Fix HttpResponse Type Mismatch
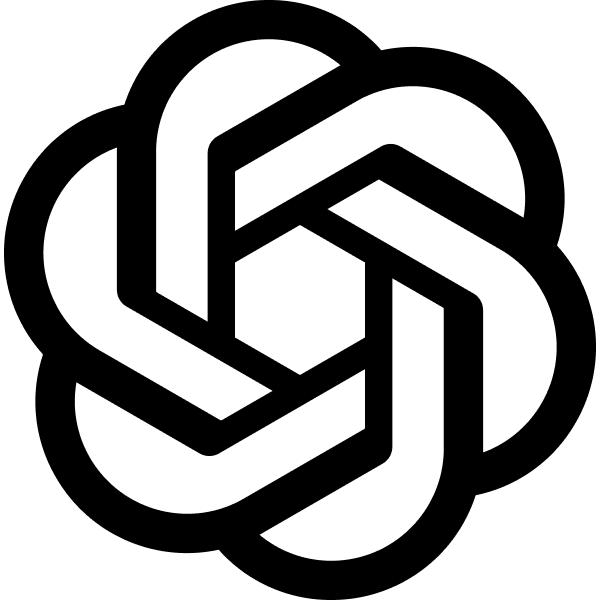
TODO: collapasable, copyable, download, etc
In my Rust actix web application, I have the following code:
use actix_web::{get, web, HttpResponse, Responder};
use crate::model::strapi_client::get_auction_lot;
#[get("/social-share-image/auction/{auction_id}/lot-{lot_number}")]
pub async fn social_share_image_controller(
path: web::Path<(u32, u32)>,
) -> impl Responder {
let (auction_id, lot_number) = path.into_inner();
let auction_lot_strapi = get_auction_lot(auction_id, lot_number, true).await;
let image_path = match auction_lot_strapi.attributes.images {
Some(images) if images.data.is_some() => {
images.data.unwrap().iter().map(|image_wrapper| -> String {
let image = image_wrapper.attributes;
println!("{:?}", image);
String::from("")
});
},
None => { return something_went_wrong("No images for this auction lot") }
};
let msg = "OK";
HttpResponse::Ok().body(format!("{{\"bid_code\": \"{msg}\"}}"))
}
fn something_went_wrong(msg: &str) -> impl Responder {
HttpResponse::BadRequest().body(format!("{{\"bid_code\": \"{msg}\"}}"))
}```
And the compiler gives me the following error
```In my Rust actix web application, I have the following code:
use actix_web::{get, web, HttpResponse, Responder};
use crate::model::strapi_client::get_auction_lot;
#[get("/social-share-image/auction/{auction_id}/lot-{lot_number}")] pub async fn social_share_image_controller( path: web::Path<(u32, u32)>, ) -> impl Responder { let (auction_id, lot_number) = path.into_inner(); let auction_lot_strapi = get_auction_lot(auction_id, lot_number, true).await;
let image_path = match auction_lot_strapi.attributes.images {
Some(images) if images.data.is_some() => {
images.data.unwrap().iter().map(|image_wrapper| -> String {
let image = image_wrapper.attributes;
println!("{:?}", image);
String::from("")
});
},
None => { return something_went_wrong("No images for this auction lot") }
};
let msg = "OK";
HttpResponse::Ok().body(format!("{{\"bid_code\": \"{msg}\"}}"))
}
fn something_went_wrong(msg: &str) -> impl Responder { HttpResponse::BadRequest().body(format!("{{"bid_code": "{msg}"}}")) }```
And the compiler gives me the following error
error[E0308]: mismatched types
--> src\controller\social_share_image.rs:26:5
|
9 | ) -> impl Responder {
| -------------- expected `impl Responder` because of return type
...
26 | HttpResponse::Ok().body(format!("{{\"bid_code\": \"{msg}\"}}"))
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^ expected opaque type, found `HttpResponse`
...
