Dropdown Button Component
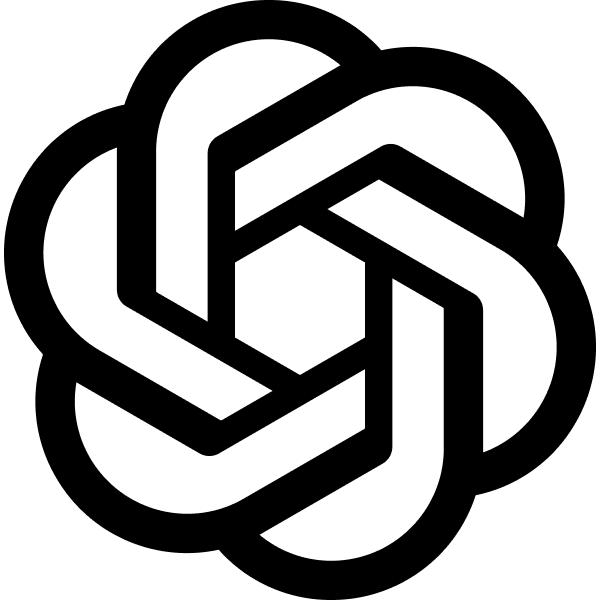
TODO: collapasable, copyable, download, etc
What do you call the UI component that looks like a button, but when you click it, it allows you to choose other options. Like a button that says [AVG] and when you click it, you see AVG, MIN, MAX?The UI component you're describing is commonly referred to as a "dropdown button" or "split button". This type of control combines the features of a button and a dropdown list. When clicked, it reveals a list of additional options (like AVG, MIN, MAX in your example), allowing the user to select one of these options. The main part of the button usually displays the currently selected option or a default action.In svelte, how can I give another component focus?In Svelte, giving focus to another component involves a few steps. Firstly, you need to use a bindable bind:this
attribute on the element you want to focus. This allows you to get a reference to the actual DOM node. Then, you can use this reference to call the .focus()
method on the desired element. Here's a basic example:
Component with Focusable Element (e.g., InputComponent.svelte):
<script> export let inputRef; </script> <input bind:this={inputRef} />
In this component,
inputRef
is an exportable variable that gets bound to the input element. This reference can be used outside this component to manipulate the input element, such as giving it focus.Parent Component:
<script> import InputComponent from './InputComponent.svelte'; let inputElement; // Function to focus the input element function focusInput() { if (inputElement) { inputElement.focus(); } } </script> <InputComponent bind:inputRef={inputElement} /> <button on:click={focusInput}>Focus the Input</button>
In the parent component, the
inputRef
fromInputComponent
is bound to a local variableinputElement
. ThefocusInput
function, when invoked (e.g., by clicking a button), will focus the input element insideInputComponent
.
Remember to use this pattern responsibly, as manipulating focus can affect accessibility and user experience. It's best used in cases where it enhances usability, like setting focus to a modal window when it opens or moving focus to an input field in a form when a specific action occurs.in svelte, what is the shortest, cleanest way to create on:click|preventDefault={handleClick}
but to give handleClick a value that such as currentValue
?In Svelte, to create an on:click|preventDefault={handleClick}
event handler and pass a value (like currentValue
) to the handleClick
function, you can use an inline arrow function within the event handler. This is a concise and clean way to pass additional arguments to your event handler. Here's an example:
Define the
handleClick
function:<script> let currentValue = 'someValue'; function handleClick(value, event) { // Handle the click event with the value console.log(value); // this will be 'someValue' console.log(event); // this will be the click event } </script>
Use an Inline Arrow Function in the Event Handler:
<button on:click|preventDefault={event => handleClick(currentValue, event)}> Click Me </button>
In this example, the inline arrow function in the on:click
handler takes the event object as its argument and calls handleClick
with currentValue
and the event. The preventDefault
modifier is applied to prevent the default action associated with the click event.
This approach is both short and clean, allowing you to pass additional data to your event handler function easily.It seems like event modifiers can't be used on custom components, only on html elements. Is that correct? Is this a flaw with SvelteYes, you are correct. In Svelte, event modifiers like preventDefault
, stopPropagation
, etc., are designed to be used on native HTML elements and not directly on custom Svelte components. This behavior is due to the way Svelte handles events and the DOM.
