Wrap in useCallback Hook
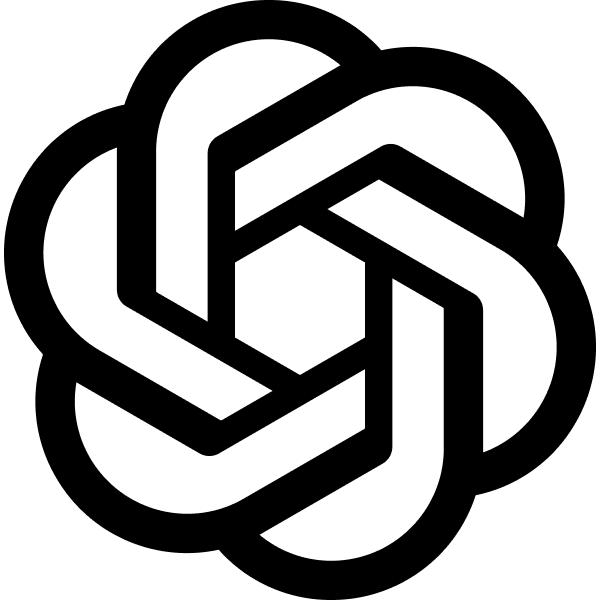
TODO: collapasable, copyable, download, etc
How would I wrap this code in useCallback
React hook? const handleNewThreadClick = () => { // We are going to show the modal which might result in a message being sent // but the user can also dismiss the modal, so we need to be able to revert the pendingSessionId setRestoreSessionId(pendingSessionId); setPendingSessionId(uuidv4()); setShowNewThreadModal(true); };
To wrap the handleNewThreadClick
function in the useCallback
React hook, you'll need to determine which variables and functions it depends on to properly define the dependency array of the hook. From your provided code, it appears that the function depends on pendingSessionId
, setRestoreSessionId
, setPendingSessionId
, and setShowNewThreadModal
.
Here's how you would wrap your function with useCallback
:
import { useCallback } from 'react';
import { v4 as uuidv4 } from 'uuid'; // make sure uuid is imported if not globally available
const handleNewThreadClick = useCallback(() => {
// We are going to show the modal which might result in a message being sent
// but the user can also dismiss the modal, so we need to be able to revert the pendingSessionId
setRestoreSessionId(pendingSessionId);
setPendingSessionId(uuidv4());
setShowNewThreadModal(true);
}, [pendingSessionId, setRestoreSessionId, setPendingSessionId, setShowNewThreadModal]);
Explanation
- Import
useCallback
: EnsureuseCallback
is imported from 'react'. - Import
uuidv4
: Ifuuidv4
is not already imported, you need to import it as shown. This is used to generate a new session ID. - Define the Callback: The function is defined exactly as it was but wrapped in
useCallback
. - Dependencies: The dependency array includes:
pendingSessionId
: Since the value ofpendingSessionId
is used within the callback.setRestoreSessionId
,setPendingSessionId
,setShowNewThreadModal
: These are setter functions fromuseState
that are unlikely to change across re-renders but are included for completeness and clarity.
Important Note
- Assumption of Stability: The setter functions from
useState
(setRestoreSessionId
,setPendingSessionId
,setShowNewThreadModal
) are stable and do not change on re-renders. They are included in the dependency array for completeness but typically do not cause unnecessary re-executions of the callback. - Performance Considerations:
useCallback
is useful for optimizing performance in cases where a function is passed as a prop to a child component or is used in a dependency array of other React hooks. If none of these scenarios apply, the use ofuseCallback
might not provide a significant benefit and could even introduce unnecessary complexity.
Related Chats
Wrap in useCallback Hook 0.999
Use Effect vs Component Body 0.540
Dismiss Pop-up on Click 0.513
Memo Once, No Dependencies 0.495
Reset Saving State Strategically 0.488
Mounting Issue with useEffect 0.481
Listen for Command+K. 0.467
Tests untuk initializeNewThread 0.463
No need setFoo. 0.461
Force Sync Read Atom. 0.421