Python Server Streaming JSON
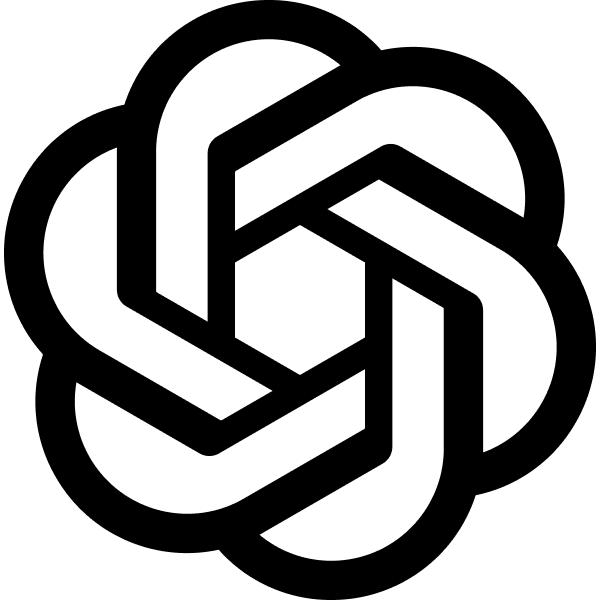
TODO: collapasable, copyable, download, etc
I have a python server that does a bunch of calculation and occasionally emits a JSON response to the POST request. I want to use Streaming JSON with newline seperation and flushing the buffer. Cna you write me a code sample in python that will be the basis for this pattern?Certainly! Below is a sample Python server using Flask that streams JSON responses with newline separation and flushes the buffer:
from flask import Flask, stream_with_context, Response
import json
import time
app = Flask(__name__)
@app.route('/stream', methods=['POST'])
def stream():
def generate():
data = [
{"id": 1, "message": "First message"},
{"id": 2, "message": "Second message"},
{"id": 3, "message": "Third message"}
]
for item in data:
# Simulate some computation
time.sleep(1)
# Emit the JSON data followed by a newline
yield json.dumps(item) + '\n'
# Here, the data gets flushed out to the client immediately
return Response(stream_with_context(generate()), content_type='application/json')
if __name__ == '__main__':
app.run(debug=True)
In this example:
- The server responds to POST requests at the
/stream
endpoint. - The
generate()
function inside thestream()
view simulates computations by sleeping for 1 second in between emitting JSON responses. - The server sends each JSON response followed by a newline character (
\n
). - The
stream_with_context()
function is used to ensure that the server streams the response to the client. The content type is set toapplication/json
. - When you POST to
/stream
, you should receive a stream of JSON responses with newline separation, one every second.
To test this, you can run the server and use a tool like curl
:
curl -X POST http://127.0.0.1:5000/stream
This will show you the streaming JSON responses.Can you update to handle if the user closes their browser, but we keep calcluating?
What should the mime-type be?To handle cases where the client (user's browser or another client) disconnects but the server is still calculating, you can check for flask.request.is_socket_closed
. If this is True
, it indicates that the client has disconnected.
As for the mime-type, when dealing with newline-delimited JSON, the more appropriate MIME type is application/x-ndjson
. This stands for "newline-delimited JSON".
Here's the updated code with the required changes:
from flask import Flask, stream_with_context, Response, request
import json
import time
app = Flask(__name__)
@app.route('/stream', methods=['POST'])
def stream():
def generate():
data = [
{"id": 1, "message": "First message"},
{"id": 2, "message": "Second message"},
{"id": 3, "message": "Third message"}
]
for item in data:
# Check if the client has disconnected
if request.is_socket_closed:
print("Client disconnected, stopping calculations.")
break
# Simulate some computation
time.sleep(1)
# Emit the JSON data followed by a newline
yield json.dumps(item) + '\n'
return Response(stream_with_context(generate()), content_type='application/x-ndjson')
if __name__ == '__main__':
app.run(debug=True)
With this code:
- The server checks if the client has disconnected using
request.is_socket_closed
before proceeding with further calculations. - The content type is set to
application/x-ndjson
.
Again, you can test this with curl
:
curl -X POST http://127.0.0.1:5000/stream
If you interrupt the curl
command before it completes, the server will print "Client disconnected, stopping calculations." and halt further calculations.
Related Chats
Python Server Streaming JSON 1.000
Node.js POST Request Stream 0.480
Stream REST Data in JavaScript 0.472
Designing Web AI Chat 0.465
Extract `key` and `value`. 0.395
New chat 0.375
Streaming JSON Parsing Issue 0.372
Non-Streaming vs Streaming Responses 0.363
Learn `jq` Basics 0.351