When you use an event modifier on a native HTML element, Svelte can directly apply the desired behavior (like preventing the default action) because it has control over the native event dispatching system. However, for custom components, Svelte does not inherently know what events the component might dispatch or how they are implemented internally.
If you want to achieve similar functionality with custom components, you have a couple of options:
Handle the Event Inside the Component: Modify the custom component to internally handle the event and apply any required logic (like
preventDefault
). The component can then emit a custom event, which the parent can listen to without needing an event modifier.Manual Event Handling in Parent: If modifying the component is not feasible, you can handle the event manually in the parent component. This involves listening to the event emitted by the custom component and then applying any required logic in the event handler.
Regarding whether this is a flaw in Svelte, it's more accurate to say that this is a design decision. Svelte aims to keep components encapsulated and predictable, and this approach to event handling is part of that philosophy. It encourages developers to handle component-specific logic within the component itself, promoting better encapsulation and reusability.Can this be simplified using bind
or another svelte technique?
import { Button, Dropdown, DropdownItem } from 'flowbite-svelte';
export let operator;
const operations = ['=', '!=', '>', '<'];
const handleClick = (chosenOperator) => {
operator = chosenOperator;
};
</script>
<Button color="dark" size="xs">{operator}</Button>
<Dropdown>
{#each operations as operatorOption}
{#if operatorOption != operator}
<DropdownItem on:click={(e) => handleClick(operatorOption)}>{operatorOption}</DropdownItem>
{/if}
{/each}
</Dropdown>
```Your Svelte code is already quite efficient, but there are a few tweaks that can be made to streamline it further. However, it's important to note that with Svelte, and UI frameworks in general, there's often a balance between making code more concise and keeping it readable and maintainable. Here are some suggestions:
1. **Direct Assignment in Event Handlers**: Instead of having a separate `handleClick` function, you can directly assign the new value to `operator` within the event handler. This is more concise but maintains clarity.
2. **Remove Redundant Check**: If your design allows showing the current `operator` in the dropdown list, you can remove the `{#if}` check. This simplifies the template, but the trade-off is that the current operator will also appear as an option in the dropdown.
3. **Using `bind`**: In this case, using `bind` is not particularly helpful because you are dealing with a list and handling click events to set a value. `bind` is more useful for two-way data bindings on form elements like input, select, etc.
Here's a revised version of your code with these considerations:
```svelte
<script>
import { Button, Dropdown, DropdownItem } from 'flowbite-svelte';
export let operator;
const operations = ['=', '!=', '>', '<'];
</script>
<Button color="dark" size="xs">{operator}</Button>
<Dropdown>
{#each operations as operatorOption}
<DropdownItem on:click={() => operator = operatorOption}>
{operatorOption}
</DropdownItem>
{/each}
</Dropdown>
In this version, clicking a DropdownItem
directly updates the operator
variable, removing the need for a separate handler function. This makes the code slightly more concise while retaining its clarity and functionality.
Remember, the best approach depends on your specific needs and preferences, especially in terms of code readability and maintainability.Should bind:this
work on a custom component ?Yes, bind:this
can be used with custom Svelte components, much like it is used with DOM elements. This binding gives you a reference to the component instance, allowing you to access its properties and methods from the parent component.
Here's a basic example of how it works:
Custom Component (CustomComponent.svelte):
<script> export let message = 'Hello from CustomComponent'; // A method you might want to call from the parent export function showAlert() { alert(message); } </script> <p>{message}</p>
Parent Component:
<script> import CustomComponent from './CustomComponent.svelte'; let customComponentInstance; // Function to call a method from the custom component function callComponentMethod() { if (customComponentInstance) { customComponentInstance.showAlert(); } } </script> <CustomComponent bind:this={customComponentInstance} /> <button on:click={callComponentMethod}>Call Component's Method</button>
In this example, bind:this
is used in the parent component to get a reference to the CustomComponent
instance. This reference (customComponentInstance
) can then be used to call methods defined in CustomComponent
, such as showAlert
in this case.
