SvelteKit REST Proxy
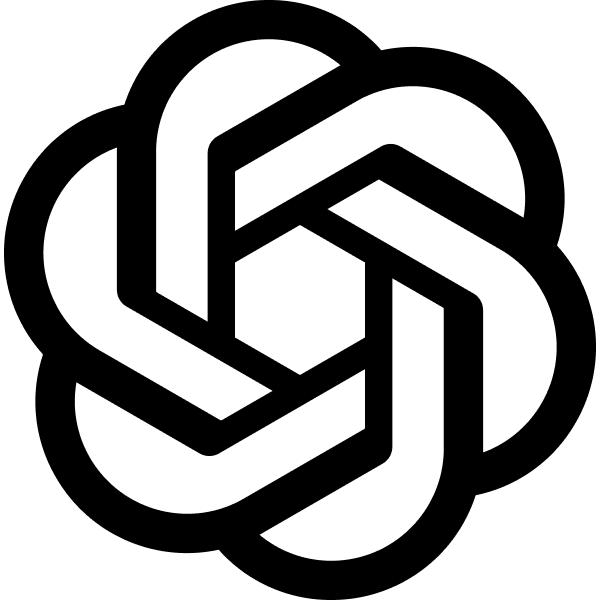
TODO: collapasable, copyable, download, etc
I have a SvelteKit app and the server side is basically like an express route. I want to provide a rest endpoint /query that has the following properties
- code will be in src/routes/query/+server.js
- Accept POST request, read payload as JSON.
- Validate some aspects and if things look good
- Proxy the request to a backend API service with exactly the same headers, payload, etc except the host will be http://query-service:8089
- if Validation fails, respond with 4xx error
Please generate this proxy code with stubs for my validation codeCertainly! Here's a basic outline of the server-side code in a SvelteKit app that fulfills your requirements:
// src/routes/query/+server.js
import fetch from 'node-fetch';
/**
* Validates the request payload.
* @param {Object} payload The request payload.
* @returns {boolean} True if the validation succeeds, otherwise false.
*/
function validatePayload(payload) {
// TODO: Add your validation logic here
// For now, always return true for demonstration purposes
return true;
}
export async function post(request) {
const { headers, body } = request;
if (!validatePayload(body)) {
return {
status: 400, // Bad Request
body: 'Validation failed'
};
}
try {
const response = await fetch('http://query-service:8089', {
method: 'POST',
headers: {
...headers,
host: undefined // remove the host header
},
body: JSON.stringify(body)
});
const responseBody = await response.text();
return {
status: response.status,
headers: {
'Content-Type': 'application/json'
},
body: responseBody
};
} catch (error) {
return {
status: 500, // Internal Server Error
body: 'Failed to proxy the request'
};
}
}
Explanation:
- The
validatePayload
function is a stub where you can add your own validation logic. - The
post
function handles the incoming POST request. If the payload fails validation, it responds with a400 Bad Request
status. - If the validation is successful, it proxies the request to your backend service using the
fetch
function, ensuring to preserve headers and payload. The host header is explicitly removed to avoid potential conflicts. - The response from your backend service is then relayed back to the client.
Remember to install and import the node-fetch
package if you haven't done so already:
npm install node-fetch
```Does this code support streaming the response back?The code I provided earlier doesn't support streaming. Instead, it waits for the entire response from the backend service to be received before sending it to the client.
To support streaming, you'd need to make some adjustments. Here's a version of the code that streams the response back to the client:
```javascript
// src/routes/query/+server.js
import fetch from 'node-fetch';
/**
* Validates the request payload.
* @param {Object} payload The request payload.
* @returns {boolean} True if the validation succeeds, otherwise false.
*/
function validatePayload(payload) {
// TODO: Add your validation logic here
// For now, always return true for demonstration purposes
return true;
}
export async function post(request) {
const { headers, body } = request;
if (!validatePayload(body)) {
return {
status: 400, // Bad Request
body: 'Validation failed'
};
}
try {
const response = await fetch('http://query-service:8089', {
method: 'POST',
headers: {
...headers,
host: undefined // remove the host header
},
body: JSON.stringify(body)
});
return {
status: response.status,
headers: {
'Content-Type': 'application/json'
},
body: response.body // Directly returning the readable stream
};
} catch (error) {
return {
status: 500, // Internal Server Error
body: 'Failed to proxy the request'
};
}
}
The key change here is that the response body from the fetch
function (which is a readable stream) is directly returned in the body
property of the response. This allows the response from the backend service to be streamed to the client as it is received, rather than waiting for the entire response before sending it.What does this nodejs server side error mean? duplex option is required when sending a body
The error message "duplex option is required when sending a body" suggests that when attempting to send a body as a readable stream (like in the case of streaming responses), the response or request handler expects a duplex stream or a specific option to be set to handle it correctly.
In the context of SvelteKit or similar frameworks, this error may arise when trying to directly pass a readable stream (like the one from node-fetch
) to the response without informing the framework that a duplex stream is being used.
A duplex stream is a stream that can be both read from and written to. When proxying or streaming requests/responses, it's often essential to ensure that the framework or server can handle the streaming properly.
To fix this error:
Ensure you're using the appropriate middleware or server configuration to handle streaming if the underlying server requires it.
If the framework provides an option to specify that the body is a duplex stream, set it. This might be specific to the framework or library you are using.
If you're using a server or framework that doesn't support streaming out-of-the-box, you might need to look into using a different server or adding additional middleware.
