Fix Warning: Use StateObject
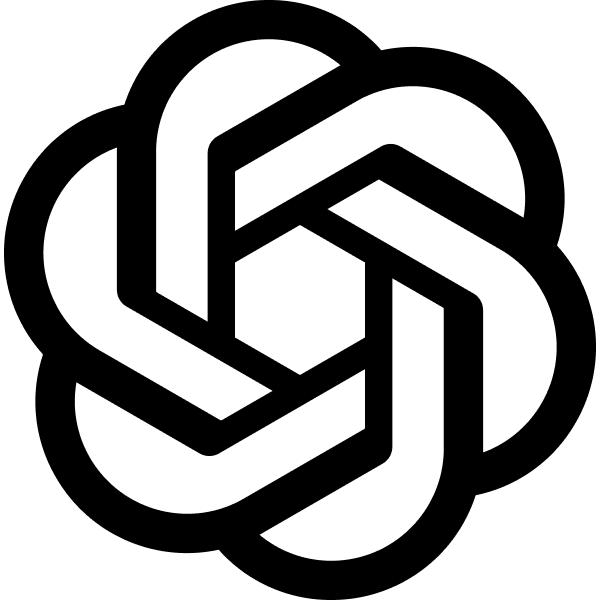
TODO: collapasable, copyable, download, etc
I'm writing a RealityKit app. In XCOde I have this warning I want to fix. Accessing StateObject's object without being installed on a View. This will create a new instance each time.
HEre is my code: ``` import SwiftUI import RealityKit import RealityKitContent
struct TrainerView: View {
@ObservedObject var multipeerManager = MultipeerManager()```The warning you're seeing occurs because StateObject
should be used instead of ObservedObject
when you want SwiftUI to manage the lifecycle of an object and create it only once.
StateObject
should be initialized only once during the lifetime of the view that owns it. Using @ObservedObject
will recreate the instance every time the view is recreated, which can lead to unexpected behavior.
Here is how you can fix the warning by using StateObject
instead:
import SwiftUI
import RealityKit
import RealityKitContent
struct TrainerView: View {
@StateObject var multipeerManager = MultipeerManager()
var body: some View {
// Your view content here
}
}
Explanation:
@StateObject
: This property wrapper is used to create and manage a reference type that conforms toObservableObject
. It should be used when you want SwiftUI to manage the lifecycle of the object and ensure that it is created only once.- Initialization: The object should be initialized when the view is created, which ensures that it is not recreated every time the view is updated.
This change will ensure that the MultipeerManager
instance is created once and managed correctly by SwiftUI, resolving the warning you are seeing.The compiler still says Accessing StateObject's object without being installed on a View. This will create a new instance each time.
The issue arises because @StateObject
must be initialized within the view body to ensure it is installed on the view correctly. Here’s how you can address this issue:
- Initialize
StateObject
inside the view:
Ensure that StateObject
is initialized correctly within the view's body.
- Use a separate initialization method if necessary:
If your MultipeerManager
requires parameters for initialization, use an initializer for the view to pass these parameters.
Here's an updated approach:
import SwiftUI
import RealityKit
import RealityKitContent
struct TrainerView: View {
@StateObject private var multipeerManager: MultipeerManager
init() {
_multipeerManager = StateObject(wrappedValue: MultipeerManager())
}
var body: some View {
// Your view content here
Text("Trainer View")
}
}
Explanation:
Initializer for the view:
- The
init
method initializes theStateObject
using the_property
syntax to properly initialize theStateObject
.
- The
Using
StateObject
:@StateObject
ensures thatMultipeerManager
is only initialized once and managed correctly by SwiftUI.
This should fix the warning and ensure that your MultipeerManager
instance is correctly managed by the view.I already have this initialization ``` init(laserSwordModel: LaserSwordViewModel, soundManager: SoundManager) {
self.laserSwordModel = laserSwordModel
self.soundManager = soundManager
self.multipeerManager.setLaserSwordViewModel(laserSwordModel: self.laserSwordModel)
SyncIPhoneSystem.multipeerManager = self.multipeerManager
SyncIPhoneSystem.handTrackingViewModel = self.model
}```
Should I add it there or create an init()
?Since you already have a custom initializer, you can add the initialization of StateObject
within this initializer. However, initializing StateObject
properties within a custom initializer requires a slightly different approach. You need to use the _property
syntax within the initializer.
