Exporting Markdown with Plugins
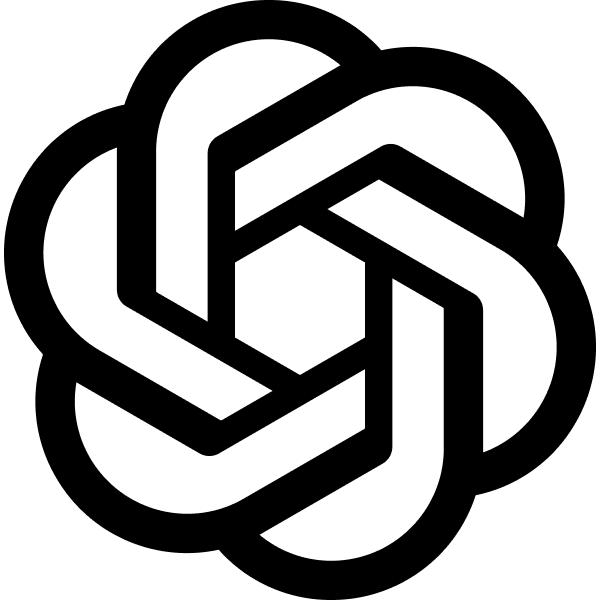
TODO: collapasable, copyable, download, etc
With Obsidian, how can I export Markdown files of a page that executes it's plugins such as dataview, to create more dyanmic snapshots of that markdown page?Exporting Markdown files from Obsidian, especially those that execute plugins like Dataview, requires a few steps since plugins often modify or add content dynamically, which might not be directly reflected in the raw Markdown files. Here's a general approach to create more dynamic snapshots of Markdown pages with plugin outputs:
Use Obsidian's In-Built Export Features:
- Obsidian natively supports exporting notes to various formats. However, this usually exports the raw Markdown content without the results of plugin executions.
- To access this, open the note, click on the 'More options' (three dots in the upper right corner), and select 'Export to PDF', 'Export to HTML', or other available formats.
Capture Plugin Outputs:
- For plugins like Dataview, which dynamically generate content based on your Markdown files, capturing the output can be tricky.
- One method is to export your note as a PDF or HTML, as these formats might include the rendered output of plugins. This depends on how Obsidian and the specific plugin handle rendering.
Use Third-Party Tools:
- There are third-party tools and plugins that can convert or print a page as it appears in Obsidian, including the results of plugins.
- You can search for plugins in Obsidian's community plugins that offer advanced export features.
Manual Approach:
- If the above methods don't work, you might need to manually copy the rendered output from the preview mode and paste it into a new Markdown file or another document format. This is not ideal for automation or handling multiple files.
Automating with Scripts:
- If you're comfortable with scripting, you could write a script to extract the rendered HTML from Obsidian and convert it back to Markdown. This requires programming knowledge and familiarity with HTML and Markdown syntax.
Check for Updates or Community Solutions:
- Obsidian has an active community and development team. Regularly check for updates or community forums for new features or plugins that might offer a more streamlined solution.
Remember, the effectiveness of these methods can vary based on the specific plugins you use and how they render their content within Obsidian. Always test with a few files first to ensure the results meet your expectations.How would I automate with a script?Automating the process of exporting Obsidian Markdown files that include plugin outputs, such as Dataview, involves several steps and requires familiarity with programming. Here's a general outline of how you might approach this with a script:
1. Choose a Scripting Language and Setup
- Python is a popular choice due to its rich set of libraries for handling files and HTML processing.
- Ensure you have the necessary environment and libraries installed (e.g., Python, BeautifulSoup for HTML parsing).
2. Access Obsidian Files
- Obsidian stores its notes as plain Markdown files in your file system.
- Write a script to navigate to the directory where Obsidian stores these files.
3. Render Plugin Outputs (if needed)
- If the plugin's output is not directly stored in the Markdown files (like Dataview), you need to render these outputs. This is the challenging part as it requires replicating Obsidian's rendering logic.
- This might involve running an Obsidian-like environment or a headless browser that can execute JavaScript and render HTML, such as Puppeteer for Node.js.
4. Convert Rendered Outputs to Desired Format
- Once you have the rendered HTML (with plugin outputs), use a library to parse and convert it back to Markdown or any other format.
- For HTML to Markdown conversion, you can use libraries like
turndown
in JavaScript orhtml2text
in Python.
5. Save the Exported Files
- Save the converted content back into Markdown files or any other format you need.
