Rust Enum for Strings
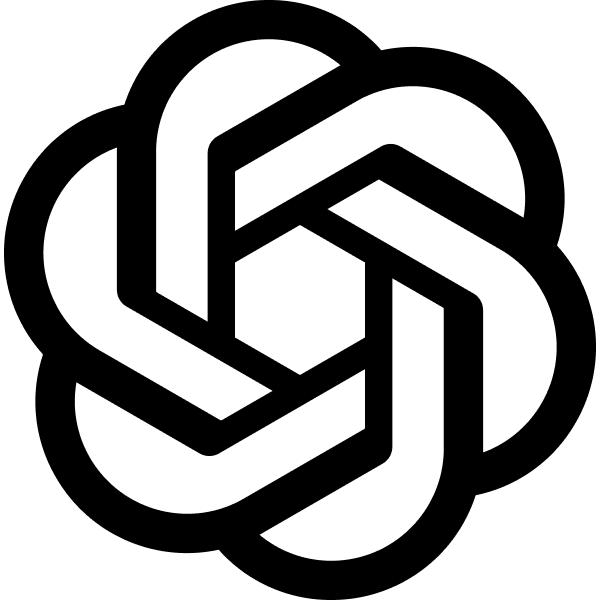
TODO: collapasable, copyable, download, etc
I want to make a Rust enum that would be useful for matching the following strings "auction", "auction-lot".To create a Rust enum
that matches the strings "auction" and "auction-lot", you can define the enum
like so:
enum AuctionType {
Auction,
AuctionLot,
}
impl AuctionType {
fn from_str(s: &str) -> Option<Self> {
match s {
"auction" => Some(AuctionType::Auction),
"auction-lot" => Some(AuctionType::AuctionLot),
_ => None,
}
}
}
fn main() {
let sample = "auction-lot";
match AuctionType::from_str(sample) {
Some(AuctionType::Auction) => println!("It's an auction!"),
Some(AuctionType::AuctionLot) => println!("It's an auction lot!"),
None => println!("Unknown type"),
}
}
In this example:
- We define an
enum
calledAuctionType
with two variants:Auction
andAuctionLot
. - We implement the
from_str
method forAuctionType
which takes a string slice and returns anOption<AuctionType>
. If the string matches "auction" or "auction-lot", it returns the correspondingAuctionType
variant wrapped in aSome
. Otherwise, it returnsNone
. - In the
main
function, we test the conversion from a string to anAuctionType
and then match on the result to print an appropriate message.
This provides a type-safe way of working with these specific strings in Rust.Is there a more idiomatic way to write this?
Some(item) => {
match item {
CmsTypes::Auction => "",
CmsTypes::AuctionLot => "",
}
},
None => panic!("Unhandled cms type")
};```Yes, your nested match can be flattened using a single match for more idiomatic and readable code. Here's how you can write it:
```rust
let url = match CmsTypes::from_str(¶ms.r#type) {
Some(CmsTypes::Auction) => "",
Some(CmsTypes::AuctionLot) => "",
None => panic!("Unhandled cms type"),
};
This way, you're directly pattern matching on the variants of CmsTypes
right within the main match statement, which makes the code clearer and removes the need for nested matches.In Rust how for an Option - how do I get the string or a default value of "foo"In Rust, you can use the unwrap_or
method of Option<T>
to get the contained value or a default if the Option
is None
.
Here's an example using an Option<String>
:
fn main() {
let maybe_string: Option<String> = Some("Hello".to_string());
let result = maybe_string.unwrap_or("foo".to_string());
println!("{}", result); // Outputs: Hello
let none_string: Option<String> = None;
let result2 = none_string.unwrap_or("foo".to_string());
println!("{}", result2); // Outputs: foo
}
In the above example:
- If
maybe_string
contains aSome
, it'll print the contained string. - If
maybe_string
isNone
(likenone_string
), it'll print the default value, which is "foo".Is there a more idomatic way in Rust to saylet publication_state = if include_draft { "preview" } else { "live" };
Yes, the line you've written is idiomatic for many Rust scenarios, but if you want to ensure thatpublication_state
is always of type&str
, you might specify it explicitly:
let publication_state: &str = if include_draft { "preview" } else { "live" };
The above is especially useful if the branches of the if
expression may infer different types, though in this specific case it's not strictly necessary.
Another slightly more concise approach would be using the ternary-like capabilities of Rust's match:
let publication_state = match include_draft {
true => "preview",
false => "live",
};
This match variant is a bit more verbose than the if-else but can be very handy when dealing with enums or more complex matching conditions.
Both the original if-else and the match construct are idiomatic in Rust, and the choice between them is largely a matter of personal preference or the specific context in which they're used.What is the Rust code to check to see if a file existsIn Rust, you can use the std::path::Path
and its exists
method to check if a file (or directory) exists. Here's an example:
use std::path::Path;
fn main() {
let file_path = "path/to/your/file.txt";
if Path::new(file_path).exists() {
println!("The file exists.");
} else {
println!("The file does not exist.");
}
}
Replace "path/to/your/file.txt"
with the path to the file you want to check. If the file exists, the program will print "The file exists."
; otherwise, it will print "The file does not exist."
.What is the Rust code to read that file?To read a file in Rust, you can use the std::fs::read_to_string
function. Here's how you can use it to read the content of a file:
use std::fs;
use std::io::{self, ErrorKind};
fn main() -> io::Result<()> {
let file_path = "path/to/your/file.txt";
match fs::read_to_string(file_path) {
Ok(content) => {
println!("File content:\n{}", content);
}
Err(error) => {
match error.kind() {
ErrorKind::NotFound => println!("Fileas a binary fileTo read a binary file, you'll use `std::fs::read`, which returns a `Vec<u8>`, representing the raw bytes from the file. Here's an example:
```rust
use std::fs;
use std::io::{self, ErrorKind};
fn main() -> io::Result<()> {
let file_path = "path/to/your/binary/file.bin";
match fs::read(file_path) {
Ok(bytes) => {
// Process the bytes. For demonstration, we're just printing the length.
println!("Read {} bytes from the file.", bytes.len());
}
Err(error) => {
match error.kind() {
ErrorKind::NotFound => println!("File not found."),
_ => println!("An error occurred: {}", error),
}
}
}
Ok(())
}
Here, fs::read
reads the entire binary file into a Vec<u8>
. Depending on what you want to do with the binary data, you can then process the bytes
vector accordingly. For this demonstration, we simply print the number of bytes read.
Related Chats
Rust Enum for Strings 1.000
Scraper Implementation with Enum 0.789
Serde Rename JSON Field 0.505
Nested vec! Macro Usage 0.498
Rust Marketplace Scraper. 0.484
Mixing Static Dynamic JSON 0.456
Rust regex pattern for prices. 0.443
MD5 Hash in Rust 0.440
Fix TS Error: useAtom 0.438