29 | fn something_went_wrong(msg: &str) -> impl Responder {
| -------------- the expected opaque type
|
= note: expected opaque type `impl Responder`
found struct `HttpResponse`
note: return type inferred to be `impl Responder` here
--> src\controller\social_share_image.rs:22:26
|
22 | None => { return something_went_wrong("No images for this auction lot") }
| ^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^^
For more information about this error, try `rustc --explain E0308`.
error: could not compile `estate-auction` (lib test) due to previous error
warning: build failed, waiting for other jobs to finish...
error: could not compile `estate-auction` (lib) due to previous error
[Finished running. Exit status: 0]```
What is the best way to fix this?
The provided Rust code and error message you've posted seem to containThe error message you're seeing indicates that the compiler expects an `impl Responder` type but finds a concrete type (`HttpResponse`). This can happen when different branches of a function produce different types, but the function signature specifies `impl Responder`. The `impl Responder` is an opaque type, which means all branches need to produce the same concrete type.
The issue arises because you're returning an `HttpResponse` directly in one case, but returning the result of the `something_went_wrong` function in another case. The function `something_went_wrong` itself returns `impl Responder`, so it's okay, but the direct `HttpResponse` return value in the main function does not match this.
To resolve this, make sure all branches of your function return the same type.
1. Change the `something_went_wrong` function to return `HttpResponse` directly:
```rust
fn something_went_wrong(msg: &str) -> HttpResponse {
HttpResponse::BadRequest().body(format!("{{\"bid_code\": \"{}\"}}", msg))
}
- Update the match arms in the
social_share_image_controller
function:
let image_path = match auction_lot_strapi.attributes.images {
Some(images) if images.data.is_some() => {
images.data.unwrap().iter().map(|image_wrapper| -> String {
let image = image_wrapper.attributes;
println!("{:?}", image);
String::from("")
});
// Assuming you'd eventually want to return an HttpResponse here too.
// Update this as per your requirements.
HttpResponse::Ok().body(format!("{{\"bid_code\": \"{}\"}}", msg))
},
None => return something_went_wrong("No images for this auction lot"),
};
HttpResponse::Ok().body(format!("{{\"bid_code\": \"{}\"}}", msg))
By making these changes, every branch and the function something_went_wrong
will now return an HttpResponse
directly, making them consistent and resolving the type mismatch issue.For this code ```let auction_lot_strapi = get_auction_lot(auction_id, lot_number, true).await;
println!("{:?}", &auction_lot_strapi);
let _image_path = match auction_lot_strapi.attributes.images {
Some(images) if images.data.is_some() => {
println!("We got images!");
let image_outer_wrapper = images.data.unwrap();
image_outer_wrapper.iter().map(|image_wrapper| -> String {
let image = &image_wrapper.attributes;
println!("{:?}", image);
String::from("")
})
},
Some(_) | None => {
println!("Man I didn't get shit");
return something_went_wrong("No images for this auction lot") }
};```
I get this error
Compiling estate-auction v0.1.0 (C:\Users\ozten\Projects\estate-auction)
error[E0597]: `image_outer_wrapper` does not live long enough
--> src\controller\social_share_image.rs:20:13
|
16 | let _image_path = match auction_lot_strapi.attributes.images {
| ----------- borrow later stored here
...
19 | let image_outer_wrapper = images.data.unwrap();
| ------------------- binding `image_outer_wrapper` declared here
20 | image_outer_wrapper.iter().map(|image_wrapper| -> String {
| ^^^^^^^^^^^^^^^^^^^^^^^^^^ borrowed value does not live long enough
...
25 | },
| - `image_outer_wrapper` dropped here while still borrowed
For more information about this error, try `rustc --explain E0597`.
error: could not compile `estate-auction` (lib test) due to previous error
warning: build failed, waiting for other jobs to finish...
error: could not compile `estate-auction` (lib) due to previous error
[Finished running. Exit status: 0]
How do I fix this?The issue arises due to Rust's borrowing rules. Let's break down the error:
image_outer_wrapper
is borrowed inside themap
function in the iterator.- The iterator created by
image_outer_wrapper.iter().map(...)
is returned from the match arm. This means the iterator holds a reference toimage_outer_wrapper
. image_outer_wrapper
gets dropped at the end of the match arm, while it's still borrowed by the iterator.
