Stream REST Data in JavaScript
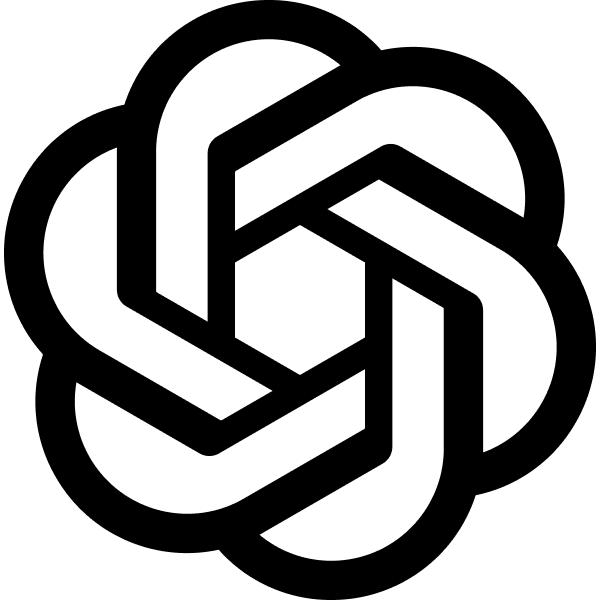
TODO: collapasable, copyable, download, etc
In JavaScript, how can I consume a REST endpoint as a stream of JSON objects, where the overall response may take a tens of seconds, but I can update the UI incrementallly?When dealing with streams of data in JavaScript, especially in the context of the web, the Streams API is a tool that you should be familiar with. With the Fetch API and ReadableStream, you can process a response incrementally.
Here's a general outline on how you can achieve this:
- Use the Fetch API to make a request to your endpoint.
- Access the response body as a ReadableStream.
- Process the stream incrementally.
- Update the UI as each chunk of the response is received and processed.
Here's an example that demonstrates consuming a REST endpoint as a stream of JSON objects:
// Make a fetch request to the endpoint.
fetch('https://example.com/data-endpoint')
.then(response => {
// Ensure that the content type is correct before processing as a stream.
const contentType = response.headers.get('content-type');
if (!contentType || !contentType.includes('application/json')) {
throw new TypeError('Expected a content type of application/json.');
}
// Obtain the readable stream of the response.
const reader = response.body.getReader();
// Create a new TextDecoder to decode the stream chunks.
const decoder = new TextDecoder();
let dataBuffer = '';
// Function to handle each chunk of data.
function handleChunk({ done, value }) {
if (done) {
console.log('Stream finished.');
return;
}
// Decode the chunk and append to the buffer.
dataBuffer += decoder.decode(value, { stream: true });
// Check for complete JSON objects in the buffer.
let endIndex;
while ((endIndex = dataBuffer.indexOf('\n')) >= 0) { // assuming newline-delimited JSON
const jsonString = dataBuffer.slice(0, endIndex);
dataBuffer = dataBuffer.slice(endIndex + 1);
try {
const jsonObject = JSON.parse(jsonString);
// Update the UI with jsonObject here.
console.log(jsonObject);
} catch (err) {
console.error('Error parsing JSON:', err);
}
}
// Read the next chunk.
return reader.read().then(handleChunk);
}
// Start reading the stream.
return reader.read().then(handleChunk);
})
.catch(err => {
console.error('Fetch error:', err);
});
A few things to note:
- This example assumes that the server sends newline-delimited JSON objects. Adjust your parsing strategy if your server uses a different approach.
- Error handling is crucial. If any part of the process fails, it can lead to incomplete data processing.
- Streams are powerful, but make sure you understand their behavior and potential pitfalls.
Remember, the efficiency of this approach also depends on how the server sends data. If it sends data in large chunks, you may not be able to achieve a smooth, incremental UI update.Can you give me an example of how the server side code would be written to emit proper streaming JSON?Certainly! Streaming JSON typically involves sending multiple JSON objects sequentially, sometimes separated by newline characters. The key is to flush the response buffer intermittently, ensuring that data is sent to the client incrementally, rather than all at once at the end.