- Organize the files in a directory structure that mirrors your Obsidian vault, if necessary.
6. Automation
- To automate, you can set up this script to run at regular intervals using a task scheduler:
- On Windows, use Task Scheduler.
- On macOS and Linux, use cron jobs.
7. Error Handling and Logging
- Make sure your script can handle errors, such as missing files or parsing errors.
- Add logging to help debug issues when the script runs.
Example Pseudocode
Here's a simplified pseudocode example in Python:
import os
from bs4 import BeautifulSoup
import markdown2
def convert_html_to_markdown(html_content):
return markdown2.markdown(html_content)
def process_files(directory):
for filename in os.listdir(directory):
if filename.endswith(".md"):
# Logic to render HTML (might involve external tools or libraries)
html_content = render_markdown_with_plugins(os.path.join(directory, filename))
# Convert HTML back to Markdown
markdown_content = convert_html_to_markdown(html_content)
# Save the file
with open(os.path.join(directory, "exported", filename), 'w') as f:
f.write(markdown_content)
# Replace 'your_obsidian_vault_path' with the path to your Obsidian vault
process_files('your_obsidian_vault_path')
Important Notes
- This process can get complex, especially when dealing with dynamic content generated by plugins like Dataview.
- The script's effectiveness depends heavily on how these plugins render their content and whether it's feasible to replicate this outside of Obsidian.
- Always back up your notes before running any scripts that modify or export them.
- The community around Obsidian might have developed similar solutions; it's worth checking community forums or plugins for any existing tools.This is a terrible strategy. Wouldn't writing an Obsidian plugin be cleaner?Indeed, writing a custom plugin for Obsidian can be a more integrated and cleaner approach for exporting Markdown files, especially those that interact with other plugins like Dataview. Here's how you might go about it:
Understanding Obsidian's Plugin Architecture
- Obsidian plugins are typically written in JavaScript or TypeScript.
- Familiarize yourself with Obsidian's API documentation and plugin development guidelines. These resources are essential for understanding how to interact with the application and its data.
Steps for Writing an Obsidian Plugin
Set Up Your Development Environment:
- Install Node.js and a code editor like Visual Studio Code.
- Set up a new project for your plugin. You can use Obsidian's sample plugin repository as a starting point.
Learn the Basics of Obsidian Plugin Development:
- Understand how to interact with Obsidian's API.
- Look at existing plugins for inspiration and understanding, especially those that modify or export files.
Implement Your Plugin Logic:
- Your plugin will need to interact with Obsidian's file system to access Markdown files.
- Integrate with other plugins like Dataview. This can be complex, depending on how these plugins expose their data and functionality. You may need to collaborate with the maintainers of these plugins or study their code to understand how to best integrate with them.
Handle the Export Functionality:
- Implement functionality to export the Markdown content, including the dynamically generated content from plugins.
- Depending on your needs, this could mean exporting to different file formats (like PDF, HTML, etc.) or simply creating a more dynamic Markdown file.
Testing and Debugging:
- Test your plugin thoroughly to ensure it works as expected and handles edge cases.
- Remember to check how it interacts with different versions of Obsidian and other plugins.
Publishing Your Plugin:
- Once your plugin is ready and tested, you can publish it to Obsidian's community plugin repository to share it with others.
- Follow Obsidian's guidelines for plugin submission.
Advantages of a Custom Plugin
- Integration: A custom plugin can seamlessly integrate with the Obsidian environment and its existing plugins.
- User Experience: Provides a native user experience without requiring external tools or scripts.
- Customization: You have complete control over how the plugin behaves and can customize it to your specific needs.
Potential Challenges
- Complexity: Writing a plugin requires good knowledge of JavaScript/TypeScript and understanding Obsidian's API.
- Maintenance: You'll need to maintain the plugin, especially as Obsidian updates or if other plugins it interacts with change.
Conclusion
Creating a custom plugin is indeed a cleaner and more integrated approach but requires a fair amount of development expertise. If you're comfortable with programming and willing to invest the time, it's a great way to add the functionality you need to Obsidian.
Exporting Markdown with Plugins created at Tue Jan 20 1970 17:45:23 GMT+0000 (Coordinated Universal Time)Related Chats
Productivity Tips for Obsidian 0.454
Read Scientific Paper Help 0.320
HTML Data Scraping. 0.315
Microservice Architecture Diagram Tools 0.297
Extracting URLs from HTML 0.255
Fantasy world map. 0.253
SSML Emphasis Usage. 0.245
No SSML Support 0.236