JS Optional Chaining Operator
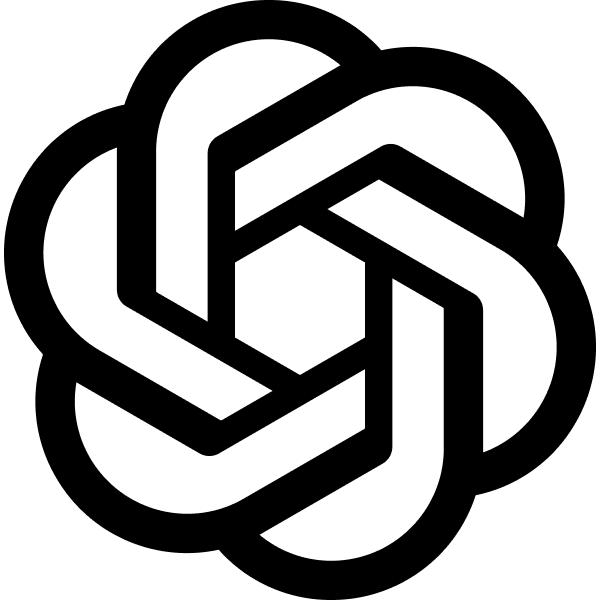
TODO: collapasable, copyable, download, etc
In client side JS in sveltekit, how does ?
work? Like if I access foo?.bar what is actually happening?In client-side JavaScript, including when working with frameworks like SvelteKit, the ?
operator used in expressions like foo?.bar
is called the optional chaining operator. This operator provides a way to simplify accessing values through connected objects when there's a possibility that a reference or function may be undefined
or null
.
Here's what happens when you use foo?.bar
:
Checking for
undefined
ornull
: The operator checks iffoo
is eitherundefined
ornull
.Accessing Property:
- If
foo
is neitherundefined
nornull
, it behaves like the normal dot notation (foo.bar
), and accesses thebar
property offoo
. - If
foo
isundefined
ornull
, it stops the evaluation and returnsundefined
. This prevents any further execution which would normally result in a TypeError (if you try to access a property ofundefined
ornull
).
- If
Benefits of Optional Chaining:
- Prevents Errors: Helps in preventing runtime errors when trying to access a property or method of something that might not exist.
- Simplifies Code: Reduces the need for repetitive and verbose checks to see if an object or its sub-properties exist.
Usage Scenarios:
- Deeply Nested Objects: Very useful for deeply nested objects where you're unsure if a certain sub-property exists.
- Conditional Calls: Can be used for conditionally calling a function. For example,
foo?.bar()
will callbar
only iffoo
is an object andbar
is a function.
Syntax Variations:
- Properties:
obj?.prop
– Accessesprop
ifobj
exists. - Array Elements:
arr?.[index]
– Accesses the index ofarr
ifarr
exists. - Function Calls:
func?.(...args)
– Callsfunc
with arguments iffunc
exists.
Example:
const object = {
a: {
b: {
c: 'Hello'
}
}
};
console.log(object.a?.b?.c); // 'Hello'
console.log(object.x?.y?.z); // undefined (instead of throwing an error)
In SvelteKit, this operator behaves the same as in any other JavaScript environment, and it's particularly useful in client-side scripts where the data structure may not be fully predictable.Is there a compiler step? How would it transform this code console.log(foo?.bar);
In SvelteKit, as in most modern JavaScript frameworks, there is indeed a build step that often involves a compilation or transformation process. This process is handled by tools like Vite, Rollup, or Webpack, often integrated into these frameworks. The purpose of this step is to transform the modern JavaScript code (which might include ES6+ features like optional chaining) into a version that is compatible with a wider range of browsers, especially older ones that don't support such features natively.
When you use a statement like console.log(foo?.bar);
in your SvelteKit project, the build tool involved will typically transpile this code into something that older JavaScript engines can understand. The optional chaining operator (?.
) is a more recent addition to the JavaScript language, and not all browsers (especially older versions) support it.
The transformation of console.log(foo?.bar);
by a transpiler like Babel would typically look something like this:
console.log(foo === null || foo === void 0 ? void 0 : foo.bar);
Here's what this transformed code does:
foo === null || foo === void 0 ? void 0 : foo.bar
: This is a ternary operator that acts as a fallback mechanism. It checks iffoo
is eithernull
orundefined
(void 0
is a compact way to writeundefined
).- If
foo
isnull
orundefined
, the expression evaluates toundefined
, mimicking the behavior of the optional chaining. - If
foo
is notnull
orundefined
, it safely accessesfoo.bar
.
This transformation ensures that the code behaves as expected, even on browsers that do not support the optional chaining operator natively. It's a part of the broader effort in modern web development to write code using the latest features for developer convenience and efficiency, while still ensuring compatibility with a wide range of user environments.so at runtime, would consolelog run? What would it log when foo is undefined?Yes, the console.log
statement will still run at runtime, even after the transpilation of the optional chaining syntax. When foo
is undefined
, the behavior of the transpiled code will be identical to the original code using optional chaining.
