SSR Error: HTMLElement Not Defined
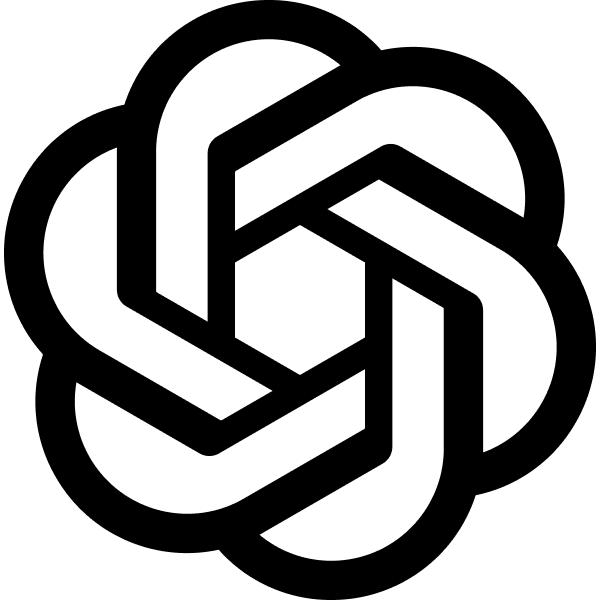
TODO: collapasable, copyable, download, etc
I copied the source code for a complicated Svelte component into my SvelteKit app. I am getting this error Error when evaluating SSR module /src/lib/components/query_builder/svelecte/lib/custom-element.js: |- ReferenceError: HTMLElement is not defined
I see the source code is class SvelecteElement extends HTMLElement { constructor() { ...
Where should HTMLElement come from? How can I make this work?The error you're encountering, "ReferenceError: HTMLElement is not defined," occurs because HTMLElement
, a part of the Web APIs, is not available in a server-side rendering (SSR) environment like SvelteKit during server-side execution. In the browser environment, HTMLElement
is automatically available as it's a standard interface for HTML elements, but during SSR, the server doesn't have access to browser-specific APIs, including the DOM and its elements.
To resolve this issue in your SvelteKit application, you have a few options:
Dynamic Import with SSR Check: You can modify your code to dynamically import the component that extends
HTMLElement
only on the client-side. This can be done by checking if the code is running in a browser environment. Here's a basic example:if (typeof window !== 'undefined') { // Dynamically import your component that extends HTMLElement import('./path/to/SvelecteElement').then((module) => { // Use your component here }); }
Client-side Only Component: If the component is only meant to be used on the client-side, you can tell SvelteKit to only render the component in the browser. This can be achieved using SvelteKit's
<svelte:component>
withthis
binding. For instance:<script> let Component; onMount(() => { Component = import('./path/to/SvelecteElement.svelte'); }); </script> {#if Component} <svelte:component this={Component}/> {/if}
Use a Polyfill: If you absolutely need to have
HTMLElement
or similar browser-specific objects available during SSR, you can use a polyfill. However, this is generally not recommended for typical use cases as it can lead to other complexities and issues.Refactor the Component: If possible, refactor the component to avoid extending
HTMLElement
or using browser-specific APIs in a way that would be executed server-side. This might involve rethinking how the component is structured or finding alternative ways to achieve the same functionality.
Remember that server-side code doesn't have access to the same global objects as client-side code in a browser. Any code that relies on browser-specific APIs should be executed only in the client-side context or should be designed to degrade gracefully in a server-side context.This works when I import this from the npm installed package... should I build the component according to it's git repo package.json and use the dist
version instead of trying to copy the source directly?Yes, using the built and distributed version of a component from an npm package is typically the preferred approach, especially for Svelte and SvelteKit applications. Here's why:
Pre-Compiled Code: The
dist
(distribution) folder usually contains pre-compiled code that is optimized for production. This means the code has already been transformed into a format that's easier for browsers and server environments to handle, potentially including the resolution of issues related to server-side rendering.Dependencies and Build Tools: When you build a component according to its
package.json
, the build process takes care of various dependencies and utilizes specific build tools and configurations (like Babel, Rollup, or Webpack). These tools often include necessary polyfills or transformations to ensure compatibility with different environments, which might be challenging to replicate manually.Simplified Integration: Using the distributed version simplifies integration into your project. It's designed to be imported and used directly, without the need for additional build steps on your end.
Consistency and Stability: The distributed version is typically the version that's been tested and verified by the package maintainers. Using it ensures that you get the same stable and consistent experience as other users of the package.
To use the dist
version of the component, you should:
Install the package via npm (if not already done).
npm install [package-name]
Import the component from the package directly in your Svelte/SvelteKit project.
import SvelecteElement from '[package-name]/dist/svelecte-element';
This approach should help avoid issues like the one you're encountering with HTMLElement
not being defined duringHow / why would this solve the HTMLELement problem?Using the dist
(distribution) version of a component from an npm package might solve the HTMLElement
problem primarily due to how the component is built and packaged for distribution. Here's how and why this approach could be effective:
Server-Side Rendering Compatibility: Components in the
dist
folder are often pre-compiled and built with compatibility in mind for both server-side and client-side environments. The build process typically addresses issues like theHTMLElement
not being defined in a server-side context. This is achieved by either avoiding the use of such browser-specific APIs during server-side rendering or by including checks to ensure that code requiring these APIs is only executed in a browser environment.Different Build Targets: The build process for a library or component can produce different outputs for different environments. For example, a package might have separate builds for Node.js and browser environments. The browser build would use browser-specific objects like
HTMLElement
, while the Node.js build might avoid them or use alternatives.Conditional Code Execution: The built code in the
dist
directory might include conditional logic to handle the server-side rendering case. For instance, it might check if the code is running in a browser and only then useHTMLElement
. This kind of environment-aware code helps prevent server-side rendering errors.Polyfills or Shims: In some cases, the build process might include polyfills or shims for browser-specific objects when the code is intended to run in a non-browser environment. This allows the code to run without errors, though it's less common for something as fundamental as
HTMLElement
.Optimized Code: The distributed version is optimized for performance and compatibility. This optimization includes addressing common issues that might arise in different environments, including both client and server-side rendering contexts.
By using the dist
version, you're leveraging the package maintainers' expertise and their efforts to ensure the component works correctly in various environments, including handling cases like the absence of HTMLElement
in a server-side rendering scenario.here is the dist file ... can you tell what technique solves this? node_modules/svelecte/dist/svelecte-element.js:var Svelecte=function(e){"use strict";function t(){}const n=e=>e;function i(e){return e()}function l(){return Object.create(null)}function r(e){e.forEach(i)}function s(e){return"function"==typeof e}function o(e,t){return e!=e?t==t:e!==t||e&&"object"==typeof e||"function"==typeof e}function a(e,...n){if(null==e)return t;const i=e.subscribe(...n);return i.unsubscribe?()=>i.unsubscribe():i}function c(e,t,n){e.$$.on_destroy.push(a(t,n))}function d(e,t,n,i){if(e){const l=u(e,t,n,i);return e[0](l)}}function u(e,t,n,i){return e[1]&&i?function(e,t){for(const n in t)e[n]=t[n];return e}(n.ctx.slice(),e[1](i(t))):n.ctx}function h(e,t,n,i){if(e[2]&&i){const l=e[2](i(n));if(void 0===t.dirty)return l;if("object"==typeof l){const e=[],n=Math.max(t.dirty.length,l.length);for(let i=0;i<n;i+=1)e[i]=t.dirty[i]|l[i];return e}return t.dirty|l}return t.dirty}function p(e,t,n,i,l,r){if(l){const s=u(t,n,i,r);e.p(s,l)}}function f(e){if(e.ctx.length>32){const t=[],n=e.ctx.length/32;for(let e=0;e<n;e++)t[e]=-1;return t}return-1}function m(e){return null==e?"":e}function g(e,t,n){return e.set(n),t}function v(e){return e&&s(e.destroy)?e.destroy:t}const b="undefined"!=typeof window;let $=b?()=>window.performance.now():()=>Date.now(),y=b?e=>requestAnimationFrame(e):t;const x=new Set;function w(e){x.forEach((t=>{t.c(e)||(x.delete(t),t.f())})),0!==x.size&&y(w)}function S(e,t){e.appendChild(t)}function A(e,t,n){const i=I(e);if(!i.getElementById(t)){const e=k("style");e.id=t,e.textContent=n,z(i,e)}}function I(e){if(!e)return document;const t=e.getRootNode?e.getRootNode():e.ownerDocument;return t&&t.host?t:e.ownerDocument}function z(e,t){S(e.head||e,t)}function C(e,t,n){e.insertBefore(t,n||null)}function O(e){e.parentNode.removeChild(e)}function F(e,t){for(let n=0;n<e.length;n+=1)e[n]&&e[n].d(t)}function k(e){return document.createElement(e)}function E(e){return document.createElementNS("http://www.w3.org/2000/svg",e)}function D(e){return document.createTextNode(e)}function T(){return D(" ")}function M(){return D("")}function L(e,t,n,i){return e.addEventListener(t,n,i),()=>e.removeEventListener(t,n,i)}function _(e){return function(t){return t.preventDefault(),e.call(this,t)}}function H(e,t,n){null==n?e.removeAttribute(t):e.getAttribute(t)!==n&&e.setAttribute(t,n)}function P(e,t){t=""+t,e.wholeText!==t&&(e.data=t)}function V(e,t){e.value=null==t?"":t}let R;function N(){if(void 0===R){R=!1;try{"undefined"!=typeof window&&window.parent&&window.parent.document}catch(e){R=!0}}return R}function B(e,t,n){e.classList[n?"add":"remove"](t)}class j{constructor(){this.e=this.n=null}c(e){this.h(e)}m(e,t,n=null){this.e||(this.e=k(t.nodeName),this.t=t,this.c(e)),this.i(n)}h(e){this.e.innerHTML=e,this.n=Array.from(this.e.childNodes)}i(e){for(let t=0;t<this.n.length;t+=1)C(this.t,this.n[t],e)}p(e){this.d(),this.h(e),this.i(this.a)}d(){this.n.forEach(O)}}const q=new Set;let G,K=0;function W(e,t,n,i,l,r,s,o=0){const a=16.666/i;let c="{\n";for(let e=0;e<=1;e+=a){const i=t+(n-t)*r(e);c+=100*e+`%{${s(i,1-i)}}\n`}const d=c+`100% {${s(n,1-n)}}\n}`,u=`__svelte_${function(e){let t=5381,n=e.length;for(;n--;)t=(t<<5)-t^e.charCodeAt(n);return t>>>0}(d)}_${o}`,h=I(e);q.add(h);const p=h.__svelte_stylesheet||(h.__svelte_stylesheet=function(e){const t=k("style");return z(I(e),t),t}(e).sheet),f=h.__svelte_rules||(h.__svelte_rules={});f[u]||(f[u]=!0,p.insertRule(`@keyframes ${u} ${d}`,p.cssRules.length));const m=e.style.animation||"";return e.style.animation=`${m?`${m}, `:""}${u} ${i}ms linear ${l}ms 1 both`,K+=1,u}function Q(e,t){const n=(e.style.animation||"").split(", "),i=n.filter(t?e=>e.indexOf(t)<0:e=>-1===e.indexOf("__svelte")),l=n.length-i.length;l&&(e.style.animation=i.join(", "),K-=l,K||y((()=>{K||(q.forEach((e=>{const t=e.__svelte_stylesheet;let n=t.cssRules.length;for(;n--;)t.deleteRule(n);e.__svelte_rules={}})),q.clear())})))}function U(e,i,l,r){if(!i)return t;const s=e.getBoundingClientRect();if(i.left===s.left&&i.right===s.right&&i.top===s.top&&i.bottom===s.bottom)return t;const{delay:o=0,duration:a=300,easing:c=n,start:d=$()+o,end:u=d+a,tick:h=t,css:p}=l(e,{from:i,to:s},r);let f,m=!0,g=!1;function v(){p&&Q(e,f),m=!1}return function(e){let t;0===x.size&&y(w),new Promise((n=>{x.add(t={c:e,f:n})}))}((e=>{if(!g&&e>=d&&(g=!0),g&&e>=u&&(h(1,0),v()),!m)return!1;if(g){const t=0+1*c((e-d)/a);h(t,1-t)}return!0})),p&&(f=W(e,0,1,a,o,c,p)),o||(g=!0),h(0,1),v}function J(e){const t=getComputedStyle(e);if("absolute"!==t.position&&"fixed"!==t.position){const{width:n,height:i}=t,l=e.getBoundingClientRect();e.style.position="absolute",e.style.width=n,e.style.height=i,function(e,t){const n=e.getBoundingClientRect();if(t.left!==n.left||t.top!==n.top){const i=getComputedStyle(e),l="none"===i.transform?"":i.transform;e.style.transform=`${l} translate(${t.left-n.left}px, ${t.top-n.top}px)`}}(e,l)}}function X(e){G=e}function Z(){if(!G)throw new Error("Function called outside component initialization");return G}function Y(e){Z().$$.on_mount.push(e)}function ee(e){Z().$$.on_destroy.push(e)}function te(){const e=Z();return(t,n)=>{const i=e.$$.callbacks[t];if(i){const l=function(e,t,n=!1){const i=document.createEvent("CustomEvent");return i.initCustomEvent(e,n,!1,t),i}(t,n);i.slice().forEach((t=>{t.call(e,l)}))}}}function ne(e,t){const n=e.$$.callbacks[t.type];n&&n.slice().forEach((e=>e.call(this,t)))}const ie=[],le=[],re=[],se=[],oe=Promise.resolve();let ae=!1;function ce(){ae||(ae=!0,oe.then(fe))}function de(){return ce(),oe}function ue(e){re.push(e)}let he=!1;const pe=new Set;function fe(){if(!he){he=!0;do{for(let e=0;e<ie.length;e+=1){const t=ie[e];X(t),me(t.$$)}for(X(null),ie.length=0;le.length;)le.pop()();for(let e=0;e<re.length;e+=1){const t=re[e];pe.has(t)||(pe.add(t),t())}re.length=0}while(ie.length);for(;se.length;)se.pop()();ae=!1,he=!1,pe.clear()}}function me(e){if(null!==e.fragment){e.update(),r(e.before_update);const t=e.dirty;e.dirty=[-1],e.fragment&&e.fragment.p(e.ctx,t),e.after_update.forEach(ue)}}const ge=new Set;let ve;function be(){ve={r:0,c:[],p:ve}}function $e(){ve.r||r(ve.c),ve=ve.p}function ye(e,t){e&&e.i&&(ge.delete(e),e.i(t))}function xe(e,t,n,i){if(e&&e.o){if(ge.has(e))return;ge.add(e),ve.c.push((()=>{ge.delete(e),i&&(n&&e.d(1),i())})),e.o(t)}}function we(e,t){xe(e,1,1,(()=>{t.delete(e.key)}))}function Se(e,t){e.f(),we(e,t)}function Ae(e,t,n,i,l,r,s,o,a,c,d,u){let h=e.length,p=r.length,f=h;const m={};for(;f--;)m[e[f].key]=f;const g=[],v=new Map,b=new Map;for(f=p;f--;){const e=u(l,r,f),o=n(e);let a=s.get(o);a?i&&a.p(e,t):(a=c(o,e),a.c()),v.set(o,g[f]=a),o in m&&b.set(o,Math.abs(f-m[o]))}const $=new Set,y=new Set;function x(e){ye(e,1),e.m(o,d),s.set(e.key,e),d=e.first,p--}for(;h&&p;){const t=g[p-1],n=e[h-1],i=t.key,l=n.key;t===n?