This technique is particularly useful for interacting with child components in a more imperative way, though it's generally recommended to prefer a declarative approach (using props and events) for most interactions in Svelte to maintain component encapsulation and reactivity.I have ``` let filterValueInputRef;
onMount(() => {
filterValueInputRef.focus();
})
<Typeahead
label=""
placeholder={(everywhere)
}
showAllResultsOnFocus
value={filter.value}
data={filterAttributeValues}
extract={(item) => item}
on:select={filterValueSelected}
on:clear={() => (events = [...events, 'clear'])}
bind:this={filterValueInputRef}
/>```
But in the console I get an error filterValueInputRef.focus is not a function
The issue you're encountering is related to how bind:this
works with custom Svelte components versus native HTML elements. When you use bind:this
on a native HTML element, it gives you a direct reference to the DOM element, which you can then use to call DOM methods like .focus()
. However, when you use bind:this
with a custom Svelte component, it gives you a reference to the component instance, not to any specific DOM element within that component.
Your Typeahead
component is a custom Svelte component, and so filterValueInputRef
refers to the component instance, not the underlying input element. That's why you're seeing the error: the component instance doesn't have a .focus()
method.
To solve this, you have a couple of options:
Expose a Focus Method in the Custom Component: Modify your
Typeahead
component to expose a method that focuses the input element. Inside this method, you can call.focus()
on the actual input element.For example, in
Typeahead.svelte
:<script> let inputElement; // Expose a method to focus the input export function focusInput() { inputElement.focus(); } </script> <input bind:this={inputElement} ... />
Then in your parent component:
onMount(() => { if (filterValueInputRef) { filterValueInputRef.focusInput(); } });
Forwarding a Focus Call: Another approach, which is more encapsulated, is to use the
forwardEvents
function from Svelte to forward a focus call to the input element inside yourTypeahead
component. This way, you can call.focus()
onfilterValueInputRef
as if it were a regular DOM element. However, this requires specific handling inside theTypeahead
component to properly forward the focus event to the input element.
If neither of these options is feasible due to the Typeahead
component being a third-party component that you cannot modify, you might need to reconsider the approach or check if the component's API offers any built-in methods for focusing. Third-party components often have their way of handling such requirements, and it might be documented in the component's API.Typeahead forwards on:focus events, so how would I trigger that?If the Typeahead
component forwards on:focus
events, it means it can emit a focus event, but that's slightly different from what you need. You want to programmatically focus the input element within the Typeahead
component, but merely having the component forward focus events doesn't provide a method to trigger focus on the component's internal input element.
To achieve what you're aiming for, you'll need a way to programmatically call a focus method on the Typeahead
component, which would then focus its internal input element. This capability depends on whether Typeahead
exposes such a method. If it does, you can call this method through the component instance reference obtained via bind:this
.
Here's how you can do it:
Check the Typeahead Component API: Look into the documentation or source code of the
Typeahead
component to see if it exposes a method to programmatically set focus on its internal input element. This method might be named something likefocus
,focusInput
, etc.Using the Exposed Method: If such a method exists, you can call it in the
onMount
function of your parent component. For example:<script> import Typeahead from '...'; import { onMount } from 'svelte'; let filterValueInputRef; onMount(() => { if (filterValueInputRef && filterValueInputRef.focusInput) { filterValueInputRef.focusInput(); // Assuming 'focusInput' is the method name } }); </script> <Typeahead bind:this={filterValueInputRef} ... />
If the Typeahead
component does not expose such a method, you have limited options. You might need to:
- Request this feature from the maintainers of the component if it's third-party.
- Modify the component yourself if it's open-source and you are able to do so.
- Use a different component or implement your own typeahead functionality that meets your requirements.
Remember, the ability to programmatically focus an internal element of a custom component depends largely on how the component is designed and what methods it exposes for external use.I have a UX where the user can choose from a large list of values. It would be good for them to be able to start typing and it only shows relevant values to choose from. What would this UI component be called?The UI component you're describing is commonly known as a "Typeahead" or "Autocomplete". This component allows users to start typing in an input field, and as they type, it dynamically filters and displays a list of options that match the entered text. This makes it easier for users to find and select from a large set of values.
