Move ModelEntity X-Axis
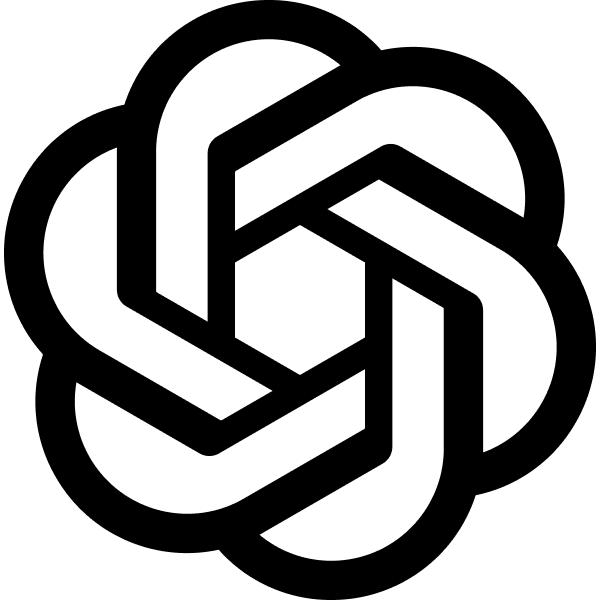
TODO: collapasable, copyable, download, etc
I am learning RealityKit. I have a ModelEntity, what is the best way to translate it along the X axis?When working with RealityKit and manipulating entities such as a ModelEntity
, you can translate an entity along the X-axis by adjusting its position or applying a transform. The best way to do this depends on your specific needs, such as whether you want to animate the translation or instantly move the entity to a new position. Below are two common approaches:
1. Direct Position Modification
You can directly modify the position
property of the entity's transform
. This is a straightforward way to move an entity to a specific location. Here's an example of how to translate an entity along the X-axis by a specific amount:
// Assuming `entity` is your ModelEntity
// Translate the entity by 1 unit along the X-axis
entity.transform.translation += SIMD3<Float>(1, 0, 0)
This method directly modifies the entity's position, instantly moving it to the new location.
2. Using Move Action for Animation
If you want to animate the movement, you can use the move(to:)
action provided by RealityKit. This method allows you to specify a destination transform, including the new position, and animate the entity's movement to that position over time. Here's an example:
// Create a target translation
let targetTranslation = SIMD3<Float>(entity.transform.translation.x + 1, entity.transform.translation.y, entity.transform.translation.z)
// Create a target transform with the new translation
let targetTransform = Transform(scale: entity.transform.scale, rotation: entity.transform.rotation, translation: targetTranslation)
// Move the entity to the new transform over 1 second
entity.move(to: targetTransform, relativeTo: nil, duration: 1, timingFunction: .linear)
This method is useful when you want the entity to animate smoothly from its current position to the new position along the X-axis.
Choosing the Best Way
- Direct Position Modification: Use this method for instant changes to the entity's position without animation. It's simple and effective for quick relocations.
- Using Move Action for Animation: Choose this method when you want the entity to move to a new position with an animation, providing a smoother transition.
Both methods are "best" in their contexts, depending on whether you require an instantaneous move or a smooth, animated transition.
Move ModelEntity X-Axis created at Tue Jan 20 1970 18:17:30 GMT+0000 (Coordinated Universal Time)Related Chats
Move ModelEntity X-Axis 1.000
Move Entity Up 0.005 0.744
Convert Entity to ModelEntity 0.579
Generate Sphere in AR. 0.571
SwiftUI Drag Rotation Implementation 0.536
Modify Cylinder Properties 0.513
Move Cube with Accelerometer 0.455
Add Function to SwiftUI. 0.434