(d=t.first,h--,p--):v.has(l)?!s.has(i)||$.has(i)?x(t):y.has(l)?h--:b.get(i)>b.get(l)?(y.add(i),x(t)):($.add(l),h--):(a(n,s),h--)}for(;h--;){const t=e[h];v.has(t.key)||a(t,s)}for(;p;)x(g[p-1]);return g}function Ie(e){e&&e.c()}function ze(e,t,n,l){const{fragment:o,on_mount:a,on_destroy:c,after_update:d}=e.$$;o&&o.m(t,n),l||ue((()=>{const t=a.map(i).filter(s);c?c.push(...t):r(t),e.$$.on_mount=[]})),d.forEach(ue)}function Ce(e,t){const n=e.$$;null!==n.fragment&&(r(n.on_destroy),n.fragment&&n.fragment.d(t),n.on_destroy=n.fragment=null,n.ctx=[])}function Oe(e,n,i,s,o,a,c,d=[-1]){const u=G;X(e);const h=e.$$={fragment:null,ctx:null,props:a,update:t,not_equal:o,bound:l(),on_mount:[],on_destroy:[],on_disconnect:[],before_update:[],after_update:[],context:new Map(n.context||(u?u.$$.context:[])),callbacks:l(),dirty:d,skip_bound:!1,root:n.target||u.$$.root};c&&c(h.root);let p=!1;if(h.ctx=i?i(e,n.props||{},((t,n,...i)=>{const l=i.length?i[0]:n;return h.ctx&&o(h.ctx[t],h.ctx[t]=l)&&(!h.skip_bound&&h.bound[t]&&h.bound[t](l),p&&function(e,t){-1===e.$$.dirty[0]&&(ie.push(e),ce(),e.$$.dirty.fill(0)),e.$$.dirty[t/31|0]|=1<<t%31}(e,t)),n})):[],h.update(),p=!0,r(h.before_update),h.fragment=!!s&&s(h.ctx),n.target){if(n.hydrate){const e=function(e){return Array.from(e.childNodes)}(n.target);h.fragment&&h.fragment.l(e),e.forEach(O)}else h.fragment&&h.fragment.c();n.intro&&ye(e.$$.fragment),ze(e,n.target,n.anchor,n.customElement),fe()}X(u)}class Fe{$destroy(){Ce(this,1),this.$destroy=t}$on(e,t){const n=this.$$.callbacks[e]||(this.$$.callbacks[e]=[]);return n.push(t),()=>{const e=n.indexOf(t);-1!==e&&n.splice(e,1)}}$set(e){var t;this.$$set&&(t=e,0!==Object.keys(t).length)&&(this.$$.skip_bound=!0,this.$$set(e),this.$$.skip_bound=!1)}}var ke=function(e,t){this.items=e,this.settings=t||{diacritics:!0}};ke.prototype.tokenize=function(e,t){if(!(e=Me(String(e||"").toLowerCase()))||!e.length)return[];var n,i,l,r,s=[],o=e.split(/ +/);for(n=0,i=o.length;n<i;n++){if(l=Le(o[n]),this.settings.diacritics)for(r in _e)_e.hasOwnProperty(r)&&(l=l.replace(new RegExp(r,"g"),_e[r]));t&&(l="\\b"+l),s.push({string:o[n],regex:new RegExp(l,"i")})}return s},ke.prototype.iterator=function(e,t){var n;n=Array.isArray(e)?Array.prototype.forEach||function(e){for(var t=0,n=this.length;t<n;t++)e(this[t],t,this)}:function(e){for(var t in this)this.hasOwnProperty(t)&&e(this[t],t,this)},n.apply(e,[t])},ke.prototype.getScoreFunction=function(e,t){var n,i,l,r;e=this.prepareSearch(e,t),i=e.tokens,n=e.options.fields,l=i.length,r=e.options.nesting;var s,o=function(e,t){var n,i;return e?-1===(i=(e=String(e||"")).search(t.regex))?0:(n=t.string.length/e.length,0===i&&(n+=.5),n):0},a=(s=n.length)?1===s?function(e,t){return o(Te(t,n[0],r),e)}:function(e,t){for(var i=0,l=0;i<s;i++)l+=o(Te(t,n[i],r),e);return l/s}:function(){return 0};return l?1===l?function(e){return a(i[0],e)}:"and"===e.options.conjunction?function(e){for(var t,n=0,r=0;n<l;n++){if((t=a(i[n],e))<=0)return 0;r+=t}return r/l}:function(e){for(var t=0,n=0;t<l;t++)n+=a(i[t],e);return n/l}:function(){return 0}},ke.prototype.getSortFunction=function(e,t){var n,i,l,r,s,o,a,c,d,u,h;if(h=!(e=(l=this).prepareSearch(e,t)).query&&t.sort_empty||t.sort,d=function(e,n){return"$score"===e?n.score:Te(l.items[n.id],e,t.nesting)},s=[],h)for(n=0,i=h.length;n<i;n++)(e.query||"$score"!==h[n].field)&&s.push(h[n]);if(e.query){for(u=!0,n=0,i=s.length;n<i;n++)if("$score"===s[n].field){u=!1;break}u&&s.unshift({field:"$score",direction:"desc"})}else for(n=0,i=s.length;n<i;n++)if("$score"===s[n].field){s.splice(n,1);break}for(c=[],n=0,i=s.length;n<i;n++)c.push("desc"===s[n].direction?-1:1);return(o=s.length)?1===o?(r=s[0].field,a=c[0],function(e,t){return a*Ee(d(r,e),d(r,t))}):function(e,t){var n,i,l;for(n=0;n<o;n++)if(l=s[n].field,i=c[n]*Ee(d(l,e),d(l,t)))return i;return 0}:null},ke.prototype.prepareSearch=function(e,t){if("object"==typeof e)return e;var n=(t=De({},t)).fields,i=t.sort,l=t.sort_empty;return n&&!Array.isArray(n)&&(t.fields=[n]),i&&!Array.isArray(i)&&(t.sort=[i]),l&&!Array.isArray(l)&&(t.sort_empty=[l]),{options:t,query:String(e||"").toLowerCase(),tokens:this.tokenize(e,t.respect_word_boundaries),total:0,items:[]}},ke.prototype.search=function(e,t){var n,i,l,r,s=this;return i=this.prepareSearch(e,t),t=i.options,e=i.query,r=t.score||s.getScoreFunction(i),e.length?s.iterator(s.items,(function(e,l){n=r(e),(!1===t.filter||n>0)&&i.items.push({score:n,id:l})})):s.iterator(s.items,(function(e,t){i.items.push({score:1,id:t})})),(l=s.getSortFunction(i,t))&&i.items.sort(l),i.total=i.items.length,"number"==typeof t.limit&&(i.items=i.items.slice(0,t.limit)),i};var Ee=function(e,t){return"number"==typeof e&&"number"==typeof t?e>t?1:e<t?-1:0:(e=He(String(e||"")))>(t=He(String(t||"")))?1:t>e?-1:0},De=function(e,t){var n,i,l,r;for(n=1,i=arguments.length;n<i;n++)if(r=arguments[n])for(l in r)r.hasOwnProperty(l)&&(e[l]=r[l]);return e},Te=function(e,t,n){if(e&&t){if(!n)return e[t];for(var i=t.split(".");i.length&&(e=e[i.shift()]););return e}},Me=function(e){return(e+"").replace(/^\s+|\s+$|/g,"")},Le=function(e){return(e+"").replace(/([.?*+^$[\]\\(){}|-])/g,"\\$1")},_e={a:"[aḀḁĂăÂâǍǎȺⱥȦȧẠạÄäÀàÁáĀāÃãÅåąĄÃąĄ]",b:"[b␢βΒB฿𐌁ᛒ]",c:"[cĆćĈĉČčĊċC̄c̄ÇçḈḉȻȼƇƈɕᴄCc]",d:"[dĎďḊḋḐḑḌḍḒḓḎḏĐđD̦d̦ƉɖƊɗƋƌᵭᶁᶑȡᴅDdð]",e:"[eÉéÈèÊêḘḙĚěĔĕẼẽḚḛẺẻĖėËëĒēȨȩĘęᶒɆɇȄȅẾếỀềỄễỂểḜḝḖḗḔḕȆȇẸẹỆệⱸᴇEeɘǝƏƐε]",f:"[fƑƒḞḟ]",g:"[gɢ₲ǤǥĜĝĞğĢģƓɠĠġ]",h:"[hĤĥĦħḨḩẖẖḤḥḢḣɦʰǶƕ]",i:"[iÍíÌìĬĭÎîǏǐÏïḮḯĨĩĮįĪīỈỉȈȉȊȋỊịḬḭƗɨɨ̆ᵻᶖİiIıɪIi]",j:"[jȷĴĵɈɉʝɟʲ]",k:"[kƘƙꝀꝁḰḱǨǩḲḳḴḵκϰ₭]",l:"[lŁłĽľĻļĹĺḶḷḸḹḼḽḺḻĿŀȽƚⱠⱡⱢɫɬᶅɭȴʟLl]",n:"[nŃńǸǹŇňÑñṄṅŅņṆṇṊṋṈṉN̈n̈ƝɲȠƞᵰᶇɳȵɴNnŊŋ]",o:"[oØøÖöÓóÒòÔôǑǒŐőŎŏȮȯỌọƟɵƠơỎỏŌōÕõǪǫȌȍՕօ]",p:"[pṔṕṖṗⱣᵽƤƥᵱ]",q:"[qꝖꝗʠɊɋꝘꝙq̃]",r:"[rŔŕɌɍŘřŖŗṘṙȐȑȒȓṚṛⱤɽ]",s:"[sŚśṠṡṢṣꞨꞩŜŝŠšŞşȘșS̈s̈]",t:"[tŤťṪṫŢţṬṭƮʈȚțṰṱṮṯƬƭ]",u:"[uŬŭɄʉỤụÜüÚúÙùÛûǓǔŰűŬŭƯưỦủŪūŨũŲųȔȕ∪]",v:"[vṼṽṾṿƲʋꝞꝟⱱʋ]",w:"[wẂẃẀẁŴŵẄẅẆẇẈẉ]",x:"[xẌẍẊẋχ]",y:"[yÝýỲỳŶŷŸÿỸỹẎẏỴỵɎɏƳƴ]",z:"[zŹźẐẑŽžŻżẒẓẔẕƵƶ]"};const He=function(){var e,t,n,i,l="",r={};for(n in _e)if(_e.hasOwnProperty(n))for(l+=i=_e[n].substring(2,_e[n].length-1),e=0,t=i.length;e<t;e++)r[i.charAt(e)]=n;var s=new RegExp("["+l+"]","g");return function(e){return e.replace(s,(function(e){return r[e]})).toLowerCase()}}();let Pe,Ve=null;function Re(e,t,n,i,l){const r=i?i(e,t,n):e;if(""==n||e.isSelected||l)return'<div class="sv-item-content">'+r+"</div>";Pe||(Pe=document.createElement("div"),Pe.className="sv-item-content"),Pe.innerHTML=r;return He(n).split(" ").filter((e=>e)).forEach((e=>{Ne(Pe,e)})),Pe.outerHTML}const Ne=function(e,t){let n=0;if(3===e.nodeType){const i=He(e.data);let l=i.indexOf(t);if(l-=i.substr(0,l).toUpperCase().length-i.substr(0,l).length,l>=0){const i=document.createElement("span");i.className="highlight";const r=e.splitText(l);r.splitText(t.length);const s=r.cloneNode(!0);i.appendChild(s),r.parentNode.replaceChild(i,r),n=1}}else if(1===e.nodeType&&e.childNodes&&!/(script|style)/i.test(e.tagName)&&("highlight"!==e.className||"SPAN"!==e.tagName))for(var i=0;i<e.childNodes.length;++i)i+=Ne(e.childNodes[i],t);return n};function Be(e,t,n){const i="value"===e;if(n.isOptionArray)return i?"value":"label";let l=i?"value":"text";if(t&&t.length){const e=t[0][n.optItems]?t[0][n.optItems][0]:t[0];if(!e)return l;const r=i?0:1,s=i?["id","value","ID"]:["name","title","label"];l=Object.keys(e).filter((e=>s.includes(e))).concat([Object.keys(e)[r]]).shift()}return l}function je(e,t){return(e||"").replace(/\t/g," ").trim().split(" ").filter((e=>e)).join(" ")}function qe(e,t,n,i){return{[n]:e,[i]:t+e}}const Ge={disabled:!1,valueField:null,labelField:null,groupLabelField:"label",groupItemsField:"options",disabledField:"$disabled",placeholder:"Select",valueAsObject:!1,searchable:!0,clearable:!1,highlightFirstItem:!0,selectOnTab:null,resetOnBlur:!0,resetOnSelect:!0,fetchResetOnBlur:!0,multiple:!1,closeAfterSelect:!1,max:0,collapseSelection:!1,alwaysCollapsed:!1,creatable:!1,creatablePrefix:"*",keepCreated:!0,allowEditing:!1,delimiter:",",fetchCallback:null,minQuery:1,lazyDropdown:!0,virtualList:!1,vlItemSize:null,vlHeight:null,i18n:{empty:"No options",nomatch:"No matching options",max:e=>`Maximum items ${e} selected`,fetchBefore:"Type to start searching",fetchQuery:(e,t)=>`Type ${e>1&&e>t?`at least ${e-t} characters `:""}to start searching`,fetchInit:"Fetching data, please wait...",fetchEmpty:"No data related to your search",collapsedSelection:e=>`${e} selected`,createRowLabel:e=>`Create '${e}'`},collapseSelectionFn:function(e,t){return this.collapsedSelection(e)}},Ke=[];function We(e,n=t){let i;const l=new Set;function r(t){if(o(e,t)&&(e=t,i)){const t=!Ke.length;for(const t of l)t[1](),Ke.push(t,e);if(t){for(let e=0;e<Ke.length;e+=2)Ke[e][0](Ke[e+1]);Ke.length=0}}}return{set:r,update:function(t){r(t(e))},subscribe:function(s,o=t){const a=[s,o];return l.add(a),1===l.size&&(i=n(r)||t),s(e),()=>{l.delete(a),0===l.size&&(i(),i=null)}}}}function Qe(e,t,n){if(t)return Array.isArray(e)?e:[e];const i=Array.isArray(e)?e:[e],l=n.labelAsValue?n.labelField:n.valueField;return this.reduce(((e,t,r)=>{if(t[n.optItems]&&t[n.optItems].length){const r=t[n.optItems].reduce(((e,t)=>(i.includes(t[l])&&e.push(t),e)),[]);if(r.length)return e.push(...r),e}return i.includes("object"==typeof t?t[l]:n.labelAsValue?t:r)&&(n.isOptionArray&&(t={[n.valueField]:r,[n.labelField]:t}),e.push(t)),e}),[]).sort(((e,t)=>i.indexOf(e[l])<i.indexOf(t[l])?-1:1))}function Ue(e,t){const n=e.reduce(((e,n,i)=>t.isOptionArray?(e.push({[t.valueField]:i,[t.labelField]:n}),e):n[t.optItems]&&Array.isArray(n[t.optItems])?(n[t.optItems].length&&(t.optionsWithGroups=!0,e.push({label:n[t.optLabel],$isGroupHeader:!0}),e.push(...n[t.optItems].map((e=>(e.$isGroupItem=!0,e))))),e):(e.push(n),e)),[]);return function(e,t){t.isOptionArray&&(t.optionProps||(t.optionProps=["value","label"]));e.some((e=>!e.$isGroupHeader&&(t.optionProps=Je(e),!0)))}(n,t),n}function Je(e){e.options&&(e=e.options[0]);const t=["$disabled","$isGroupHeader","$isGroupItem"];return Object.keys(e).filter((e=>!t.includes(e)))}function Xe(e){const t=e-1;return t*t*t+1}function Ze(e,{from:t,to:n},i={}){const l=getComputedStyle(e),r="none"===l.transform?"":l.transform,[o,a]=l.transformOrigin.split(" ").map(parseFloat),c=t.left+t.width*o/n.width-(n.left+o),d=t.top+t.height*a/n.height-(n.top+a),{delay:u=0,duration:h=(e=>120*Math.sqrt(e)),easing:p=Xe}=i;return{delay:u,duration:s(h)?h(Math.sqrt(c*c+d*d)):h,easing:p,css:(e,i)=>{const l=i*c,s=i*d,o=e+i*t.width/n.width,a=e+i*t.height/n.height;return`transform: ${r} translate(${l}px, ${s}px) scale(${o}, ${a});`}}}function Ye(e){A(e,"svelte-x1t6fd",".inputBox.svelte-x1t6fd{box-sizing:content-box;width:19px;background:rgba(0, 0, 0, 0) none repeat scroll 0px center;border:0px none;font-size:inherit;font-family:inherit;opacity:1;outline:currentcolor none 0px;padding:0px;color:inherit;margin:-2px 0 0;height:20px}.inputBox.svelte-x1t6fd::placeholder{color:var(--sv-placeholder-color, #ccccd6)}.inputBox.svelte-x1t6fd:read-only{width:100%}.shadow-text.svelte-x1t6fd{opacity:0;position:absolute;left:100%;z-index:-100;min-width:24px;white-space:nowrap;top:0;left:0}")}function et(e){let n,i,l,s,o,a,c,d;return{c(){n=k("input"),l=T(),s=k("div"),o=D(e[12]),H(n,"type","text"),H(n,"class","inputBox svelte-x1t6fd"),n.disabled=e[2],n.readOnly=i=!e[1],H(n,"id",e[0]),H(n,"style",e[11]),H(n,"placeholder",e[7]),H(n,"enterkeyhint",e[10]),H(n,"inputmode",e[5]),H(s,"class","shadow-text svelte-x1t6fd"),ue((()=>e[29].call(s)))},m(t,i){var r;C(t,n,i),e[27](n),V(n,e[8]),C(t,l,i),C(t,s,i),S(s,o),a=function(e,t){"static"===getComputedStyle(e).position&&(e.style.position="relative");const n=k("iframe");n.setAttribute("style","display: block; position: absolute; top: 0; left: 0; width: 100%; height: 100%; overflow: hidden; border: 0; opacity: 0; pointer-events: none; z-index: -1;"),n.setAttribute("aria-hidden","true"),n.tabIndex=-1;const i=N();let l;return i?(n.src="data:text/html,<script>onresize=function(){parent.postMessage(0,'*')}<\/script>",l=L(window,"message",(e=>{e.source===n.contentWindow&&t()}))):(n.src="about:blank",n.onload=()=>{l=L(n.contentWindow,"resize",t)}),S(e,n),()=>{(i||l&&n.contentWindow)&&l(),O(n)}}(s,e[29].bind(s)),c||(d=[L(n,"input",e[28]),L(n,"focus",e[23]),L(n,"blur",e[24]),L(n,"input",e[15]),L(n,"keydown",e[13]),L(n,"keyup",e[14]),L(n,"paste",e[25]),L(n,"change",(r=e[26],function(e){return e.stopPropagation(),r.call(this,e)}))],c=!0)},p(e,t){4&t[0]&&(n.disabled=e[2]),2&t[0]&&i!==(i=!e[1])&&(n.readOnly=i),1&t[0]&&H(n,"id",e[0]),2048&t[0]&&H(n,"style",e[11]),128&t[0]&&H(n,"placeholder",e[7]),1024&t[0]&&H(n,"enterkeyhint",e[10]),32&t[0]&&H(n,"inputmode",e[5]),256&t[0]&&n.value!==e[8]&&V(n,e[8]),4096&t[0]&&P(o,e[12])},i:t,o:t,d(t){t&&O(n),e[27](null),t&&O(l),t&&O(s),a(),c=!1,r(d)}}}function tt(e,n,i){let l,r,s,o,c,d,u,h,p=t,f=()=>(p(),p=a(S,(e=>i(8,u=e))),S),m=t,v=()=>(m(),m=a(A,(e=>i(31,h=e))),A);e.$$.on_destroy.push((()=>p())),e.$$.on_destroy.push((()=>m()));let{inputId:b}=n,{placeholder:$}=n,{searchable:y}=n,{disabled:x}=n,{multiple:w}=n,{inputValue:S}=n;f();let{hasDropdownOpened:A}=n;v();let{selectedOptions:I}=n,{isAndroid:z}=n,{inputMode:C="text"}=n,O=null,F=0;const k=te();let E=!1;return e.