Create 3D Models Prog. Swiftly
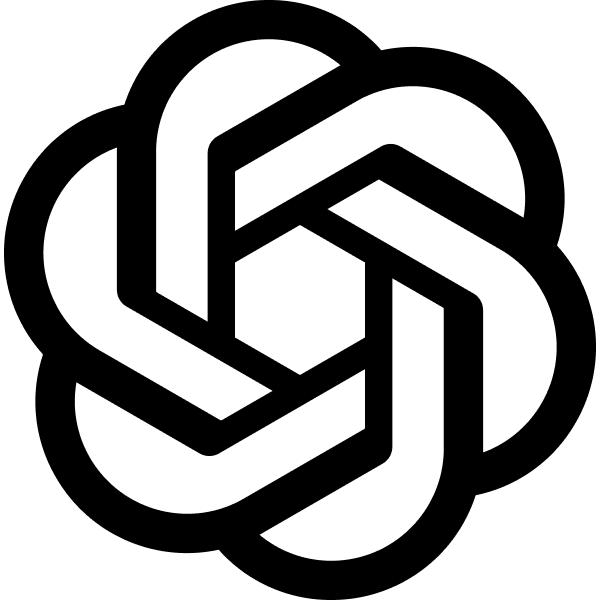
TODO: collapasable, copyable, download, etc
A swiftUI app can show a 3d model made in Reality Composer Pro. How could I programatically create a 3d model?Creating a 3D model programmatically for use in a SwiftUI app, especially one that can be displayed using Reality Composer or integrated directly into your app, involves several steps. Here's a high-level overview of the process and some technologies that can be utilized:
1. Choosing the Right Tool
- Reality Composer Pro is an intuitive tool provided by Apple for creating and editing augmented reality (AR) experiences. It allows you to work with USDZ files, which are the preferred format for 3D models in Apple's ARKit.
- SceneKit or RealityKit can be used to programmatically create 3D models in iOS applications. RealityKit is Apple's newer framework designed specifically for AR, offering more advanced features compared to SceneKit, which is a more general-purpose 3D graphics API.
2. Creating a 3D Model Programmatically
- With SceneKit: You can create basic 3D shapes (like spheres, boxes, cylinders, etc.) using SceneKit's geometry classes. For more complex models, you would typically import a 3D model created in a 3D modeling software, but you can also manually define the vertices, textures, and animations if needed.
- With RealityKit: RealityKit also allows the creation of basic shapes and supports loading USDZ files for more complex models. It's more focused on AR applications and integrates well with ARKit features.
3. Exporting or Converting to USDZ Format
- If you're starting with a programmatic model in SceneKit or RealityKit, and you want to use it in Reality Composer Pro, you'll need to export or convert it to USDZ format.
- Apple provides tools such as
usdz_converter
that can convert various 3D file formats to USDZ. This tool can be used as part of your build process or manually to prepare models for use in Reality Composer or directly in your SwiftUI app.
Example: Creating a Box in SceneKit
import SceneKit
// Create a scene
let scene = SCNScene()
// Create a box geometry
let boxGeometry = SCNBox(width: 1.0, height: 1.0, length: 1.0, chamferRadius: 0.0)
// Create a node with the box geometry
let boxNode = SCNNode(geometry: boxGeometry)
// Add the box node to the scene
scene.rootNode.addChildNode(boxNode)
// You can then display this scene in a SCNView or similar view for 3D content
Example: Creating a Box in RealityKit
import RealityKit
// Create an anchor entity
let anchor = AnchorEntity()
// Create a box model
let box = ModelEntity(mesh: .generateBox(size: 0.1), materials: [SimpleMaterial(color: .red, isMetallic: true)])
// Add the box model to the anchor
anchor.addChild(box)
// Assuming you have an ARView in your SwiftUI view
arView.scene.addAnchor(anchor)
Integrating with SwiftUI
For displaying these models in a SwiftUI application, you can wrap the SCNView
(for SceneKit) or ARView
(for RealityKit) in a UIViewRepresentable
to integrate it with your SwiftUI view hierarchy.
