Understanding 3D Transformation Matrices
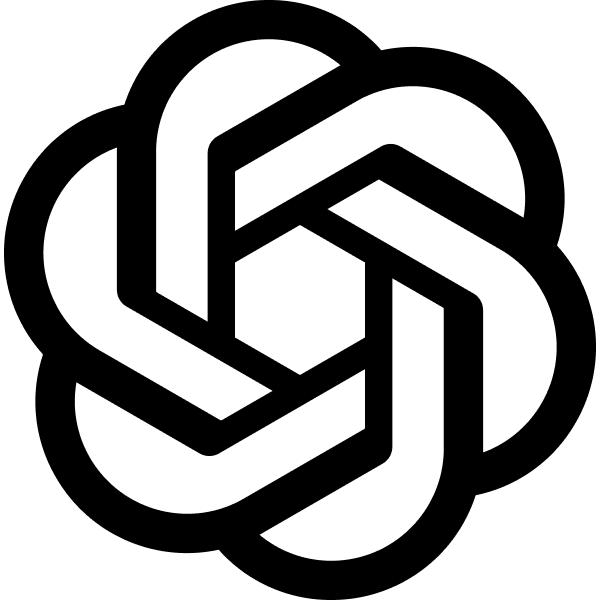
TODO: collapasable, copyable, download, etc
I need help learning RealityKit, ARKit, and 3D graphics programming / 3D maths.
I have this code ```let indexKnuckle = handAnchor.handSkeleton?.joint(.indexFingerKnuckle) guard ((indexKnuckle?.isTracked) != nil) else { continue }
// location and orienation of our hand in world space
let originFromWrist = handAnchor.originFromAnchorTransform
//
let wristFromIndex = indexKnuckle?.anchorFromJointTransform
// origin of our tip of our index finger!
var originFromIndex = originFromWrist * wristFromIndex!```
originFromIndex is a 4x4 matrix. I've found code online where people get the position out of it by originFromIndex.columns.3
. How do people know to do that? Can you help me understand this data format and APIUnderstanding how to work with 3D graphics programming, specifically with RealityKit and ARKit, involves understanding some fundamental concepts about 3D mathematics and transformations. Here's a breakdown of the code you provided and an explanation of how to interpret the transformation matrices involved:
Code Breakdown
let indexKnuckle = handAnchor.handSkeleton?.joint(.indexFingerKnuckle)
guard ((indexKnuckle?.isTracked) != nil) else { continue }
// Location and orientation of our hand in world space
let originFromWrist = handAnchor.originFromAnchorTransform
// Wrist from index
let wristFromIndex = indexKnuckle?.anchorFromJointTransform
// Origin of the tip of our index finger
var originFromIndex = originFromWrist * wristFromIndex!
3D Transformation Matrices
A 4x4 matrix is commonly used in 3D graphics to represent transformations that include translation, rotation, and scaling. In the context of ARKit and RealityKit, these matrices are used to describe the position and orientation of objects in 3D space.
Matrix Structure
A 4x4 transformation matrix generally looks like this:
[ \begin{bmatrix} m11 & m12 & m13 & m14 \ m21 & m22 & m23 & m24 \ m31 & m32 & m33 & m34 \ m41 & m42 & m43 & m44 \ \end{bmatrix} ]
In this structure:
- The upper-left 3x3 submatrix (elements m11 to m33) represents rotation and scaling.
- The first three elements of the fourth column (m14, m24, m34) represent translation (position) in x, y, and z axes.
- The last row (m41 to m44) is typically
[0, 0, 0, 1]
for affine transformations.
Extracting Position
When you see originFromIndex.columns.3
, it refers to the fourth column of the 4x4 matrix, which holds the translation components. Specifically:
originFromIndex.columns.3.x
gives the x-coordinate of the position.originFromIndex.columns.3.y
gives the y-coordinate of the position.originFromIndex.columns.3.z
gives the z-coordinate of the position.
This is because, in a column-major format (which is standard in many 3D graphics libraries, including those used by RealityKit and ARKit), the translation vector is located in the fourth column.
Understanding the API
To understand the data format and API:
- Documentation: Always refer to the official documentation for RealityKit and ARKit. They provide detailed information about the data structures and functions available.
- 3D Math Basics: Familiarize yourself with the basics of 3D mathematics, including vectors, matrices, and transformations. Resources like Khan Academy, tutorials on linear algebra, and graphics programming books can be very helpful.
- Practice: Implement small projects or follow tutorials to gain hands-on experience with the concepts.
Practical Example
Here’s a practical example to extract and print the position from originFromIndex
:
if let wristFromIndex = indexKnuckle?.anchorFromJointTransform {
let originFromIndex = originFromWrist * wristFromIndex
let position = originFromIndex.columns.3
print("Index finger position: x: \(position.x), y: \(position.y), z: \(position.z)")
}
Summary
- 4x4 Matrices: Used for transformations including translation, rotation, and scaling.
- Fourth Column: Contains the translation (position) components in 3D space.
- Documentation and Practice: Key to understanding and mastering these concepts.
