Type Chats: ChatSession Creation
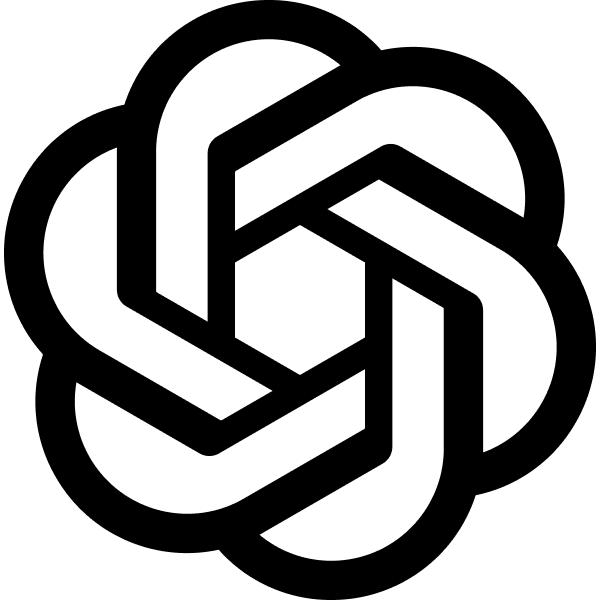
TODO: collapasable, copyable, download, etc
In TypeScript, i have a type ChatMessage
, how can I easily add a type to the messages
property below const chatSession = { guid: dbChatSession.guid, created_at: dbChatSession.created_at, updated_at: dbChatSession.updated_at, messages: [], };
To type the messages
property in your chatSession
object with TypeScript, you can define the ChatMessage
type and then explicitly specify that the messages
property is an array of ChatMessage
objects. If you haven't already defined the ChatMessage
type, you'll need to do that first. Here's an example of how you can do it:
First, define the ChatMessage
type. This example assumes a very simple structure for a chat message, but you can adjust it to fit your actual data structure:
type ChatMessage = {
id: string;
text: string;
senderId: string;
sentAt: Date;
};
Then, when defining your chatSession
object, you can specify the type of the messages
property as ChatMessage[]
to indicate it's an array of ChatMessage
objects:
type ChatSession = {
guid: string;
created_at: Date;
updated_at: Date;
messages: ChatMessage[];
};
const chatSession: ChatSession = {
guid: dbChatSession.guid,
created_at: dbChatSession.created_at,
updated_at: dbChatSession.updated_at,
messages: [], // TypeScript now knows this is an array of ChatMessage objects
};
This way, TypeScript knows that messages
is an array that should contain objects of type ChatMessage
. If you try to add something to the messages
array that doesn't fit the ChatMessage
type, TypeScript will give you a compile-time error, helping you catch and fix issues more easily.
Related Chats
JavaScript to TypeScript Transition 0.496
TS Default Value Fix 0.490
Sort Array by messageId 0.465
Conditional UserProvider Wrapper 0.445
Prisma Setup and Usage 0.427
React TypeScript - Handle Unknown 0.422
React Auth Types Enum 0.421