Prisma HMR Disconnect Solution
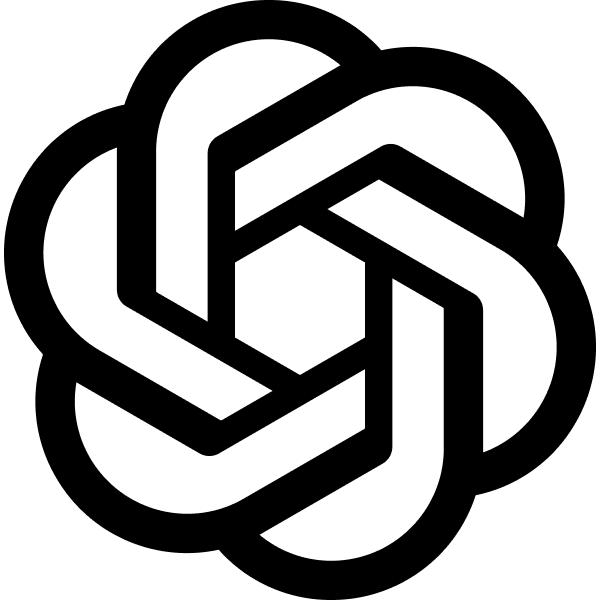
TODO: collapasable, copyable, download, etc
I'm using NextJS and prisma db client. Because of hot module reloading, prisma is creating too many open connectiosn. How can I detect that a module is being unloaded and call discconect on my singleton client?The issue you're encountering with Prisma and Next.js due to hot module reloading (HMR) creating too many open connections is common, and it generally occurs because the Prisma client creates a new connection every time a file is changed and the server module is reloaded. In development, with frequent changes, this can lead to many open connections.
To resolve this issue, you need to gracefully disconnect the Prisma client before the module is reloaded. Here’s a step-by-step approach on how to do this in a Next.js environment:
1. Create a Singleton Prisma Client
First, ensure that you are using a singleton pattern for your Prisma client, which means creating only one instance of the client throughout the application. This is commonly done in a file like prismaClient.js
:
import { PrismaClient } from '@prisma/client';
let prisma;
if (process.env.NODE_ENV === 'production') {
prisma = new PrismaClient();
} else {
if (!global.prisma) {
global.prisma = new PrismaClient();
}
prisma = global.prisma;
}
export default prisma;
2. Disconnect Prisma Client on HMR
Next, you need to detect when a module is going to be replaced and disconnect the Prisma client accordingly. You can add a check to your prismaClient.js
file that listens for HMR events:
if (process.env.NODE_ENV !== 'production') {
if (module.hot) {
module.hot.dispose(async () => {
await prisma.$disconnect();
});
}
}
This code checks if HMR is enabled (module.hot
), and if so, it uses the dispose
handler to disconnect the Prisma client whenever the current module is replaced due to an update.
3. Ensure Proper Module Replacement
It's essential to ensure that you've set up your Next.js development environment to support HMR properly. The HMR related setup should be correctly initialized in your next.config.js
or wherever you configure your Webpack settings (if you've customized them). Usually, Next.js handles this automatically unless custom configuration disrupts the default behavior.
4. Testing the Implementation
After implementing the above changes, test your application by making repeated changes to the files that use the Prisma client. Monitor the database connections to ensure they are being cleaned up correctly after each reload. Tools like the database management interface or monitoring tools provided by your database can help observe the open connections.
This approach should minimize the number of connections left open due to HMR in development mode. If you continue to face issues, ensure that the disposal logic is executed as expected and review if any other parts of your application might be interfering with the connection management.In Next.js, due to its hot module reloading (HMR) during development, modules can be frequently reloaded, which can cause issues with connections made in global scope, such as database connections using Prisma. When the module holding the Prisma client is reloaded, a new client is created without closing the previous connection, leading to too many open connections.
To handle this gracefully, you can leverage Node.js's module
system to detect when a module is being unloaded and properly close the connections. Unfortunately, there's no direct "module unloading" event in Node.js or in Next.js, but you can work around this limitation by using global variables and checking the state of these variables.
