Fix ExpandableGroupPanel Filter Error
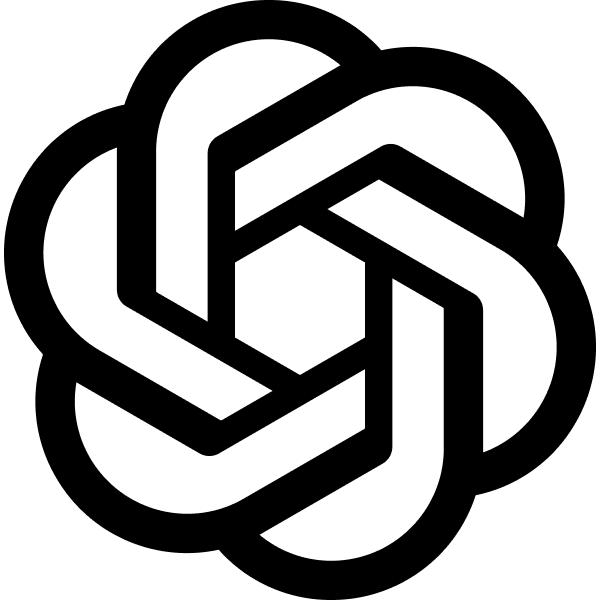
TODO: collapasable, copyable, download, etc
I have a SvelteKit app. I have three components query_builder_filter_group ```
<ExpandableGroupPanel on:click={handleAddFilter}
<!--
Button here is not DRY. Why? If filters are present, we want to wrap this in a div,
otherwise no div so that it can collapse in-line with the Group By Add button
-->
<div>
{#each filters as filter, i (filter.id)}
{JSON.stringify(filter)}
<QueryBuilderFilter {queryBuilderStore} {attributeDisplayKeyStore} filterIndex={i} />
{/each}
</div>
exapandable_panel_group ```<script>
// TODO export let class
</script>
<div
style="min-height: 38px"
class="border border-solid border-rounded p-2 mb-4 flex items-start bg-red-900" on:click>
<slot />
</div>
<style>div {
min-height: 38px;
}</style>```
and lastly query_builder_filter ```<script>
import { onMount } from 'svelte';
import { TrashBinOutline } from 'flowbite-svelte-icons';
import { Button } from 'flowbite-svelte';
import AutocompleteInput from './autocomplete_input.svelte';
import { autocompleteAttributeValues } from '$lib/query_metrics';
import QueryBuilderFilterOperator from './query_builder_filter_operator.svelte';
import {
displayValueForKey,
resolveAttributeValue
} from '$lib/stores/attribute_display_name_store';
export let queryBuilderStore;
export let attributeDisplayKeyStore;
export let filterIndex;
let events = [];
let filter;
$: filter = $queryBuilderStore.filters[filterIndex];
let selectedFilterKey = '';
let filterAttributeValues = [];
let filterValueInputRef;
onMount(() => {
if (!filter || !filter.key || filter.key.length == 0) {
return;
}
handleFilterNameSelected(
{
detail: {
selected: filter.key
}
},
filter.value
);
});
const updateFilter = (selectedFilterKey, selectedFilterValue) => {
selectedFilterValue = selectedFilterValue || '';
queryBuilderStore.update((previous) => {
let newFilters = previous.filters.slice(0);
newFilters.forEach((filter, idx) => {
if (idx === filterIndex) {
filter.key = selectedFilterKey;
filter.value = selectedFilterValue;
}
});
return {
...previous,
filters: newFilters
};
});
};
const handleFilterNameSelected = ({ detail }, filterValue) => {
// filterValue will be populated in the case we hit Edit on a chart
filterValue = filterValue || '';
selectedFilterKey = resolveAttributeValue(detail.selected, queryBuilderStore);
(async () => {
let rawFilterAttributeValues = await autocompleteAttributeValues(selectedFilterKey);
if (rawFilterAttributeValues.filter) {
filterAttributeValues = rawFilterAttributeValues.filter(
(value) => !!value && value.length > 0
);
} else {
filterAttributeValues = rawFilterAttributeValues;
}
})();
updateFilter(selectedFilterKey, filterValue); // value depends on key, clear the value
};
const handleValueChange = (newOperator) => {
queryBuilderStore.update((previous) => {
let newFilters = previous.filters.slice(0);
newFilters.forEach((filter, idx) => {
if (idx === filterIndex) {
filter.operator = newOperator;
}
});
return {
...previous,
filters: newFilters
};
});
};
const filterValueSelected = ({ detail }) => {
updateFilter(selectedFilterKey, detail.selected);
};
const handleRemoveFilter = (event) => {
console.log('abf handleRemoveFilter', event);
event.preventDefault();
queryBuilderStore.update((previous) => {
let newFilters = previous.filters.filter((filter, idx) => {
console.log('AOK filtering', filter, filterIndex, idx, idx != filterIndex);
return idx != filterIndex;});
return {
...previous,
filters: newFilters
};
});
};
const handleFilterKeyCleared = () => {
console.log('filter key cleared');
events = [...events, 'clear'];
};
const handleFilterValueCleared = () => {
console.log('filter value cleared');
