Smoothly Animate Value with Timestamp
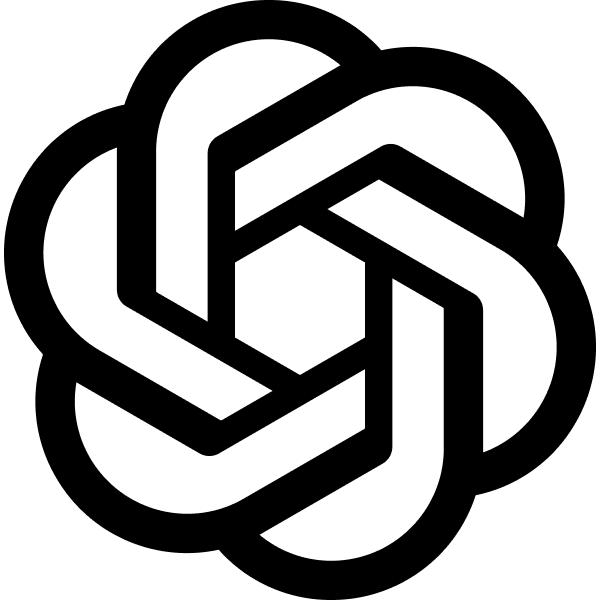
TODO: collapasable, copyable, download, etc
I have a timestamp that is always increasing. I want to animate a value smoothly up and down between 0 and 100. How could I use JS to turn that timesteamp into the value?To smoothly animate a value between 0 and 100 based on a continuously increasing timestamp in JavaScript, you can use the Math.sin
or Math.cos
functions to create a smooth oscillation effect. Here's an example of how you can do this:
function smoothAnimation(timestamp) {
// Define animation parameters
const duration = 2000; // Total duration for one full cycle (in milliseconds)
const minValue = 0;
const maxValue = 100;
// Calculate the current progress within the cycle
const progress = (timestamp % duration) / duration;
// Calculate the value based on the progress using Math.sin or Math.cos
const value = (Math.sin(2 * Math.PI * progress) + 1) / 2; // Use Math.sin for a smooth oscillation
// Map the value to the desired range (0 to 100)
const animatedValue = minValue + (maxValue - minValue) * value;
// Use the animatedValue for your animation
console.log(animatedValue);
// Request the next frame for continuous animation
requestAnimationFrame(smoothAnimation);
}
// Start the animation loop
requestAnimationFrame(smoothAnimation);
In this code, smoothAnimation
is a recursive function that continuously updates the animatedValue
based on the timestamp and then requests the next frame using requestAnimationFrame
to create a smooth animation loop. The use of Math.sin
or Math.cos
creates a smooth oscillation effect between 0 and 1, which is then mapped to the desired range of 0 to 100. You can adjust the duration
variable to control the speed of the animation.
Remember to customize the animation parameters and use animatedValue
in your application as needed.How would you alter this to have a second variable that is 33% ahead of animatedValue in the cycle?To create a second variable that lags behind animatedValue
by 33% of the cycle, you can calculate another value based on the timestamp but with a phase difference. You can achieve this by using Math.sin
or Math.cos
with a phase shift. Here's how you can modify the code to include a second variable:
function smoothAnimation(timestamp) {
// Define animation parameters
const duration = 2000; // Total duration for one full cycle (in milliseconds)
const minValue = 0;
const maxValue = 100;
const phaseShift = (2 * Math.PI) / 3; // Phase shift for the second variable (33% ahead)
// Calculate the current progress within the cycle
const progress = (timestamp % duration) / duration;
// Calculate the value based on the progress using Math.sin for the first variable
const value = (Math.sin(2 * Math.PI * progress) + 1) / 2;
// Calculate the value for the second variable with a phase shift
const value2 = (Math.sin(2 * Math.PI * progress + phaseShift) + 1) / 2;
// Map the values to the desired range (0 to 100)
const animatedValue = minValue + (maxValue - minValue) * value;
const secondAnimatedValue = minValue + (maxValue - minValue) * value2;
// Use animatedValue and secondAnimatedValue for your animation
console.log("animatedValue:", animatedValue);
console.log("secondAnimatedValue:", secondAnimatedValue);
// Request the next frame for continuous animation
requestAnimationFrame(smoothAnimation);
}
// Start the animation loop
requestAnimationFrame(smoothAnimation);
In this modified code, value2
is calculated using the same approach as value
, but with a phaseShift
of (2 * Math.PI) / 3
, which corresponds to a 33% phase shift in a full cycle. This results in secondAnimatedValue
lagging 33% behind animatedValue
in the oscillation cycle. You can customize the phase shift as needed for your specific requirements.
Related Chats
Smoothly Animate Value with Timestamp 1.000
Luxon for Human-Friendly Durations 0.414
Node.js Monte Carlo Simulation 0.391
Aggregate Highcharts data in 5-min. 0.383
JS Store Update Syntax 0.352
Mock `console.error` test. 0.328
Scale Data to New Range 0.324
Highlighting Graph Area 0.318