$$set=e=>{"inputId"in e&&i(0,b=e.inputId),"placeholder"in e&&i(17,$=e.placeholder),"searchable"in e&&i(1,y=e.searchable),"disabled"in e&&i(2,x=e.disabled),"multiple"in e&&i(18,w=e.multiple),"inputValue"in e&&f(i(3,S=e.inputValue)),"hasDropdownOpened"in e&&v(i(4,A=e.hasDropdownOpened)),"selectedOptions"in e&&i(19,I=e.selectedOptions),"isAndroid"in e&&i(20,z=e.isAndroid),"inputMode"in e&&i(5,C=e.inputMode)},e.$$.update=()=>{786432&e.$$.dirty[0]&&i(21,l=I.length>0&&!1===w),655360&e.$$.dirty[0]&&i(7,r=I.length>0?"":$),384&e.$$.dirty[0]&&i(12,s=u||r),524288&e.$$.dirty[0]&&i(22,o=0===I.length?19:12),6291520&e.$$.dirty[0]&&i(11,c=`width: ${l?2:F+o}px`),2097152&e.$$.dirty[0]&&i(10,d=l?null:"enter")},[b,y,x,S,A,C,F,r,u,O,d,c,s,function(e){if(z&&!d&&"Enter"===e.key)return!0;E=["Enter","Escape"].includes(e.key)&&h,k("keydown",e)},function(e){E&&(e.stopImmediatePropagation(),e.preventDefault()),E=!1},function(e){1!==I.length||w||g(S,u="",u)},()=>O.focus(),$,w,I,z,l,o,function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(e){le[e?"unshift":"push"]((()=>{O=e,i(9,O)}))},function(){u=this.value,S.set(u)},function(){F=this.clientWidth,i(6,F)}]}class nt extends Fe{constructor(e){super(),Oe(this,e,tt,et,o,{focus:16,inputId:0,placeholder:17,searchable:1,disabled:2,multiple:18,inputValue:3,hasDropdownOpened:4,selectedOptions:19,isAndroid:20,inputMode:5},Ye,[-1,-1])}get focus(){return this.$$.ctx[16]}}function it(e){A(e,"svelte-1l8hgl2",'.sv-control.svelte-1l8hgl2{background-color:var(--sv-bg);border:var(--sv-border);border-radius:4px;min-height:var(--sv-min-height)}.sv-control.is-active.svelte-1l8hgl2{border:var(--sv-active-border);outline:var(--sv-active-outline)}.sv-control.is-disabled.svelte-1l8hgl2{background-color:var(--sv-disabled-bg);border-color:var(--sv-disabled-border-color);cursor:default;flex-wrap:wrap;justify-content:space-between;outline:currentcolor none 0px !important;position:relative;transition:all 100ms ease 0s}.sv-control.svelte-1l8hgl2{display:flex;align-items:center;box-sizing:border-box}.sv-content.svelte-1l8hgl2{align-items:center;display:flex;flex:1 1 0%;flex-wrap:nowrap;padding:0 0 0 6px;position:relative;overflow:hidden;box-sizing:border-box}.sv-content.sv-input-row.has-multiSelection.svelte-1l8hgl2{flex-flow:wrap}.indicator.svelte-1l8hgl2{position:relative;align-items:center;align-self:stretch;display:flex;flex-shrink:0;box-sizing:border-box}.indicator-container.svelte-1l8hgl2{color:var(--sv-icon-color);display:flex;padding:8px;transition:color 150ms ease 0s;box-sizing:border-box}.indicator-container.svelte-1l8hgl2:hover{color:var(--sv-icon-hover) }.indicator-separator.svelte-1l8hgl2{align-self:stretch;background-color:var(--sv-border-color);margin-bottom:8px;margin-top:8px;width:1px;box-sizing:border-box}.is-loading.svelte-1l8hgl2:after{animation:svelte-1l8hgl2-spinAround .5s infinite linear;border:var(--sv-loader-border);border-radius:290486px;border-right-color:transparent;border-top-color:transparent;content:"";display:block;height:20px;width:20px;right:8px;top:calc(50% - 10px);position:absolute !important;box-sizing:border-box}@keyframes svelte-1l8hgl2-spinAround{from{transform:rotate(0deg)\r\n }to{transform:rotate(359deg)\r\n }}')}const lt=e=>({}),rt=e=>({}),st=e=>({hasDropdownOpened:2097152&e[0]}),ot=e=>({hasDropdownOpened:e[21]}),at=e=>({}),ct=e=>({});function dt(e,t,n){const i=e.slice();return i[41]=t[n],i}const ut=e=>({}),ht=e=>({});function pt(e){let t,n,i,l;const r=[mt,ft],s=[];function o(e,t){return e[5]&&e[6]&&e[19]?0:1}return t=o(e),n=s[t]=r[t](e),{c(){n.c(),i=M()},m(e,n){s[t].m(e,n),C(e,i,n),l=!0},p(e,l){let a=t;t=o(e),t===a?s[t].p(e,l):(be(),xe(s[a],1,1,(()=>{s[a]=null})),$e(),n=s[t],n?n.p(e,l):(n=s[t]=r[t](e),n.c()),ye(n,1),n.m(i.parentNode,i))},i(e){l||(ye(n),l=!0)},o(e){xe(n),l=!1},d(e){s[t].d(e),e&&O(i)}}}function ft(e){let t,n,i=[],l=new Map,r=e[11];const s=e=>e[41][e[14]];for(let t=0;t<r.length;t+=1){let n=dt(e,r,t),o=s(n);l.set(o,i[t]=gt(o,n))}return{c(){for(let e=0;e<i.length;e+=1)i[e].c();t=M()},m(e,l){for(let t=0;t<i.length;t+=1)i[t].m(e,l);C(e,t,l),n=!0},p(e,n){if(8439844&n[0]){r=e[11],be();for(let e=0;e<i.length;e+=1)i[e].r();i=Ae(i,n,s,1,e,r,l,t.parentNode,Se,gt,t,dt);for(let e=0;e<i.length;e+=1)i[e].a();$e()}},i(e){if(!n){for(let e=0;e<r.length;e+=1)ye(i[e]);n=!0}},o(e){for(let e=0;e<i.length;e+=1)xe(i[e]);n=!1},d(e){for(let t=0;t<i.length;t+=1)i[t].d(e);e&&O(t)}}}function mt(e){let n,i,l=e[6](e[11].length,e[11])+"";return{c(){n=new j,i=M(),n.a=i},m(e,t){n.m(l,e,t),C(e,i,t)},p(e,t){2112&t[0]&&l!==(l=e[6](e[11].length,e[11])+"")&&n.p(l)},i:t,o:t,d(e){e&&O(i),e&&n.d()}}}function gt(e,n){let i,l,r,s,o,a=t;var c=n[15];function d(e){return{props:{formatter:e[2],item:e[41],isSelected:!0,isMultiple:e[5],inputValue:e[23]}}}return c&&(l=new c(d(n)),l.$on("deselect",n[36])),{key:e,first:null,c(){i=k("div"),l&&Ie(l.$$.fragment),r=T(),this.first=i},m(e,t){C(e,i,t),l&&ze(l,i,null),S(i,r),o=!0},p(e,t){n=e;const s={};if(4&t[0]&&(s.formatter=n[2]),2048&t[0]&&(s.item=n[41]),32&t[0]&&(s.isMultiple=n[5]),8388608&t[0]&&(s.inputValue=n[23]),c!==(c=n[15])){if(l){be();const e=l;xe(e.$$.fragment,1,0,(()=>{Ce(e,1)})),$e()}c?(l=new c(d(n)),l.$on("deselect",n[36]),Ie(l.$$.fragment),ye(l.$$.fragment,1),ze(l,i,r)):l=null}else c&&l.$set(s)},r(){s=i.getBoundingClientRect()},f(){J(i),a()},a(){a(),a=U(i,s,Ze,{duration:yt})},i(e){o||(l&&ye(l.$$.fragment,e),o=!0)},o(e){l&&xe(l.$$.fragment,e),o=!1},d(e){e&&O(i),l&&Ce(l)}}}function vt(e){let t,n,i,l;const s=e[32]["clear-icon"],o=d(s,e,e[31],ct);return{c(){t=k("div"),o&&o.c(),H(t,"aria-hidden","true"),H(t,"class","indicator-container close-icon svelte-1l8hgl2")},m(r,s){C(r,t,s),o&&o.m(t,null),n=!0,i||(l=[L(t,"mousedown",_(e[33])),L(t,"click",e[40])],i=!0)},p(e,t){o&&o.p&&(!n||1&t[1])&&p(o,s,e,e[31],n?h(s,e[31],t,at):f(e[31]),ct)},i(e){n||(ye(o,e),n=!0)},o(e){xe(o,e),n=!1},d(e){e&&O(t),o&&o.d(e),i=!1,r(l)}}}function bt(e){let t;return{c(){t=k("span"),H(t,"class","indicator-separator svelte-1l8hgl2")},m(e,n){C(e,t,n)},d(e){e&&O(t)}}}function $t(e){let t,n,i,l,o,a,c,u,m,g,b,$,y,x,w;const A=e[32].icon,I=d(A,e,e[31],ht);let z=e[11].length&&pt(e),F={disabled:e[3],searchable:e[1],placeholder:e[4],multiple:e[5],inputId:e[7],inputValue:e[8],hasDropdownOpened:e[10],selectedOptions:e[11],isAndroid:e[16],inputMode:e[18]};o=new nt({props:F}),e[37](o),o.$on("focus",e[25]),o.$on("blur",e[26]),o.$on("keydown",e[38]),o.$on("paste",e[39]);let E=e[0]&&!e[3]&&vt(e),D=e[0]&&bt();const M=e[32]["indicator-icon"],_=d(M,e,e[31],ot),P=e[32]["control-end"],V=d(P,e,e[31],rt);return{c(){t=k("div"),I&&I.c(),n=T(),i=k("div"),z&&z.c(),l=T(),Ie(o.$$.fragment),c=T(),u=k("div"),E&&E.c(),m=T(),D&&D.c(),g=T(),b=k("div"),_&&_.c(),$=T(),V&&V.c(),H(i,"class","sv-content sv-input-row svelte-1l8hgl2"),B(i,"has-multiSelection",e[5]),H(b,"aria-hidden","true"),H(b,"class","indicator-container svelte-1l8hgl2"),H(u,"class","indicator svelte-1l8hgl2"),B(u,"is-loading",e[12]),H(t,"class","sv-control svelte-1l8hgl2"),B(t,"is-active",e[22]),B(t,"is-disabled",e[3])},m(r,s){C(r,t,s),I&&I.m(t,null),S(t,n),S(t,i),z&&z.m(i,null),S(i,l),ze(o,i,null),S(t,c),S(t,u),E&&E.m(u,null),S(u,m),D&&D.m(u,null),S(u,g),S(u,b),_&&_.m(b,null),S(t,$),V&&V.m(t,null),y=!0,x||(w=[v(a=e[13].call(null,i,{items:e[11],flipDurationMs:yt,type:e[7]})),L(i,"consider",e[34]),L(i,"finalize",e[35]),L(b,"mousedown",e[27]),L(t,"mousedown",e[17])],x=!0)},p(e,n){I&&I.p&&(!y||1&n[1])&&p(I,A,e,e[31],y?h(A,e[31],n,ut):f(e[31]),ht),e[11].length?z?(z.p(e,n),2048&n[0]&&ye(z,1)):(z=pt(e),z.c(),ye(z,1),z.m(i,l)):z&&(be(),xe(z,1,1,(()=>{z=null})),$e());const r={};8&n[0]&&(r.disabled=e[3]),2&n[0]&&(r.searchable=e[1]),16&n[0]&&(r.placeholder=e[4]),32&n[0]&&(r.multiple=e[5]),128&n[0]&&(r.inputId=e[7]),256&n[0]&&(r.inputValue=e[8]),1024&n[0]&&(r.hasDropdownOpened=e[10]),2048&n[0]&&(r.selectedOptions=e[11]),65536&n[0]&&(r.isAndroid=e[16]),262144&n[0]&&(r.inputMode=e[18]),o.$set(r),a&&s(a.update)&&2176&n[0]&&a.update.call(null,{items:e[11],flipDurationMs:yt,type:e[7]}),32&n[0]&&B(i,"has-multiSelection",e[5]),e[0]&&!e[3]?E?(E.p(e,n),9&n[0]&&ye(E,1)):(E=vt(e),E.c(),ye(E,1),E.m(u,m)):E&&(be(),xe(E,1,1,(()=>{E=null})),$e()),e[0]?D||(D=bt(),D.c(),D.m(u,g)):D&&(D.d(1),D=null),_&&_.p&&(!y||2097152&n[0]|1&n[1])&&p(_,M,e,e[31],y?h(M,e[31],n,st):f(e[31]),ot),4096&n[0]&&B(u,"is-loading",e[12]),V&&V.p&&(!y||1&n[1])&&p(V,P,e,e[31],y?h(P,e[31],n,lt):f(e[31]),rt),4194304&n[0]&&B(t,"is-active",e[22]),8&n[0]&&B(t,"is-disabled",e[3])},i(e){y||(ye(I,e),ye(z),ye(o.$$.fragment,e),ye(E),ye(_,e),ye(V,e),y=!0)},o(e){xe(I,e),xe(z),xe(o.$$.fragment,e),xe(E),xe(_,e),xe(V,e),y=!1},d(n){n&&O(t),I&&I.d(n),z&&z.d(),e[37](null),Ce(o),E&&E.d(),D&&D.d(),_&&_.d(n),V&&V.d(n),x=!1,r(w)}}}const yt=100;function xt(e,n,i){let l,r,s,o=t,c=()=>(o(),o=a(F,(e=>i(21,l=e))),F),d=t,u=()=>(d(),d=a(O,(e=>i(22,r=e))),O),h=t,p=()=>(h(),h=a(C,(e=>i(23,s=e))),C);e.$$.on_destroy.push((()=>o())),e.$$.on_destroy.push((()=>d())),e.$$.on_destroy.push((()=>h()));let{$$slots:f={},$$scope:m}=n,{clearable:v}=n,{searchable:b}=n,{renderer:$}=n,{disabled:y}=n,{placeholder:x}=n,{multiple:w}=n,{resetOnBlur:S}=n,{collapseSelection:A}=n,{alwaysCollapsed:I}=n,{inputId:z}=n,{inputValue:C}=n;p();let{hasFocus:O}=n;u();let{hasDropdownOpened:F}=n;c();let{selectedOptions:k}=n,{isFetchingData:E}=n,{dndzone:D}=n,{currentValueField:T}=n,{itemComponent:M}=n,{isAndroid:L}=n,{isIOS:_}=n,H="none";const P=te();let V,R=!0;return e.$$set=e=>{"clearable"in e&&i(0,v=e.clearable),"searchable"in e&&i(1,b=e.searchable),"renderer"in e&&i(2,$=e.renderer),"disabled"in e&&i(3,y=e.disabled),"placeholder"in e&&i(4,x=e.placeholder),"multiple"in e&&i(5,w=e.multiple),"resetOnBlur"in e&&i(28,S=e.resetOnBlur),"collapseSelection"in e&&i(6,A=e.collapseSelection),"alwaysCollapsed"in e&&i(29,I=e.alwaysCollapsed),"inputId"in e&&i(7,z=e.inputId),"inputValue"in e&&p(i(8,C=e.inputValue)),"hasFocus"in e&&u(i(9,O=e.hasFocus)),"hasDropdownOpened"in e&&c(i(10,F=e.hasDropdownOpened)),"selectedOptions"in e&&i(11,k=e.selectedOptions),"isFetchingData"in e&&i(12,E=e.isFetchingData),"dndzone"in e&&i(13,D=e.dndzone),"currentValueField"in e&&i(14,T=e.currentValueField),"itemComponent"in e&&i(15,M=e.itemComponent),"isAndroid"in e&&i(16,L=e.isAndroid),"isIOS"in e&&i(30,_=e.isIOS),"$$scope"in e&&i(31,m=e.$$scope)},[v,b,$,y,x,w,A,z,C,O,F,k,E,D,T,M,L,function(e){if(!y){if(!e)return!r&&V.focus(),void g(F,l=!0,l);if("INPUT"!==e?.target.tagName&&e.preventDefault(),r){if((L||_)&&"text"!==H)return void i(18,H="text");if("INPUT"===e?.target.tagName&&s)return;if("text"===H)return i(18,H="none"),void g(F,l=!0,l);g(F,l=!l,l)}else V.focus()}},H,R,V,l,r,s,P,function(){g(O,r=!0,r),g(F,l=!0,l),!I&&setTimeout((()=>{i(19,R=!1)}),150)},function(){i(18,H="none"),g(O,r=!1,r),g(F,l=!1,l),S&&g(C,s="",s),!I&&setTimeout((()=>{i(19,R=!0)}),100),P("blur")},function(e){e.preventDefault(),e.stopPropagation(),i(18,H="none"),r?g(F,l=!l,l):V.focus()},S,I,_,m,f,function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(e){le[e?"unshift":"push"]((()=>{V=e,i(20,V)}))},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},()=>P("deselect")]}class wt extends Fe{constructor(e){super(),Oe(this,e,xt,$t,o,{clearable:0,searchable:1,renderer:2,disabled:3,placeholder:4,multiple:5,resetOnBlur:28,collapseSelection:6,alwaysCollapsed:29,inputId:7,inputValue:8,hasFocus:9,hasDropdownOpened:10,selectedOptions:11,isFetchingData:12,dndzone:13,currentValueField:14,itemComponent:15,isAndroid:16,isIOS:30,focusControl:17},it,[-1,-1])}get focusControl(){return this.$$.ctx[17]}}const St="start",At="center",It="end",zt={HORIZONTAL:"horizontal",VERTICAL:"vertical"},Ct=0,Ot=1,Ft={[zt.VERTICAL]:"scrollTop",[zt.HORIZONTAL]:"scrollLeft"};class kt{constructor({itemSize:e,itemCount:t,estimatedItemSize:n}){this.itemSize=e,this.itemCount=t,this.estimatedItemSize=n,this.itemSizeAndPositionData={},this.lastMeasuredIndex=-1,this.checkForMismatchItemSizeAndItemCount(),this.justInTime||this.computeTotalSizeAndPositionData()}get justInTime(){return"function"==typeof this.itemSize}updateConfig({itemSize:e,itemCount:t,estimatedItemSize:n}){null!=t&&(this.itemCount=t),null!=n&&(this.estimatedItemSize=n),null!=e&&(this.itemSize=e),this.checkForMismatchItemSizeAndItemCount(),this.justInTime&&null!=this.totalSize?this.totalSize=void 0:this.computeTotalSizeAndPositionData()}checkForMismatchItemSizeAndItemCount(){if(Array.isArray(this.itemSize)&&this.itemSize.length<this.itemCount)throw Error("When itemSize is an array, itemSize.length can't be smaller than itemCount")}getSize(e){const{itemSize:t}=this;return"function"==typeof t?t(e):Array.isArray(t)?t[e]:t}computeTotalSizeAndPositionData(){let e=0;for(let t=0;t<this.itemCount;t++){const n=this.getSize(t),i=e;e+=n,this.itemSizeAndPositionData[t]={offset:i,size:n}}this.totalSize=e}getLastMeasuredIndex(){return this.lastMeasuredIndex}getSizeAndPositionForIndex(e){if(e<0||e>=this.itemCount)throw Error(`Requested index ${e} is outside of range 0..