Rust regex pattern for prices.
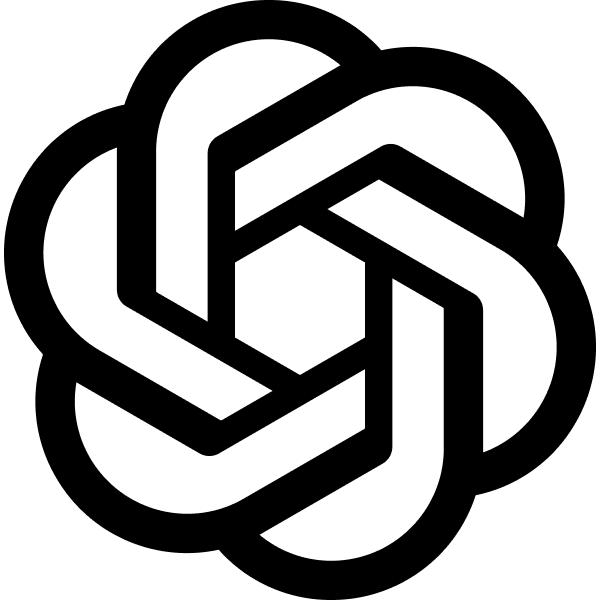
TODO: collapasable, copyable, download, etc
What is the Rust code for a regular expression that matches values like $10/month, $25/month, $50/mo, $100/year, $100/yr, etcTo create a regular expression in Rust that matches values like the examples you provided, you can use the regex
crate. First, add the regex
crate to your Cargo.toml
:
[dependencies]
regex = "1.0"
Now, here's a Rust code snippet that demonstrates how to create and use the regular expression:
use regex::Regex;
fn main() {
let pattern = r"(?x) # Enable free-spacing mode for better readability
\$ # Dollar sign
(\d+) # Match one or more digits (capture group 1)
\s* # Optional white spaces
/ # Slash
\s* # Optional white spaces
(month|mo|year|yr) # Match 'month', 'mo', 'year', or 'yr' (capture group 2)
";
let re = Regex::new(pattern).unwrap();
let test_strings = [
"$10/month",
"$25/month",
"$50/mo",
"$100/year",
"$100/yr",
"Invalid example",
];
for s in &test_strings {
if let Some(captures) = re.captures(s) {
println!("Matched: {:?}", captures);
} else {
println!("Did not match: {}", s);
}
}
}
This code snippet defines the regular expression pattern and tests it against an array of strings. The (?x)
flag at the start of the pattern enables free-spacing mode, which allows you to add comments and whitespace to the pattern for better readability.Given captures, how would I pull out the first and second value?You can use the captures.get()
method to access specific capture groups within the Captures
struct. In your case, to extract the first and second values from the captures, you can use the following code snippet:
use regex::Regex;
fn main() {
let pattern = r"(?x) # Enable free-spacing mode for better readability
\$ # Dollar sign
(\d+) # Match one or more digits (capture group 1)
\s* # Optional white spaces
/ # Slash
\s* # Optional white spaces
(month|mo|year|yr) # Match 'month', 'mo', 'year', or 'yr' (capture group 2)
";
let re = Regex::new(pattern).unwrap();
let test_strings = [
"$10/month",
"$25/month",
"$50/mo",
"$100/year",
"$100/yr",
"Invalid example",
];
for s in &test_strings {
if let Some(captures) = re.captures(s) {
// Get the first and second capture groups
let amount = captures.get(1).map_or("", |m| m.as_str());
let period = captures.get(2).map_or("", |m| m.as_str());
println!("Amount: {}, Period: {}", amount, period);
} else {
println!("Did not match: {}", s);
}
}
}
In this example, we use captures.get(1)
and captures.get(2)
to access the first and second capture groups, respectively. map_or()
is used to convert the Option<Match>
into a string (using as_str()
), defaulting to an empty string if the capture group is not present (which should not happen in this case). The extracted values are then printed out.let amount = captures.get(1).map_or("", |m| m.as_str()); the amount is a &str type. How could I get that as a f64 instead?To convert the amount
from a &str
type to an f64
, you can use the parse()
method. Here's an updated version of the code snippet that converts the amount
to an f64
:
use regex::Regex;
fn main() {
let pattern = r"(?x) # Enable free-spacing mode for better readability
\$ # Dollar sign
(\d+) # Match one or more digits (capture group 1)
\s* # Optional white spaces
/ # Slash
\s* # Optional white spaces
(month|mo|year|yr) # Match 'month', 'mo', 'year', or 'yr' (capture group 2)
";
let re = Regex::new(pattern).unwrap();
let test_strings = [
"$10/month",
"$25/month",
"$50/mo",
"$100/year",
"$100/yr",
"Invalid example",
];
for s in &test_strings {
if let Some(captures) = re.captures(s) {
// Get the first and second capture groups
let amount_str = captures.get(1).map_or("", |m| m.as_str());
let period = captures.get(2).map_or("", |m| m.as_str());
// Parse the amount as f64
let amount: f64 = match amount_str.parse() {
Ok(val) => val,
Err(_) => {
println!("Failed to parse amount: {}", amount_str);
continue;
}
};
println!("Amount: {}, Period: {}", amount, period);
} else {
println!("Did not match: {}", s);
}
}
}
In this example, we first store the amount as a &str
in the variable amount_str
. Then, we attempt to parse amount_str
as an f64
using the parse()
method. If the parsing is successful, we store the result in the variable amount
. If there is an error during parsing, we print an error message and move on to the next test string with the continue
keyword.What are 10 ways this Rust code could be improved?Here are 10 ways to improve the Rust code provided:
- Return a struct with extracted data: Instead of printing the extracted amount and period, you could return a struct containing the parsed data for easier consumption by other parts of the code.
struct PricePeriod {
amount: f64,
period: String,
}
Function to extract data: Create a separate function that takes a string as input, and returns an
Option<PricePeriod>
for better code organization.Use
map_or_else
instead ofmap_or
:map_or_else
allows you to avoid creating a new empty string when the capture is present.Error handling: Improve error handling by using a custom error type or an existing error type like
Box<dyn std::error::Error>
in the function that returnsResult<PricePeriod, Error>
instead of usingOption
.Validate input: Add additional validation checks for input strings, such as checking for negative amounts.
Use
lazy_static
for regex: Compile the regex only once using thelazy_static
crate to improve performance in case of multiple calls to the function.Use named capture groups: Named capture groups can make the code more readable and easier to understand.
let pattern = r"(?x)
\$(?P<amount>\d+)
\s*/\s*
(?P<period>month|mo|year|yr)";
- Case-insensitive matching: Use case-insensitive matching for the period to make the regex more flexible.
let re = Regex::new(r"(?i)pattern").unwrap();
Use the
FromStr
trait for custom parsing: Implement theFromStr
trait for thePricePeriod
struct to leverage Rust's standard string parsing capabilities.Add tests: Write tests for the function that extracts data from the input string to ensure the code works as expected and to help catch any regressions.
By applying these improvements, the code becomes more modular, easier to maintain, and more efficient.
Rust regex pattern for prices. created at Tue Jan 20 1970 10:21:31 GMT+0000 (Coordinated Universal Time)Related Chats
Kotlin Regex Matching Patterns. 0.666
Population Percentage: 2.41% 0.552
Rust Text File Parser 0.529
Rust: Replace String Substitution 0.464
JS Regex for Ref Replacement 0.449
Rust Enum for Strings 0.443
Refactoring code to capture GUID. 0.435
Regex find and replace 0.428
Nested vec! Macro Usage 0.415