Swift HashMap Cache Bool
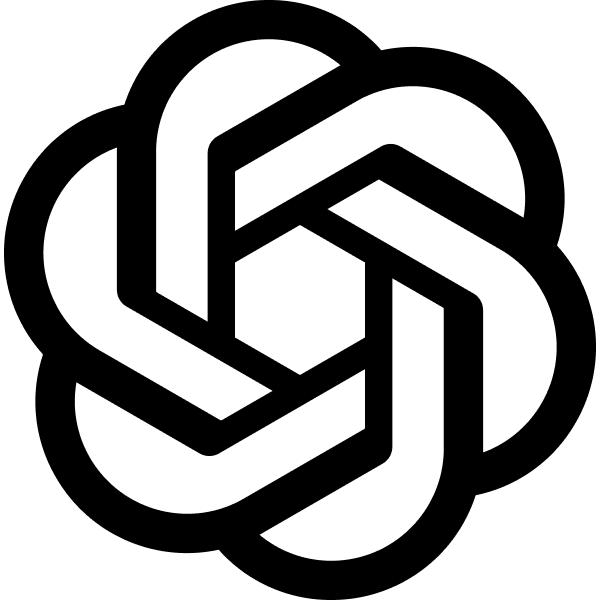
TODO: collapasable, copyable, download, etc
In swift, I want to have a hashmap from UInt64 to Bool so I can cache if I've seen a UUIdIn Swift, you can use a Dictionary
to create a hashmap from UInt64
to Bool
. This allows you to cache whether you have seen a UUID. Here's a simple example:
// Create a dictionary to store the cache
var uuidCache: [UInt64: Bool] = [:]
// Function to check if a UUID has been seen
func hasSeenUUID(_ uuid: UInt64) -> Bool {
if let seen = uuidCache[uuid] {
return seen
} else {
uuidCache[uuid] = true
return false
}
}
// Example usage
let uuid1: UInt64 = 12345678901234567890
let uuid2: UInt64 = 98765432109876543210
print(hasSeenUUID(uuid1)) // Output: false (first time seeing uuid1)
print(hasSeenUUID(uuid1)) // Output: true (already seen uuid1)
print(hasSeenUUID(uuid2)) // Output: false (first time seeing uuid2)
In this example:
uuidCache
is a dictionary that mapsUInt64
toBool
.- The function
hasSeenUUID(_:)
checks if a UUID has been seen. If it has been seen, it returnstrue
; otherwise, it adds the UUID to the cache and returnsfalse
.
This simple caching mechanism helps you keep track of whether a UUID has been encountered before.This won't compile, how can I use this ideom and make it compile? if event.entityA.name == "Enemy" && !priorCollisions[event.entityA.id]? {
To make your code compile, you need to properly handle the optional value returned by the dictionary. You can use optional binding (if let
) to unwrap the optional value and provide a default value if the key doesn't exist in the dictionary. Here’s how you can modify your code:
// Assuming you have the following dictionary to cache collisions
var priorCollisions: [UInt64: Bool] = [:]
// Example event structure
struct Event {
var entityA: Entity
}
struct Entity {
var name: String
var id: UInt64
}
// Example event
let event = Event(entityA: Entity(name: "Enemy", id: 1234567890))
// Check if the entity name is "Enemy" and if it has not been seen before
if event.entityA.name == "Enemy" && !(priorCollisions[event.entityA.id] ?? false) {
// Mark the entity as seen
priorCollisions[event.entityA.id] = true
// Handle the event for a new collision with an enemy
print("New collision with Enemy")
} else {
// Handle the event for a repeated collision or non-enemy entity
print("Collision with previously seen entity or non-enemy")
}
In this modified code:
priorCollisions[event.entityA.id] ?? false
uses the nil-coalescing operator (??
) to provide a default value offalse
if the key does not exist in the dictionary. This ensures that the expression compiles and evaluates correctly.- If the entity name is "Enemy" and the entity has not been seen before, the code will mark the entity as seen by updating the
priorCollisions
dictionary and print "New collision with Enemy". - Otherwise, it handles the event for a repeated collision or non-enemy entity and prints "Collision with previously seen entity or non-enemy".