To fix this, you have a few options:
Option 1: Collect the Iterated Values
Instead of returning an iterator directly, you can collect the iterated values into a collection (like a Vec
):
let _image_path: Vec<String> = match auction_lot_strapi.attributes.images {
Some(images) if images.data.is_some() => {
println!("We got images!");
let image_outer_wrapper = images.data.unwrap();
image_outer_wrapper.iter().map(|image_wrapper| -> String {
let image = &image_wrapper.attributes;
println!("{:?}", image);
String::from("")
}).collect()
},
Some(_) | None => {
println!("Man I didn't get shit");
return something_went_wrong("No images for this auction lot");
}
};
By collecting into a Vec
, the borrowed values get moved, so you don't face the borrowing problem anymore.
Option 2: Move the values
If you don't actually need the values inside image_outer_wrapper
afterward, you can move them out by using into_iter()
:
image_outer_wrapper.into_iter().map(|image_wrapper| -> String {
let image = &image_wrapper.attributes;
println!("{:?}", image);
String::from("")
})
This converts the owned values into iterators, avoiding the borrow.
In general, it's helpful to decide on whether you actually want to process the data right away (in which case collecting or another form of immediate processing might be ideal) or if you want to lazily process the data (in which case you'll need to deal with lifetimes and borrow checker intricacies).For the function with the signature fn create_social_share_image(images: Vec<SimpleImage>) -> Bytes {
I have the last line Bytes::from(background_image.as_bytes())
But I get the errror ```error[E0597]: background_image
does not live long enough
--> src\controller\social_share_image.rs:85:17
|
68 | let background_image = ImageReader::open(background_image_path).unwrap().decode().unwrap();
| ---------------- binding background_image
declared here
...
85 | Bytes::from(background_image.as_bytes())
| ------------^^^^^^^^^^^^^^^^^^^^^^^^^^^-
| | |
| | borrowed value does not live long enough
| argument requires that background_image
is borrowed for 'static
86 | }
| - background_image
dropped here while still borrowed
For more information about this error, try rustc --explain E0597
.```
How can I fix this?The error you're seeing indicates that background_image
is a temporary value that doesn't live long enough to be converted into Bytes
. The Bytes::from(...)
function expects a 'static
lifetime for its argument when converting from borrowed data, implying that it needs a static reference or something that lives for the entire duration of the program.
However, background_image
is a local variable that will be dropped at the end of the function, so it doesn't have a 'static
lifetime.
To fix this, you'll need to convert the image into a byte vector and then into Bytes
. Let's see a few ways to achieve this:
Option 1: Convert the Image into a Byte Vector First
If background_image
has a method to convert itself into a Vec<u8>
, use that:
let byte_vector: Vec<u8> = background_image.to_bytes(); // or similar function
Bytes::from(byte_vector)
Option 2: Use Dynamic Bytes
If you're sure that background_image.as_bytes()
gives you a &[u8]
slice, you can directly create dynamic Bytes
:
Bytes::copy_from_slice(background_image.as_bytes())
This method copies the data from the slice into a new heap-allocated buffer, so there are no references to the local background_image
.
Option 3: Serialize the Image
If background_image
needs to be serialized to get its byte representation, consider using an image library or serialization library to do this:
let mut byte_vector = Vec::new();
background_image.write_to(&mut byte_vector, image::ImageOutputFormat::Png)?; // or equivalent
Bytes::from(byte_vector)
Remember to handle errors gracefully instead of using unwrap()
. The .unwrap()
method is great for prototyping, but in production code, you should gracefully handle potential errors using the ?
operator or match expressions.
Related Chats
Fix HttpResponse Type Mismatch 1.000
Fix Return Local Reference 0.599
Use Promise.allSettled refactor code 0.468
Scraper Implementation with Enum 0.451
Fix ExpandableGroupPanel Filter Error 0.435
Rust Enum for Strings 0.413
New chat 0.391
Mounting Issue with useEffect 0.380