Developing Apple Pencil iPad App
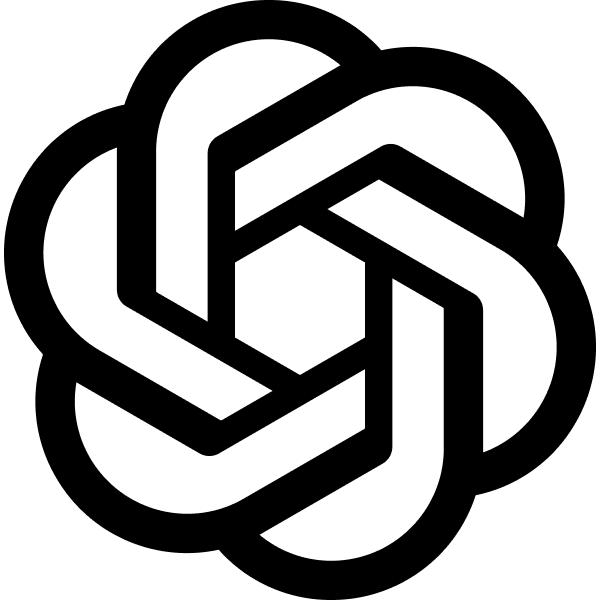
TODO: collapasable, copyable, download, etc
How do you make an iPad app that takes advantage of the Apple pencil?Creating an iPad app that leverages the capabilities of the Apple Pencil involves several steps, from conceptualization to development, using Apple's frameworks and tools. Here's an overview of the process:
1. Conceptualization and Design
- Define the App's Purpose: Determine how the Apple Pencil will enhance the user experience. Whether it's for drawing, note-taking, or another purpose, understanding the primary function will guide your development.
- Sketch the UI/UX: Design your app's interface with the Apple Pencil in mind. Consider how users will interact with your app using the Pencil and what features will be available.
2. Setup Your Development Environment
- Xcode: Install the latest version of Xcode on your Mac, as it includes all the necessary tools and frameworks to develop iOS apps.
- iPadOS SDK: Ensure you have the latest iPadOS SDK in Xcode, which provides access to the latest APIs for Apple Pencil.
3. Understand the Apple Pencil APIs
- PencilKit: Introduced in iOS 13, PencilKit provides a drawing environment that automatically responds to Apple Pencil input. It includes a canvas (PKCanvasView) and tools like pens, pencils, and erasers.
- UIKit: Look into
UIResponder
andUITouch
classes for handling touch and stylus input. These allow you to detect the presence of the Apple Pencil, its pressure, tilt, and other properties.
4. Implement Pencil-Specific Features
- Pressure Sensitivity: Use pressure data to change the thickness or opacity of strokes.
- Tilt and Orientation: Adjust the shape or texture of strokes based on the pencil's angle.
- Palm Rejection: Ensure your app can distinguish between intentional strokes and resting palms.
5. Optimize Your App for the Apple Pencil
- Latency: Minimize input latency to make drawing or writing feel responsive.
- Precision: Take advantage of the Apple Pencil's precision for detailed work.
- Gesture Support: Incorporate double-tap gestures available on the second-generation Apple Pencil for quick tool changes or other actions.
6. Test on Real Devices
- Device Testing: Test your app on actual iPad models that support the Apple Pencil to understand how well it performs and to fine-tune the user experience.
- User Testing: Get feedback from users to see how they use the Apple Pencil with your app and make adjustments based on their input.
7. Finalize and Publish
- App Store Guidelines: Review the App Store guidelines to ensure your app complies before submitting it for review.
- Marketing: Highlight how your app takes advantage of the Apple Pencil in your marketing materials to attract users looking for apps that leverage this tool.
Resources
- Apple's Developer Documentation: Provides comprehensive guides, API references, and tutorials.
- Sample Code: Look for Apple's sample projects that demonstrate how to implement specific functionalities for the Apple Pencil.
By following these steps and utilizing the available resources, you can create an iPad app that fully takes advantage of the unique features of the Apple Pencil, offering users a rich and engaging experience.Can you give me some sample code that uses the pencil sdk?Certainly! Below is a simple example of how to integrate PencilKit into an iPad app to create a drawing canvas that supports Apple Pencil input. This example demonstrates setting up a basic drawing environment using PencilKit's PKCanvasView
and PKToolPicker
.
