Popular NodeJS HTTP Libraries
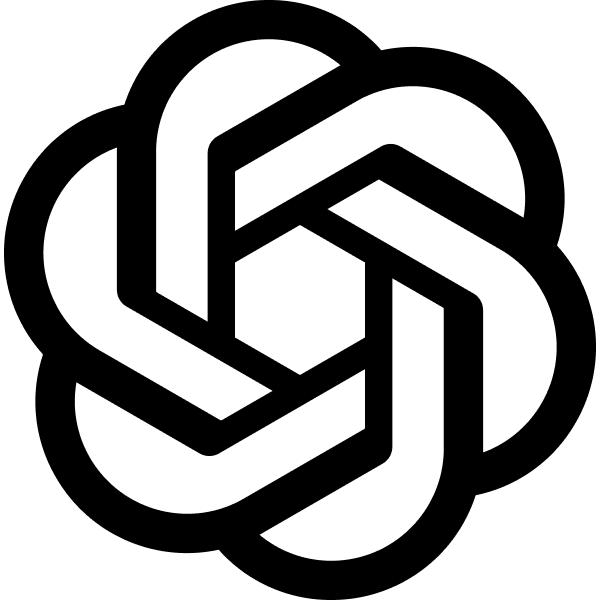
TODO: collapasable, copyable, download, etc
In 2024, what is the most popular way to make an http request in a NodeJs project?As of 2024, the most popular ways to make HTTP requests in a Node.js project include:
axios
:- Axios is a promise-based HTTP client that works both in the browser and in Node.js. It's widely appreciated for its ease of use, support for promises, ability to intercept request and response, and automatic transformation of JSON data.
node-fetch
:- Node-fetch is a light-weight module that brings
window.fetch
to Node.js. This provides a familiar way for web developers to make HTTP requests similar to how they would in the browser, making it easy to understand and use.
- Node-fetch is a light-weight module that brings
Native
http
andhttps
modules:- Node.js comes with built-in
http
andhttps
modules for making HTTP requests. While not as feature-rich or convenient as libraries like axios or node-fetch, they are robust and don't require additional dependencies, which can be an advantage in certain scenarios.
- Node.js comes with built-in
got
:- Got is another HTTP request library that is designed to be more user-friendly and powerful. It offers features like retry mechanisms, stream support, and advanced handling of cookies and caching.
superagent
:- Superagent is a flexible, progressive request library that is easy to use and extends well with plugins. It supports both callback-style and promise-based APIs.
Each of these libraries or methods offers different advantages, so the best choice can depend on the specific requirements of your project, such as support for promises, stream handling, simplicity, or the need to minimize external dependencies.Can you give me an example of node-fetch for a get request ?Certainly! Below is an example of how to use node-fetch
to perform a GET request in a Node.js application. This example assumes you've already installed node-fetch
using npm or yarn.
First, ensure you have node-fetch
installed:
npm install node-fetch
Then, you can use the following code in your Node.js script:
// Import the fetch function from node-fetch
const fetch = require('node-fetch');
// Function to perform a GET request
async function fetchData(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! status: ${response.status}`);
}
const data = await response.json(); // Assuming the server responds with JSON
return data;
} catch (error) {
console.error('Error fetching data: ', error);
}
}
// Example URL to fetch data from
const url = 'https://api.example.com/data';
// Call the function and log the response
fetchData(url).then(data => console.log(data));
In this example:
- We import the
fetch
function from thenode-fetch
module. - We define an asynchronous function
fetchData
that takes a URL as an argument. - Inside
fetchData
, we usefetch
to send a GET request to the URL. - We check if the response is successful. If not, we throw an error.
- If the response is successful, we parse the JSON from the response and return it.
- We call
fetchData
with a specific URL and log the result to the console.
This script will fetch data from the provided URL and log it to the console. Adjust the URL and handling based on your specific API and data format needs.Does nodeJS already have a fetch function?As of my last update in 2024, Node.js does not include a built-in fetch
function that mimics the browser's window.fetch
API out of the box. This is primarily because Node.js and browsers have different environments and needs, particularly around how they handle HTTP requests.
However, Node.js developers can use node-fetch
, which is a library that brings the window.fetch
functionality to Node.js. This library provides a way to make HTTP requests in Node.js using the same API that developers are familiar with from web development in browsers.
If you want to use fetch
in Node.js, you'll need to add node-fetch
to your project:
npm install node-fetch
And then you can use it in your project like this:
const fetch = require('node-fetch');
async function getExample() {
const response = await fetch('https://api.example.com');
const data = await response.json();
console.log(data);
}
getExample();
This provides a familiar and easy-to-use API for making HTTP requests in Node.js projects, adhering closely to the syntax and behavior of the browser's fetch
API.
Related Chats
Popular NodeJS HTTP Libraries 0.999
Node.js POST Request Stream 0.574
React Framework Alternatives: Blitz, Astro, Redwood. 0.522
New chat 0.487
Stream REST Data in JavaScript 0.486
DB Connection Middleware Setup 0.479
New chat 0.444
New chat 0.437