Node.js POST Request Stream
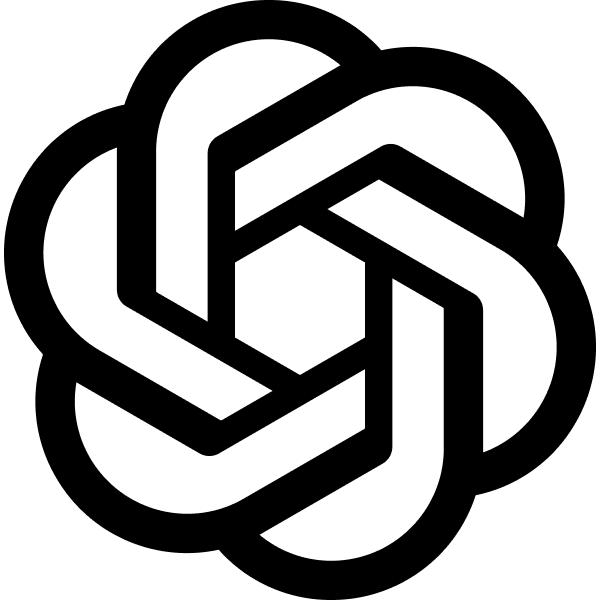
TODO: collapasable, copyable, download, etc
Can you give me a nodejs code sample using stream-chain, parser from steam-json where I made a post request to an endpoint and then execute code on each data callback?Certainly! To accomplish this, we will use the stream-chain
, stream-json
, http
, and possibly other helper libraries to parse a JSON stream received from a POST request. Here is an example of how you might set this up:
First, install the necessary packages if you haven't already:
npm install stream-chain stream-json axios
Below is an example of Node.js code that:
- Makes a POST request to an endpoint using
axios
. - Receives a stream of JSON data.
- Uses
stream-chain
andstream-json
to parse the JSON stream. - Executes code on each data callback.
const { chain } = require('stream-chain');
const { parser } = require('stream-json');
const { streamValues } = require('stream-json/streamers/StreamValues');
const axios = require('axios');
const { PassThrough } = require('stream');
const postData = {
// your POST data here
};
const config = {
headers: {
'Content-Type': 'application/json',
},
responseType: 'stream', // This tells axios to receive a stream
};
axios.post('https://your-endpoint.com', postData, config)
.then(response => {
// The response is a stream now
const pipeline = chain([
response.data, // the axios response stream
parser(), // stream-json will parse the incoming JSON stream
streamValues(), // this will ignore all the non-data JSON nodes
]);
const pass = new PassThrough({ objectMode: true });
pipeline.on('data', data => {
const value = data.value; // the parsed data object
// Execute your code for each object here
console.log(value);
// If you want to write it to the pass-through stream
pass.write(value);
});
pipeline.on('end', () => {
console.log('All data processed');
pass.end();
});
pipeline.on('error', err => {
console.error('Error processing data:', err);
pass.destroy(err);
});
// If you want to use the pass-through stream elsewhere
// return pass;
}).catch(error => {
console.error('Request failed:', error);
});
In this code:
- We're sending a POST request using axios with a configuration that specifies a stream response.
- We create a stream chain with the
response.data
as the source, then parse the JSON stream, and stream the values. - Each data event on the pipeline will trigger the callback where you can handle the parsed data.
- We use a
PassThrough
stream in case you want to pass the processed data somewhere else (like another stream).
Remember to replace 'https://your-endpoint.com'
with your actual endpoint and include the appropriate POST data.