If you provide more context or specifics about the framework or setup you're using, I can give more tailored advice.In my SvelteKit route, I am doing this let payload = JSON.stringify(await request.json());
Is there a better way to handle the response as text?If you want to handle the body of the request as raw text in your SvelteKit route, you can use request.text()
directly. The request.json()
method assumes that the body contains a JSON payload and tries to parse it. If you want the raw text representation of the body, you don't need to stringify it again.
Here's a more direct way to get the raw text from the request:
let payload = await request.text();
By using request.text()
, you'll directly retrieve the raw text payload without any intermediary JSON parsing. This is especially useful if you just want to proxy the payload without interpreting or modifying it.Can you write me the nodejs code to read a file from the filesystem and parse it as JSON into a variable data
?Certainly! Here's a simple example using Node.js's built-in fs
module to read a file from the filesystem and parse its content as JSON:
const fs = require('fs').promises; // Import the promises API of the fs module
async function readFileAndParseJSON(filePath) {
try {
const fileContent = await fs.readFile(filePath, 'utf-8'); // Read the file as a UTF-8 encoded string
const data = JSON.parse(fileContent); // Parse the string content as JSON
return data;
} catch (err) {
console.error('Error reading or parsing the file:', err);
throw err;
}
}
// Usage:
(async () => {
const data = await readFileAndParseJSON('./path/to/your/file.json');
console.log(data);
})();
Replace './path/to/your/file.json'
with the path to your JSON file. When you run this code, it will read the file, parse its contents as JSON, and log the parsed data to the console. If there's an error reading the file or if the content isn't valid JSON, it will log an error message.Can you change the const fs = require('fs').promises
import to use the import syntax?Of course! To use the ES6 import
syntax, you'll have to use the import
statement instead of require()
. Here's how you can modify the code:
import { readFile } from 'fs/promises';
async function readFileAndParseJSON(filePath) {
try {
const fileContent = await readFile(filePath, 'utf-8'); // Read the file as a UTF-8 encoded string
const data = JSON.parse(fileContent); // Parse the string content as JSON
return data;
} catch (err) {
console.error('Error reading or parsing the file:', err);
throw err;
}
}
// Usage:
(async () => {
const data = await readFileAndParseJSON('./path/to/your/file.json');
console.log(data);
})();
Note that to usewhat is the nodejs code to filter an array to a single value? val == 'Name'
?To filter an array down to a single value in Node.js (or JavaScript in general), you can use the Array.prototype.filter()
method combined with the Array.prototype.find()
method.
If you want the first value that matches the condition, use the find
method:
const arr = ['John', 'Doe', 'Name', 'Smith'];
const result = arr.find(val => val === 'Name');
console.log(result); // Outputs: 'Name'
If you anticipate there might be multiple values matching the condition but you want only the first match, the above code with the find
method is sufficient. If you want all matches (which would be an array), use the filter
method:
const arr = ['John', 'Name', 'Doe', 'Name', 'Smith'];
const results = arr.filter(val => val === 'Name');
console.log(results); // Outputs: ['Name', 'Name']
In the examples above, replace the sample array and the condition as needed for your specific use case.Is there a more idiomatic or clearner way of doing this? ```export const extractMetricNames = (data) => { const COLUMN_KEY = "Name"; let column_index = -1; for (let i=0; i < data.columns.length; i++) { if (data.columns[i] == COLUMN_KEY) { column_index = i; } } if (column_index == -1) { throw new Error("Response didn't contain the Name key"); }
return data.rows.map((row) => row[column_index]);
}```Yes, your code can be made more concise and idiomatic by utilizing some built-in array functions in JavaScript. Here's a refactored version of your function:
export const extractMetricNames = (data) => {
const COLUMN_KEY = "Name";
const columnIndex = data.columns.findIndex(col => col === COLUMN_KEY);
if (columnIndex === -1) {
throw new Error("Response didn't contain the Name key");
}
return data.rows.map(row => row[columnIndex]);
};
Changes made:
- Replaced the
for
loop with thefindIndex
method which directly gives the index of the item that matches the condition. - Removed the unnecessary
column_index
initialization and reassignment by directly getting the column index fromfindIndex
. - Used concise arrow functions for brevity.
This refactored code is shorter, and the use of findIndex
makes the intention clearer.I'm adding a helper function ```async function readFileAndParseJSON(filePath) {
try {
const fileContent = await readFile(filePath, 'utf-8');
const data = JSON.parse(fileContent);
return data;
} catch (err) {
console.error('Error reading or parsing the file:', err);
throw err;
}
}``` to my codebase. Does that already exist in nodejs or ?Your readFileAndParseJSON
function is a utility function to combine reading a file and parsing its contents as JSON. While Node.js provides the tools to do both individually, it does not have a built-in function that combines both these operations as your function does.
- Node.js has
fs.promises.readFile
(orfs.readFile
with callbacks) to read a file. - JavaScript provides
JSON.parse
to parse a JSON string.
However, there isn't a single method in Node.js that combines these two into one, so your utility function fills that gap. It's common for developers to create such utility functions to make their codebase more readable and avoid repetitive code.
SvelteKit REST Proxy created at Tue Jan 20 1970 15:25:32 GMT+0000 (Coordinated Universal Time)Related Chats
Server-Side Code Placement 0.543
Configure SvelteKit for ELB 0.483
Node.js POST Request Stream 0.469
ELB Headers Configuration 0.468
Auth Config with Dynamic URL 0.456
Metrics Middleware for Next.js 0.450
SvelteKit Route Naming Conventions 0.439