Auth Config with Dynamic URL
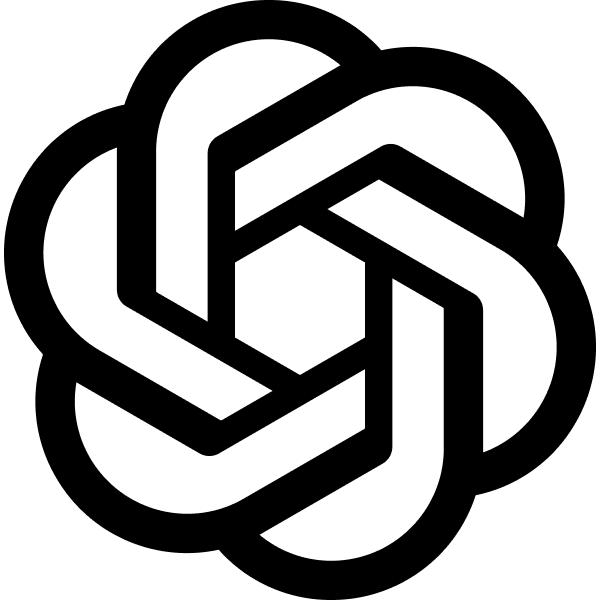
TODO: collapasable, copyable, download, etc
I'm using sveltekit the @auth
authentication model and the Credentials
provider. How do I tell the system my production hostname? It currently renders the form action to 'http://localhost:3000/auth/callbacks/credentials` In SvelteKit, when using an authentication solution like @sveltejs/auth
(assuming this is what you meant by @auth
, as @sveltejs/auth
is a common choice for authentication in SvelteKit applications) with a provider such as Credentials
, the issue you're facing with the form action URL pointing to localhost
in production typically arises due to the application not being properly configured to recognize and use the production environment's base URL.
To address this, you need to ensure that your application dynamically sets the base URL based on the environment it's running in, whether that's development (localhost) or production. This configuration often involves setting environment variables and making sure that your authentication configuration uses these variables accordingly.
Here's a general approach to fix this issue:
1. Setting Environment Variables
First, you need to set an environment variable for your production hostname. If you're deploying to a platform like Vercel, Netlify, or Heroku, they all have ways to set environment variables through their UI or CLI.
For example, you could set an environment variable named VITE_PUBLIC_BASE_URL
to your production URL (https://yourdomain.com
) and ensure that you have a default value for development.
# .env (for development)
VITE_PUBLIC_BASE_URL=http://localhost:3000
And set the production environment variable through your hosting provider's configuration settings.
2. Using the Environment Variable in SvelteKit
Next, you need to modify your authentication setup to use this environment variable for determining the base URL. SvelteKit projects can access environment variables prefixed with VITE_
directly in the code through import.meta.env
.
Here's how you could dynamically set the callback URL:
// src/routes/auth/callbacks/credentials.js
const baseUrl = import.meta.env.VITE_PUBLIC_BASE_URL;
// Use baseUrl to construct your callback URL dynamically
const callbackUrl = `${baseUrl}/auth/callbacks/credentials`;
3. Applying the Dynamic URL in the Authentication Configuration
Ensure that your authentication configuration, particularly the Credentials
provider, uses this dynamically set callback URL appropriately. This might involve passing the callbackUrl
to the configuration where your credentials provider is initialized.
Note:
- The exact implementation details can vary depending on how your authentication system is set up. If
@sveltejs/auth
does not directly expose a way to set the callback URL in its API, you might need to adjust the form action URL in your template or component dynamically using the environment variable. - Always ensure that environment variables containing sensitive information are not exposed to the client side unless it's intended to be public.
If you've followed these steps and are still facing issues, it could be helpful to review the documentation or source code of the authentication library you're using, as there might be specific configuration options or methods for handling deployment environments and callback URLs.where do I put provider.signinUrl
with @sveltejs/authWith @sveltejs/auth
or similar authentication libraries for SvelteKit, setting up a provider, such as the Credentials
provider, often involves configuring several options, including URLs for signing in, callbacks, and others. If you need to customize the signinUrl
for your Credentials
provider, this configuration typically occurs where you set up your authentication strategies, often in a server-side module or an initialization script where you configure your auth providers.
Here's a simplified example of how you might configure a Credentials
provider with @sveltejs/auth
, including specifying the signinUrl
. Note that this is a conceptual guide; the actual implementation details can vary based on the version of the library and any customizations you've applied:
Step 1: Import and Initialize Auth in hooks.js
In SvelteKit, you can use hooks.js
(or hooks.ts
if you're using TypeScript) to run code globally for your application, which is a good place to set up authentication.
// src/hooks.js
import { init } from '@sveltejs/auth';
import { Credentials } from '@sveltejs/auth/providers';
export async function handle({ event, resolve }) {
const { auth } = init(event, {
providers: [
Credentials({
name: 'credentials',
credentials: {
// Define the fields expected from the user
},
authorize: async (credentials) => {
// Implement your login logic here
},
signinUrl: '/custom-signin', // Custom signin URL
}),
],
});
return await resolve(event);
}
Step 2: Use the signinUrl
in Your Application
The signinUrl
you define in your provider's configuration is where the user will be directed when they initiate the sign-in process. Ensure that you have a corresponding route in your SvelteKit application that matches this URL and presents the user with a sign-in form or interface.
<!-- src/routes/custom-signin.svelte -->
<script>
// You might include logic here for handling form submission,
// typically sending credentials to your backend or directly to the auth provider.
</script>
<form method="post" action="/api/auth/signin/credentials">
<!-- Your sign-in form fields here -->
<button type="submit">Sign In</button>
</form>
Step 3: Adjusting for Production
As mentioned in the previous response, ensure that your application correctly handles different environments (development vs. production). For URLs specifically, this often means using environment variables or conditional logic to set paths dynamically based on the environment.
Additional Considerations
- Documentation and API Reference: Always refer to the latest documentation for
@sveltejs/auth
or the specific authentication library you're using, as APIs can change. - Custom Routes and API Endpoints: If you're implementing custom sign-in logic (as with the
Credentials
provider), ensure you have the necessary backend logic to authenticate users, set cookies or tokens, and manage sessions.
This example provides a general direction on how to configure the signinUrl
for a Credentials
provider in a SvelteKit application using @sveltejs/auth
. The exact details may vary based on your application's structure, the versions of the libraries you're using, and your specific authentication requirements.
Related Chats
Troubleshoot Authkit Redirection Loop 0.621
SvelteKit Route Naming Conventions 0.573
ELB Headers Configuration 0.550
Configure SvelteKit for ELB 0.533
Privacy Page 404 Error 0.523
Modify and Persist Session 0.482
Fix SvelteKit Playwright Test 0.475
Settings Toggle Endpoint Setup 0.460
SvelteKit REST Proxy 0.456