events = [...events, 'clear'];
};
</script>
<div class="flex justify-start item-start mb-1">
<!-- code>{JSON.stringify($attributeDisplayKeyStore)}</code -->
{#if filter}
<AutocompleteInput
label=""
placeholder={`(everywhere)`}
showAllResultsOnFocus
value={displayValueForKey(filter.key, attributeDisplayKeyStore)}
data={$attributeDisplayKeyStore}
extract={(item) => item}
onSelect={handleFilterNameSelected}
onClear={handleFilterKeyCleared}
wClass="w-64"
/>
{#if filter && !!filter.key}
<QueryBuilderFilterOperator operator={filter.operator} onValueChange={handleValueChange} />
{/if}
{#if filter && filter.value != null}
<AutocompleteInput
label=""
placeholder={`(everywhere)`}
showAllResultsOnFocus
value={filter.value}
data={filterAttributeValues}
extract={(item) => item}
onSelect={filterValueSelected}
onClear={handleFilterValueCleared}
bind:this={filterValueInputRef}
wClass="w-64"
/>
{/if}
<Button
outline
color="red"
class="self-end ml-8 ml-1"
size="xs"
on:click={handleRemoveFilter}
title="Remove Filter"><TrashBinOutline class="w-3.5 h-5" /></Button
>
{/if}
</div>
<style>
:global(input) {
margin: 0;
}
</style>
Before I put in ExpandableGroupPanel, everything was working well. I extracted the div to ExpandableGroupPanel and now I get errors around <AutocompleteInput label="" placeholder={`(everywhere)`} showAllResultsOnFocus value={displayValueForKey(filter.key, attributeDisplayKeyStore)}
that filter is null when reading key
. What changes do I need to make to be able to refactor to ExpandableGroupPanel?The issue you're encountering seems to be related to the lifecycle of the Svelte components and the way data is passed down from the parent (ExpandableGroupPanel
) to the child (QueryBuilderFilter
). When you refactored the code to use ExpandableGroupPanel
, it's possible that the timing of when the filter
object is available in the child components changed, leading to the error where filter
is null
when trying to access filter.key
.
Here are some steps to debug and potentially fix the issue:
Ensure Proper Data Flow: Make sure that the
filter
object is correctly passed down from theExpandableGroupPanel
to theQueryBuilderFilter
. This involves checking thequeryBuilderStore
updates and how they propagate through the components.Check for Null or Undefined: In
QueryBuilderFilter
, before usingfilter.key
, add a check to ensurefilter
is not null or undefined. This is a safeguard against attempts to access properties of a null object.Svelte's Reactive Statements: Svelte's reactivity is triggered when assignments are made. Ensure that your stores and reactive statements are correctly set up to respond to changes. Sometimes, restructuring or moving reactive statements (
$:
) can solve timing issues.Debugging Logs: Add console log statements at different points in the component lifecycle (
onMount
, reactive statements, event handlers) to track the value and state offilter
. This can give you insights into when and whyfilter
becomes null.Component Lifecycle: Investigate if there are lifecycle issues due to the
ExpandableGroupPanel
. The timing of child component creation in Svelte can sometimes lead to issues if the parent component's state changes after the child components are initialized.Using
bind:this
: In some cases, usingbind:this
to get a reference to the child component and then manually handling updates can solve timing issues.Async Data Loading: If the
filter
data is loaded asynchronously, ensure that the components properly handle states where data is not yet available.Svelte Context API: If the data needs to be shared across deeply nested components, consider using Svelte's context API to pass down data without prop drilling.