This is a simplified example, and in a production environment, you might need to handle edge cases, authentication, stream backpressure, and more sophisticated error handling.Is it worth using axiom? Is there a nodejs standard library that will work with steam-chainI'm using import syntax, can you re-write this? const { chain } = require('stream-chain'); const { parser } = require('stream-json'); const { streamValues } = require('stream-json/streamers/StreamValues'); const axios = require('axios'); const { PassThrough } = require('stream');
Certainly! If you're using the ES6 import
syntax, you would convert the require
statements into import
like this:
import { chain } from 'stream-chain';
import { parser } from 'stream-json';
import { streamValues } from 'stream-json/streamers/StreamValues.js';
import axios from 'axios';
import { PassThrough } from 'stream';
Make sure that your environment supports ES6 module syntax or that you are using a transpiler like Babel, or a module bundler like Webpack. Node.js supports ES modules with .mjs
extension, or "type": "module"
in the package.json
.
Remember to check the documentation for each package, as some might have default exports instead of named exports, depending on their update and ES6 support. If you encounter issues, you might need to adjust the import statements to match the actual exports of the libraries you're using.I am getting Request failed: Error: Arguments should be functions, arrays or streams. at /Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:123:15 at Array.map (<anonymous>) at new Chain (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:118:8) at Proxy.make (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:169:12) at eval (/Users/ozten/Projects/troubleshooter/app/src/routes/v2/infer/create_session/+server.js:52:42) at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
I have this code const pipeline = chain([ response.data, // the axios response stream parser(), // stream-json will parse the incoming JSON stream streamValues(), // this will ignore all the non-data JSON nodes ]);
The chain
import looks good.
If I console.log(typeof response.data, Object.keys(response.data));
I get string [ '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24', '25', '26', '27', '28', '29', '30', '31', '32', '33', '34', '35', '36', '37', '38', '39', '40', '41', '42', '43', '44', '45', '46', '47', '48', '49', '50', '51', '52', '53', '54', '55', '56', '57', '58', '59', '60', '61', '62', '63', '64', '65', '66', '67', '68', '69', '70', '71', '72', '73', '74', '75', '76', '77', '78', '79', '80', '81', '82', '83', '84', '85', '86', '87', '88', '89', '90', '91', '92', '93', '94', '95', '96', '97', '98', '99', ... 247 more items ]
I am getting Request failed: Error: Arguments should be functions, arrays or streams. at /Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:123:15 at Array.map (<anonymous>) at new Chain (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:118:8) at Proxy.make (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-chain/index.js:169:12) at eval (/Users/ozten/Projects/troubleshooter/app/src/routes/v2/infer/create_session/+server.js:52:42) at process.processTicksAndRejections (node:internal/process/task_queues:95:5)
The error message you are seeing suggests that there's an issue with the arguments being passed to chain
from the stream-chain
package. It expects functions, arrays, or streams, but it seems like it might be receiving something else. This could be due to changes or incompatibilities in the version of the stream-chain
or related packages you are using.
The error might also be due to the way imports are handled. Let's first ensure that the imports are done correctly and if the error persists, we can look into other possible causes.
Here's the corrected import statement taking into account default and named exports:
import { Chain } from 'stream-chain';
import { parser } from 'stream-json';
import { streamValues } from 'stream-json/streamers/StreamValues.js';
import axios from 'axios';
import { PassThrough } from 'stream';
And the usage would be slightly different because chain
is not a function but a class. You need to use the new
keyword to create an instance:
axios.post('https://your-endpoint.com', postData, config)
.then(response => {
// The response is a stream now
const pipeline = new Chain([
response.data, // the axios response stream
parser(), // stream-json will parse the incoming JSON stream
streamValues(), // this willBased on your console output and the error message, it seems that `response.data` is being interpreted as a string, not a stream. When you're expecting a stream but you get a string, it indicates that the response from the server is not being treated as a stream by axios.
Normally, axios should provide a stream when you set `responseType: 'stream'` in the request configuration, so let's check that part of the code to ensure it is set up correctly.
Here's how you should configure your axios request:
```javascript
const config = {
headers: {
'Content-Type': 'application/json',
},
responseType: 'stream', // This tells axios to receive a stream
};
axios.post('https://your-endpoint.com', postData, config)
.then(response => {
// The rest of your stream handling code...
