iOS Local Communication Options
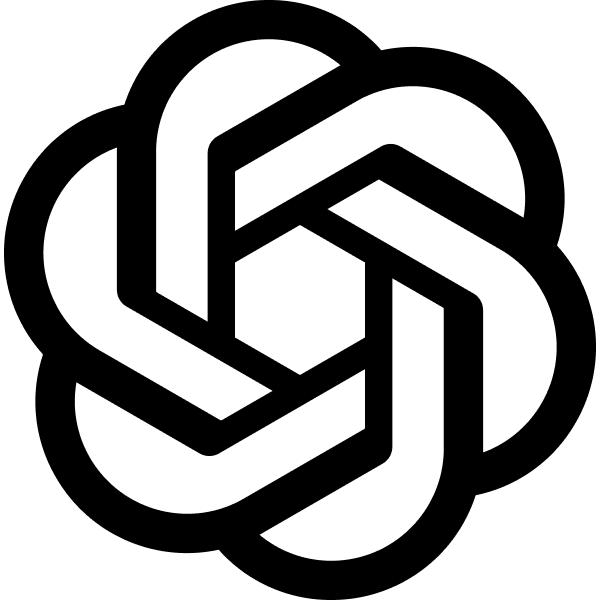
TODO: collapasable, copyable, download, etc
I'm going to write an iOS app that a user would install on two different iOS devices that were their personal devices.
What is the best apple technology for communication on a local network or in close proximity between two iOS devices available to 3rd party app developers?For communication on a local network or in close proximity between two iOS devices, Apple provides several technologies that are available to third-party app developers. The best options include:
Multipeer Connectivity Framework:
- Overview: This framework allows for the discovery of nearby devices and the establishment of peer-to-peer connections. It supports communication over Bluetooth, Wi-Fi, and peer-to-peer Wi-Fi.
- Use Cases: Ideal for apps that require local device communication without an internet connection. It can be used for sharing files, real-time collaboration, and multiplayer gaming.
- Documentation: Multipeer Connectivity Framework
Network Framework:
- Overview: This framework provides APIs for network connections, including local network and internet connections. It can be used to set up and manage connections over TCP/IP and UDP.
- Use Cases: Useful for more advanced networking needs, such as creating custom network protocols or establishing direct connections between devices on the same local network.
- Documentation: Network Framework
Bonjour:
- Overview: Bonjour is Apple's implementation of zero-configuration networking (zeroconf), which allows devices to discover each other and establish connections on a local network without requiring configuration.
- Use Cases: Suitable for service discovery and establishing local network connections for services like file sharing and media streaming.
- Documentation: Bonjour Overview
Core Bluetooth:
- Overview: This framework provides APIs for communicating with Bluetooth Low Energy (BLE) devices. It can be used to discover, connect to, and interact with BLE peripherals.
- Use Cases: Best for communication with BLE devices or when low energy consumption is a priority.
- Documentation: Core Bluetooth
Recommendation
For most peer-to-peer communication scenarios between two iOS devices that are in close proximity and do not require internet connectivity, the Multipeer Connectivity Framework is typically the best choice. It is specifically designed for this purpose, supports multiple communication methods (Bluetooth, Wi-Fi, and peer-to-peer Wi-Fi), and provides a higher-level API that simplifies the development process.
If you need more advanced network configuration or communication over a local network with more control, consider using the Network Framework or Bonjour.
Example of Using Multipeer Connectivity Framework
Here’s a simple example of how to set up a basic Multipeer Connectivity session:
import MultipeerConnectivity
class MyMultipeerSession: NSObject, MCSessionDelegate, MCBrowserViewControllerDelegate, MCNearbyServiceAdvertiserDelegate {
var peerID: MCPeerID!
var session: MCSession!
var advertiser: MCNearbyServiceAdvertiser!
var browser: MCBrowserViewController!
override init() {
super.init()
// Create a peer ID with a unique identifier
peerID = MCPeerID(displayName: UIDevice.current.name)
// Create a session
session = MCSession(peer: peerID, securityIdentity: nil, encryptionPreference: .required)
session.delegate = self
// Create an advertiser to advertise this device
advertiser = MCNearbyServiceAdvertiser(peer: peerID, discoveryInfo: nil, serviceType: "my-app")
advertiser.delegate = self
advertiser.startAdvertisingPeer()
// Create a browser to search for nearby devices
browser = MCBrowserViewController(serviceType: "my-app", session: session)
browser.delegate = self
}
// Implement required MCSessionDelegate methods
func session(_ session: MCSession, peer peerID: MCPeerID, didChange state: MCSessionState) {
// Handle state changes
}
func session(_ session: MCSession, didReceive data: Data, fromPeer peerID: MCPeerID) {
// Handle received data
}
// Implement other required methods for MCSessionDelegate, MCBrowserViewControllerDelegate, and MCNearbyServiceAdvertiserDelegate...
}
This is a starting point, and you would need to implement additional delegate methods and handle the various states and data exchanges according to your app's requirements.
iOS Local Communication Options created at Tue Jan 20 1970 20:41:14 GMT+0000 (Coordinated Universal Time)Related Chats
SwApp para Compartir Datos 0.479
SwiftUI RealityKit Integration 0.433
VR Controller iOS App 0.399
Developing Apple Pencil iPad App 0.390
Thread-Safe Multipeer Manager. 0.370
Xcode Performance Tips 0.358
Designing a Chat Event System 0.356