In the original code:
console.log(foo?.bar);
And in the transpiled code:
console.log(foo === null || foo === void 0 ? void 0 : foo.bar);
In both cases, if foo
is undefined
, the expression will evaluate to undefined
, and this is what will be logged to the console. The optional chaining operator ?.
and its transpiled equivalent using the ternary operator both ensure that if the left-hand operand (foo
) is null
or undefined
, the entire expression short-circuits and evaluates to undefined
without throwing an error.
This behavior is particularly useful for avoiding runtime errors that would occur if you attempted to access a property of undefined
or null
in JavaScript. For instance, if foo
were undefined
, attempting to access foo.bar
without optional chaining would result in a TypeError
(cannot read property 'bar' of undefined). However, with optional chaining or its transpiled equivalent, it safely evaluates to undefined
.Is ?
supported natively in modern web browsers?Yes, the optional chaining operator (?.
) is supported natively in most modern web browsers. This feature was introduced in the ECMAScript 2020 (ES11) specification, and since then, it has been widely adopted by the major browser engines like Chrome, Firefox, Safari, and Edge.
As of my last update in April 2023, here is the support status for optional chaining in major browsers:
- Google Chrome: Supported since Chrome 80.
- Mozilla Firefox: Supported since Firefox 74.
- Apple Safari: Supported since Safari 13.1.
- Microsoft Edge: Supported since Edge 80.
This means that in the latest versions of these browsers, you can use the ?.
operator directly in your JavaScript code without needing to transpile it for compatibility reasons. However, if you are developing applications that need to support older browsers or versions, you might still need to transpile this feature using a tool like Babel.
For the most current information about browser compatibility, you can always check resources like Can I Use, which provides up-to-date data on web technologies and their support across different browsers and versions.How would you re-write this test to use a Promise instead?
it('acts as a svelte store', (done) => {
// Failure of this test would be a timeout as done is nver called
unhandledClickStore.registerCallback(() => done());
unhandledClickStore.triggerCallbacks();
});
});```To rewrite the test to use a Promise instead of the `done` callback, you can return a Promise from the test function and resolve it when the desired condition is met. This approach is often cleaner and can be easier to read, especially in cases where you're dealing with asynchronous operations.
Here's how you can rewrite your test with a Promise:
```javascript
describe('unhandled clicks store', () => {
it('acts as a svelte store', () => {
return new Promise((resolve, reject) => {
// Set a timeout to reject the promise if it takes too long
const timeoutId = setTimeout(() => {
reject(new Error('Test timed out'));
}, 5000); // Adjust the timeout duration as needed
// Register the callback that resolves the promise
unhandledClickStore.registerCallback(() => {
clearTimeout(timeoutId); // Clear the timeout upon successful callback
resolve();
});
// Trigger the callbacks
unhandledClickStore.triggerCallbacks();
});
});
});
In this rewritten test:
- We return a
Promise
from the test function. - Inside the Promise, we set up a timeout that will reject the Promise if the callback isn't called within a specified time frame. This acts as a fail-safe in case the callback is never triggered, preventing the test from hanging indefinitely.
- We then register a callback with
unhandledClickStore
that will resolve the Promise when called. - Finally, we trigger the callbacks by calling
unhandledClickStore.triggerCallbacks()
.
This way, the test will pass if the callback is called (resolving the Promise), and it will fail if the timeout is reached first (rejecting the Promise). This approach aligns with modern practices of handling asynchronous operations in JavaScript.jest or whatever will timeout and fail tests, so do we really need the setTimeout?You're correct. In a testing environment like Jest, which has built-in timeout handling for asynchronous tests, the explicit use of setTimeout
to enforce a test timeout is often unnecessary. Jest will automatically fail a test if it takes longer than a specified timeout duration (default is usually 5 seconds, but it can be configured).
So, considering Jest's built-in timeout, you can simplify the test to just return a Promise that resolves when the condition is met. Here's the revised version without the setTimeout
:
describe('unhandled clicks store', () => {
it('acts as a svelte store', () => {
return new Promise((resolve) => {
// Register the callback that resolves the promise
unhandledClickStore.registerCallback(() => {
resolve();
});
// Trigger the callbacks
unhandledClickStore.triggerCallbacks();
});
});
});
In this version:
- The test returns a
Promise
. - The
Promise
is resolved when the callback passed tounhandledClickStore.registerCallback
is executed. - If
unhandledClickStore.triggerCallbacks()
correctly triggers the callback, the test will pass. - If the callback isn't triggered within Jest's default timeout period, the test will automatically fail, indicating an issue in the trigger mechanism or the callback registration.
This approach is cleaner and leverages Jest's own handling of asynchronous tests and timeouts, making your test code simpler and more focused on the actual behavior you're trying to verify.
JS Optional Chaining Operator created at Tue Jan 20 1970 18:00:57 GMT+0000 (Coordinated Universal Time)Related Chats
JS Optional Chaining Operator 0.999
Nullish Coalescing Operator Summary 0.543
Check for Not Null 0.532
Tab key not firing events 0.491
Passing inputValue to slot 0.473
Svelte Component Help 0.460
Detecting SvelteKit Environment: Logging 0.454
SvelteKit: Handling require in Client 0.440
Update Svelte Store Object 0.430
Cleaned-Up Svelte Code 0.429