${this.itemCount}`);return this.justInTime?this.getJustInTimeSizeAndPositionForIndex(e):this.itemSizeAndPositionData[e]}getJustInTimeSizeAndPositionForIndex(e){if(e>this.lastMeasuredIndex){const t=this.getSizeAndPositionOfLastMeasuredItem();let n=t.offset+t.size;for(let t=this.lastMeasuredIndex+1;t<=e;t++){const e=this.getSize(t);if(null==e||isNaN(e))throw Error(`Invalid size returned for index ${t} of value ${e}`);this.itemSizeAndPositionData[t]={offset:n,size:e},n+=e}this.lastMeasuredIndex=e}return this.itemSizeAndPositionData[e]}getSizeAndPositionOfLastMeasuredItem(){return this.lastMeasuredIndex>=0?this.itemSizeAndPositionData[this.lastMeasuredIndex]:{offset:0,size:0}}getTotalSize(){if(this.totalSize)return this.totalSize;const e=this.getSizeAndPositionOfLastMeasuredItem();return e.offset+e.size+(this.itemCount-this.lastMeasuredIndex-1)*this.estimatedItemSize}getUpdatedOffsetForIndex({align:e=St,containerSize:t,currentOffset:n,targetIndex:i}){if(t<=0)return 0;const l=this.getSizeAndPositionForIndex(i),r=l.offset,s=r-t+l.size;let o;switch(e){case It:o=s;break;case At:o=r-(t-l.size)/2;break;case St:o=r;break;default:o=Math.max(s,Math.min(r,n))}const a=this.getTotalSize();return Math.max(0,Math.min(a-t,o))}getVisibleRange({containerSize:e=0,offset:t,overscanCount:n}){if(0===this.getTotalSize())return{};const i=t+e;let l=this.findNearestItem(t);if(void 0===l)throw Error(`Invalid offset ${t} specified`);const r=this.getSizeAndPositionForIndex(l);t=r.offset+r.size;let s=l;for(;t<i&&s<this.itemCount-1;)s++,t+=this.getSizeAndPositionForIndex(s).size;return n&&(l=Math.max(0,l-n),s=Math.min(s+n,this.itemCount-1)),{start:l,stop:s}}resetItem(e){this.lastMeasuredIndex=Math.min(this.lastMeasuredIndex,e-1)}findNearestItem(e){if(isNaN(e))throw Error(`Invalid offset ${e} specified`);e=Math.max(0,e);const t=this.getSizeAndPositionOfLastMeasuredItem(),n=Math.max(0,this.lastMeasuredIndex);return t.offset>=e?this.binarySearch({high:n,low:0,offset:e}):this.exponentialSearch({index:n,offset:e})}binarySearch({low:e,high:t,offset:n}){let i=0,l=0;for(;e<=t;){if(i=e+Math.floor((t-e)/2),l=this.getSizeAndPositionForIndex(i).offset,l===n)return i;l<n?e=i+1:l>n&&(t=i-1)}return e>0?e-1:0}exponentialSearch({index:e,offset:t}){let n=1;for(;e<this.itemCount&&this.getSizeAndPositionForIndex(e).offset<t;)e+=n,n*=2;return this.binarySearch({high:Math.min(e,this.itemCount-1),low:Math.floor(e/2),offset:t})}}function Et(e){A(e,"svelte-1he1ex4",".virtual-list-wrapper.svelte-1he1ex4{overflow:auto;will-change:transform;-webkit-overflow-scrolling:touch}.virtual-list-inner.svelte-1he1ex4{position:relative;display:flex;width:100%;min-height:100%}")}const Dt=e=>({}),Tt=e=>({});function Mt(e,t,n){const i=e.slice();return i[36]=t[n],i}const Lt=e=>({style:4&e[0],index:4&e[0]}),_t=e=>({style:e[36].style,index:e[36].index}),Ht=e=>({}),Pt=e=>({});function Vt(e,t){let n,i;const l=t[20].item,r=d(l,t,t[19],_t);return{key:e,first:null,c(){n=M(),r&&r.c(),this.first=n},m(e,t){C(e,n,t),r&&r.m(e,t),i=!0},p(e,n){t=e,r&&r.p&&(!i||524292&n[0])&&p(r,l,t,t[19],i?h(l,t[19],n,Lt):f(t[19]),_t)},i(e){i||(ye(r,e),i=!0)},o(e){xe(r,e),i=!1},d(e){e&&O(n),r&&r.d(e)}}}function Rt(e){let t,n,i,l,r,s=[],o=new Map;const a=e[20].header,c=d(a,e,e[19],Pt);let u=e[2];const m=e=>e[0]?e[0](e[36].index):e[36].index;for(let t=0;t<u.length;t+=1){let n=Mt(e,u,t),i=m(n);o.set(i,s[t]=Vt(i,n))}const g=e[20].footer,v=d(g,e,e[19],Tt);return{c(){t=k("div"),c&&c.c(),n=T(),i=k("div");for(let e=0;e<s.length;e+=1)s[e].c();l=T(),v&&v.c(),H(i,"class","virtual-list-inner svelte-1he1ex4"),H(i,"style",e[4]),H(t,"class","virtual-list-wrapper svelte-1he1ex4"),H(t,"style",e[3])},m(o,a){C(o,t,a),c&&c.m(t,null),S(t,n),S(t,i);for(let e=0;e<s.length;e+=1)s[e].m(i,null);S(t,l),v&&v.m(t,null),e[21](t),r=!0},p(e,n){c&&c.p&&(!r||524288&n[0])&&p(c,a,e,e[19],r?h(a,e[19],n,Ht):f(e[19]),Pt),524293&n[0]&&(u=e[2],be(),s=Ae(s,n,m,1,e,u,o,i,we,Vt,null,Mt),$e()),(!r||16&n[0])&&H(i,"style",e[4]),v&&v.p&&(!r||524288&n[0])&&p(v,g,e,e[19],r?h(g,e[19],n,Dt):f(e[19]),Tt),(!r||8&n[0])&&H(t,"style",e[3])},i(e){if(!r){ye(c,e);for(let e=0;e<u.length;e+=1)ye(s[e]);ye(v,e),r=!0}},o(e){xe(c,e);for(let e=0;e<s.length;e+=1)xe(s[e]);xe(v,e),r=!1},d(n){n&&O(t),c&&c.d(n);for(let e=0;e<s.length;e+=1)s[e].d();v&&v.d(n),e[21](null)}}}const Nt=(()=>{let e=!1;try{const t=Object.defineProperty({},"passive",{get:()=>(e={passive:!0},!0)});window.addEventListener("testpassive",t,t),window.remove("testpassive",t,t)}catch(e){}return e})();function Bt(e,t,n){let{$$slots:i={},$$scope:l}=t,{height:r}=t,{width:s="100%"}=t,{itemCount:o}=t,{itemSize:a}=t,{estimatedItemSize:c=null}=t,{stickyIndices:d=null}=t,{getKey:u=null}=t,{scrollDirection:h=zt.VERTICAL}=t,{scrollOffset:p=null}=t,{scrollToIndex:f=null}=t,{scrollToAlignment:m=null}=t,{overscanCount:g=3}=t;const v=te(),b=new kt({itemCount:o,itemSize:a,estimatedItemSize:T()});let $,y=!1,x=[],w={offset:p||null!=f&&x.length&&E(f)||0,scrollChangeReason:Ot},S=w,A={scrollToIndex:f,scrollToAlignment:m,scrollOffset:p,itemCount:o,itemSize:a,estimatedItemSize:c},I={},z="",C="";function O(){const{offset:e}=w,{start:t,stop:i}=b.getVisibleRange({containerSize:h===zt.VERTICAL?r:s,offset:e,overscanCount:g});let l=[];const o=b.getTotalSize();h===zt.VERTICAL?(n(3,z=`height:${r}px;width:${s};`),n(4,C=`flex-direction:column;height:${o}px;`)):(n(3,z=`height:${r};width:${s}px`),n(4,C=`width:${o}px;`));const a=null!=d&&0!==d.length;if(a)for(let e=0;e<d.length;e++){const t=d[e];l.push({index:t,style:M(t,!0)})}if(void 0!==t&&void 0!==i){for(let e=t;e<=i;e++)a&&d.includes(e)||l.push({index:e,style:M(e,!1)});v("itemsUpdated",{start:t,end:i})}n(2,x=l)}function F(e){n(1,$[Ft[h]]=e,$)}function k(e=0){I={},b.resetItem(e),O()}function E(e,t=m,n=o){return(e<0||e>=n)&&(e=0),b.getUpdatedOffsetForIndex({align:t,containerSize:h===zt.VERTICAL?r:s,currentOffset:w.offset||0,targetIndex:e})}function D(e){const t=$[Ft[h]];t<0||w.offset===t||e.target!==$||(n(18,w={offset:t,scrollChangeReason:Ct}),v("afterScroll",{offset:t,event:e}))}function T(){return c||"number"==typeof a&&a||50}function M(e,t){if(I[e])return I[e];const{size:n,offset:i}=b.getSizeAndPositionForIndex(e);let l;return h===zt.VERTICAL?(l=`left:0;width:100%;height:${n}px;`,l+=t?`position:sticky;flex-grow:0;z-index:1;top:0;margin-top:${i}px;margin-bottom:${-(i+n)}px;`:`position:absolute;top:${i}px;`):(l=`top:0;width:${n}px;`,l+=t?`position:sticky;z-index:1;left:0;margin-left:${i}px;margin-right:${-(i+n)}px;`:`position:absolute;height:100%;left:${i}px;`),I[e]=l}return O(),Y((()=>{n(17,y=!0),$.addEventListener("scroll",D,Nt),null!=p?F(p):null!=f&&F(E(f))})),ee((()=>{y&&$.removeEventListener("scroll",D)})),e.$$set=e=>{"height"in e&&n(5,r=e.height),"width"in e&&n(6,s=e.width),"itemCount"in e&&n(7,o=e.itemCount),"itemSize"in e&&n(8,a=e.itemSize),"estimatedItemSize"in e&&n(9,c=e.estimatedItemSize),"stickyIndices"in e&&n(10,d=e.stickyIndices),"getKey"in e&&n(0,u=e.getKey),"scrollDirection"in e&&n(11,h=e.scrollDirection),"scrollOffset"in e&&n(12,p=e.scrollOffset),"scrollToIndex"in e&&n(13,f=e.scrollToIndex),"scrollToAlignment"in e&&n(14,m=e.scrollToAlignment),"overscanCount"in e&&n(15,g=e.overscanCount),"$$scope"in e&&n(19,l=e.$$scope)},e.$$.update=()=>{29568&e.$$.dirty[0]&&function(){if(!y)return;const e=A.scrollToIndex!==f||A.scrollToAlignment!==m,t=A.itemCount!==o||A.itemSize!==a||A.estimatedItemSize!==c;t&&(b.updateConfig({itemSize:a,itemCount:o,estimatedItemSize:T()}),k()),A.scrollOffset!==p?n(18,w={offset:p||0,scrollChangeReason:Ot}):"number"==typeof f&&(e||t)&&n(18,w={offset:E(f,m,o),scrollChangeReason:Ot}),A={scrollToIndex:f,scrollToAlignment:m,scrollOffset:p,itemCount:o,itemSize:a,estimatedItemSize:c}}(),262144&e.$$.dirty[0]&&function(){if(!y)return;const{offset:e,scrollChangeReason:t}=w;S.offset===e&&S.scrollChangeReason===t||O(),S.offset!==e&&t===Ot&&F(e),S=w}(),132192&e.$$.dirty[0]&&y&&k(r)},[u,$,x,z,C,r,s,o,a,c,d,h,p,f,m,g,k,y,w,l,i,function(e){le[e?"unshift":"push"]((()=>{$=e,n(1,$)}))}]}class jt extends Fe{constructor(e){super(),Oe(this,e,Bt,Rt,o,{height:5,width:6,itemCount:7,itemSize:8,estimatedItemSize:9,stickyIndices:10,getKey:0,scrollDirection:11,scrollOffset:12,scrollToIndex:13,scrollToAlignment:14,overscanCount:15,recomputeSizes:16},Et,[-1,-1])}get recomputeSizes(){return this.$$.ctx[16]}}function qt(e){A(e,"svelte-9227bl",'.sv-dropdown.svelte-9227bl.svelte-9227bl{box-sizing:border-box;position:absolute;background-color:var(--sv-bg);width:100%;display:none;overflow-y:auto;overflow-x:hidden;border:1px solid rgba(0,0,0,0.15);border-radius:.25rem;box-shadow:var(--sv-dropdown-shadow);z-index:2}.sv-dropdown.is-virtual.svelte-9227bl .sv-dropdown-scroll.svelte-9227bl{overflow-y:hidden}.sv-dropdown-scroll.svelte-9227bl.svelte-9227bl{padding:4px;box-sizing:border-box;max-height:var(--sv-dropdown-height);overflow-y:auto;overflow-x:hidden}.sv-dropdown-scroll.is-empty.svelte-9227bl.svelte-9227bl{padding:0}.sv-dropdown[aria-expanded="true"].svelte-9227bl.svelte-9227bl{display:block}.sv-dropdown-content.max-reached.svelte-9227bl.svelte-9227bl{opacity:0.75;cursor:not-allowed}.sv-dropdown-scroll.svelte-9227bl:not(.is-empty)+.creatable-row-wrap.svelte-9227bl{border-top:1px solid #efefef}.creatable-row-wrap.svelte-9227bl.svelte-9227bl{padding:4px}.creatable-row.svelte-9227bl.svelte-9227bl{box-sizing:border-box;display:flex;justify-content:space-between;align-items:center;border-radius:2px;padding:3px 3px 3px 6px}.creatable-row.svelte-9227bl.svelte-9227bl:hover,.creatable-row.svelte-9227bl.svelte-9227bl:active,.creatable-row.active.svelte-9227bl.svelte-9227bl{background-color:var(--sv-item-active-bg)}.creatable-row.active.is-disabled.svelte-9227bl.svelte-9227bl{opacity:0.5;background-color:rgb(252, 186, 186)}.creatable-row.is-disabled.svelte-9227bl.svelte-9227bl{opacity:0.5;cursor:not-allowed}.shortcut.svelte-9227bl.svelte-9227bl{display:flex;align-items:center;align-content:center}.shortcut.svelte-9227bl>kbd.svelte-9227bl{border:1px solid #efefef;border-radius:4px;padding:0px 6px;margin:-1px 0;background-color:white;line-height:1.6;height:22px}.empty-list-row.svelte-9227bl.svelte-9227bl{min-width:0px;box-sizing:border-box;border-radius:2px;text-overflow:ellipsis;white-space:nowrap;box-sizing:border-box;border-radius:2px;overflow:hidden;padding:7px 7px 7px 10px;text-align:left}.alwaysCollapsed-selection.has-multiSelection.svelte-9227bl.svelte-9227bl{padding:4px 4px 0;display:flex;flex-wrap:wrap}')}function Gt(e,t,n){const i=e.slice();return i[51]=t[n],i[53]=n,i}function Kt(e,t,n){const i=e.slice();return i[51]=t[n],i[53]=n,i}function Wt(e){let t,n,i,l,r,s,o,a,c,d=e[17]&&Qt(e),u=e[5].length&&Jt(e),h=(e[19]||e[2])&&tn(e),p=e[8]&&e[1]&&!e[2]&&nn(e);return{c(){t=k("div"),d&&d.c(),n=T(),i=k("div"),l=k("div"),u&&u.c(),r=T(),h&&h.c(),s=T(),p&&p.c(),H(l,"class","sv-dropdown-content svelte-9227bl"),B(l,"max-reached",e[2]),H(i,"class","sv-dropdown-scroll svelte-9227bl"),H(i,"tabindex","-1"),B(i,"is-empty",!e[5].length),H(t,"class","sv-dropdown svelte-9227bl"),H(t,"aria-expanded",e[27]),B(t,"is-virtual",e[7])},m(f,m){C(f,t,m),d&&d.m(t,null),S(t,n),S(t,i),S(i,l),u&&u.m(l,null),S(l,r),h&&h.m(l,null),e[42](l),e[43](i),S(t,s),p&&p.m(t,null),o=!0,a||(c=L(t,"mousedown",_(e[35])),a=!0)},p(e,s){e[17]?d?(d.p(e,s),131072&s[0]&&ye(d,1)):(d=Qt(e),d.c(),ye(d,1),d.m(t,n)):d&&(be(),xe(d,1,1,(()=>{d=null})),$e()),e[5].length?u?(u.p(e,s),32&s[0]&&ye(u,1)):(u=Jt(e),u.c(),ye(u,1),u.m(l,r)):u&&(be(),xe(u,1,1,(()=>{u=null})),$e()),e[19]||e[2]?h?h.p(e,s):(h=tn(e),h.c(),h.m(l,null)):h&&(h.d(1),h=null),4&s[0]&&B(l,"max-reached",e[2]),32&s[0]&&B(i,"is-empty",!e[5].length),e[8]&&e[1]&&!e[2]?p?p.p(e,s):(p=nn(e),p.c(),p.m(t,null)):p&&(p.d(1),p=null),(!o||134217728&s[0])&&H(t,"aria-expanded",e[27]),128&s[0]&&B(t,"is-virtual",e[7])},i(e){o||(ye(d),ye(u),o=!0)},o(e){xe(d),xe(u),o=!1},d(n){n&&O(t),d&&d.d(),u&&u.d(),h&&h.d(),e[42](null),e[43](null),p&&p.d(),a=!1,c()}}}function Qt(e){let t,n,i=[],l=new Map,r=e[17];const s=e=>e[53];for(let t=0;t<r.length;t+=1){let n=Kt(e,r,t),o=s(n);l.set(o,i[t]=Ut(o,n))}return{c(){t=k("div");for(let e=0;e<i.length;e+=1)i[e].c();H(t,"class","sv-content has-multiSelection alwaysCollapsed-selection svelte-9227bl")},m(e,l){C(e,t,l);for(let e=0;e<i.length;e+=1)i[e].m(t,null);n=!0},p(e,n){197384&n[0]&&(r=e[17],be(),i=Ae(i,n,s,1,e,r,l,t,we,Ut,null,Kt),$e())},i(e){if(!n){for(let e=0;e<r.length;e+=1)ye(i[e]);n=!0}},o(e){for(let e=0;e<i.length;e+=1)xe(i[e]);n=!1},d(e){e&&O(t);for(let e=0;e<i.length;e+=1)i[e].d()}}}function Ut(e,t){let n,i,l,r;var s=t[16];function o(e){return{props:{formatter:e[3],item:e[51],isSelected:!0,isMultiple:e[9],inputValue:e[8]}}}return s&&(i=new s(o(t)),i.$on("deselect",t[36])),{key:e,first:null,c(){n=M(),i&&Ie(i.$$.fragment),l=M(),this.first=n},m(e,t){C(e,n,t),i&&ze(i,e,t),C(e,l,t),r=!0},p(e,n){t=e;const r={};if(8&n[0]&&(r.formatter=t[3]),131072&n[0]&&(r.item=t[51]),512&n[0]&&(r.isMultiple=t[9]),256&n[0]&&(r.inputValue=t[8]),s!==(s=t[16])){if(i){be();const e=i;xe(e.$$.fragment,1,0,(()=>{Ce(e,1)})),$e()}s?(i=new s(o(t)),i.$on("deselect",t[36]),Ie(i.$$.fragment),ye(i.$$.fragment,1),ze(i,l.parentNode,l)):i=null}else s&&i.$set(r)},i(e){r||(i&&ye(i.$$.fragment,e),r=!0)},o(e){i&&xe(i.$$.fragment,e),r=!1},d(e){e&&O(n),e&&O(l),i&&Ce(i,e)}}}function Jt(e){let t,n,i,l;const r=[Zt,Xt],s=[];function o(e,t){return e[7]?0:1}return t=o(e),n=s[t]=r[t](e),{c(){n.c(),i=M()},m(e,n){s[t].m(e,n),C(e,i,n),l=!0},p(e,l){let a=t;t=o(e),t===a?s[t].p(e,l):(be(),xe(s[a],1,1,(()=>{s[a]=null})),$e(),n=s[t],n?n.p(e,l):(n=s[t]=r[t](e),n.c()),ye(n,1),n.m(i.parentNode,i))},i(e){l||(ye(n),l=!0)},o(e){xe(n),l=!1},d(e){s[t].d(e),e&&O(i)}}}function Xt(e){let t,n,i=e[5],l=[];for(let t=0;t<i.length;t+=1)l[t]=Yt(Gt(e,i,t));const r=e=>xe(l[e],1,1,(()=>{l[e]=null}));return{c(){for(let e=0;e<l.length;e+=1)l[e].c();t=M()},m(e,i){for(let t=0;t<l.length;t+=1)l[t].m(e,i);C(e,t,i),n=!0},p(e,n){if(75065&n[0]){let s;for(i=e[5],s=0;s<i.length;s+=1){const r=Gt(e,i,s);l[s]?