugging purposes, you can run the container interactively with a TTY allocated to see the output directly in your terminal:
docker run -it --rm your_image_name
This can help you debug if the issue is related to Docker's handling of the output or something within the application.
Check for Application Errors: There might be an error in your application that's preventing it from reaching the point where it outputs logs. Ensure your application starts without errors.
Use Proper Logging Mechanisms: Depending on how your SvelteKit application is configured, it might be beneficial to implement a logging library that's designed to work with Docker and could handle log streams more effectively than
console.log
.
Once you've gone through these troubleshooting steps, you should be closer to diagnosing why the logs are not appearing. Remember to replace placeholder text like your_image_name
with the actual names and paths relevant to your application. If you've tried all the above and still can't see the logs, please provide more details about your application, Docker configuration, and the commands you're running, and I can assist you further.How do you configure sveletkit logging levels?SvelteKit doesn't come with a built-in logging level configuration like some backend frameworks (e.g., Winston for Node.js). By default, anything that you console.log
from a SvelteKit endpoint or a server-side component (+server.js
or .svelte
) will be logged to the standard output (stdout), which Docker should capture.
However, you can manage the verbosity of your logs manually or by implementing a logging library. If you want to implement different logging levels (info, warn, error, debug, etc.), you would typically use a logging library or write your own logging utility.
Here are steps to manage logging in SvelteKit with a simple custom logger:
Create a Logger Utility: You could create a simple logger utility that checks for the current environment or a log level environment variable to determine what to log. Here's a very basic example:
// src/lib/logger.js const logLevel = process.env.LOG_LEVEL || 'info'; const levels = { debug: 0, info: 1, warn: 2, error: 3, }; function log(message, level = 'info') { if (levels[level] >= levels[logLevel]) { console.log(`[${level.toUpperCase()}]: ${message}`); } } module.exports = { debug: (msg) => log(msg, 'debug'), info: (msg) => log(msg, 'info'), warn: (msg) => log(msg, 'warn'), error: (msg) => log(msg, 'error'), };
Use Logger in Your Application: Instead of using
console.log
, you can use this logger utility.// Anywhere in your SvelteKit app const logger = require('../lib/logger'); logger.info('This is an informational message'); logger.error('This is an error message');
Set Log Level via Environment Variables: When you run your Docker container, you can pass the log level via environment variables.
docker run -e LOG_LEVEL=debug -p 3000:3000 my-sveltekit-app
This way, you can control the verbosity of your logs without changing the code.
If you are using endpoints (.js
files in the routes
directory), the above method should work since these run on the server. For code that runs on both the server and the client, remember that console.log
statements will only show up in the browser console when they are executed on the client side.
For more sophisticated logging, you may want to look into a dedicated logging library like Pino, Winston, or Bunyan, which are designed to work with Node.js and can be configured to handle different environments and logging levels more elegantly. When using such libraries, ensure they are compatible with the SvelteKit's Vite build process and are included in the appropriate parts of your application (typically server-side only).
Debugging Docker SvelteKit Logs created at Tue Jan 20 1970 16:04:54 GMT+0000 (Coordinated Universal Time)Related Chats
Debugging Docker SvelteKit Logs 0.999
New chat 0.509
Fix SvelteKit Playwright Test 0.442
Detecting SvelteKit Environment: Logging 0.428
Node.js Log Options 0.424
View logs with kubectl. 0.417
Svelte Component Help 0.415
Docker ENV Variables Not Set 0.404