Here is a simple check you can add to QueryBuilderFilter
:
<script>
// ... existing imports and exports
$: if (filter) {
// existing reactive statements that depend on filter
}
</script>
<div class="flex justify-start item-start mb-1">
{#if filter}
<!-- existing HTML and component logic -->
{/if}
</div>
This ensures that the component logic within QueryBuilderFilter
only runs when filter
is not null, potentially avoiding the errors you're encountering.You have all the code, can you check Ensure Proper Data Flow for me?Certainly! To ensure proper data flow in your SvelteKit application, let's analyze how data is passed and used across the provided components. We'll focus on the filters
object, how it's updated, and how it's passed down to child components.
1. query_builder_filter_group
Component
- Data Source: The
filters
array is derived from thequeryBuilderStore
. - Reactivity: Reactive statements (
$:
) are used to keep thefilters
array in sync with thequeryBuilderStore
. - Child Components:
filters
is iterated over, and for eachfilter
, aQueryBuilderFilter
component is instantiated.
Key Observation:
- Ensure that the
queryBuilderStore
is correctly initialized and updated. The reactivity should automatically update thefilters
array whenever the store changes.
2. ExpandableGroupPanel
Component
- Role: This component seems to be a wrapper that provides an expandable UI feature.
- Data Handling: It doesn't directly handle the
filters
array but acts as a container for the renderedQueryBuilderFilter
components.
Key Observation:
- Since
ExpandableGroupPanel
does not directly manipulatefilters
, it should not be the source of the issue unless it affects the rendering lifecycle of its child components.
3. query_builder_filter
Component
- Data Reception: Receives data related to a single filter, such as
filterIndex
,queryBuilderStore
, andattributeDisplayKeyStore
. - Initialization Check: Ensure that
filter
is defined before accessing its properties likefilter.key
.
Key Observation:
- Add a safeguard to check if
filter
is defined before using its properties. This can prevent attempts to access properties of an undefined object.
Debugging Steps:
Check Store Initialization: Verify that
queryBuilderStore
is properly initialized before being passed toquery_builder_filter_group
.Reactive Update Validation: Confirm that the reactive statements in
query_builder_filter_group
are correctly updating thefilters
array whenqueryBuilderStore
changes.Child Component Rendering: Ensure that each
QueryBuilderFilter
component receives the correctfilter
object. You might want to log thefilter
object inside eachQueryBuilderFilter
instance to verify it's receiving the correct data.Null Checks: In
query_builder_filter
, add checks to ensurefilter
is not null or undefined before accessing its properties.Lifecycle Methods: Use Svelte's lifecycle methods like
onMount
inquery_builder_filter
to handle any initialization that depends on external props.Testing Store Updates: Temporarily hard-code some filter values in the
queryBuilderStore
to see if they properly propagate to the child components. This can help identify if the issue is with the store or the component implementation.
By following these steps, you should be able to trace and fix the issue related to the filter
object being null. Remember, in reactive frameworks like Svelte, the order of operations and the timing of reactivity are crucial. Ensuring that data flows correctly through the components is key to resolving such issues.
Related Chats
Fix ExpandableGroupPanel Filter Error 1.000
New chat 0.821
Reactivity Troubleshooting in Svelte 0.720
Value Prop Reactivity Issue 0.596
Cleaned-Up Svelte Code 0.578
Reactivity Issue Resolution 0.562
Svelte Component Help 0.541
Store Iteration Order Issue 0.514