(l[s].p(r,n),ye(l[s],1)):(l[s]=Yt(r),l[s].c(),ye(l[s],1),l[s].m(t.parentNode,t))}for(be(),s=i.length;s<l.length;s+=1)r(s);$e()}},i(e){if(!n){for(let e=0;e<i.length;e+=1)ye(l[e]);n=!0}},o(e){l=l.filter(Boolean);for(let e=0;e<l.length;e+=1)xe(l[e]);n=!1},d(e){F(l,e),e&&O(t)}}}function Zt(e){let t,n,i={width:"100%",height:e[25],itemCount:e[5].length,itemSize:e[21],scrollToAlignment:"auto",scrollToIndex:!e[9]&&e[0]?parseInt(e[0]):null,$$slots:{item:[en,({style:e,index:t})=>({49:e,50:t}),({style:e,index:t})=>[0,(e?262144:0)|(t?524288:0)]]},$$scope:{ctx:e}};return t=new jt({props:i}),e[39](t),{c(){Ie(t.$$.fragment)},m(e,i){ze(t,e,i),n=!0},p(e,n){const i={};33554432&n[0]&&(i.height=e[25]),32&n[0]&&(i.itemCount=e[5].length),2097152&n[0]&&(i.itemSize=e[21]),513&n[0]&&(i.scrollToIndex=!e[9]&&e[0]?parseInt(e[0]):null),75065&n[0]|17563648&n[1]&&(i.$$scope={dirty:n,ctx:e}),t.$set(i)},i(e){n||(ye(t.$$.fragment,e),n=!0)},o(e){xe(t.$$.fragment,e),n=!1},d(n){e[39](null),Ce(t,n)}}}function Yt(e){let t,n,i,l,r;var s=e[16];function o(e){return{props:{formatter:e[3],index:e[10].map[e[53]],isDisabled:e[51][e[13]],item:e[51],inputValue:e[8],disableHighlight:e[4]}}}return s&&(n=new s(o(e)),n.$on("hover",e[40]),n.$on("select",e[41])),{c(){t=k("div"),n&&Ie(n.$$.fragment),i=T(),H(t,"data-pos",l=e[10].map[e[53]]),H(t,"class","sv-dd-item"),B(t,"sv-dd-item-active",e[10].map[e[53]]==e[0]),B(t,"sv-group-item",e[51].$isGroupItem),B(t,"sv-group-header",e[51].$isGroupHeader)},m(e,l){C(e,t,l),n&&ze(n,t,null),S(t,i),r=!0},p(e,a){const c={};if(8&a[0]&&(c.formatter=e[3]),1024&a[0]&&(c.index=e[10].map[e[53]]),8224&a[0]&&(c.isDisabled=e[51][e[13]]),32&a[0]&&(c.item=e[51]),256&a[0]&&(c.inputValue=e[8]),16&a[0]&&(c.disableHighlight=e[4]),s!==(s=e[16])){if(n){be();const e=n;xe(e.$$.fragment,1,0,(()=>{Ce(e,1)})),$e()}s?(n=new s(o(e)),n.$on("hover",e[40]),n.$on("select",e[41]),Ie(n.$$.fragment),ye(n.$$.fragment,1),ze(n,t,i)):n=null}else s&&n.$set(c);(!r||1024&a[0]&&l!==(l=e[10].map[e[53]]))&&H(t,"data-pos",l),1025&a[0]&&B(t,"sv-dd-item-active",e[10].map[e[53]]==e[0]),32&a[0]&&B(t,"sv-group-item",e[51].$isGroupItem),32&a[0]&&B(t,"sv-group-header",e[51].$isGroupHeader)},i(e){r||(n&&ye(n.$$.fragment,e),r=!0)},o(e){n&&xe(n.$$.fragment,e),r=!1},d(e){e&&O(t),n&&Ce(n)}}}function en(e){let t,n,i,l;var r=e[16];function s(e){return{props:{formatter:e[3],index:e[10].map[e[50]],isDisabled:e[5][e[50]][e[13]],item:e[5][e[50]],inputValue:e[8],disableHighlight:e[4]}}}return r&&(n=new r(s(e)),n.$on("hover",e[37]),n.$on("select",e[38])),{c(){t=k("div"),n&&Ie(n.$$.fragment),H(t,"slot","item"),H(t,"style",i=e[49]),H(t,"class","sv-dd-item"),B(t,"sv-dd-item-active",e[50]==e[0]),B(t,"sv-group-item",e[5][e[50]].$isGroupItem),B(t,"sv-group-header",e[5][e[50]].$isGroupHeader)},m(e,i){C(e,t,i),n&&ze(n,t,null),l=!0},p(e,o){const a={};if(8&o[0]&&(a.formatter=e[3]),1024&o[0]|524288&o[1]&&(a.index=e[10].map[e[50]]),8224&o[0]|524288&o[1]&&(a.isDisabled=e[5][e[50]][e[13]]),32&o[0]|524288&o[1]&&(a.item=e[5][e[50]]),256&o[0]&&(a.inputValue=e[8]),16&o[0]&&(a.disableHighlight=e[4]),r!==(r=e[16])){if(n){be();const e=n;xe(e.$$.fragment,1,0,(()=>{Ce(e,1)})),$e()}r?(n=new r(s(e)),n.$on("hover",e[37]),n.$on("select",e[38]),Ie(n.$$.fragment),ye(n.$$.fragment,1),ze(n,t,null)):n=null}else r&&n.$set(a);(!l||262144&o[1]&&i!==(i=e[49]))&&H(t,"style",i),1&o[0]|524288&o[1]&&B(t,"sv-dd-item-active",e[50]==e[0]),32&o[0]|524288&o[1]&&B(t,"sv-group-item",e[5][e[50]].$isGroupItem),32&o[0]|524288&o[1]&&B(t,"sv-group-header",e[5][e[50]].$isGroupHeader)},i(e){l||(n&&ye(n.$$.fragment,e),l=!0)},o(e){n&&xe(n.$$.fragment,e),l=!1},d(e){e&&O(t),n&&Ce(n)}}}function tn(e){let t,n;return{c(){t=k("div"),n=D(e[12]),H(t,"class","empty-list-row svelte-9227bl")},m(e,i){C(e,t,i),S(t,n)},p(e,t){4096&t[0]&&P(n,e[12])},d(e){e&&O(t)}}}function nn(e){let t,n,i,l,o,a,c=e[14](e[8])+"",d=e[26]!=e[0]&&ln(e);return{c(){t=k("div"),n=k("div"),i=new j,l=T(),d&&d.c(),i.a=l,H(n,"class","creatable-row svelte-9227bl"),B(n,"active",e[26]==e[0]),B(n,"is-disabled",e[6].includes(e[8])),H(t,"class","creatable-row-wrap svelte-9227bl")},m(r,u){C(r,t,u),S(t,n),i.m(c,n),S(n,l),d&&d.m(n,null),o||(a=[L(n,"click",(function(){s(e[28]("select",e[8]))&&e[28]("select",e[8]).apply(this,arguments)})),L(n,"mouseenter",(function(){s(e[28]("hover",e[10].last))&&e[28]("hover",e[10].last).apply(this,arguments)}))],o=!0)},p(t,l){e=t,16640&l[0]&&c!==(c=e[14](e[8])+"")&&i.p(c),e[26]!=e[0]?d?d.p(e,l):(d=ln(e),d.c(),d.m(n,null)):d&&(d.d(1),d=null),67108865&l[0]&&B(n,"active",e[26]==e[0]),320&l[0]&&B(n,"is-disabled",e[6].includes(e[8]))},d(e){e&&O(t),d&&d.d(),o=!1,r(a)}}}function ln(e){let t,n,i,l,r;return{c(){t=k("span"),n=k("kbd"),i=D(e[15]),l=D("+"),r=k("kbd"),r.textContent="Enter",H(n,"class","svelte-9227bl"),H(r,"class","svelte-9227bl"),H(t,"class","shortcut svelte-9227bl")},m(e,s){C(e,t,s),S(t,n),S(n,i),S(t,l),S(t,r)},p(e,t){32768&t[0]&&P(i,e[15])},d(e){e&&O(t)}}}function rn(e){let t,n,i=e[18]&&e[20]&&Wt(e);return{c(){i&&i.c(),t=M()},m(e,l){i&&i.m(e,l),C(e,t,l),n=!0},p(e,n){e[18]&&e[20]?i?(i.p(e,n),1310720&n[0]&&ye(i,1)):(i=Wt(e),i.c(),ye(i,1),i.m(t.parentNode,t)):i&&(be(),xe(i,1,1,(()=>{i=null})),$e())},i(e){n||(ye(i),n=!0)},o(e){xe(i),n=!1},d(e){i&&i.d(e),e&&O(t)}}}function sn(e,n,i){let l,r,s,o=t,c=()=>(o(),o=a(A,(e=>i(27,s=e))),A);e.$$.on_destroy.push((()=>o()));let{lazyDropdown:d}=n,{creatable:u}=n,{maxReached:h=!1}=n,{dropdownIndex:p=0}=n,{renderer:f}=n,{disableHighlight:m}=n,{items:g=[]}=n,{alreadyCreated:v}=n,{virtualList:b}=n,{vlItemSize:$}=n,{vlHeight:y}=n,{inputValue:x}=n,{multiple:w}=n,{listIndex:S}=n,{hasDropdownOpened:A}=n;c();let{listMessage:I}=n,{disabledField:z}=n,{createLabel:C}=n,{metaKey:O}=n,{itemComponent:F}=n,{selection:k=null}=n;function E(e){if(b)return;if(null===p&&M)return void M.scrollTo(0,0);const t=T?.querySelector(`[data-pos="${p}"]`);if(!t)return;const n=t.getBoundingClientRect(),l=M.getBoundingClientRect(),r=t.offsetHeight/3,s=e&&e.center?M.offsetHeight/2:0;switch(!0){case t.offsetTop<M.scrollTop:i(23,M.scrollTop=t.offsetTop-r+s,M);break;case t.offsetTop+n.height>M.scrollTop+l.height:i(23,M.scrollTop=t.offsetTop+n.height-M.offsetHeight+r+s,M)}}const D=te();let T,M,L,_=!1,H=!1,P=!d,V=y,R=$,N=null===y&&null===$;function B(e){if(!M&&!P)return;const t=function(e){if(!e)return!1;const t=e.parentElement.parentElement.getBoundingClientRect(),n=e.getBoundingClientRect(),i={};return i.top=t.top<0,i.left=t.left<0,i.bottom=t.bottom+n.height>(window.innerHeight||document.documentElement.clientHeight),i.right=t.right>(window.innerWidth||document.documentElement.clientWidth),i.any=i.top||i.left||i.bottom||i.right,i}(M);t.bottom&&!t.top?i(23,M.parentElement.style.bottom=M.parentElement.parentElement.clientHeight+1+"px",M):e&&!t.top||i(23,M.parentElement.style.bottom="",M)}function j(){if(!L)return;const e=(e,t)=>{const n=window.getComputedStyle(e);let{groups:{value:i,unit:l}}=n[t].match(/(?<value>\d+)(?<unit>[a-zA-Z]+)/);if(i=parseFloat(i),"px"!==l){const e="rem"===l?document.documentElement:M.parentElement.parentElement;i*=parseFloat(window.getComputedStyle(e).fontSize.match(/\d+/).shift())}return i};i(34,V=e(M,"maxHeight")-e(M,"paddingTop")-e(M,"paddingBottom")),i(23,M.parentElement.style="opacity: 0; display: block",M);const t=L.$$.ctx[1].firstElementChild.firstElementChild;if(t){t.style="";const e=t.getBoundingClientRect().height,n=L.$$.ctx[1].firstElementChild.firstElementChild.nextElementSibling;let l;if(n&&(n.style="",l=n.getBoundingClientRect().height),e!==l){const t=g[0].$isGroupHeader?e:l,n=g[0].$isGroupHeader?l:e;i(21,R=g.map((e=>e.$isGroupHeader?t:n)))}else i(21,R=e)}i(23,M.parentElement.style="",M)}let q=()=>{},G=null;return Y((()=>{q=A.subscribe((e=>{!P&&e&&i(20,P=!0),de().then((()=>{B(e),e&&E({center:!0})})),G||(G=()=>B(e)),document[e?"addEventListener":"removeEventListener"]("scroll",G,{passive:!0})})),i(18,_=!0)})),ee((()=>q())),e.$$set=e=>{"lazyDropdown"in e&&i(29,d=e.lazyDropdown),"creatable"in e&&i(1,u=e.creatable),"maxReached"in e&&i(2,h=e.maxReached),"dropdownIndex"in e&&i(0,p=e.dropdownIndex),"renderer"in e&&i(3,f=e.renderer),"disableHighlight"in e&&i(4,m=e.disableHighlight),"items"in e&&i(5,g=e.items),"alreadyCreated"in e&&i(6,v=e.alreadyCreated),"virtualList"in e&&i(7,b=e.virtualList),"vlItemSize"in e&&i(30,$=e.vlItemSize),"vlHeight"in e&&i(31,y=e.vlHeight),"inputValue"in e&&i(8,x=e.inputValue),"multiple"in e&&i(9,w=e.multiple),"listIndex"in e&&i(10,S=e.listIndex),"hasDropdownOpened"in e&&c(i(11,A=e.hasDropdownOpened)),"listMessage"in e&&i(12,I=e.listMessage),"disabledField"in e&&i(13,z=e.disabledField),"createLabel"in e&&i(14,C=e.createLabel),"metaKey"in e&&i(15,O=e.metaKey),"itemComponent"in e&&i(16,F=e.itemComponent),"selection"in e&&i(17,k=e.selection)},e.$$.update=()=>{32&e.$$.dirty[0]&&i(26,l=g.length),1835426&e.$$.dirty[0]&&(i(19,H=g.length<1&&(!u||!x)),b&&N&&_&&P&&(H&&i(0,p=null),i(21,R=0),de().then(j).then(B))),2097184&e.$$.dirty[0]|8&e.$$.dirty[1]&&i(25,r=Math.min(V,Array.isArray(R)?R.reduce(((e,t)=>e+=t),0):g.length*R))},[p,u,h,f,m,g,v,b,x,w,S,A,I,z,C,O,F,k,_,H,P,R,T,M,L,r,l,s,D,d,$,y,E,function(){return b?[M.offsetHeight,R]:[M.offsetHeight,T.firstElementChild.offsetHeight]},V,function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(e){le[e?"unshift":"push"]((()=>{L=e,i(24,L)}))},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(e){le[e?"unshift":"push"]((()=>{T=e,i(22,T)}))},function(e){le[e?"unshift":"push"]((()=>{M=e,i(23,M)}))}]}class on extends Fe{constructor(e){super(),Oe(this,e,sn,rn,o,{lazyDropdown:29,creatable:1,maxReached:2,dropdownIndex:0,renderer:3,disableHighlight:4,items:5,alreadyCreated:6,virtualList:7,vlItemSize:30,vlHeight:31,inputValue:8,multiple:9,listIndex:10,hasDropdownOpened:11,listMessage:12,disabledField:13,createLabel:14,metaKey:15,itemComponent:16,selection:17,scrollIntoView:32,getDimensions:33},qt,[-1,-1])}get scrollIntoView(){return this.$$.ctx[32]}get getDimensions(){return this.$$.ctx[33]}}function an(e){A(e,"svelte-w7c5vi",".sv-item-btn.svelte-w7c5vi.svelte-w7c5vi{position:relative;display:inline-flex;align-items:center;align-self:stretch;padding:0 4px;box-sizing:border-box;border-radius:2px;border-width:0;margin:0;cursor:pointer;background-color:var(--sv-item-btn-bg, var(--sv-item-selected-bg))}.sv-item-btn.svelte-w7c5vi.svelte-w7c5vi:hover{background-color:var(--sv-item-btn-bg-hover)}.sv-item-btn.svelte-w7c5vi>svg.svelte-w7c5vi{fill:var(--sv-item-btn-icon, var(--sv-icon-color))}")}function cn(e){let n;return{c(){n=k("button"),n.innerHTML='<svg height="16" width="16" viewBox="0 0 20 20" aria-hidden="true" focusable="false" class="svelte-w7c5vi"><path d="M14.348 14.849c-0.469 0.469-1.229 0.469-1.697 0l-2.651-3.030-2.651 3.029c-0.469 0.469-1.229 0.469-1.697 0-0.469-0.469-0.469-1.229 0-1.697l2.758-3.15-2.759-3.152c-0.469-0.469-0.469-1.228 0-1.697s1.228-0.469 1.697 0l2.652 3.031 2.651-3.031c0.469-0.469 1.228-0.469 1.697 0s0.469 1.229 0 1.697l-2.758 3.152 2.758 3.15c0.469 0.469 0.469 1.229 0 1.698z"></path></svg>',H(n,"class","sv-item-btn svelte-w7c5vi"),H(n,"tabindex","-1"),H(n,"data-action","deselect"),H(n,"type","button")},m(e,t){C(e,n,t)},p:t,i:t,o:t,d(e){e&&O(n)}}}class dn extends Fe{constructor(e){super(),Oe(this,e,null,cn,o,{},an)}}const un=e=>e.preventDefault();function hn(e,{item:t,index:n}){function i(n){const i=n.target.closest('[data-action="deselect"]')?"deselect":"select";e.dispatchEvent(new CustomEvent(i,{bubble:!0,detail:t}))}function l(){e.dispatchEvent(new CustomEvent("hover",{detail:n}))}return e.onmousedown=un,e.onclick=i,e.addEventListener("mouseenter",l),{update(e){t=e.item,n=e.index},destroy(){e.removeEventListener("mousedown",un),e.removeEventListener("click",i),e.removeEventListener("mouseenter",l)}}}function pn(e){A(e,"svelte-1e087o6",".optgroup-header.svelte-1e087o6{padding:3px 3px 3px 6px;font-weight:bold}")}function fn(e){let t,n,i,l,o,a,c,d,u=(e[3]?`<div class="sv-item-content">${e[6](e[2],e[3],e[0])}</div>`:Re(e[2],e[3],e[0],e[6],e[7]))+"",h=e[3]&&e[5]&&gn();return{c(){t=k("div"),n=new j,i=T(),h&&h.c(),n.a=i,H(t,"class","sv-item"),H(t,"title",l=e[2].$created?"Created item":""),B(t,"is-disabled",e[4])},m(l,r){C(l,t,r),n.m(u,t),S(t,i),h&&h.m(t,null),a=!0,c||(d=[v(o=hn.call(null,t,{item:e[2],index:e[1]})),L(t,"select",e[9]),L(t,"deselect",e[10]),L(t,"hover",e[11])],c=!0)},p(e,i){(!a||205&i)&&u!==(u=(e[3]?`<div class="sv-item-content">${e[6](e[2],e[3],e[0])}</div>`:Re(e[2],e[3],e[0],e[6],e[7]))+"")&&n.p(u),e[3]&&e[5]?h?40&i&&ye(h,1):(h=gn(),h.c(),ye(h,1),h.m(t,null)):h&&(be(),xe(h,1,1,(()=>{h=null})),$e()),(!a||4&i&&l!==(l=e[2].$created?"Created item":""))&&H(t,"title",l),o&&s(o.update)&&6&i&&o.update.call(null,{item:e[2],index:e[1]}),16&i&&B(t,"is-disabled",e[4])},i(e){a||(ye(h),a=!0)},o(e){xe(h),a=!1},d(e){e&&O(t),h&&h.d(),c=!1,r(d)}}}function mn(e){let n,i,l,r,s,o=e[2].label+"";return{c(){n=k("div"),i=k("b"),l=D(o),H(n,"class","optgroup-header svelte-1e087o6")},m(t,o){C(t,n,o),S(n,i),S(i,l),r||(s=L(n,"mousedown",_(e[8])),r=!0)},p(e,t){4&t&&o!==(o=e[2].label+"")&&P(l,o)},i:t,o:t,d(e){e&&O(n),r=!1,s()}}}function gn(e){let t,n;return t=new dn({}),{c(){Ie(t.$$.fragment)},m(e,i){ze(t,e,i),n=!0},i(e){n||(ye(t.$$.fragment,e),n=!0)},o(e){xe(t.$$.fragment,e),n=!1},d(e){Ce(t,e)}}}function vn(e){let t,n,i,l;const r=[mn,fn],s=[];function o(e,t){return e[2].