Animate Using withAnimation
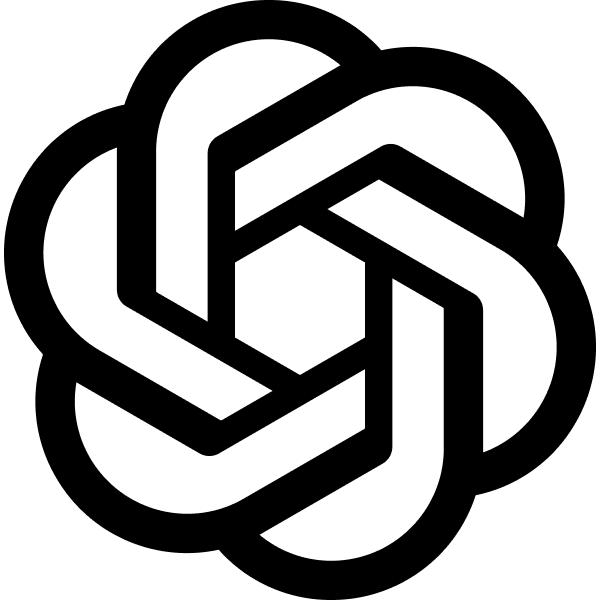
TODO: collapasable, copyable, download, etc
How would I update .animation( Animation.linear(duration: 2) .repeatForever(autoreverses: true) )
to use the withAnimation
syntax instead?
Spatial computing goes even further with visionOS
updates that bring enhanced support for
volumetric apps, powerful new enterprise APIs, the
new TabletopKit framework, and more.
visionOS Highlights
TabletopKit Create collaborative experiences centered around a table. This new framework handles the manipulation of cards and pieces, establishing placement and layout, and defining game boards.
New enterprise APIs provide access to spatial barcode scanning, the Apple Neural Engine, object tracking parameters, and more.
visionOS for enterprise
Volumetric APIs • Create apps that take full advantage of depth and space — and can
run side by side with other apps in the Shared Space.
• Resize volumes by using the SwiftUI scene
modifier windowResizability.
Decide if you want the user’s hands to appear in front of or behind your digital content.
Inputs
Getting started? Explore the visionOS Pathway on developer.apple.com
• Detect planes in all orientations.
• Allow anchoring objects on surfaces in your surroundings.
• Use Room Anchors to consider the user’s surroundings on a
per-room basis.
• Attach content to individual objects around the user with the new
Object Tracking API for visionOS.
Updates to scene understanding
To update the .animation
modifier to use the withAnimation
syntax in SwiftUI, you would encapsulate the state changes within the withAnimation
closure. This provides more control over when the animation is applied. Here is how you can transform the given animation modifier:
Original Code:
.animation(
Animation.linear(duration: 2)
.repeatForever(autoreverses: true)
)
Updated Code Using withAnimation
:
First, ensure that you trigger the animation within a state change, like toggling a boolean value.
Example:
import SwiftUI
struct ContentView: View {
@State private var animate = false
var body: some View {
Rectangle()
.fill(Color.blue)
.frame(width: 100, height: 100)
.offset(y: animate ? 300 : 0)
.onAppear {
withAnimation(Animation.linear(duration: 2).repeatForever(autoreverses: true)) {
animate.toggle()
}
}
}
}
Explanation:
- State Variable:
@State private var animate = false
is used to track the animation state. - Animation Logic:
withAnimation
encapsulates the animation configuration. - Trigger Animation: The animation is triggered in the
.onAppear
modifier, toggling theanimate
state.
This approach allows the withAnimation
closure to handle the animation logic, making the code more readable and flexible.Cannot find 'animate' in scopeIt looks like there was a mistake in the state variable definition and usage. Let's fix the code to ensure it works correctly. The withAnimation
should toggle the state variable within an action. Here's a corrected version:
Corrected Example:
import SwiftUI
struct ContentView: View {
@State private var animate = false
var body: some View {
Rectangle()
.fill(Color.blue)
.frame(width: 100, height: 100)
.offset(y: animate ? 300 : 0)
.onAppear {
withAnimation(Animation.linear(duration: 2).repeatForever(autoreverses: true)) {
animate = true
}
}
}
}
Explanation:
- State Variable:
@State private var animate = false
is defined to track the animation state. - Animation Logic:
withAnimation
encapsulates the animation configuration and setsanimate
totrue
. - Trigger Animation: The animation is triggered in the
.onAppear
modifier, setting theanimate
state totrue
.
This approach ensures that the state variable animate
is properly used within the withAnimation
closure to apply the animation.In swiftui, how can I provide a grey background for an element. Thinking like a panel with a border to set it apartIn SwiftUI, you can create a panel with a grey background and a border to set it apart using the .background
, .cornerRadius
, and .border
modifiers. Here's an example:
Example Code:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Panel Content")
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
.border(Color.gray, width: 1)
.padding()
}
.padding()
}
}
Explanation:
Text
Element: TheText
element serves as the content of the panel.padding
Modifier: Adds padding around the text to give some space between the text and the edges of the panel.background
Modifier: Applies a grey background color to the panel. Theopacity(0.2)
makes the grey color slightly transparent.cornerRadius
Modifier: Rounds the corners of the panel to make it look like a card or panel.border
Modifier: Adds a border around the panel with the specified color and width.- Outer
padding
: Adds some space around the panel within theVStack
.
Enhanced Example with More Customization:
If you want to add a shadow or more customization, you can further modify the example:
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("Panel Content")
.padding()
.background(Color.gray.opacity(0.2))
.cornerRadius(10)
.overlay(
RoundedRectangle(cornerRadius: 10)
.stroke(Color.gray, lineWidth: 1)
)
.shadow(radius: 5)
.padding()
}
.padding()
}
}
Additional Modifications:
overlay
Modifier: Adds a border with rounded corners around the panel.shadow
Modifier: Adds a shadow to give a subtle depth effect to the panel.
These examples should help you create a visually distinct panel with a grey background, a border, and other stylistic features in SwiftUI.
Animate Using withAnimation created at Tue Jan 20 1970 21:17:06 GMT+0000 (Coordinated Universal Time)Related Chats
MultipeerManager Error Fix 0.892
Preload Assets in visionOS 0.881
Immersive View Phases 0.847
Switch to guard statement 0.841
Handling App Exit 0.840
Reusable SwiftUI custom view. 0.829
SwiftUI RealityKit Integration 0.825