MD5 Hash in Rust
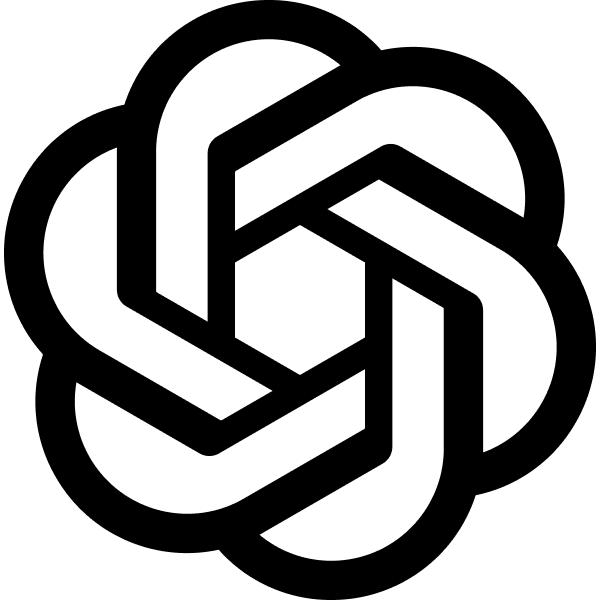
TODO: collapasable, copyable, download, etc
Can you write a snippet of Rust to take a &str and give me the md5 hash as a hexidecimal String?Certainly! Here's a simple Rust snippet that takes a &str
and returns its MD5 hash as a hexadecimal String
:
use std::fmt::Write;
use md5::{Md5, Digest};
fn compute_md5(input: &str) -> String {
let mut hasher = Md5::new();
hasher.update(input);
let result = hasher.finalize();
let mut hex_string = String::new();
for byte in result {
write!(hex_string, "{:02x}", byte).expect("Unable to write");
}
hex_string
}
fn main() {
let input = "Hello, World!";
let hash = compute_md5(input);
println!("MD5 hash of '{}': {}", input, hash);
}
To use this code, you'll need to add the md5
crate to your Cargo.toml
:
[dependencies]
md5 = "0.7"
This code initializes an Md5
hasher, updates it with the input string, finalizes the hash, and then converts the resulting bytes into a hexadecimal string.I get this error | use md5::{Md5, Digest}; | ^^^ no `Md5` in the root
I apologize for the oversight. The Md5
struct is directly available in the md5
crate's root, while Digest
is available in the digest
trait. Let's correct that.
Here's the corrected Rust snippet:
use std::fmt::Write;
use md5::Digest;
fn compute_md5(input: &str) -> String {
let mut hasher = md5::Md5::new();
hasher.update(input);
let result = hasher.finalize();
let mut hex_string = String::new();
for byte in result {
write!(hex_string, "{:02x}", byte).expect("Unable to write");
}
hex_string
}
fn main() {
let input = "Hello, World!";
let hash = compute_md5(input);
println!("MD5 hash of '{}': {}", input, hash);
}
Ensure you've added the md5
crate to your Cargo.toml
:
[dependencies]
md5 = "0.7"
With this corrected snippet, the code should compile and run without errors.What is the rust code to create a file path from a constant DISK_DIR
and a variable that is a &str
filename
?To create a file path in Rust, you can use the Path
and PathBuf
types from the std::path
module. If DISK_DIR
is a constant string representing a directory, and filename
is a &str
, you can concatenate them to form a complete path.
Here's how you can do it:
use std::path::{Path, PathBuf};
const DISK_DIR: &str = "/path/to/disk_dir"; // replace with your actual directory path
fn create_filepath(filename: &str) -> PathBuf {
let mut path = Path::new(DISK_DIR).to_path_buf();
path.push(filename);
path
}
fn main() {
let filename = "example.txt";
let full_path = create_filepath(filename);
println!("Full path: {:?}", full_path);
}
In this example:
- We use
Path::new(DISK_DIR)
to create aPath
instance from theDISK_DIR
constant. - We convert the
Path
to a
Related Chats
MD5 Hash in Rust 0.999
Rust Enum for Strings 0.440
Nested vec! Macro Usage 0.410
Rust Shell for LLM 0.387
Mixing Static Dynamic JSON 0.384
Serde Rename JSON Field 0.379
Population Percentage: 2.41% 0.369
Swift HashMap Cache Bool 0.364