Here's an example using Node.js with the Express framework to send newline-delimited JSON objects:
- Setup
Install the necessary packages:
npm init -y
npm install express
- Server Code
const express = require('express');
const app = express();
const PORT = 3000;
app.get('/data-endpoint', (req, res) => {
// Set the content type to JSON and set appropriate headers for streaming.
res.setHeader('Content-Type', 'application/json');
res.setHeader('Cache-Control', 'no-cache');
res.setHeader('Connection', 'keep-alive');
// Generate and send 10 JSON objects, one every 2 seconds.
let count = 0;
const interval = setInterval(() => {
const data = {
id: count,
message: `Data object number ${count}`
};
// Send the JSON string followed by a newline.
res.write(JSON.stringify(data) + '\n');
count++;
// After 10 objects, clear the interval and end the response.
if (count >= 10) {
clearInterval(interval);
res.end();
}
}, 2000); // 2 seconds delay
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
This server sends 10 JSON objects, with a 2-second interval between each object. The client will receive each JSON object as soon as it's written to the response, thanks to the streaming nature of the server's response.
- Considerations & Tips
- The use of newline-delimited JSON (NDJSON) is just one approach. There are other formats, such as JSON Text Sequences, that you might consider based on your needs.
- Always ensure that the content type and other headers are set appropriately. This helps clients understand the format of your streaming response.
- When dealing with real-time data, consider using technologies specifically designed for real-time communication, such as WebSockets or Server-Sent Events.
- Monitor and handle backpressure, especially when dealing with large amounts of data. In Node.js, streams naturally handle backpressure, but you should be aware of its implications when working with custom streaming solutions.
With the server and client set up as shown, your client-side code should be able to consume the server's streaming JSON response incrementally.Does nodejs exsure that this response buffer will flush? res.write(JSON.stringify(data) + '\n');
In Node.js, the behavior of res.write()
in the context of an HTTP server (as provided by the built-in http
module or by Express, which builds upon it) is to send chunks of data to the client as soon as they are written. The behavior is somewhat dependent on the size of the chunks and the underlying OS buffer. Small chunks might not be immediately sent due to the OS's TCP Nagle algorithm, which may buffer small packets. This is to reduce the overhead of sending many tiny packets over the network.
However, in the example provided, the chunks being written with res.write(JSON.stringify(data) + '\n');
will typically be flushed pretty quickly, especially given the 2-second interval between writes. The newline ('\n'
) is not significant in terms of flushing; it's there to delimit the JSON objects for easier parsing on the client side.
If you want to ensure that data is flushed immediately and bypass the TCP Nagle algorithm, you can use the socket.setNoDelay(true)
method on the underlying socket. With Express, you can access this via req.connection.setNoDelay(true)
.
For example:
app.get('/data-endpoint', (req, res) => {
// Disable Nagle's algorithm to ensure immediate flush.
req.connection.setNoDelay(true);
// ... rest of your code ...
});
However, keep in mind that disabling Nagle's algorithm could increase the network overhead if you're sending many small packets. In the context of the example given, with 2-second intervals between writes, disabling the algorithm might not have any noticeable benefit, but it's a tool you can use if more immediate flushing is necessary.
Stream REST Data in JavaScript created at Tue Jan 20 1970 14:55:43 GMT+0000 (Coordinated Universal Time)Related Chats
Stream REST Data in JavaScript 0.999
Node.js POST Request Stream 0.632
New chat 0.560
Metrics Middleware for Next.js 0.496
Popular NodeJS HTTP Libraries 0.486
Designing Web AI Chat 0.474
Python Server Streaming JSON 0.472
DB Connection Middleware Setup 0.461
Fetch Debouncing with JavaScript 0.452
Next.js Middleware & HOC 0.427