Prerequisites
- Make sure you have the latest version of Xcode installed.
- Create a new iPad app project in Xcode.
Step 1: Import PencilKit
First, import the PencilKit framework in your ViewController file.
import UIKit
import PencilKit
Step 2: Add a Canvas View
Declare a PKCanvasView
in your ViewController to serve as the drawing area.
class ViewController: UIViewController {
var canvasView: PKCanvasView!
override func viewDidLoad() {
super.viewDidLoad()
// Initialize the canvas view and add it to the view hierarchy
canvasView = PKCanvasView(frame: self.view.bounds)
canvasView.backgroundColor = .white
view.addSubview(canvasView)
// Enable drawing using the Apple Pencil and fingers
canvasView.allowsFingerDrawing = true
// Set up the tool picker
if let window = view.window, let toolPicker = PKToolPicker.shared(for: window) {
toolPicker.setVisible(true, forFirstResponder: canvasView)
toolPicker.addObserver(canvasView)
canvasView.becomeFirstResponder()
}
}
override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()
canvasView.frame = view.bounds
}
}
Step 3: Configure the Tool Picker
The PKToolPicker
provides a selection of tools like pens, pencils, and erasers. The example configures the tool picker to be visible and associates it with the canvas view.
Step 4: Allow Finger Drawing (Optional)
The allowsFingerDrawing
property enables users to draw on the canvas with their fingers in addition to using the Apple Pencil. You can set this to false
if you want to restrict drawing to the Apple Pencil only.
Running the App
Build and run your app on an iPad that supports the Apple Pencil. You should see a white canvas where you can draw with the Apple Pencil or your fingers. The tool picker at the bottom allows you to choose different drawing tools and colors.
This example provides a basic setup. Depending on your app's requirements, you might want to explore additional PencilKit features, such as saving and loading drawings, handling undo and redo operations, or customizing the drawing tools.
Remember to test your app on a real device to fully experience the Apple Pencil's capabilities, as simulators might not fully replicate the drawing experience.What APIs are being used from PencilKit?In the sample code provided for integrating PencilKit into an iPad app, two main components from the PencilKit framework are being used:
1. PKCanvasView
- Purpose:
PKCanvasView
is a view that you can add to your app's interface to provide a drawing area. It automatically handles input from the Apple Pencil, fingers, and other styluses to create and display drawings. The canvas view supports various stylus properties like pressure, tilt, and azimuth, making it ideal for creating detailed artwork or handwriting input. - API Usage: The sample code initializes a
PKCanvasView
, sets its frame to match the bounds of the view controller's view, and adds it to the view hierarchy. It also sets the background color of the canvas view to white and allows finger drawing with theallowsFingerDrawing
property.
2. PKToolPicker
- Purpose:
PKToolPicker
is a floating palette that provides users with a selection of drawing tools, including different types of pens, pencils, and an eraser. Users can choose colors and adjust the thickness of the drawing tools. The tool picker is designed to automatically work withPKCanvasView
and provides a user-friendly interface for selecting drawing tools. - API Usage: In the sample code, the
PKToolPicker
is configured to appear when thePKCanvasView
becomes the first responder. The tool picker is made visible for the canvas view's window, and the canvas view itself is registered as an observer to the tool picker. This setup ensures that the tool picker appears over the canvas view and that the selected tools and colors are applied to the user's drawings.
These components are part of the PencilKit framework, which is designed to simplify the implementation of drawing and handwriting features in apps, leveraging the precision and versatility of the Apple Pencil. The framework's integration allows developers to provide rich drawing experiences with minimal code, making it an excellent choice for apps that require drawing or note-taking capabilities.
Developing Apple Pencil iPad App created at Tue Jan 20 1970 18:12:57 GMT+0000 (Coordinated Universal Time)Related Chats
Build Mac App in Xcode 0.549
Swift iOS Dev Guide 0.489
Reusable SwiftUI custom view. 0.447
Preload Assets in visionOS 0.443
Lightsaber Trainer App 0.441
SwiftUI RealityKit Integration 0.439
Handling App Exit 0.433