Fetch Debouncing with JavaScript
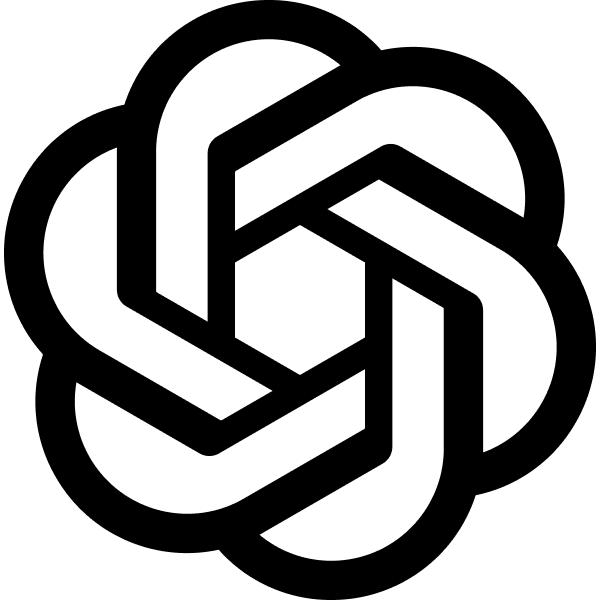
TODO: collapasable, copyable, download, etc
When I alter a form, I want to automatically send a fetch request. But I would like to avoid sending the fetch too much and the last one is the most important. So if I was going to send request r1, r2, r3, etc... What is the best way to think about structuring this?To handle a scenario where you have multiple fetch requests triggered by form alterations and you want to ensure that excessive requests are avoided while the most recent request is given priority, you can use a technique called debouncing. Debouncing is a programming practice used to ensure that time-consuming tasks do not fire so often, thus limiting the number of times a function is executed over time. This is particularly useful in your case to limit the number of fetch requests sent to the server while ensuring the most recent action is captured and processed.
Here's a high-level approach to structuring this with debouncing:
Attach an event listener to the form: This listener will detect changes in the form. For example, you could listen for the
input
,change
, orkeyup
events depending on your specific needs.Implement a debounce function: This function will delay the execution of the fetch request until a certain amount of idle time has passed since the last event trigger. If a new event is triggered before that time elapses, the previously scheduled fetch request is cancelled, and a new waiting period begins for the most recent action.
Execute the fetch request: Once the debounce timeout has been reached without any new events triggering, the fetch request is executed using the latest form data.
Here's an example of how you might implement this in JavaScript:
// Debounce function
function debounce(func, wait) {
let timeout;
return function executedFunction(...args) {
const later = () => {
clearTimeout(timeout);
func(...args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
// Function to execute fetch request
function sendFetchRequest() {
// Fetch request logic here
console.log("Fetch request sent with form data");
}
// Debounced version of the fetch request function
const debouncedFetchRequest = debounce(sendFetchRequest, 250); // Adjust wait time (ms) as needed
// Attach event listener to form
document.querySelector('form').addEventListener('input', (event) => {
// You could process form data here if necessary before sending
debouncedFetchRequest();
});
In this example, debounce
is a higher-order function that takes a function and a wait time as arguments. It returns a new function that, when called, resets the timeout if it's still counting down. If the countdown finishes (i.e., no events have occurred for the specified wait time), it calls the original function with the latest arguments.
Adjust the wait time in the debounce function to balance responsiveness with the desire to limit fetch requests. A common wait time for user input is between 200 and 500 milliseconds, but the best value depends on your specific use case and user experience requirements.Think about any bugs in your debounce function. Please re-write itUpon reviewing the provided debounce function, a potential improvement can be made to ensure that the this
context and the event arguments are correctly passed to the debounced function. This is particularly important when the debounced function relies on the context (this
) or arguments (event
) from where it was called, such as accessing form data or the event object in event listeners.
Here's a revised version of the debounce function that addresses this concern:
// Improved Debounce function
function debounce(func, wait) {
let timeout;
return function(...args) {
const context = this; // Capture 'this' context to use inside the later function
const later = function() {
timeout = null; // Reset timeout to allow the function to be called again after the wait period
func.apply(context, args); // Apply the function with the correct context and arguments
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
// If you want the function to execute immediately and then wait for a pause in events,
// you can check if timeout is not already set and call the function immediately.
// This part is optional and can be adjusted based on whether you need the leading edge execution.
};
}
// Function to execute fetch request
function sendFetchRequest() {
// Fetch request logic here
console.log("Fetch request sent with form data");
}
// Debounced version of the fetch request function
const debouncedFetchRequest = debounce(sendFetchRequest, 250); // Adjust wait time (ms) as needed
// Attach event listener to form
document.querySelector('form').addEventListener('input', (event) => {
// You could process form data here if necessary before sending
debouncedFetchRequest();
});
This revision ensures that:
- The
this
context is preserved, allowing the debounced function to be used in object methods wherethis
may refer to the calling object. - The event arguments (
...args
) are correctly passed to the function, ensuring that any data from the event can be utilized within the debounced function.
