Using Sets for Uniqueness
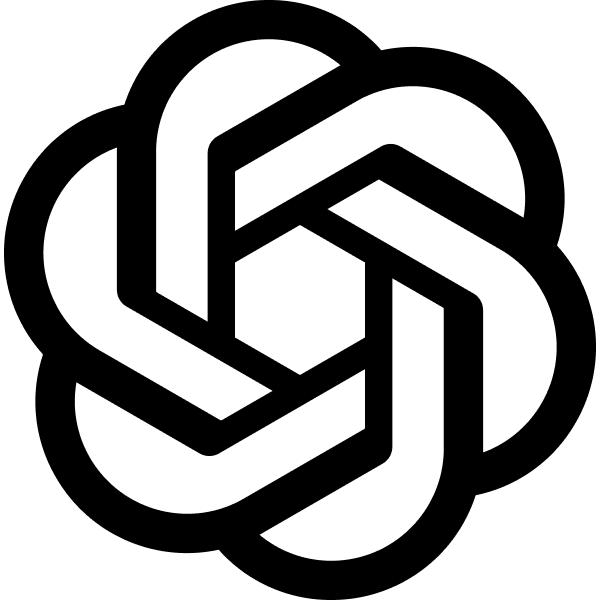
TODO: collapasable, copyable, download, etc
For vanilla code that runs in the browser, how do you use a Set so that you can be sure a collection of items are unique?Using a Set
in vanilla JavaScript (that is, JavaScript without any additional libraries or frameworks) is an efficient way to ensure a collection of items is unique. A Set
is a built-in object that stores unique values of any type, whether primitive values or object references.
Here's how you can use a Set
to ensure uniqueness in a collection:
Creating a Set: You can create a new
Set
by simply calling its constructor.let mySet = new Set();
Adding Items: To add items to the
Set
, use the.add()
method. If you try to add a duplicate item, theSet
will simply ignore it.mySet.add("apple"); mySet.add("banana"); mySet.add("apple"); // This will not be added again
Checking for Existence: You can check if an item is in the
Set
using the.has()
method.if (mySet.has("banana")) { // Item exists in the set }
Removing Items: To remove an item, use the
.delete()
method.mySet.delete("banana"); // Removes "banana" from the set
Iterating Over a Set: You can iterate over the elements of a
Set
usingfor...of
loop or.forEach()
method.for (let item of mySet) { console.log(item); } // or mySet.forEach(function(item) { console.log(item); });
Converting a Set to an Array: If you need to convert the
Set
back to an array, you can use the spread operator orArray.from()
.let myArray = [...mySet]; // or let myArray = Array.from(mySet);
Getting the Size of a Set: Use the
.size
property to find out how many items are in theSet
.console.log(mySet.size);
Here's a simple example that combines these steps:
let mySet = new Set();
mySet.add("apple");
mySet.add("banana");
mySet.add("orange");
console.log("Does my set have an apple?", mySet.has("apple")); // true
mySet.delete("banana");
for (let fruit of mySet) {
console.log(fruit);
}
// Output:
// Does my set have an apple? true
// apple
// orange
This example demonstrates how Set
ensures that each item is unique and provides various methods to manipulate and query the set.
Related Chats
Convert Objects to Set 0.718
Modern ES6 Array Filtering 0.438
Series Stay Same 0.359
Update Svelte Store Item 0.348
Check for Not Null 0.337
Svelte: Force New Component 0.329
Type Chats: ChatSession Creation 0.320
Sort Array by messageId 0.318
Random Element Selection 0.316