Here’s a step-by-step solution:
Singleton Pattern for Prisma Client: Ensure you are managing your Prisma client as a singleton. This pattern ensures that only one instance of the client is used throughout your application, which is crucial for avoiding multiple connections.
import { PrismaClient } from '@prisma/client'; let prisma; if (process.env.NODE_ENV === 'production') { prisma = new PrismaClient(); } else { if (!global.prisma) { global.prisma = new PrismaClient(); } prisma = global.prisma; } export default prisma;
Disconnect in Development Mode: Utilize the development mode check to add a disconnection hook. Since Next.js doesn't directly support a module unload event, we use an approach to add a cleanup function that Next.js does call when the module reloaded.
if (process.env.NODE_ENV !== 'production') { if (module.hot) { module.hot.dispose(() => { prisma.$disconnect(); }); } }
This code uses
module.hot.dispose
, a part of the Webpack Hot Module Replacement API, which allows you to add custom behavior when the current module is being replaced. Here, when your Prisma module gets disposed (due to HMR), it will close the Prisma client connection.Integrate the Dispose Function: Ensure that your custom
dispose
function is part of your Prisma client module or wherever you manage the lifecycle of your Prisma client.Testing: Since this involves development environment behavior, ensure you test it in your local development setup. Check that connections are being properly closed by monitoring your database connections or using logs.
Here’s how the complete module might look:
import { PrismaClient } from '@prisma/client';
let prisma;
if (process.env.NODE_ENV === 'production') {
prisma = new PrismaClient();
} else {
if (!global.prisma) {
global.prisma = new PrismaClient();
}
prisma = global.prisma;
if (module.hot) {
module.hot.dispose(() => {
console.log('Disposing old Prisma client instance');
prisma.$disconnect();
});
}
}
export default prisma;
This solution addresses the issue by taking advantage of the HMR lifecycle hooks provided by Webpack, which Next.js uses under the hood. Always make sure to test thoroughly to confirm that the connections are indeed closing as expected in your specific environment.Is this good enough? or do I need to use the global pattern to ensure a singleton?
// TODO: NextJs hot module re-loading doesn't play well with Prisma and we can get max connections error
// This is a workaround is to kill `npm run dev` and restart it
let prisma: PrismaClient | null = null;
if (module.hot) {
module.hot.dispose(async () => {
if (prisma) {
console.log('disconnecting');
await prisma.$disconnect();
}
});
}
export const prismaClient = (): PrismaClient => {
if (prisma) {
return prisma;
}
return new PrismaClient({
datasources: {
db: {
url: process.env.DATABASE_URL,
},
},
});
};```The approach you're using in your code snippet does address hot module reloading (HMR) by attempting to disconnect the Prisma client when a module is disposed. However, there are a few issues and areas for improvement that I'll point out:
1. **Instantiation of Prisma Client**:
- Your `prismaClient` function creates a new instance of `PrismaClient` every time it is called, if `prisma` is null. This does not effectively implement the singleton pattern because it only checks if `prisma` is null but doesn't update `prisma` after creating a new instance. This means a new client will be created on each call if `prisma` has been set to null or was never set.
2. **Updating the Singleton Pattern**:
- To ensure that only one instance of `PrismaClient` is used, you need to assign the new instance back to `prisma` within your function. This makes sure that the same instance is reused across your application, which is crucial for managing connections effectively.
3. **Enhancing the Disposal Logic**:
- The disposal logic you've included will work to disconnect the client when a module is replaced. However, you need to make sure that `prisma` is set back to null after disconnecting so that a new client can be correctly instantiated on the next call if needed.
Here’s how you can modify your code to address these issues:
```javascript
import { PrismaClient } from "@prisma/client";
let prisma: PrismaClient | null = null;
if (module.hot) {
module.hot.dispose(async () => {
if (prisma) {
console.log('disconnecting');
await prisma.$disconnect();
prisma = null; // Ensure prisma is set to null after disconnecting
}
});
}
export const prismaClient = (): PrismaClient => {
if (!prisma) {
prisma = new PrismaClient({
datasources: {
db: {
url: process.env.DATABASE_URL,
},
},
});
}
return prisma;
};
Key Adjustments:
- Singleton Initialization: This revised approach ensures
prisma
is only instantiated once and then reused, effectively implementing the singleton pattern. - Reset on Disconnect: Setting
prisma
to null after disconnecting ensures that a fresh instance can be created if needed post-disposal.
This setup should effectively manage database connections during development with HMR enabled, avoiding the problem of accumulating connections that could lead to reaching the connection limit.
Prisma HMR Disconnect Solution created at Tue Jan 20 1970 19:54:52 GMT+0000 (Coordinated Universal Time)Related Chats
DB Connection Middleware Setup 0.516
Next.js Middleware & HOC 0.396
Prisma Setup and Usage 0.347
Fix Warning: Use StateObject 0.342
Docker PostgreSQL Connection Issue 0.340
Check Closed Controller State 0.338
Provide EnvironmentObject TrainerView 0.316