Mock `console.error` test.
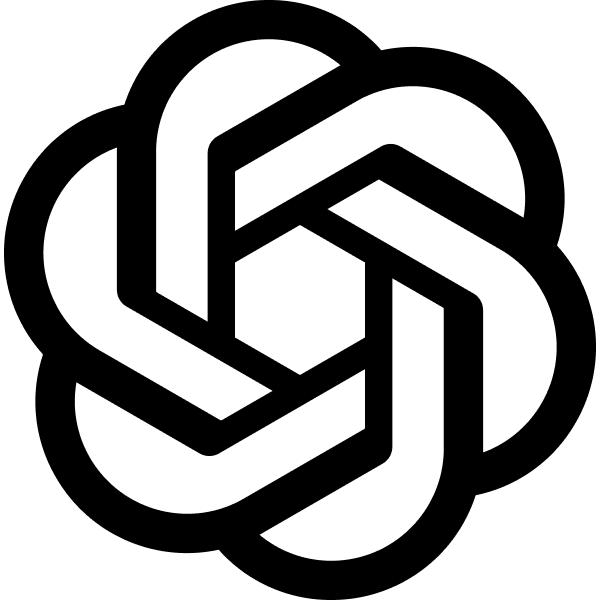
TODO: collapasable, copyable, download, etc
Using vitest
, Can you mock out console.warn
and unit test this code?
* Copyright (c) 2023, Deductive AI, Inc. All rights reserved.
*
* This software is the confidential and proprietary information of
* Deductive AI, Inc. You shall not disclose such confidential
* information and shall use it only in accordance with the terms of
* the license agreement you entered into with Deductive AI, Inc.
*/
// Returns a timestamp in seconds, a little bit in the future (30 minutes)
const nowishSeconds() = () => Math.round(new Date().getTime() / 1000) + (60 * 30);
// Timestamp in seconds for 3 years prior to server startup
const PRIOR_3_YEARS_SECONDS = Math.round(new Date().getTime() / 1000) - (3 * 356 * 24 * 60 * 60);
/**
* Log a warning if millisecond timestamp is way to early or too far in the future to be reasonable
* @param {*} ctx Caller provided string for context
* @param {*} timestamp
* @returns
*/
export const isBadMilliseconds = (ctx, timestamp) => {
if (typeof timestamp != 'number') {
console.error(
'Timespan assertion failed, unit in millis NOT A NUMBER _',
ctx,
'_',
timestamp,
new Error('stack trace').stack
);
return;
}
if (timestamp < PRIOR_3_YEARS_SECONDS * 1000) {
console.error(
'Timespan assertion failed, unit in millis too low _',
ctx,
'_',
timestamp,
'=',
new Date(timestamp).toUTCString()
);
} else if (timestamp > nowishSeconds() * 1000) {
console.error(
'Timespan assertion failed, unit in millis too hight_',
ctx,
'_',
timestamp,
'=',
new Date(timestamp).toUTCString()
);
}
};
/**
* Log a warning if seconds timestamp is way to early or too far in the future to be reasonable
* @param {*} ctx
* @param {*} timestamp
* @returns
*/
export const isBadSeconds = (ctx, timestamp) => {
if (typeof timestamp != 'number') {
console.error(
'Timespan assertion failed, unit in seconds NOT A NUMBER _',
ctx,
'_',
timestamp,
new Error('stack trace').stack
);
return;
}
if (timestamp < PRIOR_3_YEARS_SECONDS) {
console.error(
'Timespan assertion failed, unit in seconds too low _',
ctx,
'_',
timestamp,
'=',
new Date(timestamp * 1000).toUTCString()
);
} else if (timestamp > nowishSeconds()) {
console.error(
'Timespan assertion failed, unit in seconds too hight _',
ctx,
'_',
timestamp,
'=',
new Date(timestamp * 1000).toUTCString()
);
}
};
```To unit test the code you provided using `vitest`, we will mock `console.error` (since your code uses `console.error` and not `console.warn` as mentioned in your question) and create tests for different scenarios to verify the behavior of both `isBadMilliseconds` and `isBadSeconds` functions. These scenarios include passing timestamps that are:
1. Not a number
2. Too low (more than 3 years in the past)
3. Too high (more than 30 minutes in the future)
4. Within the acceptable range
First, let's set up the mocking for `console.error` using `vitest`. We'll then write the tests. Note that `vitest` provides the `vi` object to create mocks.