By studying these foundational concepts and practicing with real code, you'll gain a solid understanding of 3D graphics programming and how to use RealityKit and ARKit effectively.What does m44 mean or used for? Or is it undefined, but necissary for the 4x4 shape?The element m44
in a 4x4 transformation matrix typically holds specific significance in different contexts, especially in computer graphics and 3D transformations. Here’s a breakdown of its role and meaning:
Affine Transformations
In the context of affine transformations, which include translation, rotation, and scaling, the 4x4 transformation matrix usually looks like this:
[ \begin{bmatrix} m11 & m12 & m13 & m14 \ m21 & m22 & m23 & m24 \ m31 & m32 & m33 & m34 \ m41 & m42 & m43 & m44 \ \end{bmatrix} ]
For affine transformations:
- The upper-left 3x3 part (m11 to m33) represents rotation and scaling.
- The first three elements of the fourth column (m14, m24, m34) represent translation.
- The fourth row is usually
[0, 0, 0, 1]
.
Homogeneous Coordinates
In homogeneous coordinates, which are used to facilitate matrix operations in 3D graphics, the m44
element typically equals 1
. This ensures that the matrix can correctly represent affine transformations. Specifically, homogeneous coordinates are an extension of Cartesian coordinates, where a 3D point ((x, y, z)) is represented as ((x, y, z, 1)).
Practical Role of m44
For most practical purposes in 3D graphics:
m44
is set to1
to maintain the integrity of affine transformations.- The elements
m41
,m42
, andm43
are set to0
to ensure the matrix represents an affine transformation correctly.
Special Cases
There are special cases, such as perspective transformations, where m44
can take different values. In perspective projection matrices, m44
helps achieve the effect of perspective, making objects appear smaller as they get farther from the camera.
Summary
- Affine Transformations:
m44
is typically1
, ensuring the matrix functions correctly for translation, rotation, and scaling. - Homogeneous Coordinates: Allows representation of points and transformations in a unified manner.
- Perspective Transformations:
m44
can vary to achieve specific effects like perspective projection.
Here's an example matrix for a typical affine transformation:
[ \begin{bmatrix} 1 & 0 & 0 & tx \ 0 & 1 & 0 & ty \ 0 & 0 & 1 & tz \ 0 & 0 & 0 & 1 \ \end{bmatrix} ]
In this matrix, tx
, ty
, and tz
represent translations along the x, y, and z axes, respectively, and m44
(1) ensures the matrix adheres to affine transformation properties.
Understanding this structure helps in manipulating and interpreting transformations in 3D graphics programming effectively.What is the simpliest swift code to assign a SIMD4 to a SIMD3? Please just output the codeHere's the simplest Swift code to assign a SIMD4<Float>
to a SIMD3<Float>
:
let vec4 = SIMD4<Float>(1.0, 2.0, 3.0, 4.0)
let vec3 = SIMD3<Float>(vec4.x, vec4.y, vec4.z)
This code initializes a SIMD4<Float>
vector and then creates a SIMD3<Float>
vector using the first three components of the SIMD4<Float>
vector.What is the swift idiom for dealing with optionals and checking before using In Swift, the idiomatic way to deal with optionals and check before using them involves using optional binding (if let
or guard let
) or optional chaining. Here are examples of each approach:
Using if let
if let unwrappedValue = optionalValue {
// Use unwrappedValue safely here
print("Value is \(unwrappedValue)")
} else {
// Handle the case where optionalValue is nil
print("Value is nil")
}
Using guard let
guard let
is typically used when you want to exit the current scope early if the optional is nil. This is commonly seen in functions or methods.
func exampleFunction(optionalValue: Int?) {
guard let unwrappedValue = optionalValue else {
// Handle the case where optionalValue is nil
print("Value is nil")
return
}
// Use unwrappedValue safely here
print("Value is \(unwrappedValue)")
}
Using Optional Chaining
Optional chaining allows you to call properties, methods, and subscripts on an optional that might currently be nil. If the optional is nil, the call returns nil.
let optionalString: String? = "Hello, world"
let stringLength = optionalString?.count
print("String length is \(String(describing: stringLength))")
Using ??
(Nil-Coalescing Operator)
The nil-coalescing operator provides a default value if the optional is nil.
let optionalValue: Int? = nil
let value = optionalValue ?? 0
print("Value is \(value)") // Output: Value is 0
Example Combined Usage
Here’s a combined example showing how to use these idioms together:
func processOptionalValue(optionalValue: Int?) {
// Using guard let
guard let unwrappedValue = optionalValue else {
print("Value is nil")
return
}
// Using optional chaining
let description = optionalValue?.description ?? "No description"
// Using if let
if let anotherUnwrappedValue = optionalValue {
print("Unwrapped value is \(anotherUnwrappedValue)")
} else {
print("Value is nil")
}
print("Processed value is \(unwrappedValue) and its description is \(description)")
}
processOptionalValue(optionalValue: 42)
processOptionalValue(optionalValue: nil)
In this example:
guard let
is used to unwrap the optional and exit early if it is nil.- Optional chaining (
optionalValue?.description
) is used to safely access a property of the optional. - The nil-coalescing operator (
??
) is used to provide a default value. if let
is used to unwrap the optional within a different scope.
Related Chats
Understanding 3D Transformation Matrices 0.999
Knuckle Position Synchronization 0.548
Switch to guard statement 0.501
Move Entity Up 0.005 0.483
AR Attachments: Use Cases 0.448
Calculate pointC using SIMD3 0.416
Animate Using withAnimation 0.408
Move ModelEntity X-Axis 0.407
Create 3D Models Prog. Swiftly 0.404
Immersive View Phases 0.387