$isGroupHeader?0:1}return t=o(e),n=s[t]=r[t](e),{c(){n.c(),i=M()},m(e,n){s[t].m(e,n),C(e,i,n),l=!0},p(e,[l]){let a=t;t=o(e),t===a?s[t].p(e,l):(be(),xe(s[a],1,1,(()=>{s[a]=null})),$e(),n=s[t],n?n.p(e,l):(n=s[t]=r[t](e),n.c()),ye(n,1),n.m(i.parentNode,i))},i(e){l||(ye(n),l=!0)},o(e){xe(n),l=!1},d(e){s[t].d(e),e&&O(i)}}}function bn(e,t,n){let{inputValue:i}=t,{index:l=-1}=t,{item:r={}}=t,{isSelected:s=!1}=t,{isDisabled:o=!1}=t,{isMultiple:a=!1}=t,{formatter:c=null}=t,{disableHighlight:d=!1}=t;return e.$$set=e=>{"inputValue"in e&&n(0,i=e.inputValue),"index"in e&&n(1,l=e.index),"item"in e&&n(2,r=e.item),"isSelected"in e&&n(3,s=e.isSelected),"isDisabled"in e&&n(4,o=e.isDisabled),"isMultiple"in e&&n(5,a=e.isMultiple),"formatter"in e&&n(6,c=e.formatter),"disableHighlight"in e&&n(7,d=e.disableHighlight)},[i,l,r,s,o,a,c,d,function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)},function(t){ne.call(this,e,t)}]}class $n extends Fe{constructor(e){super(),Oe(this,e,bn,vn,o,{inputValue:0,index:1,item:2,isSelected:3,isDisabled:4,isMultiple:5,formatter:6,disableHighlight:7},pn)}}function yn(e){A(e,"svelte-17904zl",".svelecte-control.svelte-17904zl{--sv-bg:#fff;--sv-color:inherit;--sv-min-height:38px;--sv-border-color:#ccc;--sv-border:1px solid var(--sv-border-color);--sv-active-border:1px solid #555;--sv-active-outline:none;--sv-disabled-bg:#f2f2f2;--sv-disabled-border-color:#e6e6e6;--sv-placeholder-color:#ccccc6;--sv-icon-color:#ccc;--sv-icon-hover:#999;--sv-loader-border:3px solid #dbdbdb;--sv-dropdown-shadow:0 6px 12px rgba(0,0,0,0.175);--sv-dropdown-height:250px;--sv-item-selected-bg:#efefef;--sv-item-color:#333333;--sv-item-active-color:var(--sv-item-color);--sv-item-active-bg:#F2F5F8;--sv-item-btn-bg:var(--sv-item-selected-bg);--sv-item-btn-bg-hover:#ddd;--sv-item-btn-icon:var(--sv-item-color);--sv-highlight-bg:yellow;--sv-highlight-color:var(--sv-item-color)}.svelecte.svelte-17904zl{position:relative;flex:1 1 auto;color:var(--sv-color)}.svelecte.is-disabled.svelte-17904zl{pointer-events:none}.icon-slot.svelte-17904zl{display:flex}.sv-hidden-element.svelte-17904zl{opacity:0;position:absolute;z-index:-2;top:0;height:var(--sv-min-height)}.svelecte-control .has-multiSelection .sv-item,#dnd-action-dragged-el .sv-item{background-color:var(--sv-item-selected-bg);margin:2px 4px 2px 0}.svelecte-control .has-multiSelection .sv-item-content,.svelecte-control .sv-dropdown-content .sv-item,#dnd-action-dragged-el .sv-item-content{padding:3px 3px 3px 6px}.svelecte-control .sv-item,#dnd-action-dragged-el .sv-item{display:flex;min-width:0px;box-sizing:border-box;border-radius:2px;cursor:default}.svelecte-control .sv-item.is-disabled{opacity:0.5;cursor:not-allowed}.svelecte-control .sv-item-content,#dnd-action-dragged-el .sv-item-content{color:var(--sv-item-color, var(--sv-color));text-overflow:ellipsis;white-space:nowrap;box-sizing:border-box;border-radius:2px;overflow:hidden;width:100%}.svelecte-control .sv-dd-item-active > .sv-item{background-color:var(--sv-item-active-bg)}.svelecte-control .sv-dd-item-active > .sv-item .sv-item-content{color:var(--sv-item-active-color, var(--sv-item-color))}.svelecte-control .highlight{background-color:var(--sv-highlight-bg);color:var(--sv-highlight-color, var(--sv-color))}.indicator-icon.svelte-17904zl{display:inline-block;fill:currentcolor;line-height:1;stroke:currentcolor;stroke-width:0px}")}function xn(e,t,n){const i=e.slice();return i[120]=t[n],i}const wn=e=>({}),Sn=e=>({}),An=e=>({}),In=e=>({}),zn=e=>({hasDropdownOpened:8388608&e[1]}),Cn=e=>({slot:"indicator-icon",hasDropdownOpened:e[54]}),On=e=>({selectedOptions:1&e[1],inputValue:16&e[1]}),Fn=e=>({slot:"clear-icon",selectedOptions:e[31],inputValue:e[35]});function kn(e){let t,n;const i=e[96].icon,l=d(i,e,e[101],Sn);return{c(){t=k("div"),l&&l.c(),H(t,"slot","icon"),H(t,"class","icon-slot svelte-17904zl")},m(e,i){C(e,t,i),l&&l.m(t,null),n=!0},p(e,t){l&&l.p&&(!n||256&t[3])&&p(l,i,e,e[101],n?h(i,e[101],t,wn):f(e[101]),Sn)},i(e){n||(ye(l,e),n=!0)},o(e){xe(l,e),n=!1},d(e){e&&O(t),l&&l.d(e)}}}function En(e){let t,n;const i=e[96]["control-end"],l=d(i,e,e[101],In);return{c(){t=k("div"),l&&l.c(),H(t,"slot","control-end")},m(e,i){C(e,t,i),l&&l.m(t,null),n=!0},p(e,t){l&&l.p&&(!n||256&t[3])&&p(l,i,e,e[101],n?h(i,e[101],t,An):f(e[101]),In)},i(e){n||(ye(l,e),n=!0)},o(e){xe(l,e),n=!1},d(e){e&&O(t),l&&l.d(e)}}}function Dn(e){let t;const n=e[96]["indicator-icon"],i=d(n,e,e[101],Cn),l=i||function(e){let t,n;return{c(){t=E("svg"),n=E("path"),H(n,"d","M4.516 7.548c0.436-0.446 1.043-0.481 1.576 0l3.908 3.747 3.908-3.747c0.533-0.481 1.141-0.446 1.574 0 0.436 0.445 0.408 1.197 0 1.615-0.406 0.418-4.695 4.502-4.695 4.502-0.217 0.223-0.502 0.335-0.787 0.335s-0.57-0.112-0.789-0.335c0 0-4.287-4.084-4.695-4.502s-0.436-1.17 0-1.615z"),H(t,"width","20"),H(t,"height","20"),H(t,"class","indicator-icon svelte-17904zl"),H(t,"viewBox","0 0 20 20"),H(t,"aria-hidden","true"),H(t,"focusable","false")},m(e,i){C(e,t,i),S(t,n)},d(e){e&&O(t)}}}();return{c(){l&&l.c()},m(e,n){l&&l.m(e,n),t=!0},p(e,l){i&&i.p&&(!t||8388608&l[1]|256&l[3])&&p(i,n,e,e[101],t?h(n,e[101],l,zn):f(e[101]),Cn)},i(e){t||(ye(l,e),t=!0)},o(e){xe(l,e),t=!1},d(e){l&&l.d(e)}}}function Tn(e){let t,n;return{c(){t=E("svg"),n=E("path"),H(n,"d","M14.348 14.849c-0.469 0.469-1.229 0.469-1.697 0l-2.651-3.030-2.651 3.029c-0.469 0.469-1.229 0.469-1.697 0-0.469-0.469-0.469-1.229 0-1.697l2.758-3.15-2.759-3.152c-0.469-0.469-0.469-1.228 0-1.697s1.228-0.469 1.697 0l2.652 3.031 2.651-3.031c0.469-0.469 1.228-0.469 1.697 0s0.469 1.229 0 1.697l-2.758 3.152 2.758 3.15c0.469 0.469 0.469 1.229 0 1.698z"),H(t,"class","indicator-icon svelte-17904zl"),H(t,"height","20"),H(t,"width","20"),H(t,"viewBox","0 0 20 20"),H(t,"aria-hidden","true"),H(t,"focusable","false")},m(e,i){C(e,t,i),S(t,n)},d(e){e&&O(t)}}}function Mn(e){let t;const n=e[96]["clear-icon"],i=d(n,e,e[101],Fn),l=i||function(e){let t,n=e[31].length&&Tn();return{c(){n&&n.c(),t=M()},m(e,i){n&&n.m(e,i),C(e,t,i)},p(e,i){e[31].length?n||(n=Tn(),n.c(),n.m(t.parentNode,t)):n&&(n.d(1),n=null)},d(e){n&&n.d(e),e&&O(t)}}}(e);return{c(){l&&l.c()},m(e,n){l&&l.m(e,n),t=!0},p(e,r){i?i.p&&(!t||17&r[1]|256&r[3])&&p(i,n,e,e[101],t?h(n,e[101],r,On):f(e[101]),Fn):l&&l.p&&(!t||1&r[1])&&l.p(e,t?r:[-1,-1,-1,-1])},i(e){t||(ye(l,e),t=!0)},o(e){xe(l,e),t=!1},d(e){l&&l.d(e)}}}function Ln(e){let t,n,i,l,r=e[31],s=[];for(let t=0;t<r.length;t+=1)s[t]=_n(xn(e,r,t));return{c(){t=k("select");for(let e=0;e<s.length;e+=1)s[e].c();H(t,"id",e[43]),H(t,"name",e[2]),t.multiple=e[1],H(t,"class","sv-hidden-element svelte-17904zl"),H(t,"tabindex","-1"),t.required=e[4],t.disabled=e[0]},m(r,o){C(r,t,o);for(let e=0;e<s.length;e+=1)s[e].m(t,null);i||(l=v(n=e[44].call(null,t,e[45])),i=!0)},p(e,n){if(419430400&n[0]|1&n[1]){let i;for(r=e[31],i=0;i<r.length;i+=1){const l=xn(e,r,i);s[i]?s[i].p(l,n):(s[i]=_n(l),s[i].c(),s[i].m(t,null))}for(;i<s.length;i+=1)s[i].d(1);s.length=r.length}4&n[0]&&H(t,"name",e[2]),2&n[0]&&(t.multiple=e[1]),16&n[0]&&(t.required=e[4]),1&n[0]&&(t.disabled=e[0])},d(e){e&&O(t),F(s,e),i=!1,l()}}}function _n(e){let t,n,i,l=e[120][e[28]]+"";return{c(){t=k("option"),n=D(l),t.__value=i=e[120][e[24]?e[28]:e[27]],t.value=t.__value,t.selected=!0},m(e,i){C(e,t,i),S(t,n)},p(e,r){268435456&r[0]|1&r[1]&&l!==(l=e[120][e[28]]+"")&&P(n,l),419430400&r[0]|1&r[1]&&i!==(i=e[120][e[24]?e[28]:e[27]])&&(t.__value=i,t.value=t.__value)},d(e){e&&O(t)}}}function Hn(e){let t,n,i,l,r,s,o,a={renderer:e[41],disabled:e[0],clearable:e[9],searchable:e[8],placeholder:e[7],multiple:e[1],inputId:e[3]||e[43],resetOnBlur:e[11],collapseSelection:e[15]?Vn.collapseSelectionFn.bind(e[30]):null,inputValue:e[46],hasFocus:e[47],hasDropdownOpened:e[54],selectedOptions:e[31],isFetchingData:e[29],dndzone:e[12],currentValueField:e[27],isAndroid:e[38],isIOS:e[37],alwaysCollapsed:e[16],itemComponent:e[14],$$slots:{"clear-icon":[Mn],"indicator-icon":[Dn,({hasDropdownOpened:e})=>({54:e}),({hasDropdownOpened:e})=>[0,e?8388608:0]],"control-end":[En],icon:[kn]},$$scope:{ctx:e}};n=new wt({props:a}),e[97](n),n.$on("deselect",e[49]),n.$on("keydown",e[51]),n.$on("paste",e[52]),n.$on("consider",e[53]),n.$on("finalize",e[53]),n.$on("blur",e[98]);let c={renderer:e[41],disableHighlight:e[10],creatable:e[17],maxReached:e[34],alreadyCreated:e[39],virtualList:e[19],vlHeight:e[20],vlItemSize:e[21],lazyDropdown:e[19]||e[18],multiple:e[1],dropdownIndex:e[26],items:e[33],listIndex:e[32],inputValue:e[40],hasDropdownOpened:e[54],listMessage:e[42],disabledField:e[6],createLabel:e[30].createRowLabel,metaKey:e[37]?"⌘":"Ctrl",selection:e[15]&&e[16]?e[31]:null,itemComponent:e[13]};l=new on({props:c}),e[99](l),l.$on("select",e[48]),l.$on("deselect",e[49]),l.$on("hover",e[50]),l.$on("createoption",e[100]);let d=e[2]&&!e[5]&&Ln(e);return{c(){t=k("div"),Ie(n.$$.fragment),i=T(),Ie(l.$$.fragment),r=T(),d&&d.c(),H(t,"class",s=m(`svelecte ${e[22]}`)+" svelte-17904zl"),H(t,"style",e[23]),B(t,"is-disabled",e[0])},m(e,s){C(e,t,s),ze(n,t,null),S(t,i),ze(l,t,null),S(t,r),d&&d.m(t,null),o=!0},p(e,i){const r={};1024&i[1]&&(r.renderer=e[41]),1&i[0]&&(r.disabled=e[0]),512&i[0]&&(r.clearable=e[9]),256&i[0]&&(r.searchable=e[8]),128&i[0]&&(r.placeholder=e[7]),2&i[0]&&(r.multiple=e[1]),8&i[0]&&(r.inputId=e[3]||e[43]),2048&i[0]&&(r.resetOnBlur=e[11]),1073774592&i[0]&&(r.collapseSelection=e[15]?Vn.collapseSelectionFn.bind(e[30]):null),1&i[1]&&(r.selectedOptions=e[31]),536870912&i[0]&&(r.isFetchingData=e[29]),4096&i[0]&&(r.dndzone=e[12]),134217728&i[0]&&(r.currentValueField=e[27]),128&i[1]&&(r.isAndroid=e[38]),64&i[1]&&(r.isIOS=e[37]),65536&i[0]&&(r.alwaysCollapsed=e[16]),16384&i[0]&&(r.itemComponent=e[14]),8388625&i[1]|256&i[3]&&(r.$$scope={dirty:i,ctx:e}),n.$set(r);const a={};1024&i[1]&&(a.renderer=e[41]),1024&i[0]&&(a.disableHighlight=e[10]),131072&i[0]&&(a.creatable=e[17]),8&i[1]&&(a.maxReached=e[34]),256&i[1]&&(a.alreadyCreated=e[39]),524288&i[0]&&(a.virtualList=e[19]),1048576&i[0]&&(a.vlHeight=e[20]),2097152&i[0]&&(a.vlItemSize=e[21]),786432&i[0]&&(a.lazyDropdown=e[19]||e[18]),2&i[0]&&(a.multiple=e[1]),67108864&i[0]&&(a.dropdownIndex=e[26]),4&i[1]&&(a.items=e[33]),2&i[1]&&(a.listIndex=e[32]),512&i[1]&&(a.inputValue=e[40]),2048&i[1]&&(a.listMessage=e[42]),64&i[0]&&(a.disabledField=e[6]),1073741824&i[0]&&(a.createLabel=e[30].createRowLabel),64&i[1]&&(a.metaKey=e[37]?"⌘":"Ctrl"),98304&i[0]|1&i[1]&&(a.selection=e[15]&&e[16]?e[31]:null),8192&i[0]&&(a.itemComponent=e[13]),l.$set(a),e[2]&&!e[5]?d?d.p(e,i):(d=Ln(e),d.c(),d.m(t,null)):d&&(d.d(1),d=null),(!o||4194304&i[0]&&s!==(s=m(`svelecte ${e[22]}`)+" svelte-17904zl"))&&H(t,"class",s),(!o||8388608&i[0])&&H(t,"style",e[23]),4194305&i[0]&&B(t,"is-disabled",e[0])},i(e){o||(ye(n.$$.fragment,e),ye(l.$$.fragment,e),o=!0)},o(e){xe(n.$$.fragment,e),xe(l.$$.fragment,e),o=!1},d(i){i&&O(t),e[97](null),Ce(n),e[99](null),Ce(l),d&&d.d()}}}const Pn={default(e){return`${e[this.label]}`.replace(/</g,"<").replace(/>/g,">").replace(/&/g,"&").replace(/"/g,""").replace(/'/g,"'")}};const Vn=Ge;function Rn(e,t,n){let i,l,r,s,o,a,d,u,h,p,f,{$$slots:m={},$$scope:v}=t,{name:b="svelecte"}=t,{inputId:$=null}=t,{required:y=!1}=t,{hasAnchor:x=!1}=t,{disabled:w=Ge.disabled}=t,{options:S=[]}=t,{valueField:A=Ge.valueField}=t,{labelField:I=Ge.labelField}=t,{groupLabelField:z=Ge.groupLabelField}=t,{groupItemsField:C=Ge.groupItemsField}=t,{disabledField:O=Ge.disabledField}=t,{placeholder:F="Select"}=t,{searchable:k=Ge.searchable}=t,{clearable:E=Ge.clearable}=t,{renderer:D=null}=t,{disableHighlight:T=!1}=t,{highlightFirstItem:M=Ge.highlightFirstItem}=t,{selectOnTab:L=Ge.selectOnTab}=t,{resetOnBlur:_=Ge.resetOnBlur}=t,{resetOnSelect:H=Ge.resetOnSelect}=t,{closeAfterSelect:P=Ge.closeAfterSelect}=t,{dndzone:V=(()=>({noop:!0,destroy:()=>{}}))}=t,{validatorAction:R=null}=t,{dropdownItem:N=$n}=t,{controlItem:B=$n}=t,{multiple:j=Ge.multiple}=t,{max:q=Ge.max}=t,{collapseSelection:G=Ge.collapseSelection}=t,{alwaysCollapsed:K=Ge.alwaysCollapsed}=t,{creatable:W=Ge.creatable}=t,{creatablePrefix:Q=Ge.creatablePrefix}=t,{allowEditing:U=Ge.allowEditing}=t,{keepCreated:J=Ge.keepCreated}=t,{delimiter:X=Ge.delimiter}=t,{createFilter:Z=null}=t,{createTransform:ee=null}=t,{fetch:ie=null}=t,{fetchMode:re="auto"}=t,{fetchCallback:se=Ge.fetchCallback}=t,{fetchResetOnBlur:oe=!0}=t,{fetchDebounceTime:ae=500}=t,{minQuery:ce=Ge.minQuery}=t,{lazyDropdown:ue=Ge.lazyDropdown}=t,{virtualList:he=Ge.virtualList}=t,{vlHeight:pe=Ge.vlHeight}=t,{vlItemSize:fe=Ge.vlItemSize}=t,{searchField:me=null}=t,{sortField:ge=null}=t,{disableSifter:ve=!1}=t,{class:be="svelecte-control"}=t,{style:$e=null}=t,{i18n:ye=null}=t,{readSelection:xe=null}=t,{value:we=null}=t,{labelAsValue:Se=!1}=t,{valueAsObject:Ae=Ge.valueAsObject}=t;const Ie=`sv-select-${Math.random()}`.replace(".",""),ze=te(),Ce={optionsWithGroups:!1,isOptionArray:S&&S.length&&"object"!=typeof S[0],optionProps:[],valueField:A,labelField:I,labelAsValue:Se,optLabel:z,optItems:C};ie&&we&&Ae&&(!S||S&&0===S.length)&&(S=Array.isArray(we)?we:[we]);let Oe,Fe,Ee=!1,De=!1,Te=null,Me=A||Be("value",S,Ce),Le=I||Be("label",S,Ce),_e=!1,He=!1,Pe=R?R.shift():()=>({destroy:()=>{}}),Re=R||[],Ne=null;Ce.valueField=Me,Ce.labelField=Le,Ce.optionProps=we&&Ae&&(!j||!Array.isArray(we)||we.length>0)?Je(j?we.slice(0,1).shift():we):[Me,Le],j=b&&!j?b.endsWith("[]"):j,Z||(Z=je);const Ke=We("");c(e,Ke,(e=>n(35,h=e)));const Xe=We(!1);c(e,Xe,(e=>n(108,f=e)));const Ze=We(!1);c(e,Ze,(e=>n(95,p=e)));let Ye=!1,et="init"===re||"auto"===re&&"string"==typeof ie&&-1===ie.indexOf("[query]"),tt=et?we:null,nt=null;function it(){return Ee&&Ye&&(Ve&&![0,4].includes(Ve.readyState)&&Ve.abort(),n(29,Ye=!1)),!0}function lt(e){if(nt&&(nt(),nt=null),!e)return null;it(),et&&rt&&(tt=rt);const t="string"==typeof e?