With these adjustments, the debounce function becomes more versatile and reliable in different contexts, such as event listeners or object methods, where the context (this
) and arguments (event
) are important for the function's execution.What will happen if debounce gets called 3 times in a row within 100ms?If the debounce function gets called three times in a row within 100ms, here's what will happen step-by-step, assuming a debounce wait time of 250ms for illustration:
First Call: The debounce function is called for the first time. It sets up a timeout to execute the wrapped function (
func
) after 250ms. At this point, the countdown begins.Second Call (within 100ms): Before the 250ms wait time expires, the debounce function is called again. Since this happens within the wait time (and in this case, within 100ms of the first call), the previous timeout is cleared and a new 250ms timeout is set. The countdown to execute
func
restarts from this point.Third Call (also within 100ms of the second call, totaling less than 200ms from the first call): Similarly, the second timeout is cleared before it has a chance to expire, and a new 250ms timeout is set again. The countdown to execute
func
is restarted once more.
The result of these three calls is that the function func
will not execute until 250ms have passed without any further calls to the debounce function. This means that despite being called three times in quick succession, func
will only execute once, 250ms after the last of the three calls.
This behavior is the core feature of debouncing: it prevents the function func
from being executed too frequently by only allowing it to execute after a pause in the rapid succession of calls. This is particularly useful for handling events that can fire many times in a short period, such as resizing windows, scrolling, or, as in your case, form inputs being updated.Please write unit tests for debounce using import { describe, it, expect } from 'vitest';To write unit tests for a debounce function using vitest
, you'll need to simulate the scenario where the debounce function is called multiple times in a short period, and verify that the function being debounced is called the correct number of times. You will also want to ensure that the debounced function respects the specified wait time before executing.
Here's an example of how you might structure your unit tests for the debounce function with vitest
. This assumes you have a debounce function defined similarly to the improved version provided earlier. For these tests, we'll use jest
's fake timers to control the passage of time in our tests, which vitest
is compatible with.
First, ensure you have the debounce function available to test. For these examples, let's assume it's defined in a file named debounce.js
.
// debounce.js
export function debounce(func, wait) {
let timeout;
return function(...args) {
const context = this;
const later = function() {
timeout = null;
func.apply(context, args);
};
clearTimeout(timeout);
timeout = setTimeout(later, wait);
};
}
Now, let's write some tests in debounce.test.js
:
import { describe, it, expect, vi } from 'vitest';
import { debounce } from './debounce';
describe('debounce', () => {
// Use vi.useFakeTimers to control setTimeout behavior
beforeEach(() => {
vi.useFakeTimers();
});
afterEach(() => {
vi.runOnlyPendingTimers(); // Ensure timers are flushed to clean state
vi.useRealTimers(); // Restore real timers after each test
});
it('executes the function only once within the wait time', () => {
const func = vi.fn();
const debouncedFunc = debounce(func, 250);
debouncedFunc();
debouncedFunc();
debouncedFunc();
vi.advanceTimersByTime(250); // Move time forward by 250ms
expect(func).toHaveBeenCalledTimes(1); // func should only have been called once
});
it('executes the function on the leading edge when called repeatedly', () => {
const func = vi.fn();
const debouncedFunc = debounce(func, 250);
debouncedFunc(); // Call it immediately
vi.advanceTimersByTime(50); // Move time forward less than the debounce wait time
debouncedFunc();
vi.advanceTimersByTime(50);
debouncedFunc();
vi.advanceTimersByTime(150); // Still less than the debounce time from the last call
expect(func).toHaveBeenCalledTimes(0); // func should not have been called yet
vi.advanceTimersByTime(100); // Move past the debounce wait time from the last call
expect(func).toHaveBeenCalledTimes(1); // func should now have been called once
});
it('respects the wait time between calls', () => {
const func = vi.fn();
const debouncedFunc = debounce(func, 250);
debouncedFunc(); // Call it once
vi.advanceTimersByTime(250); // Move time forward by the wait time
expect(func).toHaveBeenCalledTimes(1); // func should have been called once
debouncedFunc(); // Call it again after the wait time
vi.advanceTimersByTime(250); // Move time forward by another wait time
expect(func).toHaveBeenCalledTimes(2); // func should have been called again, totaling twice
});
});
In these tests, vi.fn()
creates a mock function that we can use to track calls to the function we're debouncing. vi.useFakeTimers()
and vi.useRealTimers()
are used to control and restore the timer functions like setTimeout
, ensuring we can simulate the passage of time in our tests. vi.advanceTimersByTime(ms)
is used to simulate the passage of time by a specified number of milliseconds, allowing us to test the debounce logic effectively.
Remember, these tests assume you're using a version of the debounce function compatible with this testing approach, specifically one that relies on setTimeout
. Adjustments may be needed depending on the specifics of your debounce implementation.
Related Chats
Fetch Debouncing with JavaScript 1.000
Stream REST Data in JavaScript 0.452
DB Connection Middleware Setup 0.399
Reset Saving State Strategically 0.356
Use Effect vs Component Body 0.342
Wrap in useCallback Hook 0.332
Next.js Middleware & HOC 0.332
Memo Once, No Dependencies 0.330