Reactivity Issue Resolution
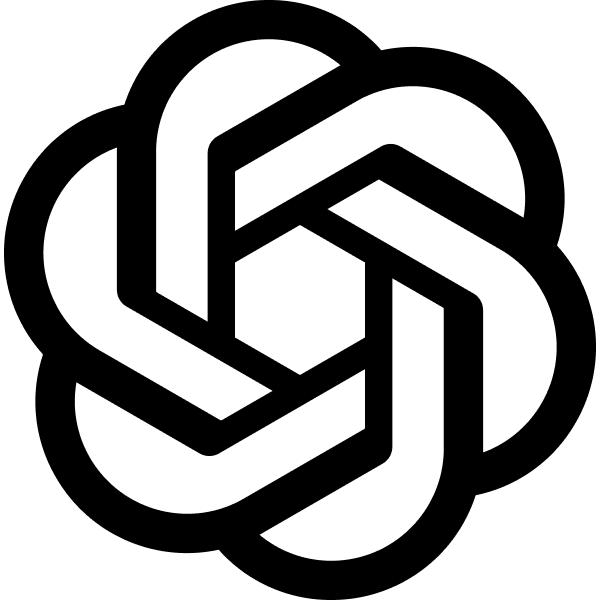
TODO: collapasable, copyable, download, etc
I am seeing missing data in my Svelte component. here is my code ```
{SYSTEM_USERNAME}
{#if item.artifacts}
{#each item.artifacts as artifact, a_idx}
{/each}
{/if}
{#if item.summary && item.summary.message}
Summary:
{/if}
{#if item.interactable}
<QuestionAnswer {index} item={item.interactable} />
{/if}
In the rendered `<SvelteMarkdown` the data is stale and missing dataThe issue with stale or missing data in your Svelte component, particularly within the `<SvelteMarkdown>` component, could stem from a few common pitfalls in reactive Svelte applications. Here's a breakdown of potential causes and solutions:
### Reactivity Issues
In Svelte, the `$:` syntax denotes a reactive statement, meaning the statement re-runs whenever the values of its dependencies change. However, this reactivity relies on the assignment of new values to trigger updates. In your script, `messageSource` is computed reactively based on `item?.summary?.message` and `$guidMap`, but ensure that:
1. **Updates to `item` or `guidMap` are made through assignments** to trigger reactivity. If these objects are mutated internally without assigning a new object/value to the variable, Svelte's reactivity system won't detect changes.
### Possible Fixes
1. **Ensure Proper Assignment for Reactivity:**
- When updating `item` or any property within it, ensure you're assigning it a new value/object rather than mutating it directly. For example, if you update `item.summary` or `item.summary.message`, ensure you do something like `item = { ...item, summary: newSummary }` to trigger reactivity.
2. **Check `$guidMap` Usage:**
- You've used `$guidMap` in your script, but the reactive declaration uses `guidMap` without the `$` prefix in the call to `refToMarkdown`. This might be a typo or misunderstanding of reactive store usage. Ensure you're correctly subscribing to Svelte stores (with `$` prefix) where needed.
3. **Debugging Reactivity:**
- Temporarily add a `console.log` or a debug statement inside your reactive statement to ensure it's being re-run as expected when `item` or `$guidMap` changes.
4. **Verify Data Integrity:**
- Ensure that the data being passed to `item` and `guidMap` is correct and complete. Missing or undefined values could result in the issues you're observing.
5. **Svelte Component Updates:**
- Svelte components are reactive, but if there's a problem with how `SvelteMarkdown` handles its `source` prop internally, it might not react to changes as expected. Review the documentation or source code of `SvelteMarkdown` to ensure it's designed to react to prop changes dynamically.
### Additional Tips
- **Use Svelte's Development Mode:** Run your Svelte app in development mode to get warnings and errors that can help identify issues like missing reactivity.
- **Immutable Updates:** Svelte's reactivity is triggered by assignments, so practicing immutable updates (assigning new objects/arrays rather than mutating existing ones) is crucial.
- **Check for External Changes:** If `item` or `guidMap` could be changed outside of Svelte's knowledge (e.g., by external libraries or callbacks), you might need to manually signal Svelte to recheck reactivity using methods like `set` from the Svelte store.
By ensuring proper reactivity and data handling, you can address issues with stale or missing data in your component.Will changes to props to a component force that component to re-render in Svelte?Yes, changes to props passed to a component in Svelte will force that component to re-render. Svelte's reactivity system is designed around the concept that a component should automatically update in response to changes in its state or props. When a prop value changes, Svelte's reactivity system detects the change and schedules the component for re-render to reflect the updated prop values in the DOM.
Here's how it works:
1. **Reactive Assignments:** In Svelte, the reactivity is based on assignments. When you assign a new value to a variable (or prop) that is being used in a component, Svelte automatically marks the component as "dirty" and schedules it for re-rendering. This ensures that the component's output reflects the latest state.
2. **Props as Reactive Variables:** Props in Svelte components are just JavaScript variables that hold values passed from a parent component. Since they are reactive, any change to these props (through reassignments in the parent component) triggers reactivity in the child component where the props are used.
3. **Component Updates:** When a prop changes, only the components that depend on those props (directly or indirectly) will re-render. This selective re-rendering is efficient and avoids unnecessary updates to parts of the application that haven't changed.
4. **Immutable Data Patterns:** Svelte encourages the use of immutable data patterns. When passing objects or arrays as props, it's a good practice to pass a new object/array rather than mutating the existing one. This makes it easier for Svelte to detect changes and update the component accordingly.
5. **Reactivity Caveats:** While Svelte's reactivity system is generally intuitive, there are cases where changes might not automatically trigger a re-render. For instance, mutating an object or array's content without assigning the variable to a new object or array (e.g., using methods like `push` on an array) won't automatically trigger reactivity. In such cases, you can trigger reactivity by re-assigning the variable to itself or using Svelte's store mechanisms for more complex state management.
In summary, yes, changes to props trigger re-renders in Svelte components. This behavior is a core part of Svelte's reactivity system, making it straightforward to build dynamic and responsive applications.
Reactivity Issue Resolution created at Tue Jan 20 1970 18:47:27 GMT+0000 (Coordinated Universal Time) Related Chats
Reactivity Issue Resolution 0.999
New chat 0.623
Svelte Component Help 0.618
Fix ExpandableGroupPanel Filter Error 0.562
Store Iteration Order Issue 0.552
SSR Error: HTMLElement Not Defined 0.536
Passing inputValue to slot 0.530
Value Prop Reactivity Issue 0.528
SvelteKit to NextJS: Migrate 0.519
Update Svelte Store Item 0.504