Tab key not firing events
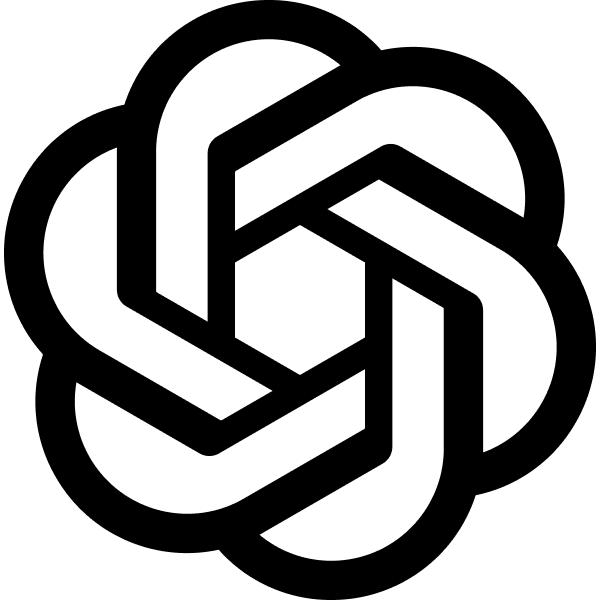
TODO: collapasable, copyable, download, etc
I have an input in Svelte with on:blur and on:keydown handlers. I am seeing that when I press the Tab key, my on:blur function is being invoked, but not my on:keydown... how is that possible?In Svelte, as in other JavaScript frameworks, different events can behave differently based on the context and the way the browser handles them. Here's an explanation of why you might be experiencing this behavior with the on:blur
and on:keydown
event handlers:
on:blur Event: The
on:blur
event is fired when an element loses focus. Pressing the Tab key is a common way to move focus from one element to another, so when you press Tab, the current input field loses focus, triggering theon:blur
event.on:keydown Event: The
on:keydown
event is fired when a key on the keyboard is pressed. However, the behavior of this event can be somewhat nuanced depending on the browser and the specific key being pressed. In many cases, certain keys like Tab are handled specially by the browser for accessibility and navigation purposes. The browser might not propagate thekeydown
event for these keys in the same way it does for other keys. This could explain why theon:keydown
event isn't firing for the Tab key.Event Propagation and Default Actions: The Tab key is often used by browsers for navigation (to move focus between elements). The browser's default action for the Tab key might be taking precedence over your JavaScript event handler. In some cases, the default action of a key can prevent certain events from being fired or from propagating in the expected manner.
Browser and Accessibility Features: Browsers have built-in accessibility features that might handle keys like Tab differently to ensure a better experience for users who rely on keyboard navigation.
If you want to capture the Tab key in the on:keydown
event, you might need to use event.preventDefault()
to stop the browser's default action. However, be cautious with this approach, as it can interfere with standard accessibility features of the browser.
Here's a basic example of how you could try to capture the Tab key in Svelte:
<script>
function handleKeydown(event) {
if (event.key === 'Tab') {
event.preventDefault();
// Your logic here
console.log('Tab key pressed');
}
}
</script>
<input type="text" on:blur="{() => console.log('Blur event')}" on:keydown="{handleKeydown}">
Remember that overriding default browser behavior should be done judiciously and with consideration for accessibility and user experience.handleKeydown never fires, so how could I prevent defaul?If the handleKeydown
function is not firing when the Tab key is pressed, it suggests that the event is not being captured by your on:keydown
handler. This could be due to the way browsers handle certain control keys, like Tab, for accessibility and navigation purposes. The browser might be handling the Tab key at a higher level, not allowing it to trigger keydown
events in the usual way.
To address this issue, you can try a few different approaches:
Use
on:keyup
Instead ofon:keydown
: Sometimes switching to thekeyup
event can help capture keys that don't triggerkeydown
events due to default browser behaviors.<script> function handleKeyup(event) { if (event.key === 'Tab') { console.log('Tab key released'); } } </script> <input type="text" on:blur="{() => console.log('Blur event')}" on:keyup="{handleKeyup}">
Focus Management: If your goal is to manage focus (like implementing custom tab navigation), you might need to approach this differently. For example, you can use a focus management library or write custom logic to manage the focus of elements on your page.
Global Event Listener: If capturing the Tab key is crucial, you could add a global event listener to the document. This is a more advanced technique and should be used with caution:
<script> function handleGlobalKeydown(event) { if (event.key === 'Tab') { console.log('Tab key pressed'); // Prevent default if necessary // event.preventDefault(); } } // Add global event listener window.addEventListener('keydown', handleGlobalKeydown); // Remember to remove the event listener when the component is destroyed onDestroy(() => { window.removeEventListener('keydown', handleGlobalKeydown); }); </script>
Rethink the Requirement: If the need to capture the Tab key is related to a specific feature or user experience, consider whether there's an alternative approach that doesn't require capturing a key that's commonly used for accessibility and navigation.
Remember, overriding default browser behaviors, especially those related to navigation and accessibility, should be done carefully. It's important to ensure that any changes you make don't negatively impact the accessibility of your application.If the handleKeydown
function isn't firing at all when the Tab key is pressed, then preventing the default action via event.preventDefault()
within that handler isn't going to work. This situation suggests that the event isn't reaching your handler due to the way the browser handles the Tab key by default for accessibility and navigation purposes.
Here are a few approaches you could consider:
Using a Different Event: Instead of
on:keydown
, you might want to try using theon:keyup
event. Sometimes, thekeyup
event can capture keys thatkeydown
does not, although this is less common.Global Event Listener: If you absolutely need to capture the Tab key press on a specific input, you might consider setting up a global event listener. However, this is a more complex solution and should be used carefully to avoid unintended side effects.
Rethink the User Interaction: If the goal is to perform an action when the user navigates away from an input field, relying on the Tab key might not be the best approach, as it can interfere with standard browser behavior and accessibility. Consider if there are other events or triggers that could achieve your goal without relying on capturing the Tab key.
Custom Tab Handling: If capturing the Tab key is essential for your application, you might consider implementing a custom tabbing mechanism within your application. This would involve manually managing focus between elements, which can be complex and should be done with careful attention to accessibility.
Debugging: Ensure that your
handleKeydown
function is correctly set up and that there aren't other issues in your code or your Svelte setup that might be preventing the event from firing.
Remember, overriding browser default behavior, especially for key navigation, should be approached with caution to maintain a good user experience and ensure accessibility. If you choose to implement a custom solution, thorough testing across different browsers and devices is important.
Tab key not firing events created at Tue Jan 20 1970 18:05:22 GMT+0000 (Coordinated Universal Time)Related Chats
Svelte Component Help 0.534
Dropdown Button Component 0.530
Passing inputValue to slot 0.498
JS Optional Chaining Operator 0.491
Input Cleared On Blur 0.485
Svelte Click Callback Structure 0.485
Svelte: Force New Component 0.458