Kotlin Regex Matching Patterns.
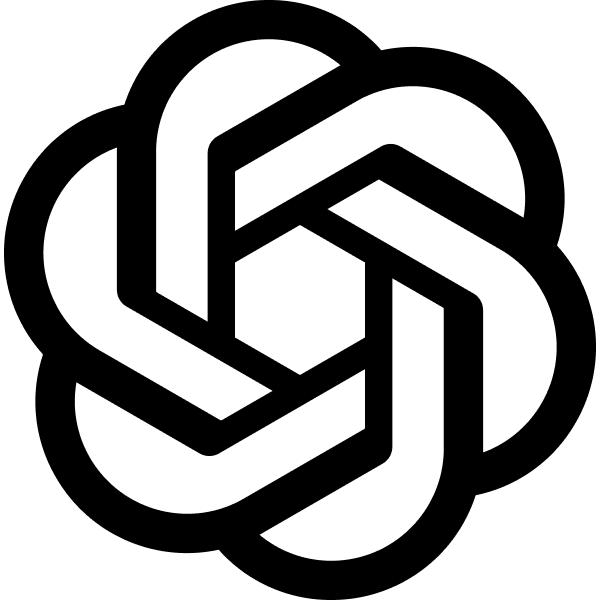
TODO: collapasable, copyable, download, etc
What is the Kotlin code for a regular expression that matches values like $10/month, $25/month, $50/mo, $100/year, $100/yr, etcYou can use the following Kotlin code with a regular expression to match the given patterns:
fun main() {
val regex = Regex("""\$\d+\s*/\s*(month|mo|year|yr)""")
val testValues = listOf(
"$10/month",
"$25/month",
"$50/mo",
"$100/year",
"$100/yr"
)
for (value in testValues) {
if (regex.matches(value)) {
println("$value matches the pattern.")
} else {
println("$value does not match the pattern.")
}
}
}
Here's the explanation of the regular expression:
\$\d+
: Match a dollar sign followed by one or more digits.\s*/\s*
: Match an optional whitespace, a forward slash, and then another optional whitespace.(month|mo|year|yr)
: Match one of the following words: month, mo, year, or yr.
This regular expression should match the given examples, but you can modify the regex to better suit your needs.Thanks for explaining how the code works, can you update the code with that explanation inline?Certainly! Here's the Kotlin code with inline comments explaining the regular expression:
fun main() {
// Regular expression pattern:
// - \$\d+ : Match a dollar sign followed by one or more digits.
// - \s*/\s* : Match an optional whitespace, a forward slash, and then another optional whitespace.
// - (month|mo|year|yr) : Match one of the following words: month, mo, year, or yr.
val regex = Regex("""\$\d+\s*/\s*(month|mo|year|yr)""")
val testValues = listOf(
"$10/month",
"$25/month",
"$50/mo",
"$100/year",
"$100/yr"
)
for (value in testValues) {
if (regex.matches(value)) {
println("$value matches the pattern.")
} else {
println("$value does not match the pattern.")
}
}
}
This version of the code includes comments explaining each part of the regular expression, making it easier to understand and modify if needed.
Kotlin Regex Matching Patterns. created at Tue Jan 20 1970 10:21:59 GMT+0000 (Coordinated Universal Time)Related Chats
Kotlin Regex Matching Patterns. 0.999
Rust regex pattern for prices. 0.666
JS Regex for Ref Replacement 0.460
Refactoring code to capture GUID. 0.363
Regex find and replace 0.346
Clickhouse REGEXP: Exclude "service" 0.341
Rust: Replace String Substitution 0.282
Rust Enum for Strings 0.282
Teste de Autenticação Vitest 0.281
Rust Text File Parser 0.279