})
.catch(error => {
console.error('Request failed:', error);
});
If you have this configuration set and you're still receiving a string, you may need to verify the following:
- Check the server's response to ensure it's actually sending a stream. You might be getting a stringified JSON instead of a raw stream.
- Make sure you are using a recent version of axios that supports the
responseType: 'stream'
configuration.
If everything seems correct but the issue persists, as a debugging step, try logging response.headers
to see the content type of the response. It's possible that the server is sending a different content type than expected.
If you have confirmed that the server sends a stream and axios is up-to-date, but the problem still occurs, you can try creating a stream from the string as a workaround:
import { Readable } from 'stream';
// Inside your axios `then` callback...
const stream = Readable.from(response.data);
const pipeline = chain([
stream, // Create a stream from the string
parser(), // stream-json will parse the incoming JSON stream
streamValues() // this will ignore all the non-data JSON nodes
]);
This workaround converts the string back into a stream, which you can then pass to the chain. But ideally, you should resolve why the response is not being treated as a stream by axios, as it's more efficient to handle streaming directly from the HTTP response.In nodejs how do I console.log a Buffer as a utf8 string?In Node.js, if you have a Buffer
object and want to log it as a UTF-8 string, you can call the .toString()
method on the Buffer, specifying 'utf8'
as the encoding. UTF-8 is actually the default encoding for .toString()
, so even if you don't specify it, the Buffer will be converted to a string assuming UTF-8 encoding.
Here is how you would do it:
const buffer = Buffer.from('Hello World', 'utf8');
console.log(buffer.toString('utf8')); // "Hello World"
Or simply:
console.log(buffer.toString()); // "Hello World"
If you have a buffer that you received and you're not sure what encoding it uses, you should be cautious, as assuming the wrong encoding could result in incorrect characters being displayed. However, if you're certain that the content is UTF-8 encoded, then using .toString()
without an encoding parameter will give you the string representation of the buffer.Here is the latest code for my pipeline ```const pipeline = chain([
response.data, // the axios response stream
(x) => {
console.log('AOK', typeof x, x.toString('utf8'));
return x;
},
streamValues(), // this will ignore all the non-data JSON nodes
parser(), // stream-json will parse the incoming JSON stream
]);
// const pass = new PassThrough({ objectMode: true });
pipeline.on('data', data => {
const value = data.value; // the parsed data object
// Execute your code for each object here
console.log(value);
// If you want to write it to the pass-through stream
// pass.write(value);
});
pipeline.on('end', () => {
console.log('All data processed');
// pass.end();
});
pipeline.on('error', err => {
console.error('Error processing data:', err);
// pass.destroy(err);
});```
But I am seeing the error codepath trigger. Any way to fix this?
"GUID": "a6a43ca6-044d-4436-a2f0-1e3d5310433c",
"type": "envelope",
"sessionId": "456"
}
AOK object {
"data": [
{
"text": {
"GUID": "5ef5bad2-0722-437b-b44b-315e6de134d7",
"type": "message",
"timestamp": "1699383559102",
"message": "Chat session created.",
"confidence": 99.9
}
}
]
}
Error processing data: Error: Parser has expected a value
at Parser._processBuffer (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/Parser.js:112:31)
at Parser._flush (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/utils/Utf8Stream.js:49:10)
at Parser._flush (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/Parser.js:83:11)
at Parser.final [as _final] (node:internal/streams/transform:132:10)
at callFinal (node:internal/streams/writable:707:12)
at prefinish (node:internal/streams/writable:719:7)
at finishMaybe (node:internal/streams/writable:729:5)
at Writable.end (node:internal/streams/writable:641:5)
at StreamValues.onend (node:internal/streams/readable:724:10)
at Object.onceWrapper (node:events:628:28)```Here is the latest code for my pipeline ```const pipeline = chain([
response.data, // the axios response stream
(x) => {
console.log('AOK', typeof x, x.toString('utf8'));
return x;
},
streamValues(), // this will ignore all the non-data JSON nodes
parser(), // stream-json will parse the incoming JSON stream
]);
// const pass = new PassThrough({ objectMode: true });
pipeline.on('data', data => {
const value = data.value; // the parsed data object
// Execute your code for each object here
console.log(value);
// If you want to write it to the pass-through stream
// pass.write(value);
});
pipeline.on('end', () => {
console.log('All data processed');
// pass.end();
});
pipeline.on('error', err => {
console.error('Error processing data:', err);
// pass.destroy(err);
});```
I know that the streaming response looks like this ```{
"GUID": "68094310-f7d1-4802-b852-71fa7046a36e",
"type": "envelope",
"sessionId": "456"
}```
wait one second and then ```
{
"data": [
{
"text": {
"GUID": "39f86d2c-653b-4238-b95a-0653579fda3e",
"type": "message",
"timestamp": "1699383709441",
"message": "Chat session created.",
"confidence": 99.9
}
}
]
}```
But I am seeing the error codepath trigger. Any way to fix this?