Here's how you can modify your existing initializer to include the StateObject
initialization:
import SwiftUI
import RealityKit
import RealityKitContent
struct TrainerView: View {
@ObservedObject var laserSwordModel: LaserSwordViewModel
@ObservedObject var soundManager: SoundManager
@StateObject private var multipeerManager = MultipeerManager()
init(laserSwordModel: LaserSwordViewModel, soundManager: SoundManager) {
_laserSwordModel = ObservedObject(wrappedValue: laserSwordModel)
_soundManager = ObservedObject(wrappedValue: soundManager)
_multipeerManager = StateObject(wrappedValue: MultipeerManager())
self.laserSwordModel = laserSwordModel
self.soundManager = soundManager
self.multipeerManager.setLaserSwordViewModel(laserSwordModel: self.laserSwordModel)
SyncIPhoneSystem.multipeerManager = self.multipeerManager
SyncIPhoneSystem.handTrackingViewModel = self.laserSwordModel
}
var body: some View {
// Your view content here
Text("Trainer View")
}
}
Explanation:
StateObject Initialization:
_multipeerManager = StateObject(wrappedValue: MultipeerManager())
ensures thatStateObject
is initialized correctly within the custom initializer.
ObservedObject Initialization:
_laserSwordModel = ObservedObject(wrappedValue: laserSwordModel)
and_soundManager = ObservedObject(wrappedValue: soundManager)
ensure thatObservedObject
is initialized correctly.
Property Wrappers:
- The
_property
syntax is used to initialize theStateObject
andObservedObject
properties within the custom initializer.
- The
By using this approach, you ensure that StateObject
and ObservedObject
are correctly managed by SwiftUI, and the warning should be resolved.This seems very wrongI apologize for the confusion earlier. You are right. There is a more appropriate way to initialize StateObject
within a view that already has a custom initializer.
You should avoid directly initializing StateObject
in the initializer and instead move the configuration logic into the body
or use a separate method that gets called from within the view's lifecycle.
Here is a revised approach to correctly handle StateObject
:
import SwiftUI
import RealityKit
import RealityKitContent
struct TrainerView: View {
@ObservedObject var laserSwordModel: LaserSwordViewModel
@ObservedObject var soundManager: SoundManager
@StateObject private var multipeerManager = MultipeerManager()
init(laserSwordModel: LaserSwordViewModel, soundManager: SoundManager) {
self.laserSwordModel = laserSwordModel
self.soundManager = soundManager
}
var body: some View {
// Configure the multipeerManager
configureMultipeerManager()
return VStack {
// Your view content here
Text("Trainer View")
}
}
private func configureMultipeerManager() {
multipeerManager.setLaserSwordViewModel(laserSwordModel: self.laserSwordModel)
SyncIPhoneSystem.multipeerManager = self.multipeerManager
SyncIPhoneSystem.handTrackingViewModel = self.laserSwordModel
}
}
Explanation:
- Initialization in the
body
: TheStateObject
is initialized once and managed by SwiftUI. - Separate Configuration Method:
configureMultipeerManager()
is called within thebody
to configuremultipeerManager
with the necessary dependencies.
This approach ensures that multipeerManager
is correctly initialized and configured within the SwiftUI lifecycle, and the warning should be resolved.
Related Chats
StateObject Error Resolution 0.824
Provide EnvironmentObject TrainerView 0.546
Thread-Safe Multipeer Manager. 0.535
Frame Photos with SwiftUI 0.513
Shared ViewModel Across Views 0.513
Add Function to SwiftUI. 0.498
Troubleshoot `hasBeenConnected` SwiftUI 0.494
SwiftUI View with Model 0.490