Key features of a Typeahead/Autocomplete component often include:
Dynamic Filtering: As the user types, the list of options is filtered to only show relevant entries based on the input.
Dropdown List of Suggestions: Matches are shown in a dropdown list from which the user can make a selection.
Keyboard Navigation: Users can navigate through the list of suggestions using the keyboard (using arrow keys, for instance).
Immediate Feedback: The list updates in real-time as the user types, providing immediate feedback.
This component is particularly useful in cases where the user needs to select from a large number of options, like choosing a country from a global list, selecting a product in an e-commerce catalog, or auto-completing a search term.When I put flex on my div, why does it change the height of the items iside?When you apply display: flex
to a container, it changes the layout behavior of its child elements (flex items) in several ways, including their height. Here are a few key points to understand why and how this happens:
Flex Direction: By default, a flex container has
flex-direction: row
, which means the flex items are laid out horizontally. The height of the flex items in this case can change based on several factors:Align Items: The default value of
align-items
in a flex container isstretch
. This means that if the flex items don't have a specified height, they will stretch to fill the height of the container. If your container has a specific height, or if it is determined by another item inside it, this can cause the child items to stretch to match this height.Content Size: If the flex items have content with a specific height, or if they have height set through CSS, they will maintain that height unless overridden by other flex properties.
Flex Wrap and Overflow: If
flex-wrap
is set tonowrap
(the default), all flex items are forced into a single line, which can affect their height if the container doesn't have enough space to accommodate the content naturally.Cross-Axis Alignment: The cross-axis in a row-direction flex container is the vertical axis. Properties like
align-items
oralign-self
on flex items control how items are aligned along this axis, which can affect their height.
To troubleshoot or control the height of flex items, consider the following:
- Explicitly set the height of flex items if a specific height is needed.
- Adjust
align-items
on the flex container. For example, setting it toflex-start
will align items to the start of the container without stretching them. - Use
align-self
on individual flex items if you want to control their alignment independently of others. - Check if the container's height (or the height of another flex item) is influencing the rest of the items.
Understanding how these properties interact in a flexbox layout is crucial to controlling the layout effectively. Flexbox is powerful, but it requires a good grasp of its properties to predict and control how elements behave within the flex container.What would be a good symbol or icon to represent the concept of a GROUP BYChoosing a symbol or icon to represent the concept of "GROUP BY", often used in database queries or data analysis to aggregate data based on certain fields, can be a bit abstract. However, you can use visuals that convey the idea of grouping, collecting, or organizing data. Here are a few ideas:
Cluster of Dots or Bars: A group of dots or bars of varying heights, clustered together, can symbolize data being grouped. This is reminiscent of a bar chart where data is categorized and visualized.
Stacked Layers: Layers of squares or circles stacked on top of each other, possibly with a number indicating the count. This represents data being aggregated in layers or groups.
Pie Chart or Segment: A pie chart or a single segment of it can represent the idea of breaking down data into parts or groups.
Folder or File Group: A folder icon with multiple files partially behind it suggests grouping or categorizing information into a single collective unit.
Linking Chains or Rings: Interlinked chains or rings can symbolize different elements being linked together into groups.
Magnifying Glass Over Data Points: A magnifying glass focusing on a group of data points can convey the idea of examining or grouping data.
Venn Diagram: Overlapping circles like in a Venn diagram, indicating a grouping based on shared characteristics.
Remember, the effectiveness of an icon depends on the context in which it's used and the familiarity of the target audience with the concept it represents. It's also important to ensure that the icon fits well with the overall design and aesthetic of the application or interface where it's being used.
Dropdown Button Component created at Tue Jan 20 1970 16:35:03 GMT+0000 (Coordinated Universal Time)Related Chats
Passing inputValue to slot 0.565
Svelte Click Callback Structure 0.554
Svelte Component Help 0.537
Client-Only Component in SvelteKit 0.533
Tab key not firing events 0.530
Svelte: Force New Component 0.508
Test Svelte components with Jest. 0.493