```AOK object {
"GUID": "a6a43ca6-044d-4436-a2f0-1e3d5310433c",
"type": "envelope",
"sessionId": "456"
}
AOK object {
"data": [
{
"text": {
"GUID": "5ef5bad2-0722-437b-b44b-315e6de134d7",
"type": "message",
"timestamp": "1699383559102",
"message": "Chat session created.",
"confidence": 99.9
}
}
]
}
Error processing data: Error: Parser has expected a value
at Parser._processBuffer (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/Parser.js:112:31)
at Parser._flush (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/utils/Utf8Stream.js:49:10)
at Parser._flush (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/Parser.js:83:11)
at Parser.final [as _final] (node:internal/streams/transform:132:10)
at callFinal (node:internal/streams/writable:707:12)
at prefinish (node:internal/streams/writable:719:7)
at finishMaybe (node:internal/streams/writable:729:5)
at Writable.end (node:internal/streams/writable:641:5)
at StreamValues.onend (node:internal/streams/readable:724:10)
at Object.onceWrapper (node:events:628:28)```The error message "Error: Parser has expected a value" from `stream-json` suggests that the parser encountered something other than valid JSON when it tried to parse the incoming stream. This can happen if the stream's content isn't well-formed JSON.
There are a few potential issues with the pipeline setup:
1. The order of the stream processors is incorrect. The parser should be directly after the raw input stream and before `streamValues()`. The `streamValues()` should be processing the output of the parser, not the other way around.
2. You are logging with `x.toString('utf8')`, which is not necessary and may be problematic if `x` is not a Buffer or a string.
3. Inserting the logging function directly in the chain might unintentionally alter the stream's data, as any function in the chain is expected to return a transformed chunk or a readable stream.
Here's the corrected pipeline:
```javascript
const pipeline = chain([
response.data, // the axios response stream
parser(), // stream-json will parse the incoming JSON stream
streamValues(), // this will ignore all the non-data JSON nodes
data => {
// Now you can handle each piece of data:
console.log('Value:', data.value);
return data;
}
]);
pipeline.on('data', data => {
// data.value is already the parsed data object from streamValues()
console.log('Data object:', data.value);
});
pipeline.on('end', () => {
console.log('All data processed');
});
pipeline.on('error', err => {
console.error('Error processingThe error message you're encountering, "Parser has expected a value," suggests that the `stream-json` parser is receiving some input that it does not recognize as a valid JSON value at some point in the stream.
The correct order in the chain should be to first parse the raw JSON chunks into JSON tokens and then stream the values:
```javascript
const pipeline = chain([
response.data, // the axios response stream
parser(), // stream-json will parse the incoming JSON stream
streamValues(), // this will ignore all the non-data JSON nodes
// any additional processing or transformation streams would go here
]);
You've reversed streamValues()
and parser()
in your pipeline setup, which is likely the cause of the error. The parser()
should come before streamValues()
because you first need to parse the raw data into JSON tokens, and then streamValues()
can work on those tokens to extract the values.