Here's an example test suite for your code:
```javascript
import { describe, it, expect, beforeEach, vi } from 'vitest';
import { isBadMilliseconds, isBadSeconds } from './yourModulePath'; // Adjust the import path as necessary
describe('Timestamp validation tests', () => {
// Mock console.error before each test
beforeEach(() => {
vi.spyOn(console, 'error').mockImplementation(() => {});
});
afterEach(() => {
vi.restoreAllMocks();
});
describe('isBadMilliseconds', () => {
it('should log an error for non-numeric timestamps', () => {
isBadMilliseconds('testContext', 'not-a-number');
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the past', () => {
const tooOldTimestamp = new Date().getTime() - (4 * 365 * 24 * 60 * 60 * 1000); // 4 years ago
isBadMilliseconds('testContext', tooOldTimestamp);
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the future', () => {
const tooFutureTimestamp = new Date().getTime() + (2 * 60 * 60 * 1000); // 2 hours in the future
isBadMilliseconds('testContext', tooFutureTimestamp);
expect(console.error).toHaveBeenCalled();
});
it('should not log an error for reasonable timestamps', () => {
const reasonableTimestamp = new Date().getTime() + (10 * 60 * 1000); // 10 minutes in the future
isBadMilliseconds('testContext', reasonableTimestamp);
expect(console.error).not.toHaveBeenCalled();
});
});
describe('isBadSeconds', () => {
it('should log an error for non-numeric timestamps', () => {
isBadSeconds('testContext', 'not-a-number');
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the past', () => {
const tooOldTimestampSeconds = Math.round(new Date().getTime() / 1000) - (4 * 365 * 24 * 60 * 60); // 4 years ago
isBadSeconds('testContext', tooOldTimestampSeconds);
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the future', () => {
const tooFutureTimestampSeconds = Math.round(new Date().getTime() / 1000) + (2 * 60 * 60); // 2 hours in the future
isBadSeconds('testContext', tooFutureTimestampSeconds);
expect(console.error).toHaveBeenCalled();
});
it('should not log an error for reasonable timestamps', () => {
const reasonableTimestampSeconds = Math.round(new Date().getTime() / 1000) + (10 * 60); // 10 minutes in the future
isBadSeconds('testContext', reasonableTimestampSeconds);
expect(console.error).not.toHaveBeenCalled();
});
});
});
Ensure you have vitest
installed and properly configured in your project to run these tests. This test suite uses the vi.spyOn
method to mock console.error
and expect
to assert that it was called or not called under different conditions. The afterEach
block restores the original console.error
function after each test to prevent mock leakage between tests. Adjust the import path for your module as necessary. FAIL src/lib/bad_time.test.js [ src/lib/bad_time.test.js ]
Error: Expected a semicolonThe error message you encountered, "Expected a semicolon," usually indicates a syntax error in your JavaScript code. In the test suite example I provided, there might have been an oversight or typo that led to this issue. Let's review the test code for missing semicolons or other syntax errors.
Upon reviewing the example code, I noticed there's a mistake in the function declaration for nowishSeconds
in your original code snippet—it was missing the function
keyword, which could cause syntax issues if copied directly into your test environment. However, the test suite itself should not have resulted in a semicolon-related syntax error as provided.
Here's a corrected version of the initial setup for your code (in case it's directly related to the test suite execution), and I'll make sure the test suite syntax is correct:
// Correcting the original function declaration
const nowishSeconds = () => Math.round(new Date().getTime() / 1000) + (60 * 30);
// Assuming the rest of your original code remains the same
And here's the test suite again with careful attention to semicolons and syntax:
import { describe, it, expect, beforeEach, afterEach, vi } from 'vitest';
import { isBadMilliseconds, isBadSeconds } from './yourModulePath'; // Adjust the import path as necessary
describe('Timestamp validation tests', () => {
beforeEach(() => {
vi.spyOn(console, 'error').mockImplementation(() => {});
});
afterEach(() => {
vi.restoreAllMocks();
});
describe('isBadMilliseconds', () => {
it('should log an error for non-numeric timestamps', () => {
isBadMilliseconds('testContext', 'not-a-number');
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the past', () => {
const tooOldTimestamp = new Date().getTime() - (4 * 365 * 24 * 60 * 60 * 1000); // 4 years ago
isBadMilliseconds('testContext', tooOldTimestamp);
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the future', () => {
const tooFutureTimestamp = new Date().getTime() + (2 * 60 * 60 * 1000); // 2 hours in the future
isBadMilliseconds('testContext', tooFutureTimestamp);
expect(console.error).toHaveBeenCalled();
});
it('should not log an error for reasonable timestamps', () => {
const reasonableTimestamp = new Date().getTime() + (10 * 60 * 1000); // 10 minutes in the future
isBadMilliseconds('testContext', reasonableTimestamp);
expect(console.error).not.toHaveBeenCalled();
});
});
describe('isBadSeconds', () => {
it('should log an error for non-numeric timestamps', () => {
isBadSeconds('testContext', 'not-a-number');
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the past', () => {
const tooOldTimestampSeconds = Math.round(new Date().getTime() / 1000) - (4 * 365 * 24 * 60 * 60); // 4 years ago
isBadSeconds('testContext', tooOldTimestampSeconds);
expect(console.error).toHaveBeenCalled();
});
it('should log an error for timestamps too far in the future', () => {
const tooFutureTimestampSeconds = Math.round(new Date().getTime() / 1000) + (2 * 60 * 60); // 2 hours in the future
isBadSeconds('testContext', tooFutureTimestampSeconds);
expect(console.error).toHaveBeenCalled();
});
it('should not log an error for reasonable timestamps', () => {
const reasonableTimestampSeconds = Math.round(new Date().getTime() / 1000) + (10 * 60); // 10 minutes in the future
isBadSeconds('testContext', reasonableTimestampSeconds);
expect(console.error).not.toHaveBeenCalled();
});
});
});
Please ensure that the test file and the file under test are correctly set up in your project. If the semicolon error persists, it might be helpful to check other parts of your codebase or the specific test file for syntax errors, as the provided test suite should not inherently cause such an issue based on the syntax presented here.
Mock `console.error` test. created at Tue Jan 20 1970 18:33:18 GMT+0000 (Coordinated Universal Time)Related Chats
Mock `console.error` test. 0.999
Luxon for Human-Friendly Durations 0.424
Tests untuk initializeNewThread 0.401
NodeJS Playwright Test Loop 0.394
Teste de Unidade Vite 0.380
Born on March 8th 0.353
Future Date: April 30th 0.342
Tests Funktion sortByMessageId 0.335
Smoothly Animate Value with Timestamp 0.328
Get shows in range 0.316