MVVM: Prefix with `$`
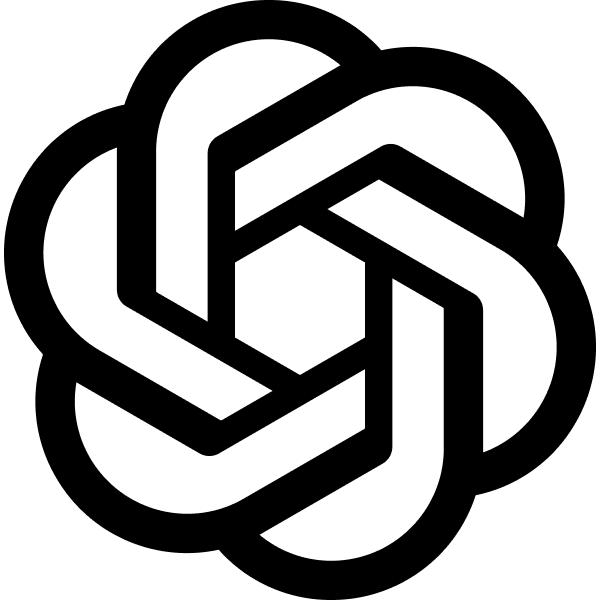
TODO: collapasable, copyable, download, etc
I'm learning mVVM. In a view, when do I prefix with $
? I see same code that is like fooViewModel.bar
and $fooViewModel.baz
Spatial computing goes even further with visionOS
updates that bring enhanced support for
volumetric apps, powerful new enterprise APIs, the
new TabletopKit framework, and more.
visionOS Highlights
TabletopKit Create collaborative experiences centered around a table. This new framework handles the manipulation of cards and pieces, establishing placement and layout, and defining game boards.
New enterprise APIs provide access to spatial barcode scanning, the Apple Neural Engine, object tracking parameters, and more.
visionOS for enterprise
Volumetric APIs • Create apps that take full advantage of depth and space — and can
run side by side with other apps in the Shared Space.
• Resize volumes by using the SwiftUI scene
modifier windowResizability.
Decide if you want the user’s hands to appear in front of or behind your digital content.
Inputs
Getting started? Explore the visionOS Pathway on developer.apple.com
• Detect planes in all orientations.
• Allow anchoring objects on surfaces in your surroundings.
• Use Room Anchors to consider the user’s surroundings on a
per-room basis.
• Attach content to individual objects around the user with the new
Object Tracking API for visionOS.
Updates to scene understanding
In SwiftUI and the Model-View-ViewModel (MVVM) pattern, you use the $
prefix to access the projected value of a property wrapper. The most common scenario is when you're dealing with @State
, @Binding
, or @ObservedObject
property wrappers. Here’s a breakdown of when and why to use the $
prefix:
@State and @Binding:
@State
is used to declare state variables within a view. These variables are managed by SwiftUI and trigger view updates when they change.@Binding
is used to pass a reference to a state variable to another view. It creates a two-way binding between the state in the parent view and the child view.
When you need to pass a
@State
variable as a@Binding
, you use the$
prefix.struct ParentView: View { @State private var isToggled: Bool = false var body: some View { Toggle("Toggle", isOn: $isToggled) } }
@ObservedObject and @EnvironmentObject:
@ObservedObject
is used to observe an object that conforms to theObservableObject
protocol. Changes to its published properties cause the view to update.@EnvironmentObject
works similarly but is used for dependency injection of observable objects across many views.
You use the
$
prefix to access the projected binding value of an@ObservedObject
.class FooViewModel: ObservableObject { @Published var bar: String = "Initial value" @Published var baz: Bool = false } struct ContentView: View { @ObservedObject var fooViewModel = FooViewModel() var body: some View { VStack { TextField("Bar", text: $fooViewModel.bar) Toggle("Baz", isOn: $fooViewModel.baz) } } }
In summary, you use $
when you need a Binding
to a state variable or a published property in an observable object. The Binding
allows for two-way data flow, ensuring that changes in the UI update the underlying state, and vice versa.
Related Chats
MVVM: Prefix with `$` 0.999
Immersive View Phases 0.802
Shared ViewModel Across Views 0.784
MultipeerManager Error Fix 0.751
SwiftUI RealityKit Integration 0.743
Preload Assets in visionOS 0.742
Handling App Exit 0.741
Switch to guard statement 0.729