(i=e,function(e,t){return new Promise(((n,l)=>{Ve=new XMLHttpRequest,Ve.open("GET",`${i.replace("[query]",encodeURIComponent(e))}`),Ve.setRequestHeader("X-Requested-With","XMLHttpRequest"),Ve.send(),Ve.onreadystatechange=function(){if(4===this.readyState)if(200===this.status)try{const e=JSON.parse(this.response);n(t?t(e):e.data||e.items||e.options||e)}catch(e){console.warn("[Svelecte]:Fetch - error handling fetch response",e),l()}else l()}}))}):e;var i;n(92,et="init"===re||"auto"===re&&"string"==typeof e&&-1===e.indexOf("[query]"));const l=function(e,t){let n;return function(){const i=this,l=arguments;clearTimeout(n),n=setTimeout((function(){e.apply(i,l)}),t)}}((e=>{!e||h.length?t(e,se).then((e=>{Array.isArray(e)||(console.warn("[Svelecte]:Fetch - array expected, invalid property provided:",e),e=[]),n(55,S=e)})).catch((()=>{n(55,S=[])})).finally((()=>{n(29,Ye=!1),f&&Ze.set(!0),n(42,a=st.fetchEmpty),de().then((()=>{et&&tt&&(ht(tt),tt=null),ze("fetch",S)}))})):n(29,Ye=!1)}),ae);return et?("string"==typeof e&&-1!==e.indexOf("[parent]")||(n(29,Ye=!0),n(55,S=[]),l(null)),null):(nt=Ke.subscribe((e=>{it(),e?e&&e.length<ce||(!et&&Ze.set(!1),n(29,Ye=!0),l(e)):Ee&&oe&&n(55,S=[])})),l)}let rt=we,st=Vn.i18n,ot=null!==we?Qe.call(S,we,Ae,Ce):[],at=ot.reduce(((e,t)=>(e.add(t[Me]),e)),new Set),ct=[""],dt=S;function ut(){de().then((()=>{ze("change",xe),Ne&&(Ne.dispatchEvent(new Event("input")),Ne.dispatchEvent(new Event("change")))}))}function ht(e){if(ft(),e){let t=Array.isArray(e)?e:[e];const s=Ce.labelAsValue?Le:Me;if(t=t.reduce(((e,t)=>{if(W&&Ae&&t.$created)return e.push(t),e;const n=i.find((e=>Ae?e[s]==t[s]:e[s]==t));return n&&e.push(n),e}),[]),!(t.every(pt)&&(j?e.length===t.length:t.length>0)))return console.warn('[Svelecte]: provided "value" property is invalid',e),n(59,we=j?[]:null),n(60,xe=we),void ze("invalidValue",e);if(n(60,xe=Array.isArray(e)?t:t.shift()),!p&&he){let e=Ze.subscribe((t=>{t&&(n(26,Te),n(66,M),n(35,h),n(32,o),n(1,j),n(95,p),n(31,ot),n(94,i),n(27,Me),n(25,Oe),n(33,r),n(17,W),n(90,Ce),n(55,S),n(91,Ee),n(113,dt),n(61,A),n(62,I),n(28,Le),n(34,l),n(83,ve),n(112,at),n(81,me),n(82,ge),n(24,Se),n(71,q),e&&e())}))}}n(93,rt=e)}function pt(e){if(!e||j&&l)return!1;if(!at.has(e[Me])){if("string"==typeof e){if(!W)return;if(e=Z(e,S),ct.includes(e))return;!ie&&ct.push(e),(e=ee(e,Q,Me,Le)).$created=!0,J&&n(55,S=[...S,e]),ze("createoption",e)}return j?(ot.push(e),n(31,ot),at.add(e[Me])):(n(31,ot=[e]),at.clear(),at.add(e[Me]),n(26,Te=S.indexOf(e))),n(94,i),n(55,S),n(90,Ce),n(91,Ee),n(113,dt),n(61,A),n(27,Me),n(62,I),n(28,Le),n(24,Se),!0}}function ft(){at.clear(),n(31,ot=[]),n(34,l=!1),n(94,i),n(55,S),n(90,Ce),n(91,Ee),n(113,dt),n(61,A),n(27,Me),n(62,I),n(28,Le),n(24,Se)}function mt(e,t){t=t||e.detail,w||t[O]||t.$isGroupHeader||(pt(t),(j&&H||!j)&&g(Ke,h="",h),!j||P?g(Ze,p=!1,p):de().then((()=>{n(26,Te=l?null:o.next(Te-1,!0))})),ut())}function gt(e,t){w||((t=t||e.detail)?function(e){e.$created&&vt&&U&&(ct.splice(ct.findIndex((t=>t===e[Se?Le:Me])),1),n(39,ct),J&&(S.splice(S.findIndex((t=>t===e)),1),n(55,S)),g(Ke,h=e[Le].replace(Q,""),h));const t=e[Me];at.delete(t),ot.splice(ot.findIndex((e=>e[Me]==t)),1),n(31,ot),n(94,i),n(55,S),n(90,Ce),n(91,Ee),n(113,dt),n(61,A),n(27,Me),n(62,I),n(28,Le),n(24,Se)}(t):ft(),de().then(Fe.focusControl),ut(),(j&&0===ot.length||!j)&&(n(26,Te=M?0:null),de().then(Oe.scrollIntoView)))}let vt=!1;return Y((()=>{if(n(91,Ee=!0),W){const e=Ce.labelAsValue?Le:Me;n(39,ct=[""].concat(i.map((t=>t[e])).filter((e=>e))))}if(rt&&!j){const e=Se?Le:Me,t=Ae?rt[e]:rt;n(26,Te=i.findIndex((n=>n[e]===t)))}n(37,_e=["iPad Simulator","iPhone Simulator","iPod Simulator","iPad","iPhone","iPod"].includes(navigator.platform)||navigator.userAgent.includes("Mac")&&"ontouchend"in document||(navigator?.userAgentData?.platform||navigator?.platform||"").toLowerCase().includes("mac")),n(38,He=navigator.userAgent.toLowerCase().includes("android")),b&&!x&&(Ne=document.getElementById(Ie))})),e.$$set=e=>{"name"in e&&n(2,b=e.name),"inputId"in e&&n(3,$=e.inputId),"required"in e&&n(4,y=e.required),"hasAnchor"in e&&n(5,x=e.hasAnchor),"disabled"in e&&n(0,w=e.disabled),"options"in e&&n(55,S=e.options),"valueField"in e&&n(61,A=e.valueField),"labelField"in e&&n(62,I=e.labelField),"groupLabelField"in e&&n(63,z=e.groupLabelField),"groupItemsField"in e&&n(64,C=e.groupItemsField),"disabledField"in e&&n(6,O=e.disabledField),"placeholder"in e&&n(7,F=e.placeholder),"searchable"in e&&n(8,k=e.searchable),"clearable"in e&&n(9,E=e.clearable),"renderer"in e&&n(65,D=e.renderer),"disableHighlight"in e&&n(10,T=e.disableHighlight),"highlightFirstItem"in e&&n(66,M=e.highlightFirstItem),"selectOnTab"in e&&n(67,L=e.selectOnTab),"resetOnBlur"in e&&n(11,_=e.resetOnBlur),"resetOnSelect"in e&&n(68,H=e.resetOnSelect),"closeAfterSelect"in e&&n(69,P=e.closeAfterSelect),"dndzone"in e&&n(12,V=e.dndzone),"validatorAction"in e&&n(70,R=e.validatorAction),"dropdownItem"in e&&n(13,N=e.dropdownItem),"controlItem"in e&&n(14,B=e.controlItem),"multiple"in e&&n(1,j=e.multiple),"max"in e&&n(71,q=e.max),"collapseSelection"in e&&n(15,G=e.collapseSelection),"alwaysCollapsed"in e&&n(16,K=e.alwaysCollapsed),"creatable"in e&&n(17,W=e.creatable),"creatablePrefix"in e&&n(72,Q=e.creatablePrefix),"allowEditing"in e&&n(73,U=e.allowEditing),"keepCreated"in e&&n(74,J=e.keepCreated),"delimiter"in e&&n(75,X=e.delimiter),"createFilter"in e&&n(56,Z=e.createFilter),"createTransform"in e&&n(57,ee=e.createTransform),"fetch"in e&&n(58,ie=e.fetch),"fetchMode"in e&&n(76,re=e.fetchMode),"fetchCallback"in e&&n(77,se=e.fetchCallback),"fetchResetOnBlur"in e&&n(78,oe=e.fetchResetOnBlur),"fetchDebounceTime"in e&&n(79,ae=e.fetchDebounceTime),"minQuery"in e&&n(80,ce=e.minQuery),"lazyDropdown"in e&&n(18,ue=e.lazyDropdown),"virtualList"in e&&n(19,he=e.virtualList),"vlHeight"in e&&n(20,pe=e.vlHeight),"vlItemSize"in e&&n(21,fe=e.vlItemSize),"searchField"in e&&n(81,me=e.searchField),"sortField"in e&&n(82,ge=e.sortField),"disableSifter"in e&&n(83,ve=e.disableSifter),"class"in e&&n(22,be=e.class),"style"in e&&n(23,$e=e.style),"i18n"in e&&n(84,ye=e.i18n),"readSelection"in e&&n(60,xe=e.readSelection),"value"in e&&n(59,we=e.value),"labelAsValue"in e&&n(24,Se=e.labelAsValue),"valueAsObject"in e&&n(85,Ae=e.valueAsObject),"$$scope"in e&&n(101,v=e.$$scope)},e.$$.update=()=>{if(67108864&e.$$.dirty[1]&&(ee||n(57,ee=qe)),134217728&e.$$.dirty[1]&<(ie),1&e.$$.dirty[0]&&w&&Ze.set(!1),4194304&e.$$.dirty[2]&&ye&&"object"==typeof ye&&n(30,st=Object.assign({},Vn.i18n,ye)),402653184&e.$$.dirty[0]|1090519040&e.$$.dirty[1]|805306369&e.$$.dirty[2]&&Ee&&dt!==S&&S.length){const e=Be("value",S||null,Ce),t=Be("label",S||null,Ce);A||Me===e||n(90,Ce.valueField=n(27,Me=e),Ce),I||Le===t||n(90,Ce.labelField=n(28,Le=t),Ce)}if(16777216&e.$$.dirty[0]&&n(90,Ce.labelAsValue=Se,Ce),16777216&e.$$.dirty[1]|268435456&e.$$.dirty[2]&&n(94,i=Ue(S,Ce)),402653186&e.$$.dirty[0]|1&e.$$.dirty[1]|276824064&e.$$.dirty[2]|1&e.$$.dirty[3]){const e=ot.map((e=>{const{$disabled:t,$isGroupItem:n,...i}=e;return i})),t=j?e:e.length?e[0]:null,i=Ce.labelAsValue?Le:Me;n(93,rt=Ae?t:j?t.map((e=>e[i])):ot.length?t[i]:null),n(59,we=rt),n(60,xe=t)}268435456&e.$$.dirty[1]|1&e.$$.dirty[3]&&rt!==we&&ht(we),1&e.$$.dirty[1]|512&e.$$.dirty[2]&&n(34,l=q&&ot.length==q),2&e.$$.dirty[0]|24&e.$$.dirty[1]|272105472&e.$$.dirty[2]|2&e.$$.dirty[3]&&n(33,r=l?[]:function(e,t,n,i,l,r){if(n&&(e=e.filter((e=>!n.has(e[r.valueField]))).filter(((e,t,n)=>!e.$isGroupHeader||!(n[t+1]&&n[t+1].$isGroupHeader||n.length<=1||n.length-1===t)))),!t)return e;const s=new ke(e);r.optionsWithGroups&&(s.getSortFunction=()=>null);let o="and";t.startsWith("|| ")&&(o="or",t=t.substr(2));const a=s.search(t,{fields:i||r.optionProps,sort:(c=l||r.labelField,[{field:c,direction:"asc"}]),conjunction:o});var c;return r.optionsWithGroups?a.items.reduce(((t,i)=>{const l=e[i.id];if(n&&l.isSelected)return t;const r=t.push(l);if(l.$isGroupItem){const n=e.slice(0,i.id);let l=null;do{l=n.pop(),l&&l.$isGroupHeader&&!t.includes(l)&&t.splice(r-1,0,l)}while(l&&!l.$isGroupHeader)}return t}),[]):a.items.map((t=>e[t.id]))}(i,ve?null:h,!!j&&at,me,ge,Ce)),131072&e.$$.dirty[0]|20&e.$$.dirty[1]&&(s=W&&h?r.length:r.length-1),131072&e.$$.dirty[0]|20&e.$$.dirty[1]|268435456&e.$$.dirty[2]&&n(32,o=function(e,t,n){const i=n.optionsWithGroups?e.reduce(((e,t,n)=>(e.push(t.$isGroupHeader?"":n),e)),[]):Object.keys(e);return{map:i,first:""!==i[0]?0:1,last:i.length?i.length-(t?0:1):0,hasCreateRow:!!t,next(e,t){const n=this.map[++e];return this.hasCreateRow&&e===this.last?this.last:""===n?this.next(e):void 0===n?this.map.length?(e>this.map.length&&(e=this.first-1),!0===t?this.prev(e):this.next(e)):0:n},prev(e){const t=this.map[--e];return this.hasCreateRow&&e===this.first?this.first:""===t?this.prev(e):t||this.last}}}(r,W&&h,Ce)),67108864&e.$$.dirty[0]|18&e.$$.dirty[1]|16&e.$$.dirty[2]&&(null===Te&&(M||h)?n(26,Te=o.first):Te>o.last?n(26,Te=o.last):Te<o.first&&n(26,Te=o.first)),167772162&e.$$.dirty[0]|1&e.$$.dirty[1]|6&e.$$.dirty[3]&&!j&&!1===p&&ot.length&&(n(26,Te=i.findIndex((e=>e[Me]===ot[0][Me]))),Oe&&de().then(Oe.scrollIntoView)),1610612736&e.$$.dirty[0]|134217756&e.$$.dirty[1]|1074004480&e.$$.dirty[2]&&n(42,a=l?st.max(q):h.length&&0===r.length&&ce<=1?st.nomatch:ie?ce<=1?et?Ye?st.fetchInit:st.empty:st.fetchBefore:st.fetchQuery(ce,h.length):st.empty),268435456&e.$$.dirty[0]|8&e.$$.dirty[2]&&n(41,d="function"==typeof D?D:Pn[D]||Pn.default.bind({label:Le})),50331664&e.$$.dirty[1]&&n(40,u=Z(h,S))},[w,j,b,$,y,x,O,F,k,E,T,_,V,N,B,G,K,W,ue,he,pe,fe,be,$e,Se,Oe,Te,Me,Le,Ye,st,ot,o,r,l,h,Fe,_e,He,ct,u,d,a,Ie,Pe,Re,Ke,Xe,mt,gt,function(e){De?De=!1:n(26,Te=e.detail)},function(e){if(e=e.detail,W&&X.indexOf(e.key)>-1)return h.length>0&&mt(null,h),void e.preventDefault();const t=L&&p&&!e.shiftKey?"Tab":"No-tab";let i=_e?e.metaKey:e.ctrlKey,l=["PageUp","PageDown"].includes(e.key);switch(e.key){case"End":if(0!==h.length)return;n(26,Te=o.first);case"PageDown":if(l){const[e,t]=Oe.getDimensions();n(26,Te=Math.ceil((t*Te+e)/t))}case"ArrowUp":if(e.preventDefault(),!p)return g(Ze,p=!0,p),void(null===Te&&n(26,Te=o.first));n(26,Te=o.prev(Te)),de().then(Oe.scrollIntoView),De=!0;break;case"Home":if(0!==h.length||0===h.length&&0===r.length)return;n(26,Te=o.last);case"PageUp":if(l){const[e,t]=Oe.getDimensions();n(26,Te=Math.floor((t*Te-e)/t))}case"ArrowDown":if(e.preventDefault(),!p)return g(Ze,p=!0,p),void(null===Te&&n(26,Te=o.first));n(26,Te=null===Te?o.first:o.next(Te)),de().then(Oe.scrollIntoView),De=!0;break;case"Escape":p&&(e.preventDefault(),e.stopPropagation()),h||g(Ze,p=!1,p),it(),g(Ke,h="",h);break;case t:case"Enter":if(!p)return void(e.key!==t&&ze("enterKey",e));let a=i?null:r[Te];if(W&&h&&(a=!a||i?h:a,i=!1),!i&&a&&mt(null,a),r.length<=Te&&n(26,Te=s>0?s:o.first),!a&&ot.length)return g(Ze,p=!1,p),void(e.key!==t&&ze("enterKey",e));(e.key!==t||e.key===t&&"select-navigate"!==L)&&e.preventDefault();break;case" ":ie||p||(g(Ze,p=!0,p),e.preventDefault()),!j&&ot.length&&e.preventDefault();break;case"Backspace":vt=!0;case"Delete":""===h&&ot.length&&(i?gt({}):gt(null,ot[ot.length-1]),e.preventDefault()),vt=!1;default:i||["Tab","Shift"].includes(e.key)||p||Ye||g(Ze,p=!0,p),!j&&ot.length&&"Tab"!==e.key&&(e.preventDefault(),e.stopPropagation())}},function(e){if(W){e.preventDefault();const t=new RegExp("([^"+X+"\\n]+)","g"),n=e.clipboardData.getData("text/plain").replace(/\//g,"/").replace(/\t/g," "),i=n.match(t);1===i.length&&-1===n.indexOf(",")&&g(Ke,h=i.pop().trim(),h),i.forEach((e=>mt(null,e.trim())))}},function(e){n(31,ot=e.detail.items)},Ze,S,Z,ee,ie,we,xe,A,I,z,C,D,M,L,H,P,R,q,Q,U,J,X,re,se,oe,ae,ce,me,ge,ve,ye,Ae,e=>{Fe.focusControl(e)},e=>{if(!ot.length)return j?[]:null;const t=ot.map((t=>e?t[Se?Le:Me]:Object.assign({},t)));return j?t:t[0]},(e,t)=>{ht(e),t&&ut()},e=>{ft(),ut(),e&&(n(0,w=!0),n(58,ie=null))},Ce,Ee,et,rt,i,p,m,function(e){le[e?"unshift":"push"]((()=>{Fe=e,n(36,Fe)}))},function(t){ne.call(this,e,t)},function(e){le[e?"unshift":"push"]((()=>{Oe=e,n(25,Oe)}))},function(t){ne.call(this,e,t)},v]}class Nn extends Fe{constructor(e){super(),Oe(this,e,Rn,Hn,o,{name:2,inputId:3,required:4,hasAnchor:5,disabled:0,options:55,valueField:61,labelField:62,groupLabelField:63,groupItemsField:64,disabledField:6,placeholder:7,searchable:8,clearable:9,renderer:65,disableHighlight:10,highlightFirstItem:66,selectOnTab:67,resetOnBlur:11,resetOnSelect:68,closeAfterSelect:69,dndzone:12,validatorAction:70,dropdownItem:13,controlItem:14,multiple:1,max:71,collapseSelection:15,alwaysCollapsed:16,creatable:17,creatablePrefix:72,allowEditing:73,keepCreated:74,delimiter:75,createFilter:56,createTransform:57,fetch:58,fetchMode:76,fetchCallback:77,fetchResetOnBlur:78,fetchDebounceTime:79,minQuery:80,lazyDropdown:18,virtualList:19,vlHeight:20,vlItemSize:21,searchField:81,sortField:82,disableSifter:83,class:22,style:23,i18n:84,readSelection:60,value:59,labelAsValue:24,valueAsObject:85,focus:86,getSelection:87,setSelection:88,clearByParent:89},yn,[-1,-1,-1,-1])}get focus(){return this.$$.ctx[86]}get getSelection(){return this.$$.ctx[87]}get setSelection(){return this.$$.ctx[88]}get clearByParent(){return this.