Tests Funktion sortByMessageId
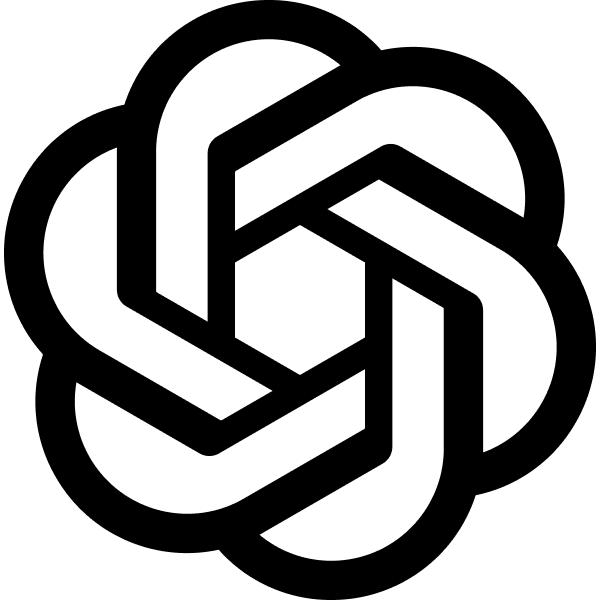
TODO: collapasable, copyable, download, etc
Given this code ```export function sortByMessageId(m1: ChatStreamMessage, m2: ChatStreamMessage): number {
const left = parseInt(m1.messageId, 10);
const right = parseInt(m2.messageId, 10);
// throw error if either is NaN
if (isNaN(left) || isNaN(right)) {
throw new Error("Invalid message ID");
}
if (left < right) {
return -1;
} else if (left > right) {
return 1;
} else {
return 0;
}
};```
Please write tests that start with ```import { describe, it, expect } from "vitest";
import { sortByMessageId } from "@/app/state/chat_history_state";
```To thoroughly test the sortByMessageId
function you provided, we need to consider various scenarios, including valid inputs where the function should correctly order the message IDs, inputs that might cause the function to throw errors, and edge cases like passing strings that don't convert neatly to integers. Below are some example test cases using Vitest's testing framework, including the necessary imports and setup:
import { describe, it, expect } from "vitest";
import { sortByMessageId } from "@/app/state/chat_history_state";
describe('sortByMessageId', () => {
it('should return -1 if first message ID is less than second message ID', () => {
const message1 = { messageId: '123' };
const message2 = { messageId: '124' };
expect(sortByMessageId(message1, message2)).toBe(-1);
});
it('should return 1 if first message ID is greater than second message ID', () => {
const message1 = { messageId: '125' };
const message2 = { messageId: '124' };
expect(sortByMessageId(message1, message2)).toBe(1);
});
it('should return 0 if both message IDs are equal', () => {
const message1 = { messageId: '123' };
const message2 = { messageId: '123' };
expect(sortByMessageId(message1, message2)).toBe(0);
});
it('should throw an error if first message ID is not a valid number', () => {
const message1 = { messageId: 'abc' };
const message2 = { messageId: '123' };
expect(() => sortByMessageId(message1, message2)).toThrow("Invalid message ID");
});
it('should throw an error if second message ID is not a valid number', () => {
const message1 = { messageId: '123' };
const message2 = { messageId: 'def' };
expect(() => sortByMessageId(message1, message2)).toThrow("Invalid message ID");
});
it('should throw an error if both message IDs are not valid numbers', () => {
const message1 = { messageId: 'abc' };
const message2 = { messageId: 'def' };
expect(() => sortByMessageId(message1, message2)).toThrow("Invalid message ID");
});
it('handles message IDs with leading zeros correctly', () => {
const message1 = { messageId: '007' };
const message2 = { messageId: '008' };
expect(sortByMessageId(message1, message2)).toBe(-1);
});
it('correctly compares large number message IDs', () => {
const message1 = { messageId: '999999999999999999999999999' };
const message2 = { messageId: '1000000000000000000000000000' };
expect(sortByMessageId(message1, message2)).toBe(-1);
});
});
This set of tests covers a wide array of situations:
- Basic Ordering: Tests basic scenarios where one ID is smaller, larger, or equal to another.
- Error Handling: Tests to ensure the function throws an error when the IDs cannot be converted to valid numbers.
- Edge Cases: Tests scenarios with leading zeros and very large numbers to ensure proper string to integer conversion and comparison.
These tests will help ensure that the sortByMessageId
function behaves as expected across various normal and edge cases.Once sorted, how would I reduce messages by concatenating all of the chatMessage.message items?To concatenate all chatMessage.message
items from a sorted array of chat messages, you can first sort the messages using the sortByMessageId
function and then use the reduce
method to concatenate the messages. This approach involves iterating over the sorted array and accumulating all messages into a single string.
Here is an example that demonstrates this process in TypeScript:
interface ChatStreamMessage {
messageId: string;
message: string;
}
// Function to sort messages by messageId
function sortByMessageId(m1: ChatStreamMessage, m2: ChatStreamMessage): number {
const left = parseInt(m1.messageId, 10);
const right = parseInt(m2.messageId, 10);
if (isNaN(left) || isNaN(right)) {
throw new Error("Invalid message ID");
}
return left - right;
}
// Function to concatenate messages from sorted chat messages
function concatenateMessages(messages: ChatStreamMessage[]): string {
// First, sort the messages by their IDs
const sortedMessages = messages.sort(sortByMessageId);
// Then, reduce the sorted array to a single string
const concatenatedMessages = sortedMessages.reduce((acc, message) => acc + message.message, "");
return concatenatedMessages;
}
// Example usage:
const messages: ChatStreamMessage[] = [
{ messageId: '002', message: 'Hello, ' },
{ messageId: '003', message: 'how are you?' },
{ messageId: '001', message: 'Hi ' }
];
const combinedMessage = concatenateMessages(messages);
console.log(combinedMessage); // Output: "Hi Hello, how are you?"
This code does the following:
sortByMessageId
Function: It sorts the messages based on theirmessageId
. The sorting is necessary to ensure the messages are concatenated in the correct order.concatenateMessages
Function: It takes an array ofChatStreamMessage
objects, sorts them, and then concatenates theirmessage
properties into a single string.
Make sure that each message's messageId
is correctly formatted to represent a valid number, as this example assumes that the IDs can be converted to integers without error. The approach effectively concatenates messages in order of their message IDs.
Related Chats
Tests untuk initializeNewThread 0.544
Sort Array by messageId 0.511
Teste de Autenticação Vitest 0.500
Mounting Issue with useEffect 0.401
Sort Array with JS 0.394
Nullish Coalescing Operator Summary 0.391
Fix Return Local Reference 0.388
Rust Chat App UI 0.380