SwiftUI View with Model
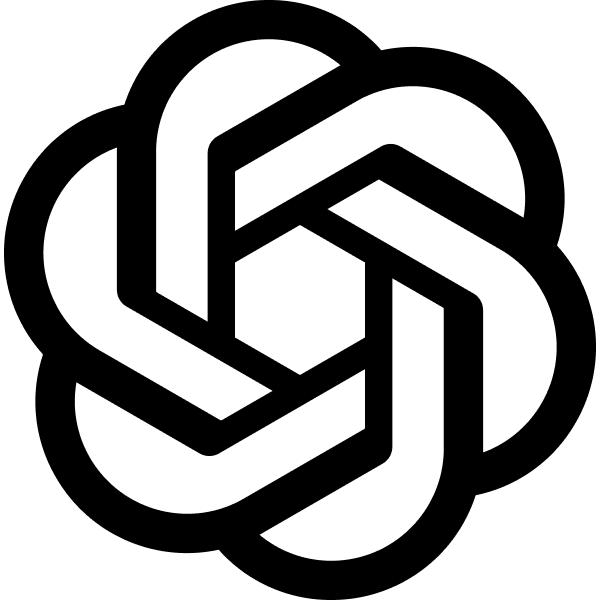
TODO: collapasable, copyable, download, etc
How can I make a SwiftUI view take an argument like ContentView(laserSwordModel: laserSwordModel)
To make a SwiftUI view take an argument like ContentView(laserSwordModel: laserSwordModel)
, you need to define a custom initializer for your view that accepts the argument. Here's an example of how you can achieve this:
Define your model class or struct. For this example, we'll assume
LaserSwordModel
is a struct:struct LaserSwordModel { var color: Color var length: CGFloat }
Define your
ContentView
struct with a property to hold the model and create an initializer for it:import SwiftUI struct ContentView: View { var laserSwordModel: LaserSwordModel // Custom initializer init(laserSwordModel: LaserSwordModel) { self.laserSwordModel = laserSwordModel } var body: some View { VStack { Text("Laser Sword") .font(.largeTitle) .foregroundColor(laserSwordModel.color) Rectangle() .fill(laserSwordModel.color) .frame(width: 10, height: laserSwordModel.length) } .padding() } }
Use the
ContentView
in your SwiftUI application, passing theLaserSwordModel
as an argument:@main struct MyApp: App { var body: some Scene { WindowGroup { let laserSwordModel = LaserSwordModel(color: .blue, length: 200) ContentView(laserSwordModel: laserSwordModel) } } }
This example demonstrates how to define a custom initializer for a SwiftUI view and how to pass a model to the view as an argument. You can adjust the LaserSwordModel
and the ContentView
to fit your specific use case.
Related Chats
SwiftUI View with Model 1.000
StateObject Error Resolution 0.609
Pass Data to SwiftUI Window. 0.599
Add Function to SwiftUI. 0.584
New chat 0.575
Pass Data to SwiftUI 0.549
Control Window Size: VisionOS 0.543
Troubleshoot `hasBeenConnected` SwiftUI 0.514