$$.ctx[89]}}const Bn=["options","value","name","required","disabled","value-field","label-field","disabled-field","placeholder","searchable","clearable","renderer","disable-highlight","select-on-tab","reset-on-blur","reset-on-select","multiple","max","collapse-selection","creatable","creatable-prefix","allow-editing","keepCreated","delimiter","fetch","fetch-reset-on-blur","min-query","lazy-dropdown","virtual-list","vl-height","vl-item-size","search-field","sort-field","disable-sifter","label-as-value"];function jn(e,t){switch(e){case"options":if(Array.isArray(t))return t;try{t=JSON.parse(t),Array.isArray(t)||(t=[])}catch(e){t=[]}return t;case"renderer":return t||"default";case"required":case"disabled":case"searchable":case"clearable":case"disable-highlight":case"select-on-tab":case"reset-on-blur":case"reset-on-select":case"multiple":case"collapse-selection":case"creatable":case"allow-editing":case"keep-created":case"fetch-reset-on-blur":case"lazy-dropdown":case"virtual-list":case"disable-sifter":case"label-as-value":return null!==t&&"false"!==t;case"max":return isNaN(parseInt(t))?0:parseInt(t);case"min-query":return isNaN(parseInt(t))?Vn.minQuery:parseInt(t)}return t}function qn(e){return e.includes("-")?e.split("-").reduce(((e,t,n)=>(n&&(t=t[0].toUpperCase()+t.substr(1)),e+t)),""):e}let Gn=!1;class Kn extends HTMLElement{constructor(){super(),this.svelecte=void 0,this.anchorSelect=null,this._fetchOpts=null,this._selfSetValue=!1;const e={name:{get(){this.getAttribute("name")},set(e){this.setAttribute("name",e)}},selection:{get(){return this.svelecte?this.svelecte.getSelection():null}},value:{get(){return this.svelecte?this.svelecte.getSelection(!0):null},set(e){const t=this.getAttribute("value-delimiter")||",";this.setAttribute("value",Array.isArray(e)?e.join(t):e)}},options:{get(){return this.hasAttribute("options")?JSON.parse(this.getAttribute("options")):this._fetchOpts||[]},set(e){this.setAttribute("options",Array.isArray(e)?JSON.stringify(e):e)}},hasAnchor:{get(){return!!this.anchorSelect}},form:{get(){return this.closest("form")}},emitChange:{get(){return Gn=!0,this}},valueField:{get(){return this.getAttribute("value-field")||Vn.valueField},set(e){this.setAttribute("value-field",e)}},labelField:{get(){return this.getAttribute("label-field")||Vn.labelField},set(e){this.setAttribute("label-field",e)}},delimiter:{get(){return this.getAttribute("delimiter")||Vn.delimiter},set(e){this.setAttribute("delimiter",e)}},lazyDropdown:{get(){return!!this.hasAttribute("lazy-dropdown")||Vn.lazyDropdown},set(){console.warn("⚠ this setter has no effect after component has been created")}},placeholder:{get(){return this.getAttribute("placeholder")||Vn.placeholder},set(e){this.setAttribute("placeholder",e)}},max:{get(){return this.getAttribute("max")||Vn.max},set(e){try{(e=parseInt(e))<0&&(e=0)}catch(t){e=0}this.setAttribute("max",e)}},minQuery:{get(){return this.getAttribute("min-query")||Vn.minQuery},set(e){try{(e=parseInt(e))<1&&(e=1)}catch(t){e=Vn.minQuery}this.setAttribute("min-query",e)}},creatablePrefix:{get(){return this.getAttribute("creatable-prefix")||Vn.creatablePrefix},set(e){this.setAttribute("creatable-prefix",e)}},renderer:{get(){return this.getAttribute("renderer")||"default"},set(e){e?this.setAttribute("renderer",e):this.removeAttribute("renderer")}}},t=["searchable","clearable","disable-highlight","required","select-on-tab","reset-on-blur","reset-on-select","multiple","collapse-selection","creatable","allow-editing","keep-created","fetch-reset-on-blur","virtual-list","disable-sifter","label-as-value","disabled"].reduce(((e,t)=>{const n=qn(t);return e[n]={get(){const e=this.hasAttribute(t),i=!e||"false"!==this.getAttribute(t);return e?i:Vn[n]},set(e){e?this.setAttribute(t,e=""):this.hasAttribute(t)?this.removeAttribute(t):this.svelecte&&this.svelecte.$set({[n]:e})}},e}),{});Object.defineProperties(this,Object.assign({},e,t))}focus(){!this.disabled&&this.querySelector("input").focus()}static get observedAttributes(){return Bn}attributeChangedCallback(e,t,n){if(this.svelecte&&t!==n){if("value"===e)return this._selfSetValue||(n?this.svelecte.setSelection(jn(e,n),Gn):this.svelecte.clearByParent(!!this.parent)),this._selfSetValue=!1,Gn=!1,void(this.anchorSelect&&setTimeout((()=>{const e=this.svelecte.getSelection(!0);this.anchorSelect.innerHTML=(Array.isArray(e)?e.length?e:[null]:[e]).reduce(((e,t)=>e+=t?`<option value="${t}" selected>${t}</option>`:'<option value="" selected="">Empty</option>'),"")})));this.svelecte.$set({[qn(e)]:jn(e,n)})}}connectedCallback(){setTimeout((()=>{this.render()}))}render(){if(this.svelecte)return;let e={};for(const i of Bn)this.hasAttribute(i)&&(e[qn(i)]="value"!==i?jn(i,this.getAttribute(i)):(t=this.getAttribute("value"),n=this.getAttribute("value-delimiter")||",",t?t.split(n).map((e=>{const t=parseInt(e);return isNaN(t)?"null"!==e?e:null:t})):""));var t,n;if(this.hasAttribute("i18n")){const t=JSON.parse(this.getAttribute("i18n"));if(t.createRowLabel){const e=t.createRowLabel;t.createRowLabel=t=>e.replace("#value",t)}e.i18n=t}if(this.hasAttribute("class")&&(e.class=this.getAttribute("class")),this.hasAttribute("parent")){if(this.parent=document.getElementById(this.getAttribute("parent")),!this.parent.value&&this.svelecte)return;const t=this.parent.value||this.parent.getAttribute("value");t?(e.disabled=!1,e.fetch=this.getAttribute("fetch").replace("[parent]",t)):(delete e.fetch,e.disabled=!0),this.parentCallback=e=>{if(!e.target.selection||Array.isArray(e.target.selection)&&!e.target.selection.length)this.svelecte.clearByParent(!0);else if(!this.parent.disabled&&this.removeAttribute("disabled"),this.hasAttribute("fetch")){this.svelecte.clearByParent(!0);const t=this.getAttribute("fetch").replace("[parent]",e.target.value);this.svelecte.$set({fetch:t,disabled:!1})}},this.parent.addEventListener("change",this.parentCallback)}const i=this.querySelector("select");return i&&(e.hasAnchor=!0,i.style="opacity: 0; position: absolute; z-index: -2; top: 0; height: 38px",i.tabIndex=-1,this.anchorSelect=i,this.anchorSelect.multiple=e.multiple||i.name.includes("[]"),this.anchorSelect.innerHTML="",(Array.isArray(e.value)?e.value:[e.value||null]).forEach((e=>{this.anchorSelect.innerHTML+=`<option value="${e||""}" selected>${e||"No value"}</option>`}))),this.svelecte=new Nn({target:this,anchor:i,props:e}),this.svelecte.$on("change",(e=>{const t=this.svelecte.getSelection(!0);this._selfSetValue=!0,this.value=t,setTimeout((()=>{this._selfSetValue=!1}),100),this.anchorSelect&&(this.anchorSelect.innerHTML=(Array.isArray(t)?t.length?t:[null]:[t]).reduce(((e,t)=>e+=t?`<option value="${t}" selected>${t}</option>`:'<option value="" selected="">Empty</option>'),""),this.anchorSelect.dispatchEvent(new Event("change"))),this.dispatchEvent(e)})),this.svelecte.$on("fetch",(e=>{this._fetchOpts=e.detail,this.dispatchEvent(e)})),this.svelecte.$on("createoption",(e=>{this.dispatchEvent(e)})),!0}disconnectedCallback(){this.svelecte&&this.svelecte.$destroy(),this.parent&&this.parent.removeEventListener("change",this.parentCallback)}}return e.addFormatter=function(e,t){if(e instanceof Object)for(let t in e)Pn[t]=e[t];else Pn[e]=t},e.config=Vn,e.registerSvelecte=function(e){window.customElements.define(e||"el-svelecte",Kn)},Object.defineProperty(e,"__esModule",{value:!0}),e}({});
The code you've shared appears to be a part of a complex JavaScript or TypeScript library or application, possibly related to a web UI component. The code seems to define behavior and rendering logic for a custom UI component, potentially a select box or dropdown menu, judging by variable and function names like dropdownIndex
, selectOnTab
, resetOnBlur
, clearable
, searchable
, itemComponent
, etc.
Given the complexity and length of the code, it's challenging to deduce the specific technique or problem-solving approach used without more context or specific questions. However, some general observations can be made:
Modular Structure: The code is modular, with functions and components organized in a way that suggests a focus on reusability and separation of concerns.
Event Handling: There's a significant focus on event handling (
addEventListener
,dispatchEvent
, etc.), which is typical in interactive UI components to respond to user actions.State Management: The use of state management is evident (
We
,Ke
,Ze
, etc.), likely to keep track of the component's current state (like selected items, open/close state of dropdowns).Conditional Rendering: There are conditions that determine how different parts of the component are rendered (
if...else
statements, ternary operators).Accessibility Features: Some parts of the code suggest attention to accessibility (like
aria-hidden
), which is crucial for modern web development.Customization and Configuration: The presence of many options and settings (
clearable
,searchable
,placeholder
, etc.) indicates that the component is designed to be highly customizable.CSS Styling: The use of classes and styling (
svelte-1e087o6
,svelte-17904zl
, etc.) suggests that CSS is being used for styling, which is typical in web development.Svelte Framework: The use of
svelte
in class names and the way the code is structured suggest that this component might be built using the Svelte framework, a modern tool for building reactive web interfaces.Complex Interactions: The complexity of functions and the variety of features imply that the component is designed for complex interactions, possibly in a sophisticated web application.
If you have specific questions about parts of this code or need clarification on a certain technique, please feel free to ask!is there conditional logic around HTMLELement? -blur":case"reset-on-select":case"multiple":case"collapse-selection":case"creatable":case"allow-editing":case"keep-created":case"fetch-reset-on-blur":case"lazy-dropdown":case"virtual-list":case"disable-sifter":case"label-as-value":return null!==t&&"false"!==t;case"max":return isNaN(parseInt(t))?0:parseInt(t);case"min-query":return isNaN(parseInt(t))?Vn.minQuery:parseInt(t)}return t}function qn(e){return e.includes("-")?e.split("-").reduce(((e,t,n)=>(n&&(t=t[0].toUpperCase()+t.substr(1)),e+t)),""):e}let Gn=!1;class Kn extends HTMLElement{constructor(){super(),this.svelecte=void 0,this.anchorSelect=null,this._fetchOpts=null,this._selfSetValue=!1;const e={name:{get(){this.getAttribute("name")},set(e){this.setAttribute("name",e)}},selection:{get(){return this.svelecte?this.svelecte.getSelection():null}},value:{get(){return this.svelecte?this.svelecte.getSelection(!0):null},set(e){const t=this.getAttribute("value-delimiter")||",";this.setAttribute("value",Array.isArray(e)?e.join(t):e)}},options:{get(){return this.hasAttribute("options")?JSON.parse(this.getAttribute("options")):this._fetchOpts||[]},set(e){this.setAttribute("options",Array.isArray(e)?JSON.stringify(e):e)}},hasAnchor:{get(){return!!this.anchorSelect}},form:{get(){return this.closest("form")}},emitChange:{get(){return Gn=!0,this}},valueField:{get(){return this.getAttribute("value-field")||Vn.valueField},set(e){this.setAttribute("value-field",e)}},labelField:{get(){return this.getAttribute("label-field")||Vn.labelField},set(e){this.setAttribute("label-field",e)}},delimiter:{get(){return this.getAttribute("delimiter")||Vn.delimiter},set(e){this.setAttribute("delimiter",e)}},lazyDropdown:{get(){return!!this.hasAttribute("lazy-dropdown")||Vn.lazyDropdown},set(){console.warn("⚠ this setter has no effect after component has been created")}},placehol
The provided code snippet appears to be a part of a JavaScript class definition, specifically for a custom HTML element. This class, Kn
, extends HTMLElement
, suggesting it's meant to define a new type of HTML element with custom behavior.
The code includes several cases within a switch
statement that seem to handle different attribute values of the custom element. The switch
statement is part of a function (not fully visible in the snippet) that likely processes attribute values.
Here’s a breakdown of the key parts:
Attribute Processing (
switch
statement): This part checks the attribute names (like"max"
,"min-query"
, etc.) and processes their values accordingly. For example, in the case of"max"
, it parses the attribute value as an integer, usingparseInt(t)
. If it's not a number (isNaN
), it defaults to0
.Utility Function (
qn
): This function seems to convert hyphenated strings into camelCase. For instance,"this-is-a-test"
would become"thisIsATest"
.Class Definition (
Kn
): The class extendsHTMLElement
, indicating it's a custom web component. It has properties defined in its constructor (likethis.svelecte
) and getters/setters for various attributes (name
,selection
,value
, etc.).Custom Logic and Warnings: There's a
lazyDropdown
getter and an empty setter with a warning, indicating that this property can't be set after the component is created.
The code is incomplete and out of context, so certain assumptions have to be made about its overall functionality. The custom element seems to be designed for enhanced select/input functionality in a web application, possibly a component for a dropdown or a complex input field. The use of custom attributes and their processing suggests a highly configurable element, perhaps part of a larger UI framework or library.
SSR Error: HTMLElement Not Defined created at Tue Jan 20 1970 17:44:07 GMT+0000 (Coordinated Universal Time)Related Chats
SSR Error: HTMLElement Not Defined 1.000
New chat 0.587
Test Svelte components with Jest. 0.587
Svelte Component Help 0.580
Client-Only Component in SvelteKit 0.563
Reactivity Issue Resolution 0.536
New chat 0.518
Passing inputValue to slot 0.517