The purpose of streamValues()
is to take the tokenized JSON produced by the parser and convert it into JavaScript objects. It expects the tokens from the parser, not the raw stream.
Here's how you can fix the pipeline:
const pipeline = chain([
response.data, // the axios response stream
parser(), // stream-json will parse the incoming JSON stream
streamValues(), // this will ignore all the non-data JSON nodes
]);
Then, your event handlers should work as intended, handling each parsed data object:
pipeline.on('data', data => {
const value = data.value; // the parsed data object
console.log(value);
});
pipeline.on('end', () => {
console.log('All data processed');
});
pipeline.on('error', err => {
console.error('Error processing data:', err);
});
With this corrected chain order, your code should process the streaming JSON correctly without encountering the parser error.If I do that, I get this error Error processing data: Error: Parser cannot parse input: expected a value at Parser._processBuffer (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/Parser.js:111:64) at Parser._transformBuffer (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/utils/Utf8Stream.js:24:10) at Parser._transform (/Users/ozten/Projects/troubleshooter/app/node_modules/stream-json/utils/Utf8Stream.js:19:10) at Transform._write (node:internal/streams/transform:175:8) at writeOrBuffer (node:internal/streams/writable:399:12) at _write (node:internal/streams/writable:340:10) at Writable.write (node:internal/streams/writable:344:10) at IncomingMessage.ondata (node:internal/streams/readable:785:22) at IncomingMessage.emit (node:events:514:28) at Readable.read (node:internal/streams/readable:558:10)
The error message you're getting now indicates that the parser is receiving data that it does not recognize as valid JSON. This can happen for several reasons:
- The JSON is not well-formed or has errors (e.g., missing commas, brackets, etc.).
- The data chunks received by the parser are not complete JSON elements, which can occur if the stream is split at arbitrary points.
- The data stream might be encoded incorrectly or have leading/trailing characters that are not part of the JSON.
Here's what you can try:
- Ensure the response is indeed JSON and check for JSON formatting issues.
- Log the raw buffer before it gets to the parser to verify that what's being received is valid JSON.
- Ensure the server is sending the response with
Content-Type: application/json
.
Let's update the pipeline to include a raw buffer logging step before parsing to diagnose the issue:
import { chain } from 'stream-chain';
import { parser } from 'stream-json';
import { streamValues } from 'stream-json/streamers/StreamValues.js';
import { Writable } from 'stream';
// Assuming response.data is a readable stream
const pipeline = chain([
response.data,
data => {
// Only log if data is a Buffer
if (Buffer.isBuffer(data)) {
const rawJson = data.toString('utf8');
console.log('Raw JSON:', rawJson);
}
return data;
},
parser(),
streamValues()
]);
const writable = new Writable({
objectMode: true,
write(chunk, encoding, callback) {
console.log('Value:', chunk.value);
callback();
}
});
pipeline.on('data', data => {
// No need to do anything here, as the Writable handles the data.
});
pipeline.on('end', () => {
console.log('All data processed');
});
pipeline.on('error', err => {
console.error('Error processing data:', err);
});
// Pipe the output of the pipeline into the writable stream to process each data chunk
pipeline.pipe(writable);
The Writable
stream is where you can process each JSON value. It is part of the pipeline after streamValues()
, which ensures that each chunk is a complete JSON value.
Make sure to check the raw JSON log. If you see a complete JSON object logged and still receive the error when it reaches the parser, then the issue might be within the stream-json
parser handling. If the raw JSON is not well-formed, you'll need to fix the source of the JSON or handle the malformed JSON in some way before it reaches the parser.
Related Chats
Stream REST Data in JavaScript 0.632
Streaming JSON Parsing Issue 0.583
Popular NodeJS HTTP Libraries 0.574
New chat 0.523
Metrics Middleware for Next.js 0.522
Python Server Streaming JSON 0.480
SvelteKit REST Proxy 0.469
DB Connection Middleware Setup 0.463
Designing Web AI Chat 0.449