This approach ensures that your code compiles and behaves as expected.I have var priorCollisions: [UInt64: Bool] = [:]
on my top level struct TrainerView: View {
When I try to do func subscribeToCollisions(content: RealityViewContent) { // TODO: do we need to cache the entity id to avoid spawning multiple times? let sub = content.subscribe(to: CollisionEvents.Began.self) {event in if event.entityA.name == "Enemy" && !(priorCollisions[event.entityA.id] ?? false) { Task { priorCollisions[event.entityA.id] = true
It says Cannot assign through subscript: 'self' is immutable
. What is the best way to structure my code and fix this? Is there a simple fix? a better, but more complex fix?The user is working on Swift code involving collision events and entity handling in a view.Model set context updated.The issue you're encountering is because self
is immutable within the closure. One way to handle this is to use a class to manage the state that needs to be mutable within the closure. Here's an example of how you can structure your code:
- Create a class to manage the collision state.
- Use this class within your
TrainerView
.
Here's how you can implement this:
Step 1: Create a Class to Manage Collision State
class CollisionManager: ObservableObject {
@Published var priorCollisions: [UInt64: Bool] = [:]
func checkAndMarkCollision(for entityID: UInt64) -> Bool {
if priorCollisions[entityID] == true {
return true
} else {
priorCollisions[entityID] = true
return false
}
}
}
Step 2: Use This Class Within Your TrainerView
struct TrainerView: View {
@StateObject private var collisionManager = CollisionManager()
var body: some View {
// Your view content
}
func subscribeToCollisions(content: RealityViewContent) {
let sub = content.subscribe(to: CollisionEvents.Began.self) { event in
if event.entityA.name == "Enemy" && !self.collisionManager.checkAndMarkCollision(for: event.entityA.id) {
Task {
// Handle the event for a new collision with an enemy
print("New collision with Enemy")
}
} else {
// Handle the event for a repeated collision or non-enemy entity
print("Collision with previously seen entity or non-enemy")
}
}
}
}
Explanation
CollisionManager Class: This class manages the state of prior collisions and provides a method to check and mark collisions. The
@Published
property wrapper ensures that any changes topriorCollisions
will notify SwiftUI to update the view if needed.TrainerView Struct: The
@StateObject
property wrapper is used to create and manage theCollisionManager
instance. This allows the state to be mutable and shared across the view.subscribeToCollisions Method: This method subscribes to collision events and uses the
collisionManager
to check and mark collisions. By using theself.collisionManager.checkAndMarkCollision
method, we can mutate the state within the closure.
This approach separates the state management from the view logic, making the code more modular and easier to maintain.All I want to do is only run the code once per enemy, but collisions can be generated several times for the same enemy. Is there a simpler way to accomplish this?To achieve the goal of running code only once per enemy despite multiple collision events for the same entity, you can leverage Swift's built-in Set
to track seen enemies by their IDs. This approach is simpler and avoids the complexity of managing a Dictionary
. Here's how you can do it:
- Use a
Set
to store the IDs of enemies you've already processed. - Check for membership in the
Set
before running your code, and insert the ID into theSet
afterward.
Implementation
Here's how you can implement this in your TrainerView
:
struct TrainerView: View {
// Use a Set to track seen enemy IDs
@State private var seenEnemies: Set<UInt64> = []
var body: some View {
// Your view content
}
func subscribeToCollisions(content: RealityViewContent) {
let sub = content.subscribe(to: CollisionEvents.Began.self) { event in
if event.entityA.name == "Enemy" && !self.seenEnemies.contains(event.entityA.id) {
self.seenEnemies.insert(event.entityA.id)
Task {
// Your code to handle the first collision with this enemy
print("New collision with Enemy")
}
} else {
// Handle the event for a repeated collision or non-enemy entity
print("Collision with previously seen entity or non-enemy")
}
}
}
}
Explanation
State Management: A
Set
namedseenEnemies
is used to store the IDs of enemies.Set
is chosen because it provides efficient O(1) complexity for bothcontains
andinsert
operations.Collision Handling: In the
subscribeToCollisions
method, before running your collision handling code, you check if the enemy's ID is already in theseenEnemies
set. If not, you insert the ID into the set and proceed with your code.State Mutation: Using the
@State
property wrapper allows you to mutate theseenEnemies
set within the closure.
This approach ensures that your collision handling code runs only once per enemy, regardless of how many collision events are generated for the same entity.
Swift HashMap Cache Bool created at Tue Jan 20 1970 20:59:38 GMT+0000 (Coordinated Universal Time)Related Chats
Swift HashMap Cache Bool 1.000
Toggle System Image: Swift 0.414
Random Element Selection 0.408
Arrays de Arrays Swift. 0.380
Rust Enum for Strings 0.379
MD5 Hash in Rust 0.364
Looping in Swift 0.350
Swift: Convert Objective-C. 0.349