Conclusion
The process of creating a 3D model programmatically involves selecting the right tool or framework, creating or defining the model in code, and possibly converting it to a compatible format for use in your app or AR experiences. Depending on your specific requirements (e.g., complexity of the model, need for AR features), you might choose between SceneKit and RealityKit for your implementation.Let's do creating a box in realitykit. Can you give me the minimal SwiftUI code necissary?To create and display a simple 3D box using RealityKit in a SwiftUI application, you'll need to integrate an ARView
into your SwiftUI view. Below is a minimal example of how to do this:
Step 1: Import Required Frameworks
Make sure to import SwiftUI and RealityKit at the top of your Swift file.
import SwiftUI
import RealityKit
Step 2: Create a SwiftUI View for the ARView
You will create a SwiftUI view that contains an ARView
, where the 3D box will be displayed.
struct ContentView: View {
var body: some View {
ARViewContainer().edgesIgnoringSafeArea(.all)
}
}
Step 3: Define the ARViewContainer
ARViewContainer
is a struct that conforms to UIViewRepresentable
. This struct is responsible for creating and managing your ARView
.
struct ARViewContainer: UIViewRepresentable {
func makeUIView(context: Context) -> ARView {
let arView = ARView(frame: .zero)
// Create a box
let boxMesh = MeshResource.generateBox(size: 0.1)
let material = SimpleMaterial(color: .blue, isMetallic: true)
let boxModel = ModelEntity(mesh: boxMesh, materials: [material])
// Create an anchor and add the box to it
let anchor = AnchorEntity(world: .zero)
anchor.addChild(boxModel)
// Add the anchor to the ARView's scene
arView.scene.addAnchor(anchor)
return arView
}
func updateUIView(_ uiView: ARView, context: Context) {}
}
Step 4: Run Your SwiftUI App
Ensure you have the necessary permissions set in your Info.plist
file for camera usage (NSCameraUsageDescription
), then run your app. You should see a 3D blue box rendered in the ARView.
Conclusion
This minimal code snippet integrates RealityKit with SwiftUI to display a simple 3D box. This setup can be expanded with more complex RealityKit entities, interactions, and AR experiences as needed for your application.I'm working on VisionOS which doesn't ahve ARView. Can you use RealityView instead?For platforms like VisionOS, where ARView
is not available, and you're asked to use RealityView
instead, you can adapt the SwiftUI integration similarly to the example provided for ARView
, but with the necessary changes for RealityView
. Here's how you can do it:
Step 1: Import Required Frameworks
First, ensure you import SwiftUI and RealityKit. Since VisionOS is a hypothetical or specific context where RealityView
is used instead of ARView
, adjust the import statements as needed based on the actual development environment.
import SwiftUI
import RealityKit
Step 2: Create a SwiftUI View for the RealityView
Create a SwiftUI view that hosts the RealityView
. This view will be where your 3D content is displayed.
struct ContentView: View {
var body: some View {
RealityViewContainer().edgesIgnoringSafeArea(.all)
}
}
Step 3: Define the RealityViewContainer
Define a RealityViewContainer
struct that conforms to UIViewRepresentable
. This struct will create and manage the RealityView
. Since RealityView
is used as a replacement for ARView
, ensure you adapt this part based on the actual API provided by VisionOS for RealityView
.
struct RealityViewContainer: UIViewRepresentable {
func makeUIView(context: Context) -> RealityView {
let realityView = RealityView(frame: .zero)
// Assuming RealityView follows a similar pattern to ARView for adding entities
let boxMesh = MeshResource.generateBox(size: 0.1)
let material = SimpleMaterial(color: .blue, isMetallic: true)
let boxModel = ModelEntity(mesh: boxMesh, materials: [material])
// Create an anchor and add the box to it
let anchor = AnchorEntity(world: .zero)
anchor.addChild(boxModel)
// Add the anchor to the RealityView's scene
realityView.scene.addAnchor(anchor)
return realityView
}
func updateUIView(_ uiView: RealityView, context: Context) {}
}
Additional Notes
- The actual implementation details for
RealityView
might differ, so you'll need to adjust the example code based on its documentation or API reference. - Ensure that you have handled any necessary permissions or setup specific to VisionOS or the RealityKit framework being used in your context.
Conclusion
This guide provides a conceptual approach to integrating a 3D view similar to ARView
within a SwiftUI application, adapted for RealityView
. Due to the hypothetical nature of VisionOS and RealityView
, please refer to the specific documentation and APIs available for your development environment to make the necessary adjustments.
Related Chats
SwiftUI RealityKit Integration 0.608
Reusable SwiftUI custom view. 0.599
Preload Assets in visionOS 0.586
Handling App Exit 0.583
Shared ViewModel Across Views 0.577
Immersive View Phases 0.577
MultipeerManager Error Fix 0.576